Answered step by step
Verified Expert Solution
Question
1 Approved Answer
height ( ) Returns the height of the tree ( empty tree has height of 0 ) . Must run in O ( 1 )
height
Returns the height of the tree empty tree has height of
Must run in O time.
int height const
throw notimplemented;
empty
Returns true if the tree is empty.
Must run in O time.
bool empty const
throw notimplemented;
findx
Returns a pointer to the node containing x or nullptr if x is not
found in the tree.
Must run in Olog n time.
NOTE: If you want to write find recursively, you'll need to add a
private helper function
node findnode n int x;
to do the actual recursion.
node findint x
throw notimplemented;
insertx
Insert x into the tree. If x already exists in the tree, then the
tree should be left unchanged. Otherwise, if x is added to the tree,
then the tree should be rebalanced as necessary, so that the AVL height
property still holds.
Must run in Olog n time.
void insertint x
throw notimplemented;
sizen
Returns the size of the tree under the node n using recursion. This
is the recursive implementation of the size method, above.
Must run in On time. Note that because you are not allowed to add
additional private data members, you cannot use the int sz; trick to
make this O
static int sizenode root
throw notimplemented;
destroyroot
Destroy root and all its descendants.
Must run in On time, where
n sizeroot
This is called from the destructor to destroy the tree.
static void destroynode root
Your code here.
copyroot parent
Return a copy of the tree under root with root's parent
being parent
Must run in On time.
static node copynode root, node parent nullptr
Your code here.
return nullptr;
I have an AVL tree class and am not sure how to proceed with the following functions
Step by Step Solution
There are 3 Steps involved in it
Step: 1
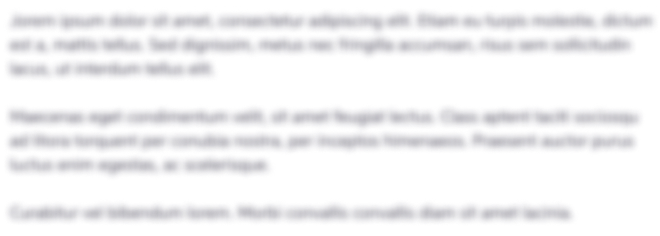
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started