Question
Hello Everyone I am having an issue debugging this assignment and I cant seem to find the error. I currently have a code with an
Hello Everyone I am having an issue debugging this assignment and I cant seem to find the error.
I currently have a code with an arrayList that works great but I have been told to redo the assignment using only an Array:
I AM NOT ALLOWED TO USE ARRAYCOPY OR Arrays.copyOf OR System.arraycopy
Instructions:
The Bashemin Parking Garage contains a single lane that can hold up to ten cars. Arriving cars enter the garage at the rear and are parked in the empty space nearest to the front. Departing cars exit only from the front.
If a customer needs to pick up a car that is not nearest to the exit, then all cars blocking its path are moved out temporarily, the customer's car is driven out, and the other cars are restored in the order they were in originally. Whenever a car departs, all cars behind it in the garage are moved up one space.
Write a Java program to operate the garage.
The program will read and process lines of input from a file until end-of-file. Each input line contains a license plate number and an operation (ARRIVE or DEPART), separated by spaces. Cars arrive and depart in the order specified by the input. Each input operation must be echo printed to an output file, along with an appropriate message showing the status of the operation.
When a car arrives, the message will include the license number and state whether the car is being parked or turned away because the garage is full. If the garage is full, the car leaves without ever having entered the garage.
When a car departs, the message will include the license number and the number of times the car was moved. The number of moves does not include the one where the car departs from the garage, or the number of times the car was moved within the garage. It is only the number of times it was moved out of the garage temporarily to allow a car behind it to depart.
If a DEPART operation calls for a car that is not in the garage, the message should so state.
My current code that works with an Arryalist:
/******** GARAGE CLASS *******/
public class Garage { | |
private Car justLeft; | |
private ArrayList Garage; | |
/** | |
* Constructor to intialize empty array list. | |
*/ | |
public Garage() | |
{ | |
Garage = new ArrayList<>() ; | |
} | |
/** | |
* Adds car to the array if there is room and turns car away if not. | |
* @param number the plate numbers for the car | |
* @return true if car object is added to array and false if list is full. | |
*/ | |
public boolean arrive(Car number) // Add a car to list if list < 10 | |
{ | |
if (Garage.size() < 10) | |
{ | |
Garage.add(number); | |
return true; | |
} | |
return false; | |
} | |
/** Check for car in garage and remove it. Pass true if car was removed. | |
* Removed car object is assigned to the variable justLeft. | |
* @param name the plate number of the car. | |
* @return true if car is found in list but false if not. | |
*/ | |
public boolean depart(String name) | |
{ | |
int position = -1; | |
// Traverse array to check for car position. | |
for (int i = 0; i < Garage.size(); i++) | |
{ | |
String matchName = Garage.get(i).getName(); //get plate number | |
if ( name.equals(matchName) ) //compare plate numbers | |
{ | |
position = i; // Get index if car is found | |
} | |
} | |
// if car is in garage then increment moves for cars ahead of it. | |
// Remove car and add its values to justLeft variable. | |
if (position != -1) | |
{ | |
for (int i=position - 1; i >= 0; i--) | |
{ | |
Garage.get(i).addMove(); | |
} | |
justLeft = Garage.remove(position); | |
return true; | |
} | |
return false; | |
} | |
/** | |
* Gets the value of the variable justLeft. | |
* @return justLeft the variable that contains the last car to leave. | |
*/ | |
public Car getLeft() | |
{ | |
return justLeft; | |
} | |
} |
/****** CORRECT OUTPUT ******************/
Car with plate number: JAV001 is arriving. Car has been parked.
Car with plate number: JAV002 is arriving. Car has been parked.
Car with plate number: JAV003 is arriving. Car has been parked.
Car with plate number: JAV004 is arriving. Car has been parked.
Car with plate number: JAV005 is arriving. Car has been parked.
Car with plate number: JAV001 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV004 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV006 is arriving. Car has been parked.
Car with plate number: JAV007 is arriving. Car has been parked.
Car with plate number: JAV008 is arriving. Car has been parked.
Car with plate number: JAV009 is arriving. Car has been parked.
Car with plate number: JAV010 is arriving. Car has been parked.
Car with plate number: JAV011 is arriving. Car has been parked.
Car with plate number: JAV012 is arriving. Car has been parked.
Car with plate number: JAV013 is arriving. No room for this car!
Car with plate number: JAV014 is arriving. No room for this car!
Car with plate number: JAV006 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV014 is departing. Car not found in Garage!
Car with plate number: JAV013 is departing. Car not found in Garage!
Car with plate number: JAV005 is departing. Car has left the garage. Car moved: 1 times.
Car with plate number: JAV015 is arriving. Car has been parked.
Car with plate number: JAV010 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV002 is departing. Car has left the garage. Car moved: 4 times.
Car with plate number: JAV015 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV014 is departing. Car not found in Garage!
Car with plate number: JAV009 is departing. Car has left the garage. Car moved: 2 times.
Car with plate number: JAV003 is departing. Car has left the garage. Car moved: 6 times.
Car with plate number: JAV008 is departing. Car has left the garage. Car moved: 3 times.
Car with plate number: JAV007 is departing. Car has left the garage. Car moved: 4 times.
Car with plate number: JAV012 is departing. Car has left the garage. Car moved: 1 times.
Car with plate number: JAV011 is departing. Car has left the garage. Car moved: 2 times.
/************* NEW GARAGE CLASS CODE USING ARRAY***************/
public class Garage {
private Car justLeft; private Car[] garage; private int carCount; public Garage() { // setting an array size of 10 garage = new Car[10]; carCount = 0; } public boolean arrive(Car number) { if (carCount < 10) { garage[carCount] = number; carCount++; return true; } return false; }
public boolean depart(String name){ int position = -1; // Traverse array to check for car position. for (int i = 0; i < carCount; i++) { String matchName = garage[i].getName(); // get plate number if (name.equals(matchName)) // compare plate numbers { position = i; // Get index if car is found } }
if (position != -1) { for (int i = position - 1; i >= 0; i--) { garage[i].addMove(); } justLeft = garage[position]; carCount--; return true; } return false; }
public Car getLeft(){ return justLeft; }
}
/************* BROKEN OUTPUT*************************/
Car with plate number: JAV001 is arriving. Car has been parked.
Car with plate number: JAV002 is arriving. Car has been parked.
Car with plate number: JAV003 is arriving. Car has been parked.
Car with plate number: JAV004 is arriving. Car has been parked.
Car with plate number: JAV005 is arriving. Car has been parked.
Car with plate number: JAV001 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV004 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV006 is arriving. Car has been parked.
Car with plate number: JAV007 is arriving. Car has been parked.
Car with plate number: JAV008 is arriving. Car has been parked.
Car with plate number: JAV009 is arriving. Car has been parked.
Car with plate number: JAV010 is arriving. Car has been parked.
Car with plate number: JAV011 is arriving. Car has been parked.
Car with plate number: JAV012 is arriving. Car has been parked.
Car with plate number: JAV013 is arriving. No room for this car!
Car with plate number: JAV014 is arriving. No room for this car!
Car with plate number: JAV006 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV014 is departing. Car not found in Garage!
Car with plate number: JAV013 is departing. Car not found in Garage!
ERROR STARTS HERE BEFORE THAT IT PRINTED CORRECT
Car with plate number: JAV005 is departing. Car not found in Garage!
Car with plate number: JAV015 is arriving. Car has been parked.
Car with plate number: JAV010 is departing. Car has left the garage. Car moved: 0 times.
Car with plate number: JAV002 is departing. Car has left the garage. Car moved: 3 times.
Car with plate number: JAV015 is departing. Car not found in Garage!
Car with plate number: JAV014 is departing. Car not found in Garage!
Car with plate number: JAV009 is departing. Car has left the garage. Car moved: 1 times.
Car with plate number: JAV003 is departing. Car has left the garage. Car moved: 4 times.
Car with plate number: JAV008 is departing. Car has left the garage. Car moved: 2 times.
Car with plate number: JAV007 is departing. Car has left the garage. Car moved: 3 times.
Car with plate number: JAV012 is departing. Car not found in Garage!
Car with plate number: JAV011 is departing. Car not found in Garage!
/******** CAR CLASS WHICH IT USES *************/
public class Car { private String name; // license plate number private int moves = 0; // number of moves car has endured public Car(String number) { name = number; } public void addMove() //increment number of moves { moves = moves + 1; } public String getName() { return name; } public int getMoves() { return moves; } }
Please help me fix this I will post any additionally needed code in the comments if needed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
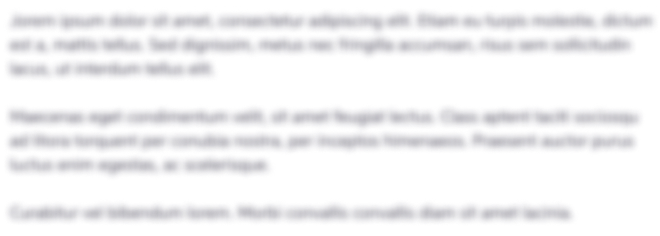
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started