Question
Hello I am working on the following assignment which is as follow **You have been tasked with developing a complete, modular, object-oriented program that will
Hello I am working on the following assignment which is as follow
**You have been tasked with developing a complete, modular, object-oriented program that will allow for the management of a collection. The scenario provided to you outlines all of the program's requirements. Refer to the provided scenario to make the determinations for all data types, algorithms and control structures, methods, and classes used in your program. Your final submission should be a self-contained, fully functional program that includes all necessary supporting classes. Furthermore, you must provide inline comments in your program design that software engineers would be able to utilize for the ongoing maintenance of your program. Your programmatic solution should also be communicated through application programming interface (API) documentation to other programmers. Specifically, the following critical elements must be addressed: I. Data Types: Your final program should properly employ each of the following data types that meet the scenario's requirements where necessary: A. Utilize numerical data types that represent quantitative values for variables and attributes in your program. B. Utilize strings that represent a sequence of characters needed as a value in your program. C. Populate a list or array that allows the management of a set of values as a single unit in your program. D. Utilize inline comments directed toward software engineers for the ongoing maintenance of your program that explain your choices of data types you selected for your program. II. Algorithms and Control Structure: Your final program should properly employ each of the following control structures as required or defined by the scenario where necessary: A. Utilize expressions or statements that carry out appropriate actions or that make appropriate changes to your program's state as represented in your program's variables. B. Employ the appropriate conditional control structures that enable choosing between options in your program. C. Utilize iterative control structures that repeat actions as needed to achieve the program's goal. D. Utilize inline comments directed toward software engineers for the ongoing maintenance of your program that explain how your use of algorithms and control structures appropriately addresses the scenario's information management problem. III. Methods: Your final program should properly employ each of the following aspects of method definition as determined by the scenario's requirements where necessary: A. Use formal parameters that provide local variables in a function's definition. B. Use actual parameters that send data as arguments in function calls. C. Create both value-returning and void functions to be parts of expressions or stand-alone statements in your program. D. Create unit tests that ensure validity of the methods. E. Invoke methods that access the services provided by an object. F. Employ user-defined methods that provide custom services for an object. G. Utilize inline comments directed toward software engineers for the ongoing maintenance of your program that explain the purpose of the methods you implemented in your program. IV. Classes: Construct classes for your program that include the following as required by the scenario where necessary: A. Include attributes that allow for encapsulation and information hiding in your program. B. Include appropriate methods that provide an object's behaviors. C. Create a driver class that instantiates objects for testing the constructed classes. D. Utilize inline comments directed toward software engineers for the ongoing maintenance of your program that explain the decisions you made in the construction of the classes in your program.**
The code I am working with is
file1
public class Milestone1ingredient {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
/*below will set variable*/
String nameOfIngredient = "";
String unitMeasurement = "";
float ingredientAmount = 0;
int numberCaloriesPerUnit = 0;
double totalCalories = 0.0;
/*below will ask the user to enter the name of ingredient*/
System.out.println("Enter the type of ingredient: ");
nameOfIngredient = scnr.nextLine();
/*to set a readable type of measurement*/
System.out.println("From the following enter the unit of measurement: ");
System.out.println("Cups");
System.out.println("Tbsp");
System.out.println("Tsp");
System.out.println("Gram");
System.out.println("Thank you for choosing between, cups, tbsp, tsp and grams");
/*below will check the validity of the entered input*/
if (scnr.hasNext()) {
unitMeasurement = scnr.next();
if (!"Gram".equals(unitMeasurement) && !"cup".equals(unitMeasurement)
&& !"tbsp".equals(unitMeasurement) && !"Tsp".equals(unitMeasurement)) {
System.out.println("Please choose from our provided list");
}
else {
System.out.println("Thank you for choosing " + unitMeasurement);
}
}
else {
System.out.println("Please choose from our provided list");
if (scnr.hasNext()) {
unitMeasurement = scnr.next();
if (!"Gram".equals(unitMeasurement) && !"Cup".equals(unitMeasurement)
&& !"Tbsp".equals(unitMeasurement) && !"Tsp".equals(unitMeasurement)) {
System.out.println("Measurement not realized. Please start over.");
}
else {
System.out.println("Thank you for choosing " + unitMeasurement);
}
}
}
/*We will set a range to ensure integrity of amounts entered*/
System.out.println("Enter amount of " + unitMeasurement + " for " + nameOfIngredient
+ " between 1 and 100: ");
if (scnr.hasNextInt()) {
ingredientAmount = scnr.nextInt();
if((ingredientAmount >= 1) && (ingredientAmount <= 100)) {
System.out.println(ingredientAmount + "is a valid amount your"
+ " recipie will require " + nameOfIngredient +
"in the amount of " + ingredientAmount + " " + unitMeasurement);
}
else {
System.out.println(ingredientAmount + "invalid");
System.out.println("Enter a number between 1 and 1000: ");
if (scnr.hasNextInt()) {
ingredientAmount = scnr.nextInt();
if ((ingredientAmount >= 1) && (ingredientAmount <= 100)) {
System.out.println(ingredientAmount + "is valid");
}
else if (ingredientAmount < 1 ) {
System.out.println(ingredientAmount + "is an invalid number please try again.");
}
else {
System.out.println(ingredientAmount + "Is too large a number, please try again");
}
}
}
}
else {
System.out.println("You've entered an invalid number please try again.");
}
System.out.println("Enter the amount of calories in one " + unitMeasurement +
" of " + nameOfIngredient + " between 1 and 1000: ");
if (scnr.hasNextInt()) {
numberCaloriesPerUnit = scnr.nextInt();
if ((numberCaloriesPerUnit >= 1) && (numberCaloriesPerUnit <= 1000)) {
System.out.println(numberCaloriesPerUnit + "is a valid amount.");
}
else {
System.out.println(numberCaloriesPerUnit + "is an invalid amount.");
System.out.println("Enter the amount of calories in " + unitMeasurement +
"between 1 and 1000: ");
if (scnr.hasNextInt()) {
numberCaloriesPerUnit = scnr.nextInt();
if((numberCaloriesPerUnit >= 1) && (numberCaloriesPerUnit <= 1000)) {
System.out.println(numberCaloriesPerUnit + "is a valid amount.");
}
else {
System.out.println(numberCaloriesPerUnit + "is invalid.");
System.out.println("Enter a valid amount of calories in one" + unitMeasurement + " between 1 and 1000: ");
if (scnr.hasNextInt()) {
numberCaloriesPerUnit = scnr.nextInt();
if ((numberCaloriesPerUnit >= 1) && (numberCaloriesPerUnit <= 1000)) {
System.out.println(numberCaloriesPerUnit + "is valid.");
}
else if (numberCaloriesPerUnit < 1 ) {
System.out.println(numberCaloriesPerUnit + " is an invalid amount. Please try again.");
}
else {
System.out.println(numberCaloriesPerUnit + "is too big a number. Please try again");
}
}
}
}
else {
System.out.println("not a number. Please try again");
}
totalCalories = ingredientAmount * numberCaloriesPerUnit;
System.out.println("Well Done!");
System.out.println("Your recipie using " + nameOfIngredient + "will use" + ingredientAmount + " " + unitMeasurement + "with a total amount of calories of " + totalCalories);
System.out.println("The Ingredient " + nameOfIngredient + "has been stored.");
}
/*above will check and calculate the total amount of calories and
successfully add the ingredient to the list*/
}
}
}
*****
file2
*****
class Recipe {
private String recipeName;
private int servings;
private List
private int totalRecipeCalories;
/*default*/
public Recipe() {
this.recipeName = "";
this.servings = 0;
this.recipeIngredients = new ArrayList
this.totalRecipeCalories = 0;
}
/*overloaded*/
public Recipe(String recipeName, int servings, List
this.recipeName = recipeName;
this.servings = servings;
this.recipeIngredients = recipeIngredients;
this.totalRecipeCalories = totalRecipeCalories;
}
/*accesor and mutator*/
public String getRecipeName() {
return recipeName;
}
public void setRecipeName(String recipeName) {
this.recipeName = recipeName;
}
public int getServings() {
return servings;
}
public void setServings(int servings) {
this.servings = servings;
}
public List
return recipeIngredients;
}
public void setRecipeIngredients(List
this.recipeIngredients = recipeIngredients;
}
public int getTotalRecipeCalories() {
return totalRecipeCalories;
}
public void setTotalRecipeCalories(int totalRecipeCalories) {
this.totalRecipeCalories = totalRecipeCalories;
}
public void printRecipe() {
int singleServingCalories = (totalRecipeCalories / servings);
System.out.println("Recipe: " + recipeName);
System.out.println("Serves: " + servings);
System.out.println("Ingredients: ");
for (int i = 0; i < recipeIngredients.size(); i++) {
String ingredient = recipeIngredients.get(i);
System.out.println(ingredient);
}
System.out.println("Each Serving Has " + singleServingCalories + " Calories.");
}
public static Recipe createNewRecipe() {
Scanner sc = new Scanner(System.in);
System.out.print("Enter recipe name: ");
String recipeName = sc.nextLine();
System.out.print("Enter number of servings: ");
int numServings = sc.nextInt();
sc.nextLine();
System.out.println("Enter all ingredients separated by commas: ");
List
System.out.println("Enter total calories of the recipe: ");
int totalCalories = sc.nextInt();
sc.nextLine();
return new Recipe(recipeName, numServings, ingredients, totalCalories);
}
}
***********
public class SteppingStone6_RecipeBox {
/**
* Declare instance variables:
* a private ArrayList of the type SteppingStone5_Recipe named listOfRecipes
*
*/
private ArrayList
/**
* Add accessor and mutator for listOfRecipes
*
*/
public void setListOfRecipes(ArrayList
this.listOfRecipes = listOfRecipes;
}
public ArrayList
return listOfRecipes;
}
/**
* Add constructors for the SteppingStone6_RecipeBox()
*
*/
public void SteppingStone6_RecipeBox() {
this.listOfRecipes = new ArrayList
}
public void SteppingStone6_RecipeBox(ArrayList
this.listOfRecipes = listOfRecipes;
}
/**
* Add the following custom methods:
*
* //printAllRecipeDetails(SteppingStone5_Recipe selectedRecipe)
* This method should accept a recipe from the listOfRecipes ArrayList
* recipeName and use the SteppingStone5_Recipe.printRecipe() method
* to print the recipe
*
* //printAllRecipeNames() <-- This method should print just the recipe
* names of each of the recipes in the listOfRecipes ArrayList
*
* //addRecipe(SteppingStone5_Recipe tmpRecipe) <-- This method should use
* the SteppingStone5_Recipe.addRecipe() method to add a new
* SteppingStone5_Recipe to the listOfRecipes
*
*/
public void printAllRecipeDetails(){
SteppingStone5_Recipe tempRecipe = new SteppingStone5_Recipe();
int i = 0;
for (i = 0; i < listOfRecipes.size(); i++){
tempRecipe.printRecipe();
}
}
public void printAllRecipeNames() {
int i = 0;
for (i = 0; i < listOfRecipes.size(); i++){
System.out.println(listOfRecipes.get(i));
}
}
/**
* A variation of following menu method should be used as the actual main
* once you are ready to submit your final application.For this
* submission and for using it to do stand-alone tests, replace the
* public void menu() with the standard
* public static main(String[] args)
* method
*
*/
public void addNewRecipe() {
SteppingStone5_Recipe tempRecipe2 = new SteppingStone5_Recipe();
tempRecipe2.addNewRecipe();
}
public void menu() {
// Create a Recipe Box
//SteppingStone6_RecipeBox myRecipeBox = new SteppingStone6_RecipeBox(); //Uncomment for main method
Scanner menuScnr = new Scanner(System.in);
/**
* Print a menu for the user to select one of the three options:
*
*/
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) {
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
int input = menuScnr.nextInt();
/**
* The code below has two variations:
* 1. Code used with the SteppingStone6_RecipeBox_tester.
* 2. Code used with the public static main() method
*
* One of the sections should be commented out depending on the use.
*/
/**
* This could should remain uncommented when using the
* SteppingStone6_RecipeBox_tester.
*
* Comment out this section when using the
* public static main() method
*/
if (input == 1) {
addNewRecipe();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
/**
* This could should be uncommented when using the
* public static main() method
*
* Comment out this section when using the
* SteppingStone6_RecipeBox_tester.
*
if (input == 1) {
myRecipeBox.newRecipe();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
myRecipesBox.printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) {
for (int j = 0; j < myRecipesBox.listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + myRecipesBox.listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:");
}
*
*/
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
}
}
}
/**
*
* Final Project Details:
*
* For your final project submission, you should add a menu item and a method
* to access the custom method you developed for the Recipe class
* based on the Stepping Stone 5 Lab.
*
*/
**********
file 4
********
private String recipeName;
private int servings;
ArrayList
private int totalRecipeCalories;
public void setRecipeName(String recipeName) {
this.recipeName = recipeName;
}
public String getRecipeName(){
return recipeName;
}
public void setServings(int servings){
this.servings = servings;
}
public int getServings(){
return servings;
}
public void setRecipeIngredients(ArrayList
this.recipeIngredients = recipeIngredients;
}
public ArrayList
return recipeIngredients;
}
public void setTotalRecipeCalories(int totalRecipeCalories){
this.totalRecipeCalories = totalRecipeCalories;
}
public int getTotalRecipeCalories(){
return totalRecipeCalories;
}
public SteppingStone5_Recipe(){
this.recipeName = "";
this.servings = 0;
this.recipeIngredients = new ArrayList<>();
this.totalRecipeCalories=0;
}
public SteppingStone5_Recipe(String recipeName, int servings,
ArrayList
{
this.recipeName = recipeName;
this.servings = servings;
this.recipeIngredients = recipeIngredients;
this.totalRecipeCalories = totalRecipeCalories;
}
public void printRecipe() {
int singleServingCalories = (totalRecipeCalories / servings);
System.out.println("Recipe: " + recipeName);
System.out.println("Serves: " + servings);
System.out.println("Ingredients: ");
for (int i = 0; i < recipeIngredients.size(); i++){
String ingredient = recipeIngredients.get(i);
System.out.println(ingredient);
}
System.out.println("one serving has " + singleServingCalories + " calories");
}
public static void main(String[] args) {
int totalRecipeCalories = 0;
ArrayList
boolean addMoreIngredients= true;
Scanner scnr = new Scanner(System.in);
System.out.println("Please enter the recipe name: ");
String recipeName = scnr.nextLine();
System.out.println("Please enter the number of servings: ");
//correct data type & Scanner assignment method for servings variable
int servings = scnr.nextInt();
do {
System.out.println("Please enter the ingredient name or type end if you are finished entering ingredients: ");
String ingredientName = scnr.next();
if (ingredientName.toLowerCase().equals("end")) {
addMoreIngredients = false;
break;
} else {
recipeIngredients.add(ingredientName);
addMoreIngredients = true;
System.out.println("Please enter the ingredient amount: ");
float ingredientAmount = scnr.nextFloat();
System.out.println("Please enter the ingredient Calories: ");
int ingredientCalories = scnr.nextInt();
totalRecipeCalories = (int) (ingredientCalories * ingredientAmount);
}
} while (addMoreIngredients == true) ;
SteppingStone5_Recipe recipe1 = new SteppingStone5_Recipe(recipeName,
servings, recipeIngredients, totalRecipeCalories);
recipe1.printRecipe();
}
}
/**
* Final Project
*
* For your Final Project:
*
* 1. Modify this code to develop a Recipe class:
* a. change the void main method createNewRecipe() that returns a Recipe
*
* 2. FOR FINAL SUBMISSION ONLY:Change the ArrayList type to an
* Ingredient object.When a user adds an ingredient to the recipe,
* instead of adding just the ingredient name, you will be adding the
* actual ingredient including name, amount, measurement type, calories.
* For the Milestone Two submission, the recipeIngredients ArrayList can
* remain as a String type.
*
* 3. Adapt the printRecipe() method to print the amount and measurement
* type as well as the ingredient name.
*
* 4. Create a custom method in the Recipe class.
*Choose one of the following options:
*
* a. print out a recipe with amounts adjusted for a different
* number of servings
*
* b. create an additional list or ArrayList that allow users to
* insert step-by-step recipe instructions
*
* c. conversion of ingredient amounts from
* English to metric (or vice versa)
*
* d. calculate select nutritional information
*
* e. calculate recipe cost
*
*f. propose a suitable alternative to your instructor
*
*/
*******
I am having immense trouble if some guidance could be available on how to get everything flowing together it would be great
Step by Step Solution
There are 3 Steps involved in it
Step: 1
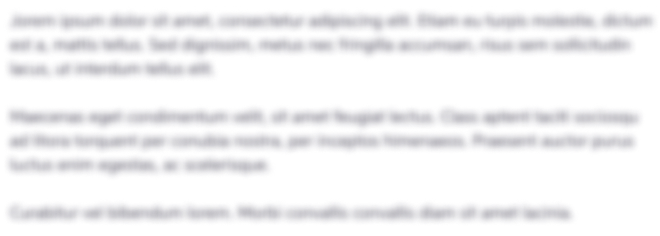
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started