Question
Hello i have an bouncing ball game in java, but i need an solution for my question to add in my code: Modify the jumper
Hello i have an bouncing ball game in java, but i need an solution for my question to add in my code:
Modify the jumper ball application so that it has a small rectangular barrier in the center of the box and the ball changes the direction of movement in contact with the barrier
My source code for 4 classes:
package orginal;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.Rectangle;
import java.util.ArrayList;
public class Obstacles {
private static final int BOX_LENGTH = 12;
private ArrayList
private WormChase wc;
public Obstacles(WormChase wc) {
this.wc = wc;
}
public synchronized void add(int x, int y) {
Rectangle box = new Rectangle(x,y,BOX_LENGTH,BOX_LENGTH);
boxes.add(box);
wc.setBoxNumbers(boxes.size());
}
public synchronized void draw(Graphics g) {
for (Rectangle box: boxes) {
g.setColor(Color.blue);
g.fillRect(box.x, box.y, box.width, box.height);
}
}
public boolean hits(Point p, int size) {
Rectangle cell = new Rectangle(p.x,p.y,size,size);
for (Rectangle box: boxes) {
if (box.intersects(cell))
return true;
}
return false;
}
public int getNumObstacles() {
return boxes.size();
}
}
package orginal;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.geom.Point2D;
public class Worm {
private static final int MAXPOINTS = 40;
private static final int DOTSIZE = 12;
private static final int RADIUS = DOTSIZE/2;
private static final int NUM_DIRS = 8;
private static final int N = 0;
private static final int NE = 1;
private static final int E = 2;
private static final int SE = 3;
private static final int S = 4;
private static final int SW = 5;
private static final int W = 6;
private static final int NW = 7;
private int curCompass;
private Obstacles obs;
private int nPoints;
private Point[] cells;
private int headPosn, tailPosn;
private int pWidth,pHeight;
private Point2D.Double[] incrs;
private static final int MAXPROBS = 9;;
private int probsForOffset[] = new int[MAXPROBS];
public Worm(int width, int height,Obstacles obs) {
this.pWidth = width;
this.pHeight = height;
this.obs = obs;
cells = new Point[MAXPOINTS];
headPosn = tailPosn = -1;
probsForOffset[0] = 0;
probsForOffset[1] = 0;
probsForOffset[2] = 0;
probsForOffset[3] = 1;
probsForOffset[4] = 1;
probsForOffset[5] = 2;
probsForOffset[6] = -1;
probsForOffset[7] = -1;
probsForOffset[8] = -2;
incrs = new Point2D.Double[NUM_DIRS];
incrs[N] = new Point2D.Double(0,-1);
incrs[NE] = new Point2D.Double(0.7,-0.7);
incrs[E] = new Point2D.Double(1,0);
incrs[SE] = new Point2D.Double(0.7,0.7);
incrs[S] = new Point2D.Double(0,1);
incrs[SW] = new Point2D.Double(-0.7,0.7);
incrs[W] = new Point2D.Double(-1,0);
incrs[NW] = new Point2D.Double(-0.7,-0.7);
}
private int varyBearing(){
int newOfsset = probsForOffset[(int) (Math.random() * MAXPROBS)];
return calcBearing(newOfsset);
}
private int calcBearing(int offset) {
int turn = curCompass + offset;
if (turn >= NUM_DIRS)
turn -= NUM_DIRS;
else if (turn < 0 )
turn += NUM_DIRS;
return turn;
}
private Point nextPoint(int prevPosn, int bearing) {
Point2D.Double incr = incrs[bearing];
int newX = cells[prevPosn].x + (int)(incr.x * DOTSIZE);
int newY = cells[prevPosn].y + (int)(incr.y * DOTSIZE);
if (newX + DOTSIZE < 0)
newX += pWidth;
else if (newX > pWidth)
newX -= pWidth;
if (newY + DOTSIZE < 0)
newY += pHeight;
else if (newY > pHeight)
newY -= pHeight;
return new Point(newX,newY);
}
private void newHead(int prevPosn) {
int newBearing = varyBearing();
Point newPt = nextPoint(prevPosn, newBearing);
int[] fixedOffs = {-2,2,-4};
if (obs.hits(newPt, DOTSIZE))
for (int num :fixedOffs ) {
newBearing = calcBearing(num);
newPt = nextPoint(prevPosn, newBearing);
if (!obs.hits(newPt, DOTSIZE))
break;
}
cells[headPosn] = newPt;
curCompass = newBearing;
}
public void move() {
int prevPosn = headPosn;
headPosn = (headPosn + 1) % MAXPOINTS;
if (nPoints == 0) {
tailPosn = headPosn;
curCompass = (int)(Math.random() * NUM_DIRS);
cells[headPosn] = new Point(pWidth/2,pHeight/2);
nPoints++;
}
else if (nPoints == MAXPOINTS) {
tailPosn = (tailPosn + 1) % MAXPOINTS;
newHead(prevPosn);
}
else {
newHead(prevPosn);
nPoints++;
}
}
public void draw(Graphics g) {
if (nPoints > 0) {
g.setColor(Color.black);
int i = tailPosn;
while (i != headPosn) {
g.fillOval(cells[i].x, cells[i].y, DOTSIZE, DOTSIZE);
i = (i + 1) % MAXPOINTS;
}
g.setColor(Color.red);
g.fillOval(cells[headPosn].x, cells[headPosn].y, DOTSIZE, DOTSIZE);
}
}
public boolean nearHead(int x, int y) {
if ( Math.abs(cells[headPosn].x + RADIUS - x ) <= DOTSIZE
&& Math.abs(cells[headPosn].y + RADIUS - y ) <= DOTSIZE )
return true;
return false;
}
public boolean touchedAt(int x, int y) {
int i = tailPosn;
while (i != headPosn) {
if ( Math.abs(cells[i].x + RADIUS - x ) <= DOTSIZE
&& Math.abs(cells[i].y + RADIUS - y ) <= DOTSIZE )
return true;
i = (i + 1) % MAXPOINTS;
}
return false;
}
}
package orginal;
import java.awt.BorderLayout;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
import javax.swing.BoxLayout;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class WormChase extends JFrame implements WindowListener {
private WormPanel gp;
private JTextField boxesTf, timeTf;
private static final int DEFAULT_FPS = 80;
public static void main(String[] args) {
int fps = DEFAULT_FPS;
if (args.length != 0)
fps = Integer.parseInt(args[0]);
long period = 1000 / fps;
new WormChase(period);
}
public WormChase(long period) {
super("Worm Chase");
makeGUI(period);
addWindowListener(this);
pack();
setResizable(false);
setVisible(true);
}
private void makeGUI(long period){
gp = new WormPanel(period,this);
boxesTf = new JTextField();
boxesTf.setText("Box: 0");
boxesTf.setEditable(false);
timeTf = new JTextField();
timeTf.setText("Time: 0 sec");
timeTf.setEditable(false);
JPanel ctrls = new JPanel();
ctrls.setLayout(new BoxLayout(ctrls,BoxLayout.X_AXIS));
ctrls.add(boxesTf);
ctrls.add(timeTf);
getContentPane().add(gp, BorderLayout.CENTER);
getContentPane().add(ctrls, BorderLayout.SOUTH);
}
public void setTimeSpent(int t){
timeTf.setText(String.format("Time: %d sec",t));
}
public void setBoxNumbers(int n) {
boxesTf.setText(String.format("Box: %d", n));
}
@Override
public void windowActivated(WindowEvent arg0) {
gp.resumeGame();
}
@Override
public void windowClosed(WindowEvent arg0) {}
@Override
public void windowClosing(WindowEvent arg0) {
gp.stopGame();
}
@Override
public void windowDeactivated(WindowEvent arg0) {
gp.pauseGame();
}
@Override
public void windowDeiconified(WindowEvent arg0) {
gp.resumeGame();
}
@Override
public void windowIconified(WindowEvent arg0) {
gp.pauseGame();
}
@Override
public void windowOpened(WindowEvent arg0) { }
}
package orginal;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Toolkit;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JPanel;
public class WormPanel extends JPanel implements Runnable {
static final int PWIDTH = 500;
static final int PHEIGHT = 400;
private volatile boolean running = false;
private volatile boolean gameOver = false;
private volatile boolean isPaused = false;
private Thread animator;
private Graphics dbg;
private Image dbImage;
private long period = 10; // 100 FPS
// ...
private WormChase wc;
private Worm fred;
private Obstacles obs;
private Font font;
private FontMetrics metrics;
private int timeSpentInGame; // sec
public WormPanel(long period, WormChase wc) {
this.period = period;
this.wc = wc;
obs = new Obstacles(wc);
fred = new Worm(PWIDTH, PHEIGHT, obs);
font = new Font("SansSerif", Font.BOLD, 24);
metrics = getFontMetrics(font);
setBackground(Color.WHITE);
setPreferredSize(new Dimension(PWIDTH, PHEIGHT));
// ...
setFocusable(true);
requestFocus();
addMouseListener(new MouseAdapter() {
@Override
public void mousePressed(MouseEvent e) {
testPress(e.getX(), e.getY());
}
});
readyForKeys();
}
private void readyForKeys() {
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if (keyCode == KeyEvent.VK_ESCAPE || keyCode == KeyEvent.VK_Q || keyCode == KeyEvent.VK_END
|| (keyCode == KeyEvent.VK_C && e.isControlDown()))
running = false;
}
});
}
private int score;
private void testPress(int x, int y) {
if (!gameOver && !isPaused) {
if (fred.nearHead(x, y)) {
gameOver = true;
score = (40 - timeSpentInGame) + (40 - obs.getNumObstacles());
} else if (!fred.touchedAt(x, y))
obs.add(x, y);
}
}
@Override
public void addNotify() {
super.addNotify();
startGame();
}
private void startGame() {
if (animator == null || !running) {
animator = new Thread(this);
animator.start();
}
}
public synchronized void stopGame() {
running = false;
notify();
}
public void pauseGame() {
isPaused = true;
}
public synchronized void resumeGame() {
isPaused = false;
notify();
}
private long gameStartTime;
private int frameCount;
private double averageFPS;
@Override
public void run() {
gameStartTime = System.currentTimeMillis();
running = true;
long beforeTime, timeDiff, sleepTime;
beforeTime = System.currentTimeMillis();
while (running) {
try {
if (isPaused) {
synchronized (this) {
while (running && isPaused)
wait();
}
}
} catch (InterruptedException e) {
}
gameUpdate();
gameRender();
paintScreen();
timeDiff = System.currentTimeMillis() - beforeTime;
sleepTime = period - timeDiff;
if (sleepTime < 0)
sleepTime = 5;
try {
Thread.sleep(sleepTime);
} catch (InterruptedException e) {
}
beforeTime = System.currentTimeMillis();
storeStats();
}
// printStats();
System.exit(0);
}
private void storeStats() {
frameCount++;
long timeNow = System.currentTimeMillis();
timeSpentInGame = (int) ((timeNow - gameStartTime) / 1000);
if (!gameOver)
wc.setTimeSpent(timeSpentInGame);
averageFPS = frameCount / ((timeNow - gameStartTime) / 1000.0);
}
private void gameUpdate() {
if (!gameOver && !isPaused) {
// ... n
fred.move();
}
}
private void gameRender() {
if (dbImage == null) {
dbImage = createImage(PWIDTH, PHEIGHT);
if (dbImage == null) {
System.out.println("dbImage is null");
return;
} else
dbg = dbImage.getGraphics();
}
dbg.setColor(Color.white);
dbg.fillRect(0, 0, PWIDTH, PHEIGHT);
// ...
dbg.setColor(Color.blue);
dbg.setFont(font);
dbg.drawString(String.format("Average FPS: %.2f", averageFPS),
20, 25);
obs.draw(dbg);
fred.draw(dbg);
if (gameOver) {
gameOverMessage(dbg);
}
}
private void gameOverMessage(Graphics g) {
String msg = String.format("Game Over: Result is %d", score);
int x = (PWIDTH - metrics.stringWidth(msg)) / 2;
int y = (PHEIGHT - font.getSize()) / 2;
g.setColor(Color.red);
g.drawString(msg, x, y);
}
private void paintScreen() {
Graphics g;
try {
g = getGraphics();
if (g != null && dbImage != null) {
g.drawImage(dbImage, 0, 0, null);
Toolkit.getDefaultToolkit().sync();
g.dispose();
}
} catch (Exception e) {
System.out.println("Gabim ne kontekstin grafik");
}
}
/*
* @Override public void paintComponent(Graphics g) { if (dbImage != null)
* g.drawImage(dbImage, 0, 0, null); }
*/
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
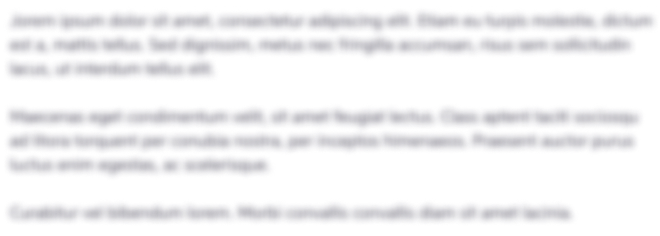
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started