Question
HELLO I have the base done, but am having some erros in getting this to run. This needs to be in C++. The problem is
HELLO I have the base done, but am having some erros in getting this to run. This needs to be in C++. The problem is as follows and below the hypens are the different files. thank you!
"
Package-delivery services, such as FedEx, DHL and UPS, offer a number of different shipping options, each with specific costs associated.
Create an inheritance hierarchy containing super class Package and subclasses RegularPackage, TwoDayPackage and OvernightPackage.
Super class Package.java
It includes data members representing the package id, first and last name for both the sender and the recipient of the package (in fact, address, city, state and ZIP code for both the sender and the recipient should be included).
It includes data members that store the weight (in ounces) and cost per ounce to ship the package.
Package
"
-------------------------------------------------
/* PACKAGE.CPP*/
#include "stdafx.h"
#include "Package.h"
#include "Package.h"
// Package.cpp
// Member-function definitions of class Package.
#include
#include "Package.h" // Package class definition
using namespace std;
// constructor initializes data members
Package::Package(const string& sName, const string& sAddress,
const string& sCity, const string& sState, int sZIP,
const string& rName, const string& rAddress, const string& rCity,
const string& rState, int rZIP, double w, double cost)
: senderName(sName), senderAddress(sAddress), senderCity(sCity),
senderState(sState), senderZIP(sZIP), recipientName(rName),
recipientAddress(rAddress), recipientCity(rCity),
recipientState(rState), recipientZIP(rZIP) {
setWeight(w); // validate and store weight
setCostPerOunce(cost); // validate and store cost per ounce
}
// set sender's name
void Package::setSenderName(const string& name) {
senderName = name;
}
// return sender's name
string Package::getSenderName() const {
return senderName;
}
// set sender's address
void Package::setSenderAddress(const string& address) {
senderAddress = address;
}
// return sender's address
string Package::getSenderAddress() const {
return senderAddress;
}
// set sender's city
void Package::setSenderCity(const string& city) {
senderCity = city;
}
// return sender's city
string Package::getSenderCity() const {
return senderCity;
}
// set sender's state
void Package::setSenderState(const string& state) {
senderState = state;
}
// return sender's state
string Package::getSenderState() const {
return senderState;
}
// set sender's ZIP code
void Package::setSenderZIP(int zip) {
senderZIP = zip;
}
// return sender's ZIP code
int Package::getSenderZIP() const {
return senderZIP;
}
// set recipient's name
void Package::setRecipientName(const string& name) {
recipientName = name;
}
// return recipient's name
string Package::getRecipientName() const {
return recipientName;
}
// set recipient's address
void Package::setRecipientAddress(const string& address) {
recipientAddress = address;
}
// return recipient's address
string Package::getRecipientAddress() const {
return recipientAddress;
}
// set recipient's city
void Package::setRecipientCity(const string& city) {
recipientCity = city;
}
// return recipient's city
string Package::getRecipientCity() const {
return recipientCity;
}
// set recipient's state
void Package::setRecipientState(const string& state) {
recipientState = state;
}
// return recipient's state
string Package::getRecipientState() const {
return recipientState;
}
// set recipient's ZIP code
void Package::setRecipientZIP(int zip) {
recipientZIP = zip;
}
// return recipient's ZIP code
int Package::getRecipientZIP() const {
return recipientZIP;
}
// validate and store weight
void Package::setWeight(double w) {
if (w >= 0.0) {
weight = w;
}
else {
throw invalid_argument("Weight must be >= 0.0");
}
}
// return weight of package
double Package::getWeight() const {
return weight;
}
// validate and store cost per ounce
void Package::setCostPerOunce(double cost) {
if (cost >= 0.0) {
costPerOunce = cost;
}
else {
throw invalid_argument("Cost must be >= 0.0");
}
}
// return cost per ounce
double Package::getCostPerOunce() const {
return costPerOunce;
}
// calculate shipping cost for package
double Package::calculateCost() const {
return getWeight() * getCostPerOunce();
}
/* END PACKAGE.CPP*/
---------------------------------------------------------------------
/* PackageDeliveryApplication2.CPP*/
// PackageDeliveryApplication2.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
// PackageDeliveryApplication.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
// Driver program for Package hierarchy.
#include
#include
#include "Package.h" // Package class definition
#include "TwoDayPackage.h" // TwoDayPackage class definition
#include "OvernightDelivery.h" // OvernightPackage class definition
using namespace std;
int main() {
Package package1{ "Lou Brown", "1 Main St", "Boston", "MA", 11111,
"Mary Smith", "7 Elm St", "New York", "NY", 22222, 8.5, .5 };
TwoDayPackage package2{ "Lisa Klein", "5 Broadway", "Somerville", "MA",
33333, "Bob George", "21 Pine Rd", "Cambridge", "MA", 44444,
10.5, .65, 2.0 };
OvernightDelivery package3{ "Ed Lewis", "2 Oak St", "Boston", "MA",
55555, "Don Kelly", "9 Main St", "Denver", "CO", 66666,
12.25, .7, .25 };
cout << fixed << setprecision(2);
//print each package's information and cost
cout << "Package 1: Sender: " << package1.getSenderName()
<< ' ' << package1.getSenderAddress() << ' '
<< package1.getSenderCity() << ", " << package1.getSenderState()
<< ' ' << package1.getSenderZIP();
cout << " Recipient: " << package1.getRecipientName()
<< ' ' << package1.getRecipientAddress() << ' '
<< package1.getRecipientCity() << ", "
<< package1.getRecipientState() << ' '
<< package1.getRecipientZIP();
cout << " Cost: $" << package1.calculateCost() << endl;
cout << " Package 2: Sender: " << package2.getSenderName()
<< ' ' << package2.getSenderAddress() << ' '
<< package2.getSenderCity() << ", " << package2.getSenderState()
<< ' ' << package2.getSenderZIP();
cout << " Recipient: " << package2.getRecipientName()
<< ' ' << package2.getRecipientAddress() << ' '
<< package2.getRecipientCity() << ", "
<< package2.getRecipientState() << ' '
<< package2.getRecipientZIP();
cout << " Cost: $" << package2.calculateCost() << endl;
cout << " Package 3: Sender: " << package3.getSenderName()
<< ' ' << package3.getSenderAddress() << ' '
<< package3.getSenderCity() << ", " << package3.getSenderState()
<< ' ' << package3.getSenderZIP();
cout << " Recipient: " << package3.getRecipientName()
<< ' ' << package3.getRecipientAddress() << ' '
<< package3.getRecipientCity() << ", "
<< package3.getRecipientState() << ' '
<< package3.getRecipientZIP();
cout << " Cost: $" << package3.calculateCost() << endl;
system("pause");
return 0;
}
/*END PackageDeliveryApplication2.CPP*/
---------------------------------------------------------------------
/* stdafx.CPP*/
// stdafx.cpp : source file that includes just the standard includes
// PackageDeliveryApplication2.pch will be the pre-compiled header
// stdafx.obj will contain the pre-compiled type information
#include "stdafx.h"
// TODO: reference any additional headers you need in STDAFX.H
// and not in this file
/*END stdafx.CPP*/
---------------------------------------------------------------------
/*TwoDayDeliveryCPP*/
#include "stdafx.h"
#include "TwoDayDelivery.h"
TwoDayDelivery::TwoDayDelivery()
{
}
TwoDayDelivery::~TwoDayDelivery()
{
}
/*END TwoDayDelivery.CPP*/
---------------------------------------------------------------------
/*.h files*/
---------------------------------------------------------------------
/*TwoDayDelivery.h*/
#pragma once
class TwoDayDelivery
{
public:
TwoDayDelivery();
~TwoDayDelivery();
};
/*END TwoDayDelivery.h*/
---------------------------------------------------------------------
/*targetver.h*/
#pragma once
// Including SDKDDKVer.h defines the highest available Windows platform.
// If you wish to build your application for a previous Windows platform, include WinSDKVer.h and
// set the _WIN32_WINNT macro to the platform you wish to support before including SDKDDKVer.h.
#include
/*END targetver.h*/
---------------------------------------------------------------------
/*stdafx.h*/
// stdafx.h : include file for standard system include files,
// or project specific include files that are used frequently, but
// are changed infrequently
//
#pragma once
#include "targetver.h"
#include
#include
// TODO: reference additional headers your program requires here
/*END stdafx.h*/
---------------------------------------------------------------------
/*Package.h*/
#pragma once
// Package.h
// Definition of base class Package.
#ifndef PACKAGE_H
#define PACKAGE_H
#include
class Package {
public:
// constructor initializes data members
Package(const std::string&, const std::string&, const std::string&,
const std::string&, int, const std::string&, const std::string&, const std::string&,
const std::string&, int, double, double);
void setSenderName(const std::string&); // set sender's name
std::string getSenderName() const; // return sender's name
void setSenderAddress(const std::string&); // set sender's address
std::string getSenderAddress() const; // return sender's address
void setSenderCity(const std::string&); // set sender's city
std::string getSenderCity() const; // return sender's city
void setSenderState(const std::string&); // set sender's state
std::string getSenderState() const; // return sender's state
void setSenderZIP(int); // set sender's ZIP code
int getSenderZIP() const; // return sender's ZIP code
void setRecipientName(const std::string&); // set recipient's name
std::string getRecipientName() const; // return recipient's name
void setRecipientAddress(const std::string&); // set recipient's address
std::string getRecipientAddress() const; // return recipient's address
void setRecipientCity(const std::string&); // set recipient's city
std::string getRecipientCity() const; // return recipient's city
void setRecipientState(const std::string&); // set recipient's state
std::string getRecipientState() const; // return recipient's state
void setRecipientZIP(int); // set recipient's ZIP code
int getRecipientZIP() const; // return recipient's ZIP code
void setWeight(double); // validate and store weight
double getWeight() const; // return weight of package
void setCostPerOunce(double); // validate and store cost per ounce
double getCostPerOunce() const; // return cost per ounce
double calculateCost() const; // calculate shipping cost for package
private:
// data members to store sender and recipient's address information
std::string senderName;
std::string senderAddress;
std::string senderCity;
std::string senderState;
int senderZIP;
std::string recipientName;
std::string recipientAddress;
std::string recipientCity;
std::string recipientState;
int recipientZIP;
double weight; // weight of the package
double costPerOunce; // cost per ounce to ship the package
};
#endif
/*END Package.h*/
---------------------------------------------------------------------
/*OvernightDelivery.h*/
#pragma once
#pragma once
// Exercise 11.9 Solution: OvernightPackage.h
// Definition of derived class OvernightPackage.
#ifndef OVERNIGHT_H
#define OVERNIGHT_H
#include "Package.h"
using namespace std;
class OvernightDelivery: public Package {
public:
OvernightDelivery(const std::string&, const std::string&, const std::string&,
const std::string&, int, const std::string&, const std::string&, const std::string&,
const std::string&, int, double, double, double);
void setOvernightFeePerOunce(double); // set overnight fee
double getOvernightFeePerOunce() const; // return overnight fee
double calculateCost() const; // calculate shipping cost for package
private:
double overnightFeePerOunce; // fee per ounce for overnight delivery
};
#endif
/*END OvernightDelivery.h*/
---------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
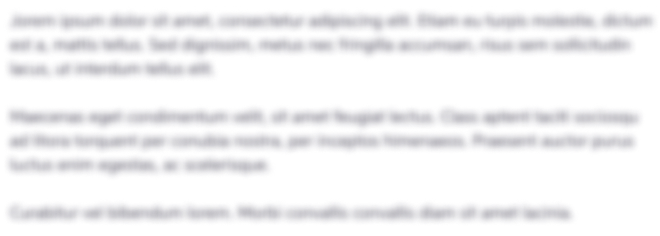
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started