Question
Hello, I have the source code of a java calculator, but I want to make it can be controlled by keyboard too, so could you
Hello, I have the source code of a java calculator, but I want to make it can be controlled by keyboard too, so could you please help out? And when you finish it, could you please compress the whole project to a zip file, and reach to my google, whose id is soaking12138. Thank you so much.
Here is the source code:
package calculator;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.EventQueue;
import javax.swing.DefaultListModel;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.border.EmptyBorder;
import javax.swing.JButton;
import javax.swing.JTextField;
import java.awt.GridLayout;
import javax.swing.JList;
import java.awt.Font;
import javax.swing.SwingConstants;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.text.DecimalFormat;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JScrollBar;
public class Calculator extends JFrame{
private JPanel contentPane;
private JPanel pnDisplay;
private JPanel pnInput;
private JPanel pnHistory;
private JTextField textField;
private JButton btn7;
private JButton btn8;
private JButton btn9;
private JButton btnAdd;
private JButton btnClearAll;
private JButton btn4;
private JButton btn5;
private JButton btn6;
private JButton btnMinus;
private JButton btnClearText;
private JButton btn1;
private JButton btn2;
private JButton btn3;
private JButton btnMultiply;
private JButton btnMemSet;
private JButton btn0;
private JButton btnDot;
private JButton btnEqual;
private JButton btnDivide;
private JButton btnMemRead;
private DefaultListModel
private JList
JScrollPane scrollPane = new JScrollPane();
// String to store input data
private String exp="";
/**
* Launch the application.
*/
void updateTextDigit(String s){
exp+=s;
textField.setText(exp);
model.addElement(s);
}
public static double eval(final String str) {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < str.length()) ? str.charAt(pos) : -1;
}
boolean eat(int charToEat) {
while (ch == ' ') nextChar();
if (ch == charToEat) {
nextChar();
return true;
}
return false;
}
double parse() {
nextChar();
double x = parseExpression();
if (pos < str.length()) throw new RuntimeException("Unexpected: " + (char)ch);
return x;
}
// Grammar:
// expression = term | expression `+` term | expression `-` term
// term = factor | term `*` factor | term `/` factor
// factor = `+` factor | `-` factor | `(` expression `)`
//| number | functionName factor | factor `^` factor
double parseExpression() {
double x = parseTerm();
for (;;) {
if(eat('+')) x += parseTerm(); // addition
else if (eat('-')) x -= parseTerm(); // subtraction
else return x;
}
}
double parseTerm() {
double x = parseFactor();
for (;;) {
if(eat('x')) x *= parseFactor(); // multiplication
else if (eat('/')) x /= parseFactor(); // division
else return x;
}
}
double parseFactor() {
if (eat('+')) return parseFactor(); // unary plus
if (eat('-')) return -parseFactor(); // unary minus
double x;
int startPos = this.pos;
if (eat('(')) { // parentheses
x = parseExpression();
eat(')');
} else if ((ch >= '0' && ch <= '9') || ch == '.') { // numbers
while ((ch >= '0' && ch <= '9') || ch == '.') nextChar();
x = Double.parseDouble(str.substring(startPos, this.pos));
} else if (ch >= 'a' && ch <= 'z') { // functions
while (ch >= 'a' && ch <= 'z') nextChar();
String func = str.substring(startPos, this.pos);
x = parseFactor();
if (func.equals("sqrt")) x = Math.sqrt(x);
else if (func.equals("sin")) x = Math.sin(Math.toRadians(x));
else if (func.equals("cos")) x = Math.cos(Math.toRadians(x));
else if (func.equals("tan")) x = Math.tan(Math.toRadians(x));
else throw new RuntimeException("Unknown function: " + func);
} else {
throw new RuntimeException("Unexpected: " + (char)ch);
}
if (eat('^')) x = Math.pow(x, parseFactor()); // exponentiation
return x;
}
}.parse();
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Calculator frame = new Calculator();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Calculator() {
super("Simple Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
pnDisplay = new JPanel();
contentPane.add(pnDisplay, BorderLayout.NORTH);
pnDisplay.setLayout(new GridLayout(0, 1, 0, 0));
textField = new JTextField();
textField.setHorizontalAlignment(SwingConstants.RIGHT);
textField.setFont(new Font("Courier New", Font.PLAIN, 28));
textField.disable();
pnDisplay.add(textField);
textField.setDisabledTextColor(Color.black);
textField.setColumns(10);
pnInput = new JPanel();
contentPane.add(pnInput, BorderLayout.CENTER);
pnInput.setLayout(new GridLayout(4, 5, 5, 5));
btn7 = new JButton("7");
btn7.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("7");
}
});
btn7.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn7);
btn8 = new JButton("8");
btn8.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("8");
}
});
btn8.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn8);
btn9 = new JButton("9");
btn9.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("9");
}
});
btn9.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn9);
btnAdd = new JButton("+");
btnAdd.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int len=exp.length();
len=len-1;
String c="";
int f=0;
try {
c = Character.toString(exp.charAt(len));
}catch (Exception e){
f=1;
}
if(f!=1){
if(c.equals("+")||c.equals("-")||c.equals("x")||c.equals("/")||c.equals(".")){
}
else{
updateTextDigit("+");
}}
}
});
btnAdd.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnAdd);
btnClearAll = new JButton("C");
btnClearAll.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
// reset all the data
textField.setText("");
exp="";
}
});
btnClearAll.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnClearAll);
btn4 = new JButton("4");
btn4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("4"); }
});
btn4.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn4);
btn5 = new JButton("5");
btn5.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("5");
}
});
btn5.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn5);
btn6 = new JButton("6");
btn6.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("6");
}
});
btn6.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn6);
btnMinus = new JButton("-");
btnMinus.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int len=exp.length();
len=len-1;
String c="";
int f=0;
try {
c = Character.toString(exp.charAt(len));
}catch (Exception e){
f=1;
}
if(f!=1){
if(c.equals("+")||c.equals("-")||c.equals("x")||c.equals("/")||c.equals(".")){
}
else{
updateTextDigit("-");
}}
}
});
btnMinus.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnMinus);
btnClearText = new JButton("CE");
btnClearText.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// reset the textfield only
textField.setText("");
exp="";
model.clear();
}
});
btnClearText.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnClearText);
btn1 = new JButton("1");
btn1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("1");
}
});
btn1.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn1);
btn2 = new JButton("2");
btn2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("2");
}
});
btn2.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn2);
btn3 = new JButton("3");
btn3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("3");
}
});
btn3.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn3);
btnMultiply = new JButton("x");
btnMultiply.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int len=exp.length();
len=len-1;
String c="";
int f=0;
try {
c = Character.toString(exp.charAt(len));
}catch (Exception e){
f=1;
}
if(f!=1){
if(c.equals("+")||c.equals("-")||c.equals("x")||c.equals("/")||c.equals(".")){
}
else{
updateTextDigit("x");
}}
}
});
btnMultiply.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnMultiply);
btnMemSet = new JButton("MS");
btnMemSet.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnMemSet);
btn0 = new JButton("0");
btn0.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
updateTextDigit("0");
}
});
btn0.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btn0);
btnDot = new JButton(".");
btnDot.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int len=exp.length();
len=len-1;
String c="";
int f=0;
try {
c = Character.toString(exp.charAt(len));
}catch (Exception e){
f=1;
}
if(f!=1){
if(c.equals("+")||c.equals("-")||c.equals("*." +
"")||c.equals("/")||c.equals(".")){
}
else{
updateTextDigit(".");
}}
}
});
btnDot.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnDot);
btnEqual = new JButton("=");
btnEqual.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
DecimalFormat f = new DecimalFormat("##.0000");
if(exp!="") {
try {
exp = Double.toString(eval(exp));
textField.setText(exp);
model.addElement("=");
model.addElement(exp);
} catch (Exception e) {
exp = "Error";
textField.setText(exp);
model.addElement("=");
model.addElement(exp);
exp="";
}
}
}
});
btnEqual.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnEqual);
btnDivide = new JButton("/");
btnDivide.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int len=exp.length();
len=len-1;
String c="";
int f=0;
try {
c = Character.toString(exp.charAt(len));
}catch (Exception e){
f=1;
}
if(f!=1){
if(c.equals("+")||c.equals("-")||c.equals("x")||c.equals("/")||c.equals(".")){
}
else{
updateTextDigit("/");}}
}
});
btnDivide.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnDivide);
btnMemRead = new JButton("MR");
btnMemRead.setFont(new Font("Times Roman", Font.BOLD, 14));
pnInput.add(btnMemRead);
pnHistory = new JPanel();
contentPane.add(pnHistory, BorderLayout.EAST);
pnHistory.setPreferredSize(new Dimension(120, 200));
pnHistory.setLayout(new BorderLayout(5, 5));
model =new DefaultListModel
list = new JList
scrollPane.setViewportView(list);
list.setLayoutOrientation(JList.VERTICAL);
list.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent arg0) {
if((String)list.getSelectedValue()!=null) {
exp="";
updateTextDigit((String)list.getSelectedValue());
}
}
});
pnHistory.add(scrollPane);
/*
* End of GUI code
*/
// initialize data
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
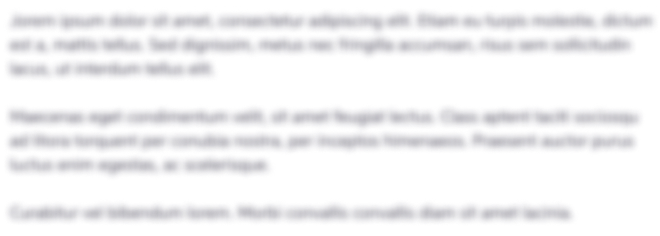
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started