Question
Hello I have this code that for the most part it works. in part 8 of the choices from menu the outputs needs to be
Hello I have this code that for the most part it works. in part 8 of the choices from menu the outputs needs to be in hexadecimal, but I cant it to work. can someone help me get part 8 to display the values is hex.
////////////////////////////////////////////////////////////////////////////
here is the code::
//Name: Joseph Arce
//Name: Mitchell Matos
//Team 2
//Date:2/15/2021
//School: NJIt
//Project Name: Lab#5.V1.0
//
//
/// This program implement the IEEE single precision 754 floating point
// The purpose here is to promt the user for a value once they value is capture
// the user will be prompt again to selct from a menu what kind of operation
//he/she want the promgram to do with the value entered.
// This program consist of different function that perfrom on the value
// this program convert a base-10 decimal value positive or negative
// to the IEEE standard floating point(IEEE 754)
#include
#include
#include
// STL
// More header files in future we save them in header files
using namespace std;// subset of // Function prototypes void prompt_user(double& numb); void sign(double& numb); // this funtions uses and if-statemnt to check if the value if positive or negative void intconvert(int& x, double& numb, double& y); void decconvert(int& x, double& numb, double& y); void floattobinary(float f); int base10(int n, int i); void printBinary(int n, int i); /*this function will prompt the user for the value and display the menu. Using a switch menu this function will control the flow of the program as selcted by the user*/ // the function only takes one pass by reffenrence value /*we use union and structure for IEEE 754 REPRESENTATION*/ typedef union { float f; struct { unsigned int mantissa : 23; unsigned int exponent : 8; unsigned int sign : 1; } raw; } myfloat; void sign(double& numb) { if (numb >= 0) cout << "SIGN BIT IS(1) the number is positive "; else cout << "SIGN BIT IS(0) the number is negative "; } /////////////////////// // // separate the integer form the decimal //function to conver int to binary // iont x= 000001 (x=1) // stringz = 000000100 (z=000000100) void intconvert(int& x, double& numb, double& y) { //type case (only the integer part) x = (int)numb; //set intbin to empty //intbin = ""; //print value in base-10 use absolute value cout << "IN BASE-10 : " << abs(x) << endl; //print value in binary obtained for thw while loop cout << "IN BINARY : "; // setiing x to abs of x to make sure number stays positive x = abs(x); //set array size to 8 and assign to 0 int binary_numb[8] = { 0 }, i; //array size to loop through each array //initilize i = 0; //while loop to go through each array while (x > 0) { //set each array = to x%2 binary_numb[i] = x % 2; x = x / 2;//intger division i++; //increment to move next array } //for loop to only display from the array numbers 0 to 7 for (int j = 7; j >= 0; j--) cout << binary_numb[j]; cout << " "; } //////////////////// // //function to convert decimal part to bianry void decconvert(int& x, double& numb, double& y) { //type case (only the integer part) x = (int)numb; //subtract the integer part and store the decimal value in y y = numb - x; //only the decimal part //only the decimal part y = abs(y); //display decimal value in base-10 cout << "IN BASE 10 : " << y << endl; //display decimal value in binary cout << "IN BINARY : 0."; /*while loop to perform multiplicatopn and obtain the binary */ while ((y < 1)) { cout << (int)(y * 2); y = y * 2; //y++; } } /*for converting float to binary*/ void floattobinary(float f) { int bit = 0; int* b = reinterpret_cast cout << ((*b >> 31) & 1) << " "; for (int k = 30; k >= 23; k--) { bit = ((*b >> k) & 1); cout << bit; } cout << " "; for (int k = 30; k >= 23; k--) { bit = ((*b >> k) & 1); cout << bit; } } /*for converting into base 10*/ int base10(int n, int i) { long int x = pow(2, i - 1); long int sum = 0; for (int k = i - 1; k >= 0; k--) { if ((n >> k) & 1) sum += x; x /= 2; } return sum; } /*for printing in IEEE 754 representation*/ void printIEEE(myfloat var) { printf("%d | ", var.raw.sign); printBinary(var.raw.exponent, 8); printf(" | "); printBinary(var.raw.mantissa, 23); printf(" "); } ////////////////////////////////////////////////hex to binary // function to find hexadecimal // equivalent of binary voidconvertBinToHex(string &ieee) { int length = ieee.length(); int start = 0, end = 4; string str = ""; int count = 1; for (int t = 0; t < ieee.length(); t++) { cout << ieee.at(t); if (count % 4 == 0) { cout << "\t"; } count++; } cout << endl; count = 1; for (int t = 0; t < ieee.length(); t++) { cout << "-"; if (count % 4 == 0) { cout << "\t"; } count++; } for (int i = 0; i < length + 1; i += 4) { str = ieee.substr(start, end); start += 4; if (str == "0000") cout << "0\t"; if (str == "0001") cout << "1\t"; if (str == "0010") cout << "2\t"; if (str == "0011") cout << "3\t"; if (str == "0100") cout << "4\t"; if (str == "0101") cout << "5\t"; if (str == "0110") cout << "6\t"; if (str == "0111") cout << "7\t"; if (str == "1000") cout << "8\t"; if (str == "1001") cout << "9\t"; if (str == "1010") cout << "A\t"; if (str == "1011") cout << "B\t"; if (str == "1100") cout << "C\t"; if (str == "1101") cout << "D\t"; if (str == "1110") cout << "E\t"; if (str == "1111") cout << "F\t"; str = ""; } cout << str << hex << endl; } /*for printing binary*/ void printBinary(int n, int i) { int k; for (k = i - 1; k >= 0; k--) { if ((n >> k) & 1) printf("1"); else printf("0"); } } void prompt_user(double& numb,string& ieee) { // declaration myfloat var; double y;// double y to store the decimalpart int x; float x1; // int x to store the integer par //initalization y = 0.0; x = 0; //decconvert(y, decbin); //concat(intbin, decbin, numbin); //give a descrition of the program cout << "THIS PROGRAM CONVERTS A BASE-10 DECIMAL VALUE " << "TO THE IEEE FLOATING POINT 754 STANDARD "; //prompt for a base-10 decimal value cout << " Enter a number: "; //capturw value cin >> numb; x1 = (float)numb; var.f = x1; //menu to be desiplay showing the options for the user cout << "PLEASE CHOOSE ONE OF THE FOLLOWING OPERARATIONS: " << "\t1. DISPLAY THE SIGN BIT VALUE " << "\t2.DISPLAY THE INTEGER PART IN BOTH BASE-10 AND BINARY FORMATS " << "\t3.DISPLAY THE DECIMAL PART IN BOTH BASE-10 AND BINARY FORMATS " << "\t4.DISPLAY THE NUMBER ENTERED IN BOTH BASE-10 AND BINARY FORMATS " << "\t5.DISPLAY THE MANTISSA IN BINARY FORMAT " << "\t6.DISPLAY THE EXPONENT IN BOTH BASE-10 AND BINARY FORMAT " << "\t7.DISPLAY THE IEEE 754 SINGLE PRECISION BINARY LAYOUT " << "\t8.DISPLAY THE IEEE 754 SINGLE PRECISION HEX LAYOUT "; // number for menu options int choice; //prompt the user for the coice cout << " Please select the type of function " << " you wish the program to execute "; // captured it cin >> choice; // the switch statment is used to control the flow of the program // based on the choice of the user // switch statement for execution choice switch (choice) { case 1: sign(numb); break; case 2: intconvert(x, numb, y); break; case 3: decconvert(x, numb, y); break; case 4: cout << "IN BASE 10 : " << numb << endl; cout << "IN BINARY : " << endl; floattobinary(x1); cout << endl; break; case 5: cout << "MANTISSA IN BINARY : "; printBinary(var.raw.mantissa, 23); cout << endl; break; case 6: cout << "IN BASE 10 : " << base10(var.raw.exponent, 8) << endl; cout << "IN BINAERY : "; printBinary(var.raw.exponent, 8); cout << " "; break; case 7: cout << "IEEE 754 REPRESENTATION OF " << numb << " is : "; printIEEE(var); cout << endl; break; case 8: //cout << "Number entered is " << var.f << " "; //cout << "IEEE 754 is "; //printIEEE(var); ieee = ieee + to_string(var.f); //cout << "and \t"; cout << " Hexadecimal representation is = " << endl; convertBinToHex(ieee); // displaying the number in IEEE format ,32 bits cout << hex <<" "; break; default: cout << "invalid choice "; break; } } int main() { //local variables double numb; string ieee; prompt_user(numb, ieee); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
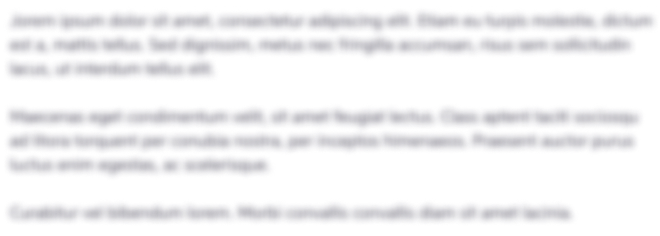
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started