Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Hello, I need help finishing LessonsWithRentalDemo.java I keep receiving errors LessonWithRentalDemo.java You may want to use RentalDemo as a starting point or extend it. You
Hello, I need help finishing LessonsWithRentalDemo.java
I keep receiving errors
- LessonWithRentalDemo.java
- You may want to use RentalDemo as a starting point or extend it. You no longer will need to sort rentals.
- You will need to make an array of 3 LessonWithRental objects based on user input
- After creating the objects and filling the array, you will then display the details of each LessonWithRental. You need to show a summary of rental details and need to also display the information from the getInstructor method call.
rental class
public class Rental { public static final int MINUTES_IN_HOUR = 60; public static final int HOUR_RATE = 40; // The rental rate for an hour public static final int CONTRACT_NUM_LENGTH = 4; // The required length of the contract number private String contractNumber; private int hours; private int extraMinutes; private double totalPrice; // Total cost of the rental int equipmentType; public final String[] equipment = {"personal watercraft", "pontoon boat", "rowboat", "canoe", "kayak", "beach chair", "umbrella", "other"}; /** * Creates a Rental object with a contract number of "A000" and rental time of 0. * Price is also set to 0. * Any additional instance variables are set to a default value. */ public Rental() { this("A000", 0, 0); } /** * Creates a Rental object based on given contract number and rental minutes. * * @param num The contract number for this Rental * @param minutes The total number of minutes for this Rental */ public Rental(String num, int minutes, int equipmentType) { setContractNumber(num); setHoursAndMinutes(minutes); setEquipmentType(equipmentType); } /** * Sets the contract number of this Event based on the given value. * If it meets the format requirements it sets it to the uppercase version of the * given value. Otherwise, it sets it to "A000" * * @param num The desired contract number */ private void setContractNumber(String num) { if (num.length() != CONTRACT_NUM_LENGTH || !Character.isLetter(num.charAt(0)) || !Character.isDigit(num.charAt(1)) || !Character.isDigit(num.charAt(2)) || !Character.isDigit(num.charAt(3))) { contractNumber = "A000"; } else { contractNumber = num.toUpperCase(); } } /** * Sets the hours, extra minutes, and price based on the given minutes. * * @param minutes The total number of minutes for this Rental */ private void setHoursAndMinutes(int minutes) { hours = minutes / MINUTES_IN_HOUR; extraMinutes = minutes % MINUTES_IN_HOUR; if (extraMinutes <= HOUR_RATE) { totalPrice = hours * HOUR_RATE + extraMinutes; } else { totalPrice = hours * HOUR_RATE + HOUR_RATE; } } /** * Gets the contract number of the Rental * * @return this Rental's contractNumber */ public String getContractNumber() { return contractNumber; } /** * Gets the number of hours for this Rental * * @return this Rental's hour */ public int getHours() { return hours; } /** * Gets the number of extra minutes beyond an hour for this Rental * * @return this Rental's extraMinutes */ public int getExtraMinutes() { return extraMinutes; } /** * Gets the total price of this Rental * * @return this Rental's price */ public double getPrice() { return totalPrice; } //get method for equipment type public int getEquipmentType() { return equipmentType; } public String getEquipmentTypeString() { var equipment = this.equipment[equipmentType]; return equipment; } //set method for equipment type public void setEquipmentType(int num) { if(num > equipment.length) this.equipmentType = 7; //sets to equipment to other if value is higher else {this.equipmentType = num;} //equipment besides other } }
rentaldemo
public class RentalDemo { public static void main(String[] args) { // Create 8 Rental objects based on user input int equipmentType = 0; int SIZE = 8; int selection = 0; Rental[] rental = new Rental[SIZE]; Scanner input = new Scanner(System.in); for (int i = 0; i < rental.length; i++) { //Initialize event object rental[i] = new Rental(); //Inputs and sets contract number askUserForContractNumber(); //Inputs number of minutes askUserForMinutes(); //sets price rental[i].getPrice(); //Inputs event type System.out.print("Enter event type (0-7): "); equipmentType = input.nextInt(); //Validate event type while (equipmentType < 0 || equipmentType > 7) { System.out.println("Event type must be between 0 and 7. "); System.out.print("Please re-enter event type (0-7): "); equipmentType = input.nextInt(); } //set it to event object rental[i].setEquipmentType(equipmentType); } //Sorting options. Selecting 4 will exit. while (selection != 4) { //Displays options System.out.println("Sort events according to : "); System.out.println("1. Contract number, 2. Number of Guests, 3. Event type, 99. Exit"); //Read user selection System.out.print(" Enter number: "); selection = input.nextInt(); //temp rental object + bubble sort algorithms Rental temp = rental[0]; switch (selection) { case 1: //Sorts according to event number for (int i = 0; i < rental.length; i++) { for (int j = 0; j < rental.length; j++) { if (rental[i].getContractNumber().charAt(0) < rental[j].getContractNumber().charAt(0)) { temp = rental[i]; rental[i] = rental[j]; rental[j] = temp; } } } break; case 2: //Sorts according to price for (int i = 0; i < rental.length; i++) { for (int j = 0; j < rental.length; j++) { if (rental[i].getPrice() < rental[j].getPrice()) { temp = rental[i]; rental[i] = rental[j]; rental[j] = temp; } } } break; case 3: //Sorts according to event type for (int i = 0; i < rental.length; i++) { for (int j = 0; j < rental.length; j++) { if (rental[i].getEquipmentType() < rental[j].getEquipmentType()) { temp = rental[i]; rental[i] = rental[j]; rental[j] = temp; } } } break; case 99: //exits program System.out.println("Exiting program"); System.exit(0); default: System.out.println("Invalid selection. Please try again."); } //Displays events in selected order for (int i = 0; i < rental.length; i++) { System.out.println("" + rental[i].getContractNumber() + " Equipment type: " + rental[i].getEquipmentType() + " Price: " + rental[i].getPrice()); } } } /** * Asks the user for the contract number * * @return The contract number provided by the user */ public static String askUserForContractNumber() { String num; Scanner input = new Scanner(System.in); System.out.print("Enter contract number >> "); num = input.nextLine(); return num; } /** * Asks the user for the number of minutes for the rental until a valid * amount is provided. * * @return The number of minutes for the rental provided by the user */ public static int askUserForMinutes() { int minutes; final int LOW_MIN = 60; final int HIGH_MIN = 7200; Scanner input = new Scanner(System.in); System.out.print("Enter minutes >> "); minutes = input.nextInt(); while (minutes < LOW_MIN || minutes > HIGH_MIN) { System.out.println("Time must be between " + LOW_MIN + " minutes and " + HIGH_MIN + " minutes"); System.out.print("Please reenter minutes >> "); minutes = input.nextInt(); } return minutes; } }
lessonswithrental
class LessonsWithRental extends Rental { private boolean isLessonRequired; String equipmentType; Scanner input = new Scanner(System.in); final String[] INSTRUCTORS = {"Blade", "Laser", "Tazer", "Meshell", "Fran", "Peter", "White", "Patches"}; public LessonsWithRental(String num, int min, int equipmentType) { super("A000", 0, 0); this.setEquipmentType(equipmentType); this.setIsLessonRequired(); } public LessonsWithRental() { } public void setIsLessonRequired() { if(this.getEquipmentTypeString().equals(equipment[0]) || this.getEquipmentTypeString().equals(equipment[1])) { isLessonRequired = true; } else { isLessonRequired=false; } } public String getInstructor() { for(int i=0;iif(getEquipmentTypeString().equals(equipment[i])) { if(isLessonRequired) return("Lesson is required for "+getEquipmentType()+". Instructor name = "+INSTRUCTORS[i]); else return("Lesson is not required for "+getEquipmentType()+". Instructor name = "+INSTRUCTORS[i]); } return null; } }
lessonswithrentaldemo (this is where i'm stuck)
public class LessonsWithRentalDemo { public static void main(String[] args) { // Create 3 Rental objects based on user input int equipmentType; int minutes; String contractNum; int SIZE = 3; LessonsWithRental[] rental = new LessonsWithRental[SIZE]; Scanner input = new Scanner(System.in); for (int i = 0; i < rental.length; i++) { askUserForContractNumber(); askUserForMinutes(); } // display the initial array System.out.println(" Rental Information: "); for(int i=0;iout.println("Contract Number: " + rental[i].getContractNumber()); System.out.println("Hours: " + rental[i].getHours()); System.out.println("Minutes: " + rental[i].getExtraMinutes()); System.out.println("TotalCost: " + rental[i].getPrice()); System.out.println("Equipment type: " + rental[i].getEquipmentType()); System.out.println(rental[i].getInstructor()+" "); } } /** * Asks the user for the contract number * * @return The contract number provided by the user */ public static String askUserForContractNumber() { String num; Scanner input = new Scanner(System.in); System.out.print("Enter contract number >> "); num = input.nextLine(); return num; } /** * Asks the user for the number of minutes for the rental until a valid * amount is provided. * * @return The number of minutes for the rental provided by the user */ public static int askUserForMinutes() { int minutes; final int LOW_MIN = 60; final int HIGH_MIN = 7200; Scanner input = new Scanner(System.in); System.out.print("Enter minutes >> "); minutes = input.nextInt(); while (minutes < LOW_MIN || minutes > HIGH_MIN) { System.out.println("Time must be between " + LOW_MIN + " minutes and " + HIGH_MIN + " minutes"); System.out.print("Please reenter minutes >> "); minutes = input.nextInt(); } return minutes; } }
thank you in advance
Step by Step Solution
There are 3 Steps involved in it
Step: 1
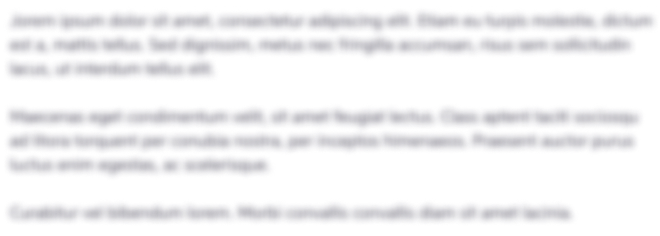
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started