Question
Hello! I need help finishing my python program that is supposed to be a lunar lander. Here's a description: The Lunar Lander Simulation has a
Hello! I need help finishing my python program that is supposed to be a lunar lander. Here's a description:
The Lunar Lander Simulation has a number of initial values. G = 1.622 Pull of Gravity (m/s^2 ) A =50 Altitude, (m) V =0 Initial Velocity (m/s) F =150 Units of Fuel s =0 Number of Seconds passed. The Lander starts 50 meters above the lunar surface. It starts with no initial velocity. The lander has 150 units of fuel it can use to slow its descent. The moon pulls the lander down at -1.622 m/s^2 . The game will simulate time in 1 second steps. At each second, the player will be asked how many units of fuel to burn. Burning fuel slows down the landers descent by 0.1m/s^2
Here is an example input:
yes
0
15
0
15
0
50
0
50
0
20
0
0
0
0
0
no
Here is an example output:
Welcome to Lunar Lander Game.
Do you want to play level 1? (yes/no)
Entering Level 1
Landing on the Moon
Gravity is -1.62 m/s^2
Initial Altitude: 50 meters
Initial Velocity: 0.00 m/s
Burning a unit of fuel causes 0.10 m/s slowdown.
Initial Fuel Level: 150 units
GO
Enter units of fuel to use:
After 1 seconds Altitude is 48.38 meters, velocity is -1.62 m/s.
Remaining Fuel: 150 units.
Enter units of fuel to use:
After 2 seconds Altitude is 46.63 meters, velocity is -1.74 m/s.
Remaining Fuel: 135 units.
Enter units of fuel to use:
After 3 seconds Altitude is 43.27 meters, velocity is -3.37 m/s.
Remaining Fuel: 135 units.
Enter units of fuel to use:
After 4 seconds Altitude is 39.78 meters, velocity is -3.49 m/s.
Remaining Fuel: 120 units.
Enter units of fuel to use:
After 5 seconds Altitude is 34.67 meters, velocity is -5.11 m/s.
Remaining Fuel: 120 units.
Enter units of fuel to use:
After 6 seconds Altitude is 32.94 meters, velocity is -1.73 m/s.
Remaining Fuel: 70 units.
Enter units of fuel to use:
After 7 seconds Altitude is 29.58 meters, velocity is -3.35 m/s.
Remaining Fuel: 70 units.
Enter units of fuel to use:
After 8 seconds Altitude is 29.61 meters, velocity is 0.02 m/s.
Remaining Fuel: 20 units.
Enter units of fuel to use:
After 9 seconds Altitude is 28.01 meters, velocity is -1.60 m/s.
Remaining Fuel: 20 units.
Enter units of fuel to use:
After 10 seconds Altitude is 26.79 meters, velocity is -1.22 m/s.
Remaining Fuel: 0 units.
Enter units of fuel to use:
After 11 seconds Altitude is 23.95 meters, velocity is -2.84 m/s.
Remaining Fuel: 0 units.
Enter units of fuel to use:
After 12 seconds Altitude is 19.48 meters, velocity is -4.46 m/s.
Remaining Fuel: 0 units.
Enter units of fuel to use:
After 13 seconds Altitude is 13.40 meters, velocity is -6.09 m/s.
Remaining Fuel: 0 units.
Enter units of fuel to use:
After 14 seconds Altitude is 5.69 meters, velocity is -7.71 m/s.
Remaining Fuel: 0 units.
Enter units of fuel to use:
After 15 seconds Altitude is 0.00 meters, velocity is -9.33 m/s.
Remaining Fuel: 0 units.
Crashed!
Do you want to play level 1? (yes/no)
You made it past 0 levels.
Thanks you Playing.
f =Ask Player Fuel to Burn
F =F f Remove Fuel from tank
V =V + G + T f Update Velocity
A =A + V Update Altitude
s =s + 1 Record that a second passed
The simulation should continue until the altitude is 0 or less. When the altitude reaches 0, that means the lander has landed. For the landing to be safe, the speed must be between 2m/s and 2m/s. If the landing is outside this range, then the lander will be destroyed when it lands. The program requires at least two functions:
1. Create a function def ask fuel(current fuel)) that gets the amount of fuel from the user. The amount of fuel entered is only valid if it meets certain 2 requirements. Repeatedly ask the player for an input until they give you a valid amount. The fuel to burn must meet the following requirements:
Is an integer amount. The lander cannot burn decimal amounts.
Is a positive number. The lander cannot burn negative fuel.
Is less than or equal to the remaining fuel. The lander cannot burn more fuel then it has.
2. Create a function def play level(name,G,fuel) that allows the player to land on a planet. For the moon, this function would be called play level(Moon,-1.622,150). The function should print an introduction with all the information about the current level. For example:
Landing on the Moon
Gravity is 1.62 m/ s 2
Initial Altitude: 50 meters
Initial Velocity: 0. 0 0 m/ s
Burning a unit of fuel causes 0. 1 0 m/ s slowdown .
Initial Fuel Level: 150 units
GO
The simulation should then run until the player either dies in a horrible crash or lands safely.
After 1 seconds, Altitude is 48. 38 meters, velocity is 1.62 m/ s.
Remaining Fuel : 150 units.
Enter units of fuel to use:
15
After 2 seconds altitude is 46.63 meters, velocity is 1.74 m/ s.
Remaining Fuel: 135 units.
Enter units of fuel to use:
0
The altitude should never print a negative number. The lander stops at altitude 0. It is either safe or a wreck, but it cannot be at a negative altitude. If the lander was going to fast to land print:
Crashed!
If the lander was at a slow speed then:
Landed Successfully.
Here is the program I have so far.
print('Welcome to Lunar Lander Game.')
do_you_want_to_play = input('Do you want to play level 1? (yes/no) ')
print('Entering Level 1')
print('Landing on the Moon')
print('Gravity is -1.62 m/s^2')
print('Initial Altitude: 50 meters')
print('Initial Velocity: 0.00 m/s')
print('Burning a unit of fuel causes 0.10 m/s slowdown.')
print('Initial Fuel Level: 150 units')
print('')
print('GO')
G = -1.622 #Pull of Gravity (m/s^2)
A = 50 #Altitude (m)
V = 0
F = 150
s = 0
T = 0.1
def ask_fuel(current_fuel):
try:
current_fuel = input('Enter units of fuel to use: ')
current_fuel = int(current_fuel)
return current_fuel
except ValueError:
print("Enter units of fuel to use:")
def play_level(name, G, F):
Remove_Fuel_From_Tank = F - f
Update_V = V + G + T * f
Update_A = A + Update_V
second_passed = s + 1
print('After 1 seconds Altitude is', Update_A, 'meters, velocity is', round(Update_V,2), ".")
print("Remaining Fuel:", Remove_Fuel_From_Tank, "units.")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
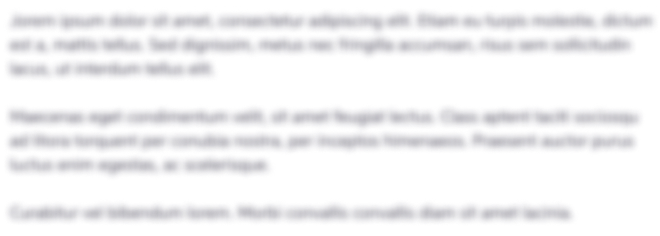
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started