Question
Hello, I need help with this prime number program. Just need add short wait methods in multi-thread in LongFifo void add(long value) throws InterruptedException and
Hello, I need help with this prime number program. Just need add short wait methods in multi-thread in LongFifo "void add(long value) throws InterruptedException" and "long remove() throws InterruptedException;". Here are class. Thank you! (PS: Chegg said the question is too long when I attached all 7 short class. So, I took the PrimeMain with screenshot))
LongFifo.class
public interface LongFifo { /** Returns the number if items currently in the FIFO. */ int getCount();
/** Returns true if {@link #getCount()} == 0. */ boolean isEmpty();
/** Returns true if {@link #getCount()} == {@link #getCapacity()}. */ boolean isFull();
/** Removes any and all items in the FIFO leaving it in an empty state. */ void clear();
/** * Returns the maximum number of items which can be stored in this FIFO. * This value never changes. */ int getCapacity();
/** * Add the specified item to the fifo. * If currently full, the calling thread waits until there is space and * then adds the item. * If this method doesn't throw InterruptedException, then the item was * successfully added. */ void add(long value) throws InterruptedException;
/** * Removes and returns the next item. * If currently empty, the calling thread waits until another thread adds * an item. * If this method doesn't throw InterruptedException, then the item was * successfully removed. */ long remove() throws InterruptedException;
/** * Returns a reference to use for synchronized blocks which need to * call multiple methods without other threads being able to get in. * Never returns null. */ Object getLockObject(); }
CircularArrayLongFifo.class
public class CircularArrayLongFifo implements LongFifo { // do not change any of these fields: private final long[] slots; private int head; private int tail; private int count; private final Object lockObject;
// this constructor is correct as written - do not change public CircularArrayLongFifo(int fixedCapacity, Object proposedLockObject) {
lockObject = proposedLockObject != null ? proposedLockObject : new Object();
slots = new long[fixedCapacity]; head = 0; tail = 0; count = 0; }
// this constructor is correct as written - do not change public CircularArrayLongFifo(int fixedCapacity) { this(fixedCapacity, null); }
// this method is correct as written - do not change @Override public int getCount() { synchronized ( lockObject ) { return count; } }
@Override public boolean isEmpty() { synchronized ( lockObject ) { return count == 0; } }
@Override public boolean isFull() { synchronized ( lockObject ) { return count == slots.length; } }
@Override public void clear() { synchronized ( lockObject ) { // No need - just keep the old junk (harmless): // Arrays.fill(slots, 0); head = 0; tail = 0; count = 0; } }
@Override public int getCapacity() { return slots.length; }
@Override public void add(long value) throws InterruptedException { }
@Override public long remove() throws InterruptedException { return 0L; }
// this method is correct as written - do not change @Override public Object getLockObject() { return lockObject; } }
CandidateGenerator.class
public class CandidateGenerator { private final LongFifo outputFifo;
public CandidateGenerator(LongFifo outputFifo) { this.outputFifo = outputFifo;
Runnable r = new Runnable() { @Override public void run() { runWork(); } };
Thread t = new Thread(r, "CandidateGenerator"); t.start(); }
private void runWork() { try { outputFifo.add(2); long number = 3; while ( true ) { outputFifo.add(number); number += 2; } } catch ( InterruptedException x ) { // ignore and let the thread die } } }
NanoTimer.class
public class NanoTimer { private final double NS_PER_MILLISECOND = 1000000.0; private final double NS_PER_SECOND = NS_PER_MILLISECOND * 1000.0; private final double NS_PER_MINUTE = NS_PER_SECOND * 60.0; private final double NS_PER_HOUR = NS_PER_MINUTE * 60.0; private final double NS_PER_DAY = NS_PER_HOUR * 24.0;
private long nsStartTimeOfInterval; private long nsTotalOfStoppedIntervals = 0L; private boolean paused = true;
// private, use createXYZ methods private NanoTimer() { }
public static NanoTimer createStarted() { NanoTimer timer = new NanoTimer(); timer.start(); return timer; }
public static NanoTimer createStopped() { return new NanoTimer(); }
public synchronized void start() { if ( paused ) { paused = false; nsStartTimeOfInterval = System.nanoTime(); } }
public synchronized void stop() { if ( !paused ) { paused = true; nsTotalOfStoppedIntervals += System.nanoTime() - nsStartTimeOfInterval; } }
public synchronized void reset() { stop(); nsTotalOfStoppedIntervals = 0L; }
public synchronized long getElapsedNanoseconds() { return nsTotalOfStoppedIntervals + (paused ? 0 : System.nanoTime() - nsStartTimeOfInterval); }
public double getElapsedMilliseconds() { return getElapsedNanoseconds() / NS_PER_MILLISECOND; }
public double getElapsedSeconds() { return getElapsedNanoseconds() / NS_PER_SECOND; }
public double getElapsedMinutes() { return getElapsedNanoseconds() / NS_PER_MINUTE; }
public double getElapsedHours() { return getElapsedNanoseconds() / NS_PER_HOUR; }
public double getElapsedDays() { return getElapsedNanoseconds() / NS_PER_DAY; } }
PrimeChecker.class
public class PrimeChecker { private final LongFifo inputFifo; private final LongFifo outputFifo;
public PrimeChecker(LongFifo inputFifo, LongFifo outputFifo) { this.inputFifo = inputFifo; this.outputFifo = outputFifo;
new Thread(new Runnable() { @Override public void run() { runWork(); } }, "PrimeChecker").start(); }
private void runWork() { try { while ( true ) { long candidate = inputFifo.remove(); if ( isPrime(candidate) ) { outputFifo.add(candidate); } } } catch ( InterruptedException x ) { // ignore and let the thread die } }
private boolean isPrime(long number) { if ( number
long divisorLimit = 1 + (long) Math.sqrt(number); for ( long divisor = 2; divisor
PrimePrinter.class
public class PrimePrinter { private final LongFifo inputFifo;
public PrimePrinter(LongFifo inputFifo) { this.inputFifo = inputFifo;
new Thread(new Runnable() { @Override public void run() { runWork(); } }, "PrimePrinter").start(); }
private void runWork() { // take a prime off the "known to be prime" FIFO and print it out... // ... if there aren't any, sleep a bit, then try again.... try { NanoTimer timer = NanoTimer.createStarted(); int maxCountPerLine = 10; int primeCount = 0; int currCount = 0; while ( true ) { long primeNumber = inputFifo.remove(); primeCount++; System.out.printf("%7d ", primeNumber); currCount++; if (currCount == maxCountPerLine) { System.out.println(); currCount = 0; } if (primeNumber > 100000L) { if ( currCount > 0 ) { System.out.println(); } System.out.printf( "Found %,d primes in %.5f seconds with " + "the last one %,d%n", primeCount, timer.getElapsedSeconds(), primeNumber); return; } } } catch ( InterruptedException x ) { // ignore and let the thread die } } }
public class PrimeMain @SuppressWarnings ("unused") public static void main(String] args) LongFifo candidateFifo new CircularArrayLongFifo(500000); LongFifo primeFifo new CircularArrayLongFifo (100); System.out.println("loading up candidate fifo..."); new CandidateGenerator(candidateFifo); while (!candidateFifo.isFull)) Thread.sleep (200); catch InterruptedException x) x.printStackTrace); System.out.println("DONE loading up candidate fifo..."); new PrimeChecker(candidateFifo, primeFifo); /ew PrimeChecker(candidateFifo, primeFifo); /ew PrimeChecker(candidateFifo, primeFifo); new PrimePrinter(primeFifo); public class PrimeMain @SuppressWarnings ("unused") public static void main(String] args) LongFifo candidateFifo new CircularArrayLongFifo(500000); LongFifo primeFifo new CircularArrayLongFifo (100); System.out.println("loading up candidate fifo..."); new CandidateGenerator(candidateFifo); while (!candidateFifo.isFull)) Thread.sleep (200); catch InterruptedException x) x.printStackTrace); System.out.println("DONE loading up candidate fifo..."); new PrimeChecker(candidateFifo, primeFifo); /ew PrimeChecker(candidateFifo, primeFifo); /ew PrimeChecker(candidateFifo, primeFifo); new PrimePrinter(primeFifo)Step by Step Solution
There are 3 Steps involved in it
Step: 1
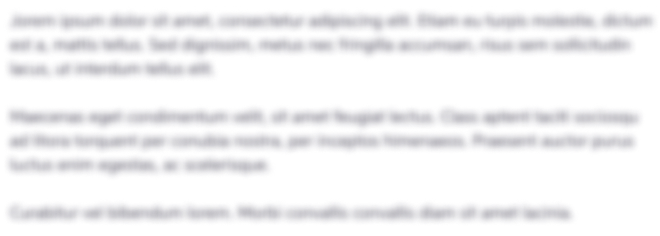
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started