Question
Hello, I need this to be done in java using eclipse. Please create a unit test called EmployeeTest to test every method for the employee
Hello,
I need this to be done in java using eclipse.
Please create a unit test called EmployeeTest to test every method for the employee class. Use the XmlHelperTest as a reference for implementing specific tests or other sources like JUnit.org and other test references.
Please make sure that the test harness is a success whenexecuted
package week03;
import java.text.DecimalFormat;
/**
* Represents an employee
*
* @author scottl
*
*/
public class Employee
{
/**
* Default constructor
*/
public Employee()
{
this("New First", "New Last");
}
/**
* Overloaded constructor
*
* @param first
* First name
* @param last
* Last name
*/
public Employee(String first, String last)
{
this(first, last, 0.0);
}
/**
* Parameterized constructor
*
* @param first
* First name
* @param last
* Last name
* @param salary
* Salary
*/
public Employee(String first, String last, double salary)
{
m_first = first;
m_last = last;
m_salary = salary;
m_decimalFormatter = new DecimalFormat(MONEY_PATTERN);
}
/** Setter for first name */
public void setFirstName(String first)
{
m_first = first;
}
/** Setter for last name */
public void setLastName(String last)
{
m_last = last;
}
/** Setter for last name */
public void setSalary(double salary)
{
m_salary = salary;
}
/** Getter for first name */
public String getFirstName()
{
return m_first;
}
/** Getter for last name */
public String getLastName()
{
return m_last;
}
/**
* Returns a formatted display name For example: LaChance, Scott Display
* format is last, first.
*
* @return Formatted display name as last, first
*/
public String getDisplayName()
{
return String.format("%s, %s", m_last, m_first);
}
/** Getter for salary */
public double getSalary()
{
return m_salary;
}
/**
* Provides the Salary as a string in the following format: "###,##0.00"
* Examples: 5,005.65, 0.65, 5.85, 202,333.99
*
* @return Formated salary string
*/
public String getFormattedSalary()
{
return m_decimalFormatter.format(getSalary());
}
@Override
public int hashCode()
{
int arbitraryPrimeNumber = 31;
int newHash = this.getFirstName().hashCode() * arbitraryPrimeNumber
+ this.getLastName().hashCode() * arbitraryPrimeNumber
+ this.getFormattedSalary().hashCode() * arbitraryPrimeNumber;
return newHash;
};
/**
* This override compares two Employee instances. They are equal if the
* first, last and salary data elements are equal.
*/
@Override
public boolean equals(Object obj)
{
boolean result = false;
if(obj instanceof Employee)
{
Employee rhs = (Employee)obj;
if(this.getFirstName().equals(rhs.getFirstName())
&& this.getLastName().equals(rhs.getLastName())
&& this.getFormattedSalary().equals(
rhs.getFormattedSalary())) // This helps avoid
// issues with double
// precision
{
result = true;
}
}
return result;
}
/**
* Formats the content of the Employee object.
* "LaChance, Scott Salary: $5,005.65"
*/
@Override
public String toString()
{
String output = m_decimalFormatter.format(getSalary());
return String.format("%s Salary: $%s", getDisplayName(), output);
}
/** First name attribute */
private String m_first;
/** Last name attribute */
private String m_last;
/** Salary attribute */
private double m_salary;
/** The format for the salary */
private static String MONEY_PATTERN = "###,##0.00";
/** Formatter instance for formatting the salary */
private DecimalFormat m_decimalFormatter;
}
/*********************************/
package week03;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
/**
* This class executes the JUnit Test specified from the command line This will
* be used by the reference system for testing your code.
*
*
*
*/
public class TestHarness
{
public static void main(String[] args)
{
trace(" testing student JUnit execution");
boolean success1 = testEmployeeTestJUnit();
boolean success2 = inspectEmployeeTestJunit();
if(success1 && success2)
{
trace("****** Test Success!");
}
else
{
trace("****** Test failed!");
}
}
/**
* This opens the employee test file and looks for all the methods that need
* to be tested.
*
* @return true if all the Employee methods are executed, otherwise false
*/
private static boolean inspectEmployeeTestJunit()
{
boolean success = true;
FileReader fileReader = null;
BufferedReader reader = null;
try
{
// read in the file into memory
//File employeeTestFile = new File("src/week03/EmployeeTest.java");
File employeeTestFile = new File("../src/EmployeeTest.java");
if(employeeTestFile.exists())
{
fileReader = new FileReader(employeeTestFile);
reader = new BufferedReader(fileReader);
StringBuilder buffer = new StringBuilder();
String line = "";
while((line = reader.readLine()) != null)
{
buffer.append(line);
buffer.append(' ');
}
success = inspectFile(buffer);
}
else
{
String msg = String.format("File doesn't exist at '%s'", employeeTestFile.getCanonicalPath());
trace(msg);
success = false;
}
}
catch(IOException ex)
{
trace(ex.getMessage());
success = false;
}
finally
{
if( reader != null )try{reader.close();}catch(IOException ex){}
}
return success;
}
private static boolean inspectFile(StringBuilder buffer)
{
boolean success = true;
// Look for the following strings
ArrayList
testsToFind.add("getFirstName(");
testsToFind.add("getLastName(");
testsToFind.add("setFirstName(");
testsToFind.add("setLastName(");
testsToFind.add("getFirstName(");
testsToFind.add("getSalary(");
testsToFind.add("getFormattedSalary(");
testsToFind.add("Employee()");
String twoParameterConstructorRegEx = ".*Employee\\(\".*\", \".*\"\\).*";
String threeParameterConstructorRegEx = ".*Employee\\(\".*\", \".*\", .*\\).*" ;
String text = buffer.toString();
for(String s : testsToFind)
{
if(!text.contains(s))
{
String msg = String.format("test doesn't execute '%s'", s);
trace(msg);
success = false;
}
}
// Check the constructors
trace("testing two parameter constructor");
Pattern p1 = Pattern.compile(twoParameterConstructorRegEx);
Matcher m = p1.matcher(text);
if(!m.find())
{
String msg = String.format(
"failed to match parameterized constructor '%s'",
twoParameterConstructorRegEx);
trace(msg);
success = false;
}
trace("testing three parameter constructor");
Pattern p2 = Pattern.compile(threeParameterConstructorRegEx);
m = p2.matcher(text);
if(!m.find())
{
String msg = String.format(
"failed to match parameterized constructor '%s'",
threeParameterConstructorRegEx);
trace(msg);
success = false;
}
if(!success)
{
// dump the text for analysis
trace(text);
}
return success;
}
private static boolean testEmployeeTestJUnit()
{
boolean success = true;
Result result = org.junit.runner.JUnitCore
.runClasses(EmployeeTest.class);
int failCount = result.getFailureCount();
if(failCount > 0)
{
List
for(Failure fail : failures)
{
trace("FAILED: " + fail.getMessage());
success = false;
}
}
return success;
}
private static void trace(String msg)
{
System.out.println(msg);
}
}
/***********************************/
package week03;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.DOMException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
/**
* Provides support for serializing the Employees
*
* @author scottl
*
*/
public class XmlHelper
{
/**
* Default constructor
*/
public XmlHelper()
{
}
/**
* Saves an employee to an xml file
*
*
To persist the xml to a file we need to use java.io
* * The method works by creating a default document from the default XML
* It then builds the document adding one employee at a time.
* Finally it saves the XML using the transform classes to file.
*
*
The sequence for using the classes is the following:
*
- Use DocumentBuilderFactory to create a new factory instance()
- Create a new File instance with the file name as defined in the UML, m_EMPLOYEE_XML_FILENAME
- Create a ByteArrayInputStream from the default XML string m_INITIAL_XML
*
*
*
*
*
- Create a FileOutputStream using the File instance for the employee)
- Use the DocumentBuilderFactory instance to create a new DocumentBuilder;)
- Use the DocumentBuilder instance to parse the ByteArrayInputStream.
*
*
*
*
*
*
*
* This creates a Document instance.
*
*
* Get the root element of the Document instance)
*
*
- Now for each employee in the list, create the XML entry for the employee
- Use the Document instance to create a new "employee" Element.
- Then create elements for First, Last and Salary.)
- Append these to the employee element.
- Append the employee element to the Document instance
- Set the text content for First, Last and Salary using the Employee data.
*
*
*
*
*
*
*
*
This creates the XML representation of the data.
*
* Now save to an XML file.
*
- Create a TransformerFactory instance
- Use the TransformerFactory to create a Transformer instance
- Set the Transform instance properties as follows:
* transformer.setOutputProperty(OutputKeys.INDENT, "yes");
* transformer.setOutputProperty("{http://xml.apache.org/xslt}indent-amount", "2");
- This pretty prints the XML when it is saved.
- Create a DOMSource and pass in the Document instance
- Create a StreamResult instance using the output file instance
- Use the Transformer instance to transform the source to the result
*
*
*
*
*
*
*
*
*
* @see File
* @see FileOutputStream
* @see ByteArrayInputStream
*
* Uses the following java XML classes
* @see DocumentBuilderFactory
* @see DocumentBuilder
* @see Document
* @see Element
*
* To save as XML we use the transformer classes
* @see TransformerFactory
* @see Transformer
* @see DOMSource
* @see StreamResult
*
*
* @param list
* List of Employees to save
* @throws CollectionsAppDataException
* On error;
*/
public void saveEmployeeList(List
throws Exception
{
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
File outFile = new File(m_EMPLOYEE_XML_FILENAME);
FileOutputStream sOut = null;
ByteArrayInputStream inStream;
try
{
// Create an InputStream from the default XML
inStream = new ByteArrayInputStream(m_INITIAL_XML.getBytes("UTF-8"));
sOut = new FileOutputStream(outFile);
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inStream);
doc.getDocumentElement().normalize();
Element root = doc.getDocumentElement();
for(Employee emp : list)
{
Element employeeElement = doc.createElement("employee");
Element first = doc.createElement("First");
Element last = doc.createElement("Last");
Element salary = doc.createElement("Salary");
employeeElement.appendChild(first);
employeeElement.appendChild(last);
employeeElement.appendChild(salary);
first.setTextContent(emp.getFirstName());
last.setTextContent(emp.getLastName());
salary.setTextContent(String.format("%f", emp.getSalary()));
root.appendChild(employeeElement);
}
// Write the parsed document to an xml file
TransformerFactory transformerFactory = TransformerFactory
.newInstance();
Transformer transformer = transformerFactory.newTransformer();
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
transformer.setOutputProperty("{http://xml.apache.org/xslt}indent-amount", "2");
DOMSource source = new DOMSource(doc);
StreamResult result = new StreamResult(outFile);
transformer.transform(source, result);
}
catch(ParserConfigurationException ex)
{
throw new Exception("Error saving data", ex);
}
catch(DOMException ex)
{
ex.printStackTrace();
throw new Exception("Error creating XML DOM", ex);
}
catch(SAXException ex)
{
ex.printStackTrace();
throw new Exception("Error saving data", ex);
}
catch(FileNotFoundException ex)
{
ex.printStackTrace();
throw new Exception("Error saving data", ex);
}
catch(UnsupportedEncodingException ex)
{
ex.printStackTrace();
throw new Exception("Error saving data", ex);
}
catch(IOException ex)
{
ex.printStackTrace();
throw new Exception("Error saving data", ex);
}
catch(TransformerConfigurationException ex)
{
ex.printStackTrace();
throw new Exception("Error saving to xml", ex);
}
catch(TransformerException ex)
{
ex.printStackTrace();
throw new Exception("Error saving to xml", ex);
}
finally
{
if(sOut != null)
try
{
sOut.close();
}
catch(IOException ex)
{
ex.printStackTrace();
}
}
}
/**
* This reads the source XML file and returns the list of employees. There
* is no caching in this implementation, so it won't scale to thousands when
* it comes to performance.
*
* @see File
* @see FileInputStream
*
* Uses the following java XML classes
* @see DocumentBuilderFactory
* @see DocumentBuilder
* @see Document
* @see Element
*
* @return List of employees from the saved file.
* @throws CollectionsAppDataException
*/
public List
{
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
List
File fileIn = new File(m_EMPLOYEE_XML_FILENAME);
FileInputStream inStream = null;
try
{
inStream = new FileInputStream(fileIn);
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(inStream);
Element root = doc.getDocumentElement();
employeeList = getEmployeesFromXml(root);
}
catch(FileNotFoundException ex)
{
ex.printStackTrace();
throw new Exception("Error loading data", ex);
}
catch(ParserConfigurationException ex)
{
ex.printStackTrace();
throw new Exception("Error creating XML DOM", ex);
}
catch(SAXException ex)
{
ex.printStackTrace();
throw new Exception("Error creating XML DOM", ex);
}
catch(IOException ex)
{
ex.printStackTrace();
throw new Exception("Error creating XML DOM", ex);
}
finally
{
if(inStream != null)
try
{
inStream.close();
}
catch(IOException ex)
{
ex.printStackTrace();
}
}
return employeeList;
}
/**
* Starting from the root node, get the Employee elements using XPath and
* generate marshal the XML data to Employee objects.
*
* @param root The root of the XML DOM
* @return the list of Employees
* @throws CollectionsAppDataException
*/
private List
{
List
NodeList list = root.getElementsByTagName("employee");
for(int i = 0; i < list.getLength(); i++)
{
Element curElement = (Element)list.item(i);
Element firstElements = (Element)curElement.getElementsByTagName("First").item(0);
Element lastElements = (Element)curElement.getElementsByTagName("Last").item(0);
Element salaryElements = (Element)curElement.getElementsByTagName("Salary").item(0);
String first = firstElements.getTextContent();
String last = lastElements.getTextContent();
String salaryString = salaryElements.getTextContent();
try
{
Double salary = Double.parseDouble(salaryString);
Employee newEmployee = new Employee(first, last, salary);
employeeList.add(newEmployee);
}
catch(NumberFormatException ex)
{
throw new Exception("Error loading data", ex);
}
}
return employeeList;
}
private static String m_INITIAL_XML = "
private static String m_EMPLOYEE_XML_FILENAME = "employees.xml";
}
/***********************************/
* XmlHelperTest.java
*
* COP4802 Advanced Java
*/
package week03;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.validation.Validator;
import static org.junit.Assert.*;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.xml.sax.SAXException;
//import junit.framework.TestCase;
/**
* @author scottl
*
*/
public class XmlHelperTest
{
/**
* @throws java.lang.Exception
*/
@Before
public void setUp() throws Exception
{
List
employees.add(new Employee("Scott", "LaChance", 35000.00));
XmlHelper helper = new XmlHelper();
try
{
trace("Testing save");
helper.saveEmployeeList(employees);
}
catch(Exception e)
{
String msg = "Failed to setup xml test file" + e.getMessage();
fail(msg);
}
}
/**
* @throws java.lang.Exception
*/
@After
public void tearDown() throws Exception
{
}
/**
* Test method for {@link week03.XmlHelper#saveEmployeeList(java.util.List)}
* .
*/
@Test
public void testSaveEmployeeList()
{
List
employees.add(new Employee("Scott", "LaChance", 35000.00));
employees.add(new Employee("Jodi", "Boeddeker", 45000.00));
employees.add(new Employee("Jim", "Taubitz", 65000.00));
employees.add(new Employee("Jeff", "Nichols", 6522.45));
XmlHelper helper = new XmlHelper();
try
{
trace("Testing save");
helper.saveEmployeeList(employees);
trace("Testing load");
// try to read the data
List
if(employees.size() != list.size())
{
fail("Sizes don't match for test data vs actual data");
}
else
{
String msg = "";
boolean fSuccess = true;
for(int i = 0; i < employees.size(); i++)
{
Employee expected = employees.get(i);
Employee actual = list.get(i);
if(!expected.equals(actual))
{
fSuccess = false;
msg += String.format(
"Expected: %s, found %s not equal ",
expected.toString(), actual.toString());
}
}
if(!fSuccess)
{
fail("Error comparing expected with actual data " + msg);
}
}
}
catch(Exception e)
{
e.printStackTrace();
}
}
/**
* This test verifies the XmlHelper creates the XML
* IAW with the specified schema
*
*/
@Test
public void testXmlSchemaValidation()
{
String schemaFileName = "src/week03/employees.xsd";
File file = new File(schemaFileName);
try
{
trace(file.getCanonicalPath());
}
catch(IOException e1)
{
// TODO Auto-generated catch block
e1.printStackTrace();
}
String xmlFileName = "employees.xml";
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
dbFactory.setValidating(true);
try
{
// define the type of schema - we use W3C:
String schemaLang = "http://www.w3.org/2001/XMLSchema";
// get validation driver:
SchemaFactory factory = SchemaFactory.newInstance(schemaLang);
// create schema by reading it from an XSD file:
Schema schema = factory.newSchema(new StreamSource(schemaFileName));
Validator validator = schema.newValidator();
// at last perform validation:
validator.validate(new StreamSource(xmlFileName));
}
catch(IllegalArgumentException x)
{
String msg = "Error: JAXP DocumentBuilderFactory attribute "
+ "not recognized: " + JAXP_SCHEMA_LANGUAGE + " " + x.getMessage();
trace(msg);
trace("Check to see if parser conforms to JAXP spec.");
fail(msg);
}
catch(SAXException ex)
{
String msg = "Error: SAXException: " + ex.getMessage();
trace(msg);
fail(msg);
}
catch(IOException e)
{
e.printStackTrace();
}
}
/**
* Writes message to standard out
*
* @param msg
* The message to write
*/
private void trace(String msg)
{
System.out.println(msg);
}
static final String JAXP_SCHEMA_LANGUAGE = "http://java.sun.com/xml/jaxp/properties/schemaLanguage";
static final String JAXP_SCHEMA_SOURCE = "http://java.sun.com/xml/jaxp/properties/schemaSource";
static final String W3C_XML_SCHEMA = "http://www.w3.org/2001/XMLSchema";
}
/***********************/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
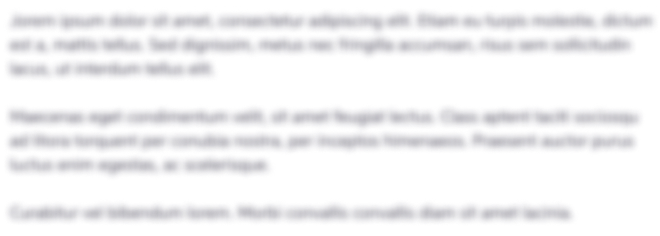
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started