Question
Hello, I need to make library program. problem is given as below followed by code I've got so far. Not sure why it's not working
Hello, I need to make library program. problem is given as below followed by code I've got so far. Not sure why it's not working as it should be.
we are supposed to write two modules(book and library)
When you scroll down, you will see my code. Please help me how to fix my code in library module. I think two functions (initialize and display) are the problem. Please help me!!!
The Library Module
Private Member Variables (properties)
m_name
This module will hold the name of the library up to 30 characters in a Cstring of 31 characters.
m_books
A Book pointer to hold a dynamic array of Book objects.
m_sizeOfBooksArray
An integer to hold the size of the dynamic array of books
m_addedBooks
An integer to keep track of the number of books that are set to valid values in the books array.
Private Member functions
void setEmpty(); setEmpty sets the name to an empty Cstring and all the other properties to zero and nullptr.
bool isEmpty()const; Returns true if the Library is in a safe invalid empty state
void header(const char* title)const; Prints the header of the loaned books report as follows:
prints dashes ('-') 78 times and goes to a new line prints "** ", library name and " **" then goes to a new line prints the title argument and goes to a new line prints dashes ('-') 78 times and goes to a new line prints "Row Book title SKU loan days penalty" and goes to a new line
prints "--- ------------------------------------------------ ------- --------- -------" and goes to a new line
void footer()const; prints dashes ('-') 78 times and goes to a new line
Public Member functions
void initialize(const char* name, int noOfBooks); If the name is not null and not an empty Cstring and noOfBooks is greater than zero, it will:
copy the name into the library name set the size of books array to the noOfBooks allocate a dynamic array of Books to the noOfBooks. (if the allocation fails it will set the Library into a safe empty state) sets the added books member vairable to zero. Otherwise, it will set the Library into a safe empty state.
bool addBook(const char* book_title, int sku, int loanDays); If the number of added books is less than the size of the books array, it will use the set method of the book to set the values of the next book in the array to the incoming arguments. If after setting the book, the newly added book is valid (not in a safe empty state) it will add one to the number of books and return true.
In all the other cases it will return false.
void clear(); Releases the allocated memory and sets the books pointer to nullptr.
void display(const char* substr); This function performs the following only if the Library is not in a safe empty state, otherwise, it will print "Invalid Library", goes to a new line and exits the function. defines an integer for row number and sets it to one. defines a search flag and sets it to false;
prints ">>> Searching for: \"" prints the substr prints "\" <<<" and goes to a new line calls header function with "Substring search" as an argument In a loop to the number of added books it will print the elements of the books array as follows (with a condition): if the subTitle function of the book element returns true having the substr as an argument the function will print: in 4 spaces, left-justified it will print the row number and add one to it. calls the display of the book element. sets the search flag to true If the search flag is false it will print: "No book title contains \"" substr value "\"" and goes to a new line prints the footer void display(bool overdueOnly=false)const; This function performs the following only if the Library is not in a safe empty state, otherwise, it will print "Invalid Library", goes to a new line and exits the function.
defines an integer for row number and sets it to one.
prints the header with one of the following titles: overdueOnly is true: "Overdue Books" overdueOnly is false: "Books on Loan" In a loop to the number of added books it will print the elements of the books array as follows (with the following condition): if the overdueOnly is true, it will only print those books that are overdue (hasPenalty()) otherwise it will print them all. in 4 spaces, left-justified it will print the row number and add one to it. calls the display of the book element. prints the footer
*************MY CODE*****************
>>library.h
#ifndef SDDS_LIBRARY_H
#define SDDS_LIBRARY_H
namespace sdds
{
class Library
{
char m_name[51];
int *m_books;
int m_sizeOfBooksArray;
int m_addedBooks;
void setEmpty();
bool isEmpty()const;
void header(const char* title)const;
void footer()const;
public:
void initialize(const char* name, int noOfBooks);
bool addBook(const char* book_title, int sku, int loanDays);
void clear();
void display(const char* substr);
void display(bool overdueOnly = false)const;
};
}
#endif // !SDDS_LIBRARY_H
>>library.cpp
#define _CRT_SECURE_NO_WARNINGS
#include
#include
using namespace std;
#include "library.h"
#include "Book.h"
namespace sdds
{
Books B;
void Library::setEmpty()
{
m_name[0] = 0;
m_books = nullptr;
m_sizeOfBooksArray = 0;
m_addedBooks = 0;
}
bool Library::isEmpty()const
{
if ((m_name[0] == 0) && (m_books == nullptr) && (m_sizeOfBooksArray == 0) && (m_addedBooks == 0))
{
return true;
}
return false;
}
void Library::header(const char* title)const
{
footer();
cout << "** " << m_name << " **" << endl;
cout << title << endl;
footer();
cout << endl;
cout << "Row Book title SKU loan days penalty" << endl;
cout << "--- ------------------------------------------------ ------- --------- -------" << endl;
}
void Library::footer()const
{
for (int i = 0; i < 78; i++)
{
cout << "-";
}
cout << endl;
}
void Library::initialize(const char* name, int noOfBooks)
{
if (name != nullptr && name[0] != 0 && noOfBooks > 0)
{
strcpy(m_name,name);
// set the size of books array to noOfBooks
m_sizeOfBooksArray = noOfBooks;
// allocate dynamic array of Books to noOfBooks
m_books = new int[noOfBooks + 1];
if (m_books != nullptr)
{
for (int i=0;i< noOfBooks;i++)
{
m_books[i] = name[i];
}
m_addedBooks = 0;
}
}
setEmpty();
}
bool Library::addBook(const char* book_title, int sku, int loanDays)
{
if (m_addedBooks < m_sizeOfBooksArray)
{
// use set method of book to set values of next book in array to incoming arguments
B.set(book_title,sku,loanDays);
// if newly added book is valid
if (!isEmpty())
{
m_addedBooks++;
return true;
}
}
return false;
}
void Library::clear()
{
delete[] m_books;
m_books = nullptr;
}
void Library::display(const char* substr)
{
if (!isEmpty())
{
cout << ">>> Searching for: \"" << substr << "\" <<<" << endl;
header("Substring search");
for (int i = 0; i < m_addedBooks; i++)
{
if (B.subTitle(substr))
{
cout.fill(' ');
cout.width(4);
cout.setf(ios::left);
cout << i + 1;
B.display();
// sets the search flag to true
}
else
{
cout << "No book title contains \"" << substr << "\"" << endl;
}
}
footer();
}
else
{
cout << "Invalid Library" << endl;
}
}
void Library::display(bool overdueOnly)const
{
if (!isEmpty())
{
if (!overdueOnly)
{
header("Overdue Books");
for (int i = 0; i < m_addedBooks; i++)
{
// only print books that are overdue(hasPenalty())
if (B.hasPenalty())
{
cout.fill(' ');
cout.width(4);
cout.setf(ios::left);
cout << m_books[i];
B.display();
}
}
}
else
{
header("Books on Loan");
for (int i = 0; i < m_addedBooks; i++)
{
cout << i + 1 << m_books[i];
}
}
footer();
}
else
{
cout << "Invalid Library" << endl;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
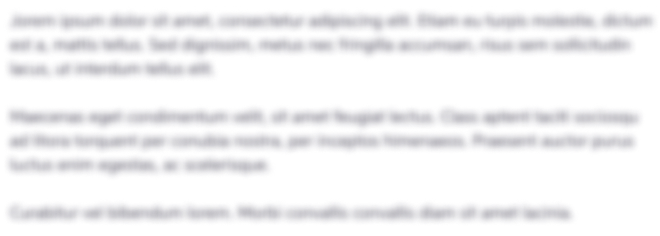
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started