Question
Hello, I would like some help with fixing my errors in my code, please. The text file I use is called 'stockData.txt' and this is
Hello, I would like some help with fixing my errors in my code, please.
The text file I use is called 'stockData.txt' and this is the information it contains.
ABC 123.45 130.95 132.00 125.00 120.50 10000 MSET 120.00 140.00 145.00 140.00 115.00 30920 AOLK 80 75 82 74 83 5000 CSCO 100 102 105 98 101 25000 IBD 68 71 72 67 75 15000
myString.h:
-----------------------------------------------------------------
#ifndef MYSTRING_H #define MYSTRING_H
#include using namespace std;
class newString{ public: const newString& operator=(const newString&); //overload the assignment operator newString(const char*); //constructor; conversion from the char string newString(); //default constructor to initialize the string to nullptr newString(const newString&); //copy constructor ~newString(); //destructor char &operator[] (int); const char &operator[](int) const; //overloading the relational operators bool operator==(const newString&) const; bool operator!=(const newString&) const; bool operator<=(const newString&) const; bool operator<(const newString&) const; bool operator>=(const newString&) const; bool operator>(const newString&) const; private: char* strcopy(const char *str2); char* strcopy(char *str1, const char *str2); char *strPtr; //pointer to the char array //that holds the string int strLength; //variable to store the length //of the string };
#endif /* MYSTRING_H */
-----------------------------------------------------------------
myStringImp.cpp
-----------------------------------------------------------------
//implementing file myStringImp.cpp #include #include #include #include #include "myString.h"
using namespace std;
//constructor: conversion from the char string to newString newString::newString(const char *str){ strLength = strlen(str); strPtr = new char[strLength + 1]; //allocate memory to store char string strcopy(str); }
//default constructor to store the null string newString::newString(){ strLength = 0; strPtr = new char[1]; strPtr[0] = '\0'; }
//copy constructor newString::newString(const newString& rightStr){ strLength = rightStr.strLength; strPtr = new char[strLength + 1]; strcopy(rightStr.strPtr); }
newString::~newString(){ //destructor delete [] strPtr; }
//overload the assignment operator const newString& newString::operator=(const newString& rightStr){ if(this != &rightStr){ //avoid self copy delete [] strPtr; strLength = rightStr.strLength; strPtr = new char[strLength + 1]; strcopy(rightStr.strPtr); } return *this; }
char& newString::operator[] (int index){ assert(0 <= index && index < strLength); return strPtr[index]; }
const char& newString::operator[](int index) const{ assert(0 <= index && index < strLength); return strPtr[index]; }
bool newString::operator==(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) == 0); }
bool newString::operator<(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) < 0); }
bool newString::operator<=(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) <= 0); }
bool newString::operator>(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) > 0); }
bool newString::operator>=(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) >= 0); }
bool newString::operator!=(const newString& rightStr) const{ return (strcmp(strPtr, rightStr.strPtr) != 0); }
//overload the stream extraction operator << ostream& operator << (ostream& osObject, const newString& str){ osObject << str.strPtr; return osObject; }
//overload the stream extraction operator >> ostream& operator >> (istream& isObject, const newString& str){ char temp[81]; isObject >> setw(81) >> temp; str = temp; return isObject; }
char* newString::strcopy(const char *str){ for(int i = 0; i < strLength; i++){ srePtr[i] = str[i]; } strPtr[strLength] = '\0'; return strPtr; }
char* newString::strcopy(char *str1, const char *str2){ for (usigned int i = 0; i < strlen(str1); i++){ str1[i] = str2[i]; } str1[strlen(str1)] = '\0'; return str1; }
-------------------------------------------------------------------
stockListType.h
------------------------------------------------------------------
#ifndef STOCKLISTTYPE_H #define STOCKLISTTYPE_H
#include #include
#include "stockType.h"
using namespace std;
class stockListType { public: void printBySymbol(); void printByGain(); void printReports(); void sortStockList(); void sortByGain(); void insert(const stockType& item); private: vector indexByGain; vector list; };
#endif /* STOCKLISTTYPE_H */
------------------------------------------------------------
stockType.h
------------------------------------------------------------
#ifndef STOCKTYPE_H #define STOCKTYPE_H
#include #include #include
using namespace std;
class stockType { friend ostream& operator<<(ostream&, const stockType&); friend ifstream& operator>>(ifstream&, stockType&);
public: void setStockInfo(string symbol, double openPrice, double closeProce, double high, double Low, double prevClose, int shares); string getSymbol(); double getPercentChange(); double getOpenPrice(); double getClosePrice(); double getHighPrice(); double getLowPrice(); double getPrevPrice(); int getnoOfShares();
stockType(); stockType(string symbol, double openPrice, double closeProce, double high, double Low, double prevClose, int shares);
bool operator ==(const stockType& other) const; bool operator !=(const stockType& other) const; bool operator <=(const stockType& other) const; bool operator >=(const stockType& other) const; bool operator >(const stockType& other) const; bool operator <(const stockType& other) const;
private: string stockSymbol; double todayOpenPrice; double todayClosePrice; double todayHigh; double todayLow; double yesterdayClose; double percentChange; int noOfShares; };
#endif /* STOCKTYPE_H */
-------------------------------------------------------------------
stockTypeImp.cpp
-------------------------------------------------------------------
#include #include #include #include #include "stockType.h"
using namespace std;
void stockType::setStockInfo(string symbol, double openPrice, double closeProce, double high, double low, double prevClose, int shares) { stockSymbol = symbol; todayOpenPrice = openPrice; todayClosePrice = closeProce; todayHigh = high; todayLow = low; yesterdayClose = prevClose; percentChange = (todayClosePrice - yesterdayClose) / yesterdayClose; noOfShares = shares; }
string stockType::getSymbol() { return stockSymbol; }
double stockType::getPercentChange() { return percentChange; }
double stockType::getOpenPrice() { return todayOpenPrice; }
double stockType::getClosePrice() { return todayClosePrice; }
double stockType::getHighPrice() { return todayHigh; }
double stockType::getLowPrice() { return todayLow; }
double stockType::getPrevPrice() { return yesterdayClose; }
int stockType::getnoOfShares() { return noOfShares; }
stockType::stockType() { stockSymbol = ""; todayOpenPrice = 0; todayClosePrice = 0; todayHigh = 0; todayLow = 0; yesterdayClose = 0; percentChange = 0; noOfShares = 0; } stockType::stockType(string symbol, double openPrice, double closeProce, double high, double low, double prevClose, int shares) { stockSymbol = symbol; todayOpenPrice = openPrice; todayClosePrice = closeProce; todayHigh = high; todayLow = low; yesterdayClose = prevClose; percentChange = (todayClosePrice - yesterdayClose) / yesterdayClose; noOfShares = shares; }
bool stockType::operator ==(const stockType& other) const { return (stockSymbol == other.stockSymbol); }
bool stockType::operator !=(const stockType& other) const { return (stockSymbol != other.stockSymbol); }
bool stockType::operator <=(const stockType& other) const { return (stockSymbol <= other.stockSymbol); }
bool stockType::operator >=(const stockType& other) const { return (stockSymbol >= other.stockSymbol); }
bool stockType::operator >(const stockType& other) const { return (stockSymbol > other.stockSymbol); }
bool stockType::operator <(const stockType& other) const { return (stockSymbol < other.stockSymbol); }
ostream& operator<<(ostream& os, const stockType& stock) { os << setw(6) << stock.stockSymbol << " " << setw(6) << stock.todayOpenPrice << " " << setw(6) << stock.todayClosePrice << " " << setw(6) << stock.todayHigh << " " << setw(6) << stock.todayLow << " " << setw(6) << stock.yesterdayClose << " " << setw(8) << stock.percentChange * 100 << "% " << setw(10) << stock.noOfShares; return os; }
ifstream& operator>>(ifstream& inf, stockType& stock) { inf >> stock.stockSymbol>>stock.todayOpenPrice >> stock.todayClosePrice>>stock.todayHigh >> stock.todayLow>>stock.yesterdayClose >> stock.noOfShares; stock.percentChange = (stock.todayClosePrice - stock.yesterdayClose) / stock.yesterdayClose;
return inf; }
-----------------------------------------------------------------
main.cpp
----------------------------------------------------------------
#include #include #include #include #include #include "stockType.h" #include "stockListType.h"
using namespace std;
const int noOfStocks = 5;
void getData(stockListType& list);
int main() { stockListType stockList; cout << fixed << showpoint; cout << setprecision(2);
getData(stockList);
stockList.sortStockList(); stockList.printBySymbol(); cout << endl; stockList.printByGain();
return 0; }
void getData(stockListType& list) { ifstream infile; string symbol; double OpenPrice; double ClosePrice; double tHigh; double tLow; double yClose; int shares; stockType temp;
infile.open("stockdat.txt");
if (!infile) { cerr << "Input file does not exist. Program terminates." << endl; return; }
infile >> symbol; while (infile) { infile >> OpenPrice >> ClosePrice >> tHigh >> tLow >> yClose >> shares; temp.setStockInfo(symbol,OpenPrice,ClosePrice,tHigh,tLow,yClose,shares); list.insert(temp); infile >> symbol; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
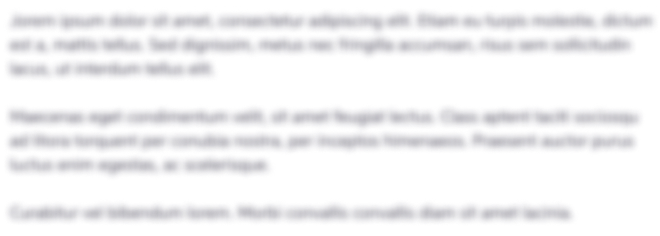
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started