Question
Hello, I would like to know how I can print out a calendar like shown in the picture from my code please. This is my
Hello, I would like to know how I can print out a calendar like shown in the picture from my code please.
This is my code:
-----------------------------------------------------------------------------------------------------
Copyable Code: -----------------------------------------------------------------------------------------------------
main.cpp #include "calendarType.h" #include
using namespace std;
int main() { int month; int year; int SENTINEL = -9999; int userSent = 0;
while (userSent != SENTINEL) {
cout > month; cout
cout > year; cout
calendarType cal(month, year); //for 2014, first day for each month, //6 is sunday //9 is monday //4 tuesday //10 wednesday //5 is thursday //8 is friday //11 is saturday
cal.printCalendar();
cout > userSent; cout
}
system("pause");
return 0; }
calendarType.h /* * This class will create a calendar printout for any month and year after * January 1, 1500. */ #ifndef calendarType_h #define calendarType_h #include "dateType.h" #include "extDateType.h" #include "dayType.h" #include
int getMonth(); int getYear();
void printCalendar();
calendarType(); calendarType(int m, int y);
private:
// Note that member functions can also be private which means only functions // within this class can call them. dayType firstDayOfMonth(); void printTitle(); void printDates();
// Composition rather than inheritance, although firstDate is a derived class // from the base class dateType. extDateType firstDate; dayType firstDay;
};
#endif
dateType.h
#ifndef dateType_H #define dateType_H class dateType { public: void setDate(int, int, int); void setMonth(int); void setDay(int); void setYear(int); void print() const;
int numberOfDaysPassed();
int numberOfDaysLeft();
void incrementDate(int nDays);
int getMonth() const; int getDay() const; int getYear() const;
int getDaysInMonth();
bool isLeapYear();
dateType(int = 1, int = 1, int = 1900);
private: int dMonth; int dDay; int dYear; };
#endif
dayType.h
#ifndef dayType_H #define dayType_H #include
class dayType { public: static string weekDays[7]; void print() const; string nextDay() const; string prevDay() const; void addDay(int nDays);
void setDay(string d); string getDay() const;
dayType(); dayType(string d);
private: string weekDay; };
#endif
extDateType.h
#ifndef extDateType_H #define extDateType_H #include
#include "dateType.h" using namespace std;
class extDateType: public dateType { public: static string eMonths[12]; void printLongDate(); void setDate(int, int, int); void setMonth(int m);
void printLongMonthYear();
extDateType(); extDateType(int, int, int);
private: string eMonth; };
#endif
dateTypeImp.cpp
#include
void dateType::setDate(int month, int day, int year) { if (year >= 1) dYear = year; else dYear = 1900; if (1
switch (dMonth) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: if (1
void dateType::setMonth(int m) { dMonth = m; }
void dateType::setDay(int d) { dDay = d; }
void dateType::setYear(int y) { dYear = y; }
void dateType::print() const { cout
int dateType::getMonth() const { return dMonth; }
int dateType::getDay() const { return dDay; }
int dateType::getYear() const { return dYear; }
int dateType::getDaysInMonth() { int noOfDays;
switch (dMonth) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: noOfDays = 31; break; case 4: case 6: case 9: case 11: noOfDays = 30; break; case 2: if (isLeapYear()) noOfDays = 29; else noOfDays = 28; }
return noOfDays; }
bool dateType::isLeapYear() { if (((dYear % 4 == 0) && (dYear % 100 != 0)) || dYear % 400 == 0) return true; else return false; }
dateType::dateType(int month, int day, int year) { setDate(month, day, year); }
int dateType::numberOfDaysPassed() { int monthArr[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int sumDays = 0; int i;
for (i = 1; i
if (isLeapYear() && dMonth > 2) sumDays = sumDays + dDay + 1; else sumDays = sumDays + dDay;
return sumDays; }
int dateType::numberOfDaysLeft() { if (isLeapYear()) return 366 - numberOfDaysPassed(); else return 365 - numberOfDaysPassed(); }
void dateType::incrementDate(int nDays) { int monthArr[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; int daysLeftInMonth;
daysLeftInMonth = monthArr[dMonth] - dDay;
if (daysLeftInMonth >= nDays) dDay = dDay + nDays; else { dDay = 1; dMonth++; nDays = nDays - (daysLeftInMonth + 1);
while (nDays > 0) if (nDays >= monthArr[dMonth]) { nDays = nDays - monthArr[dMonth];
if ((dMonth == 2) && isLeapYear()) nDays--; dMonth++; if (dMonth > 12) { dMonth = 1; dYear++; }
} else { dDay = dDay+nDays; nDays = 0; } } }
dayTypeImp.cpp
#include
string dayType::weekDays[7] = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}; void dayType::print() const { cout
string dayType::nextDay() const { int i;
for (i = 0; i
string dayType::prevDay() const { if (weekDay == "Sunday") return "Saturday"; else { int i;
for (i = 0; i
void dayType::addDay(int nDays) { int i;
for (i = 0; i
void dayType::setDay(string d) { weekDay = d; }
string dayType::getDay() const { return weekDay; }
dayType::dayType() { weekDay = "Sunday"; }
dayType::dayType(string d) { weekDay = d; }
extDateTypeImp.cpp
#include
void extDateType::printLongDate() { cout
void extDateType::printLongMonthYear() { cout
void extDateType::setDate(int m, int d, int y) { dateType::setDate(m, d, y);
eMonth = eMonths[m - 1]; }
void extDateType::setMonth(int m) { dateType::setMonth(m); eMonth = eMonths[m - 1]; }
extDateType::extDateType() { eMonth = "January"; }
extDateType::extDateType(int m, int n, int d) : dateType(m, n, d) { eMonth = eMonths[m - 1]; }
calendarTypeImp.cpp
#include "calendarType.h"
//defaults constructor to January 1, 1500 calendarType::calendarType() { setMonth(1); setYear(1500); }
//sets input month and year calendarType::calendarType(int m, int y) { setMonth(m); setYear(y); }
void calendarType::setMonth(int m) { firstDate.setMonth(m); }
void calendarType::setYear(int y) { firstDate.setYear(y); }
int calendarType::getMonth() { return firstDate.getMonth(); }
int calendarType::getYear() { return firstDate.getYear(); }
void calendarType::printCalendar() { printTitle(); printDates(); }
//private void calendarType::printTitle() { firstDate.printLongMonthYear();
cout
for (int i = 0; i != 8; i++) { string shortName = firstDay.weekDays[i].substr(0, 3);
cout
void calendarType::printDates() {
int i = 0; dayType day = firstDayOfMonth();
if (day.getDay() == "Sunday") {
while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
if (day.getDay() == "Monday")
{ // day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
if (day.getDay() == "Tuesday")
{
// day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
if (day.getDay() == "Wednesday")
{
// day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
if (day.getDay() == "Thursday")
{
// day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
if (day.getDay() == "Friday")
{
// day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
if (day.getDay() == "Saturday")
{
// day specific loop
cout
for (int k = 0; k
while (i
if (firstDate.getDay()
i++; }
//copypaste loop while (firstDate.getDay()
while (i
if (firstDate.getDay()
i++; } } cout
}
}
dayType calendarType::firstDayOfMonth() { dayType day; int countResult; int y = getYear(); int m = getMonth(); int d = 1;
if (firstDate.getMonth() == 1 && firstDate.getYear() == 1500) { day.setDay(firstDay.weekDays[1]); } else { static int t[] = { 0, 3, 2, 5, 0, 3, 5, 1, 4, 6, 2, 4 }; y -= m
day.setDay(firstDay.weekDays[countResult]); }
return day; }
HOMEWORK #16-Calendar Program Write a program to print the calendar for a particular month or a particular year. For example, the : calendar for February 2017 is Feburary 201'7 SunMon Tue Wed Thu Fri Sat 2 12 19 26 13 20 27 14 21 28 10 17 24 15 16 23 18 25 Specifications: To solve this problem, you will create a number of classes using inheritance and composition In Chapter 11 of Malik's book, he uses a class called dateType (see pages 774-776), which is designed to store the month, day, and year for calendar dates. Use the dateType class as the initial basis for your program. The class uses the following public and private members Public: void setDate (int month, int day, int year); int getDay) consti int getMonth () const; int getYear) const void printDate () const; dateType (int month-1, int day-l, int year-1900) Private int dMonth; int dDay int dYear; Create the class, and copy the definitions of the class member functions into an appropriate .cpp file. Next, modify the definitions of the function setDate () and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. If an incorrect month, day, or year is used, use the default values of 1, 1, and 1900 respectively. Add a new public member function, isLeapYear ), to check weather a year is a leap yearStep by Step Solution
There are 3 Steps involved in it
Step: 1
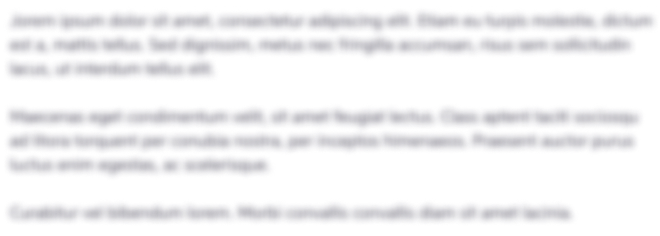
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started