Question
(HELLO, NEED HELP CODING THIS IN C++, I AM HAVING A HARD TIME. THANK YOU.) Purpose The purpose of this lab is to practice overloading
(HELLO, NEED HELP CODING THIS IN C++, I AM HAVING A HARD TIME. THANK YOU.)
Purpose
The purpose of this lab is to practice overloading operators, using header files and creating a static data member.
Deliverables
A Word document with a copy of the completed code and screenshots showing the console window with the program running. Use Alt+Print Screen so only the console window is copied.
The zipped Project folder.
Scenario/Summary
This program will create a class called Time that has hours and minutes. We will overload operators to be able to add times, subtract times, product a negative time, be able to add one hour with ++ operator and print out the time with << operator. We will also use a static counter to keep track of how many time objects are created in the program and a static method to print the counter. We will put our class definition in a header file; the class code will be in a separate header file from the main function code.
Step 1
Create a C++ Console Application project and name it CIS247_Lab3_YourLastName.
In the Project menu, select Add Class. In the window that pops up, select C++ Class and click Add. In the next window, enter a class name of: Time. You will see that two files will be created: Time.h and Time.cpp. Click Finish to create the files.
In the Time.h file, you will see a default constructor and destructor are provided. Use this UML to create the basic header file and .cpp file for the Time class; the information on the overloaded operators will be added later. Be sure to put all code in the Time.cpp file including initializing the static counter attribute.
Time |
-hours : int -minutes: int -counter : static int //initialize to zero in the code file |
+Time(int h = 0, int m = 0) //this will be the only constructor. It has default parameters that are set to 0; remove the default constructor provided. Code should set attributes to parameters; increment the counter variable by one. +~Time() //destructor prototype; +setHours(int h) : void +setMinutes(m): void +getHours() : int +getMinutes() : int +getCounter() : static int //static method that returns the value of counter |
Step 2
We will overload some operators to work with our Time objects:
Operator | Description | Example |
+ | addition | time3 = time1 + time2; |
- | subtraction | time3 = time1 time2; |
- | negation | time2 = -time1; //negative |
++ | post-increment (add 1 hour) | time++; |
= | assignment | time1 = time2; |
<< | output stream operator | cout << time << endl; |
DIRECTIONS:
For each of these overloaded operators, create a prototype in the Time.h header file and put the code in the Time.cpp file.
For the addition, you want the operator+ method to return a Time object. It will receive a Time object as a parameter (time2); the code should create a time3 object and set its hours to this->hours plus time2.hours. The same for minutes. Add code to adjust data in time3 if the minutes are 60 or above. Then return the time3 object. (example: if after adding you have 4 hours and 75 minutes, this should be adjusted to 5 hours and 15 minutes).
Similarly for subtraction, you want the operator- method to return a Time object. It will receive a Time object as a parameter (time2); the code should create a time3 object and set its hours to this->hours minus time2.hours. The same for minutes. Add code to adjust data in time3 if needed. Then return the time3 object. (example: if after subtracting you have 2 hours and -10 minutes, this should be adjusted to 1 hour and 50 minutes.)
HERE IS SOME HELP:
voidTime::adjustTime(Time &tm)
{
inttotalTime;
totalTime = (tm.hours * 60) + (tm.minutes);
tm.hours = totalTime / 60;
tm.minutes = totalTime - (tm.hours * 60);
}
For the unary minus sign, negation, you want to create an overloaded operator- method that takes no parameters but returns a Time object. The code will create a time2 object and set its hours to this->hours and the minutes to this->minutes; return the time2 object.
For the post-increment++ sign, you want the operator++ method to be void as a return type. It receives no parameters. The code simply adds one to the hours attribute.
For the assignment operator, you want the operator= method to have a void return. It receives a Time parameter (time2). The code sets this->hours equal to time2.hours; same for minutes.
For the << output object, follow the example in MyProgrammingLab 14.5 11226. The video shows how to code this, but here is some information. The return type for the operator<< method is: friend ostream&. Only use the word friend in the header file. Also note that in the Time.cpp files, you do not put Time:: in front of the operator<< in this case! There will be 2 parameters: ostream &output and const Time &time. The code will look like this:
output << [see note A below]; return output;
Note A: create a normal cout stream with text strings and variables, substituting time.hours and time.minutes for the numbers shown:
The time is 6 hours and 17 minutes.
Step 3
Create a main method in a separate .cpp file to test your class. Suggestions: create three time objects named t1, t2 and t3. Pass some actual numbers to t1 and t2 when you create them.
Make your output meaningful. Here is just a sample output but yours can look different. Just be sure to test each overloaded operator and show the results afterwards. To print times, remember because we overloaded the << operator, you can just put:
cout << t1 << endl;
IMPORTANT: At the very end of your program, print out the count of all objects created using the static method, getCounter without an object (Time::getCounter()).
SUGGESTION: either add these overloaded operators one at a time and test each one or comment out the ones you havent tested yet. Things will go a lot smoother.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
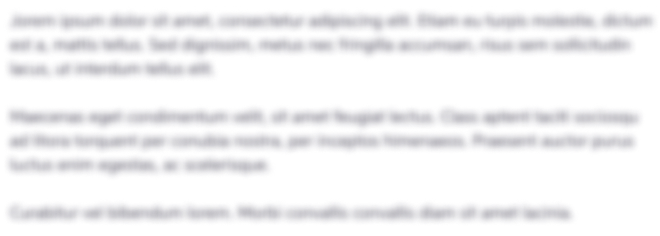
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started