Question
hello please can you help me with the following JAVA CODING Specifications You must define a class named MediaRentalManager (we did not provide it) that
hello please can you help me with the following JAVA CODING
Specifications
You must define a class namedMediaRentalManager(we did not provide it) that implements the MediaRentalManagerInt interface functionality. You must define classes that support the functionality specified by the interface. The following specifications are associated with the project:
- You should study the public tests and the output (files with .txt extension) to familiarize yourself with the expected system functionality.
- Define a class namedMediaRentalManager. Feel free to add any instance variables you understand are needed or any private methods. Do not add any public methods (beyond the ones specified in the MediaRentalManagerInt interface).
- The media rental system keeps track of customers and media (movies and music albums). A customer has a name, address, a plan and two lists (queues). One queue represent the media the customer is interested in receiving and the second one represents the media already received (rented) by the customer. There are two plans a customer can have: UNLIMITED and LIMITED. UNLIMITED allows a customer to receive as many media as they want; LIMITED restricts the media to a default value of 2 (this value can be change via a manager method). A movie has a title, a number of copies available and a rating (e.g., "PG"). An album has a title, number of copies available, an artist and the songs that are part of the album.
- You must define and use at least three classes / interfaces (not including MediaRentalManager) as part of your design.These clases must support the functionality of the system, otherwise you will not receive any credit.
- The database for your system needs to be represented using two ArrayList objects. One ArrayList will represent the customers present in the database; the second will represent the media (movies and albums). This is the ArrayList requirement provided in theGradingsection.
- You must define javadoc for the classes you implement. You do not need to provide javadoc comments for the MediaRentalManager class. Notice you do not need to run the javadoc utility.
- Regarding the searchMedia method: the songs parameter represents a substring (fragment) or the full list of songs associated with the album. If the full list is provided, you can assume commas will be part of the string.Hint:you may want to consider using the indexOf method of the String class.
- Feel free to use Collections.sort to sort your data.
- Although you are not require to write student tests you are encourage to do so.
- Not all the details associated with the project can be fully specified in this description. The sooner you start working on the project the sooner you will be able to address any doubts you may have.
mediaRentalManager
Interface MediaRentalManagerInt
public interface MediaRentalManagerInt
- Interface that defines the functionality expected from the media rental manager. The two possible media we can have are movies and music albums. A movie has a title, a number of copies that are available for rent, and a rating (e.g., "PG"). An album has a title, a number of copies, an artist, and a list of songs (String with title of songs separated by commas).
IMPORTANT:The database of the media rental manager, must define and use two ArrayList. One stores the media (both Movies and Album information) and one stores Customer information. You will lose significant credit if you do not define and use these ArrayList objects.
- Method Summary
- All MethodsInstance MethodsAbstract Methods
- Modifier and TypeMethod and Description
. voidaddAlbum(java.lang.String title, int copiesAvailable, java.lang.String artist, java.lang.String songs)
- Adds the specified album to the database.
- voidaddCustomer(java.lang.String name, java.lang.String address, java.lang.String plan)
- Adds the specified customer to the database.
- voidaddMovie(java.lang.String title, int copiesAvailable, java.lang.String rating)
- Adds the specified movie to the database.
- booleanaddToQueue(java.lang.String customerName, java.lang.String mediaTitle)
- Adds the specified media title to the queue associated with a customer.
- java.lang.StringgetAllCustomersInfo()
- Returns information about the customers in the database.
- java.lang.StringgetAllMediaInfo()
- Returns information about all the media (movies and albums) that are part of the database.
- java.lang.StringprocessRequests()
- Processes the requests queue of each customer.
- booleanremoveFromQueue(java.lang.String customerName, java.lang.String mediaTitle)
- Removes the specified media title from the customer's queue.
- booleanreturnMedia(java.lang.String customerName, java.lang.String mediaTitle)
- This is how a customer returns a rented media.
- java.util.ArrayListsearchMedia(java.lang.String title, java.lang.String rating, java.lang.String artist, java.lang.String songs)
- Returns a SORTED ArrayList with media titles that satisfy the provided parameter values.
- voidsetLimitedPlanLimit(int value)
- This set the number of media associated with the LIMITED plan.
- Method Detail
- addCustomer
void addCustomer(java.lang.String name, java.lang.String address, java.lang.String plan)
- Adds the specified customer to the database. The address is a physical address (not e-mail). The plan options available are:LIMITEDandUNLIMITED. LIMITED defines a default maximum of two media that can be rented.
- Parameters:
- name -
- address -
- plan -
- addMovie
void addMovie(java.lang.String title, int copiesAvailable, java.lang.String rating)
- Adds the specified movie to the database. The possible values for rating are "PG", "R", "NR".
- Parameters:
- title -
- copiesAvailable -
- rating -
- addAlbum
void addAlbum(java.lang.String title, int copiesAvailable, java.lang.String artist, java.lang.String songs)
- Adds the specified album to the database. The songs String includes a list of the title of songs in the album (song titles are separated by commas).
- Parameters:
- title -
- copiesAvailable -
- artist -
- songs -
- setLimitedPlanLimit
void setLimitedPlanLimit(int value)
- This set the number of media associated with the LIMITED plan.
- Parameters:
- value -
- getAllCustomersInfo
java.lang.String getAllCustomersInfo()
- Returns information about the customers in the database. The information is presented sorted by customer name. See the public tests for the format to use.
- Returns:
- getAllMediaInfo
java.lang.String getAllMediaInfo()
- Returns information about all the media (movies and albums) that are part of the database. The information is presented sorted by media title. See the public tests for the format to use.
- Returns:
- addToQueue
boolean addToQueue(java.lang.String customerName, java.lang.String mediaTitle)
- Adds the specified media title to the queue associated with a customer.
- Parameters:
- customerName -
- mediaTitle -
- Returns:
- false if the mediaTitle is already part of the queue (it will not be added)
- removeFromQueue
boolean removeFromQueue(java.lang.String customerName, java.lang.String mediaTitle)
- Removes the specified media title from the customer's queue.
- Parameters:
- customerName -
- mediaTitle -
- Returns:
- false if removal failed for any reason (e.g., customerName not found)
- processRequests
java.lang.String processRequests()
- Processes the requests queue of each customer. The customers will be processed in alphabetical order. For each customer, the requests queue will be checked and media will be added to the rented queue, if the plan associated with the customer allows it, and if there is a copy of the media available. For UNLIMITED plans the media will be added to the rented queue always, as long as there are copies associated with the media available. For LIMITED plans, the number of entries moved from the requests queue to the rented queue will depend on the number of currently rented media, and whether copies associated with the media are available.
- For each media that is rented, the following message will be generated:
- "Sending [mediaTitle] to [customerName]"
- Returns:
- returnMedia
boolean returnMedia(java.lang.String customerName, java.lang.String mediaTitle)
- This is how a customer returns a rented media. This method will remove the item from the rented queue and adjust any other values that are necessary (e.g., copiesAvailable)
- Parameters:
- customerName -
- mediaTitle -
- Returns:
- searchMedia
java.util.ArrayList searchMedia(java.lang.String title, java.lang.String rating, java.lang.String artist, java.lang.String songs)
- Returns a SORTED ArrayList with media titles that satisfy the provided parameter values. If null is specified for a parameter, then that parameter should be ignore in the search. Providing null for all parameters will return all media titles.
- Parameters:
- title -
- rating -
- artist -
- songs -
- Returns:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
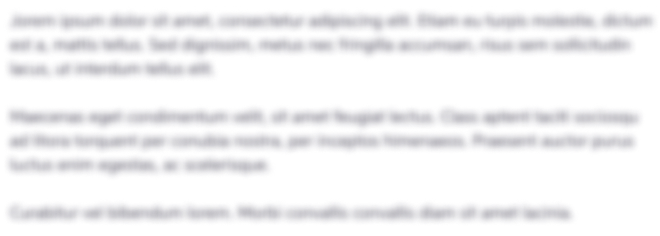
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started