Question
Hello Please READ THE INSTRUCTIONS CAREFULLU AND DRAW the DIAGRAM CLEARLY AND CORRECTLY . The required code for the question is given in C++ Instructions
Hello Please READ THE INSTRUCTIONS CAREFULLU AND DRAW the DIAGRAM CLEARLY AND CORRECTLY . The required code for the question is given in C++
Instructions In this assignment you will essentially convert the program you created in Assignment 1 into a program written in C++ using classes and object oriented design principles. A large part of this program has been provided to you to build from. It was designed using the modelviewcontroller design pattern that you should be familiar with. The various classes are described below. Controller The ShopController class acts as the controller for this program. It is the only class used by our main function (in main.cc). It maintains the model as well as the view. It prompts both the user for information and displays information via the View class (described below) and modifies the various model classes as appropriate. View The View class as the view for this program. All interaction with the user (including both input and output) is done using the view. These actions are prompted by the controller who then modifies the model in response. Model The Model is made up of a number of different classes. The Shop class will collect all of the objects that make up this automotive business. Currently it will only contain a list of customers ( Customer class). Each of these customers will contain a list of vehicles ( Vehicle class). These collections are stored in their own respective collection classes ( CustomerArray and VehicleArray classes, respectively). Task 1: UML Diagram You will first study the provided code to get an understanding of how all of the classes work together. Based solely on what is provided, draw a UML diagram for this program. Refer to the lecture notes for exactly what is expected to be included and omitted on a UML diagram. This diagram (a scan of a hand drawn diagram is acceptable) will be a part of your assignment submission.
REQUIRED CODE FOR PROGRAM
VIEW.CC
#include
#include "View.h" #include "CustomerArray.h" #include "Customer.h" #include "VehicleArray.h" #include "Vehicle.h"
void View::mainMenu(int& choice) { string str;
choice = -1;
cout<< " **** Toby's Auto Mechanic Information Management System **** "; cout<< " MAIN MENU "; cout<< " 1. Print Customer Database "; cout<< " 0. Exit ";
while (choice < 0 || choice > 1) { cout << "Enter your selection: "; choice = readInt(); }
if (choice == 0) { cout << endl; } }
void View::printCustomers(CustomerArray& arr) { cout << endl << "CUSTOMERS: "<< endl << endl;
for (int i = 0; i < arr.getSize(); i++) { Customer* cust = arr.get(i); ostringstream name; name << cust->getFname() << " " << cust->getLname();
cout << "Customer ID " << cust->getId() << endl << endl << " Name: " << setw(40) << name.str()<< endl << " Address: " << setw(37) << cust->getAddress() << endl << " Phone Number: " << setw(32)<< cust->getPhoneNumber() << endl; if (cust->getNumVehicles() > 0) { cout << endl << " " << cust->getNumVehicles() << " vehicle(s): " << endl << endl; }
VehicleArray& varr = cust->getVehicles(); for (int j = 0; j < varr.getSize(); j++) { Vehicle* v = varr.get(j); ostringstream make_model; make_model << v->getMake() << " " << v->getModel();
cout << "\t" << j+1 << ") " << setw(7)<< v->getColour() << " " << v->getYear() << " " << setw(17) << make_model.str() << " (" << v->getMilage() << "km)" << endl; }
cout << endl<< endl; } }
void View::pause() { string str;
cout << "Press enter to continue..."; getline(cin, str); }
int View::readInt() { string str; int num;
getline(cin, str); stringstream ss(str); ss >> num;
return num; }
VIEW.H
#ifndef VIEW_H #define VIEW_H
#include "CustomerArray.h"
class View {
public: void mainMenu(int&); void printCustomers(CustomerArray&); void pause();
private: int readInt(); };
#endif
VEHICLEARRAY.H
#ifndef VEHICLEARRAY_H #define VEHICLEARRAY_H
#include "defs.h" #include "Vehicle.h"
class VehicleArray { public: VehicleArray(); ~VehicleArray(); int add(Vehicle*); Vehicle* get(int); int getSize(); private: Vehicle* elements[MAX_VEHICLES]; int size; };
#endif
VEHICLEARRAY.CC
#include "VehicleArray.h" #include "Vehicle.h" #include "defs.h"
VEHIICLE.H
#ifndef VEHICLE_H #define VEHICLE_H
#include
class Vehicle {
public: Vehicle(string, string, string, int, int); string getMake(); string getModel(); string getColour(); int getYear(); int getMilage();
private: string make; string model; string colour; int year; int mileage; };
#endif
VEHICLE.CC
#include "Vehicle.h"
SHOPCONTROLLER.H
#ifndef SHOPCONTROLLER_H #define SHOPCONTROLLER_H
#include "View.h" #include "Shop.h"
class ShopController {
public: ShopController(); void launch(); private: Shop mechanicShop; View view; void initCustomers(); };
#endif
SHOPCONTROLLER.CC
#include "ShopController.h"
ShopController::ShopController() {
initCustomers();
}
void ShopController::launch() {
int choice;
while (1) { choice = -1; view.mainMenu(choice); if (choice == 1) { view.printCustomers(mechanicShop.getCustomers()); view.pause(); } /*else if (choice == 2) {
} else if (choice == 3) {
} else if (choice == 4) { } ... */ else { break; } } }
void ShopController::initCustomers() {
//add data fill here
}
SHOP.H
#ifndef SHOP_H #define SHOP_H
#include "Customer.h" #include "CustomerArray.h"
class Shop{
public: int addCustomer(Customer*); Customer& getCustomer(int); CustomerArray& getCustomers();
private: CustomerArray customers;
};
#endif
SHOP.CC
#include "Shop.h" #include "defs.h"
int Shop::addCustomer(Customer* c) { return customers.add(c); }
Customer& Shop::getCustomer(int i) { return *(customers.get(i)); }
CustomerArray& Shop::getCustomers() { return customers; }
MAKEFILE
OBJ = main.o ShopController.o View.o Shop.o CustomerArray.o VehicleArray.o Customer.o Vehicle.o
mechanicshop: $(OBJ) g++ -o mechanicshop $(OBJ)
main.o: main.cc g++ -c main.cc
ShopController.o: ShopController.cc ShopController.h Shop.h View.h g++ -c ShopController.cc
View.o: View.cc View.h g++ -c View.cc
Shop.o: Shop.cc Shop.h CustomerArray.h g++ -c Shop.cc
CustomerArray.o: CustomerArray.cc CustomerArray.h Customer.h defs.h g++ -c CustomerArray.cc
VehicleArray.o: VehicleArray.cc VehicleArray.h Vehicle.h defs.h g++ -c VehicleArray.cc
Customer.o: Customer.cc Customer.h g++ -c Customer.cc
Vehicle.o: Vehicle.cc Vehicle.h g++ -c Vehicle.cc
clean: rm -f $(OBJ) mechanicshop
MAIN.CC
#include "ShopController.h"
int main(int argc, char* argv[]) { ShopController control;
control.launch();
return 0; }
DEFS.H
#ifndef DEFS_H #define DEFS_H
#define MAX_VEHICLES 4 #define MAX_CUSTOMERS 6
#define C_OK 0 #define C_NOK -1
#endif
CUSTOMERARRAY.H
#ifndef CUSTOMERARRAY_H #define CUSTOMERARRAY_H
#include "Customer.h"
class CustomerArray { public: CustomerArray(); ~CustomerArray(); int add(Customer*); Customer* get(int); int getSize(); private: Customer* elements[MAX_CUSTOMERS]; int size; };
#endif
CUSTOMERARRAY.CC
#include "CustomerArray.h" #include "Customer.h" #include "defs.h"
CUSTOMER.H
#ifndef CUSTOMER_H #define CUSTOMER_H
#include
class Customer {
public: Customer(string="", string="", string="", string=""); int getId(); string getFname(); string getLname(); string getAddress(); string getPhoneNumber(); int getNumVehicles(); VehicleArray& getVehicles(); int addVehicle(Vehicle*); private:
static int nextId; int id; string firstName; string lastName; string address; string phoneNumber; VehicleArray vehicles; };
#endif
CUSTOMER.CC
#include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
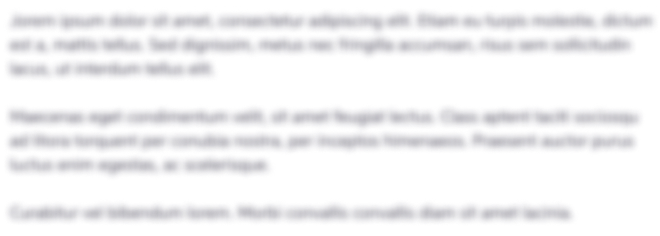
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started