Hello
Please solve this problem using c++ language.
will provide bignum.h below, please follow the instruction.
Thanks for your time!
SOURCE CODE:
#ifndef BIGNUM_H
#define BIGNUM_H
// main data structure to store the number of digits in the array digits
struct BigNum
{
int numDigits; // the number of digits
int *digits; // an array of numDigits digits
};
// the base cannot be greater than hexadecimal
const int BIGGEST_BASE = 16;
// given a character ch with a base, return its numerical equivalent
// precondition:
// 2
// postcondition:
// return ch as a number
// return -1 if not a valid digit in the base
int number(char ch, int base = 10);
// convert the digit x into a character
// precondition:
// 0
// postcondition:
// 0 -> '0' .... 10 ->'A' 11 -> 'B' .... 15 -> 'F'
char character(int x);
// allocate space for the zero constant as a BigNum
// and put the one digit 0 in it
BigNum *zeroConstant();
// read from standard input std::cin a number big number (read as a string)
// convert each character of the string read into a digit using the base (defaulted to 10)
// store all the digits read into a dynamic array in the struct BigNum without any leading zeros
//
// if the string read has invalid digits, output an error message and ask the user to try again
// until a successful number (string) is read
// precondition:
// 2
// postcondition:
// return a pointer to the new struct
// suggestion:
// the digits in the array are stored from least significant to most significant
BigNum *readBigNum(int base = 10);
// outputs a big number to standard output std::cout
void printBigNum(const BigNum *x);
// x plus y returning the sum as a pointer to a BigNum
// do the addition in base 'base'
// precondition:
// suggestion:
// the digits of x and y are stored from least significant to most significant
// postcondition:
// returned in the allocated BigNum the digits' sum and number of digits
BigNum *add(const BigNum *x, const BigNum *y, int base = 10);
// do x minus y returning the difference as a pointer to a BigNum
// do the subtraction in base 'base'
// precondition:
// x >= y so that the result will not be negative
// suggestion:
// the digits of x and y are stored from least significant to most significant
// postcondition:
// returned in the allocated BigNum the digits' difference and number of digits
BigNum *subtract(const BigNum *x, const BigNum *y, int base = 10);
// x times y returning the product as a pointer to a BigNum
// do the multiplication in base 'base'
// precondition:
// suggestion:
// the digits of x and y are stored from least significant to most significant
// postcondition:
// returned in the allocated BigNum the digits' product and number of digits
BigNum *multiply(const BigNum *x, const BigNum *y, int base = 10);
// deallocate the memory allocated for the BigNum struct and deallocate the
// memory of its digits array
// postcondition:
// x is the nullptr (x is passed by reference)
void deleteBigNum(BigNum *&x);
NOTES: These pictures are from the same problem. I copied and pasted the source code from the picture.
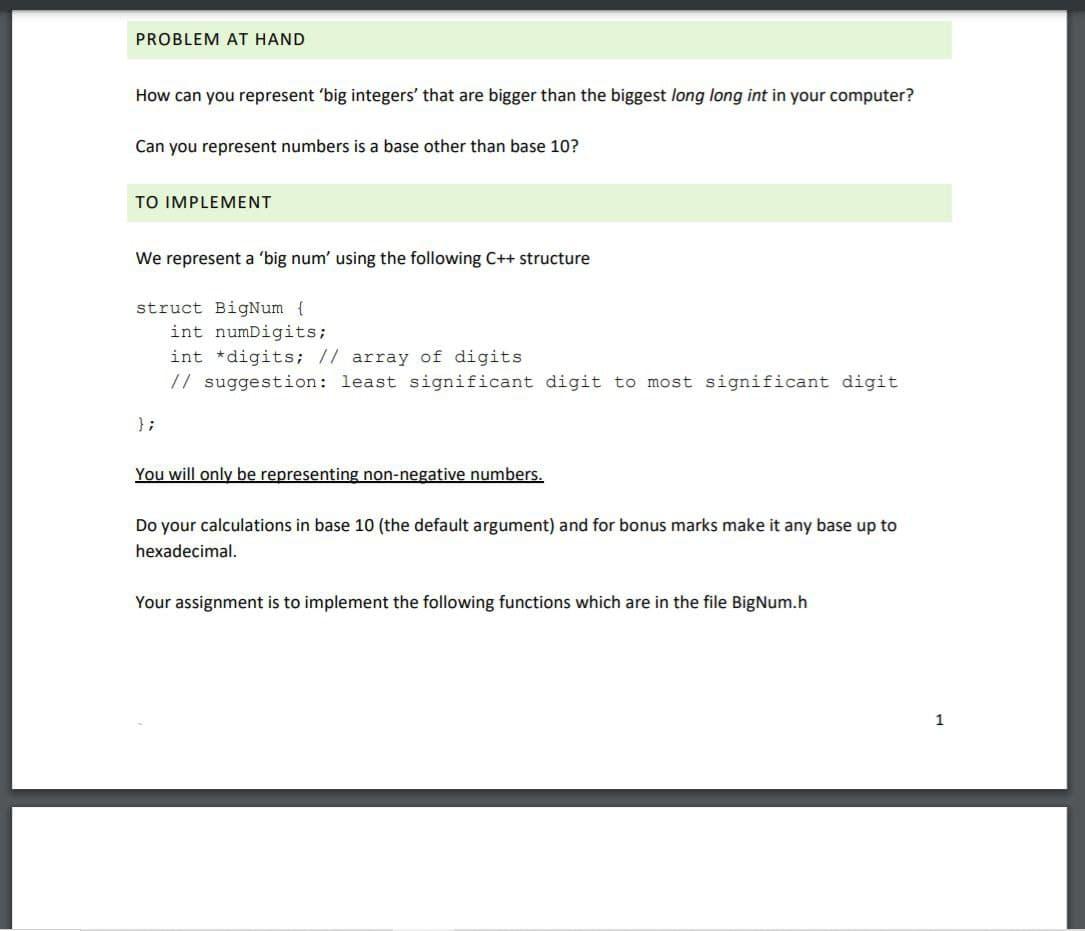
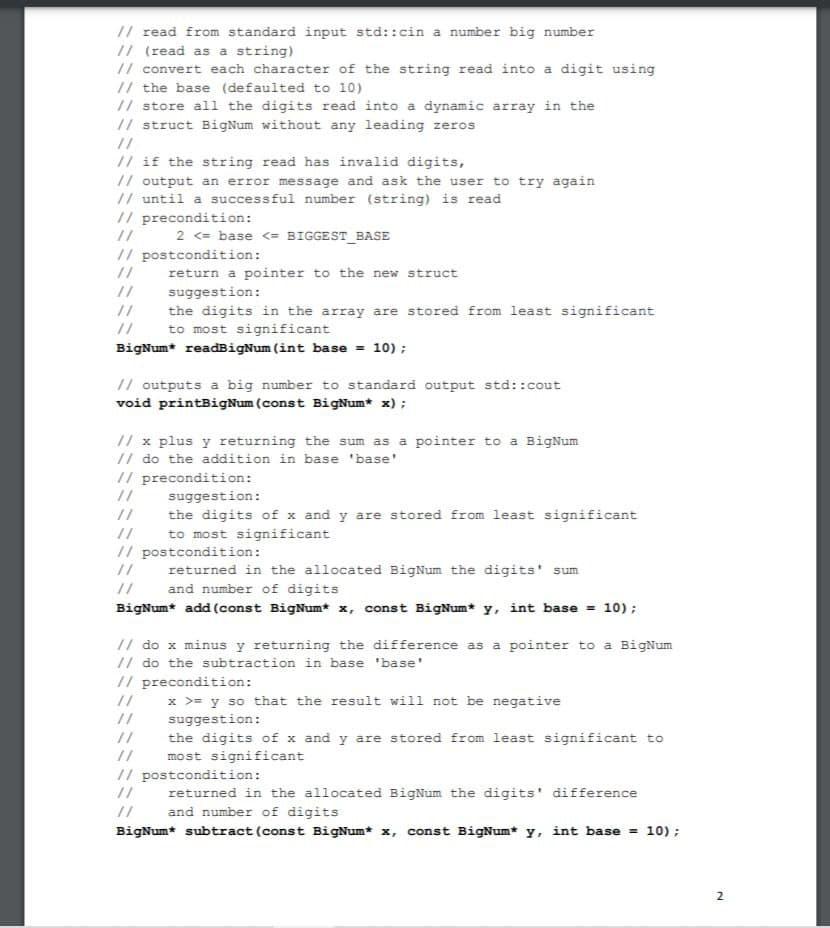
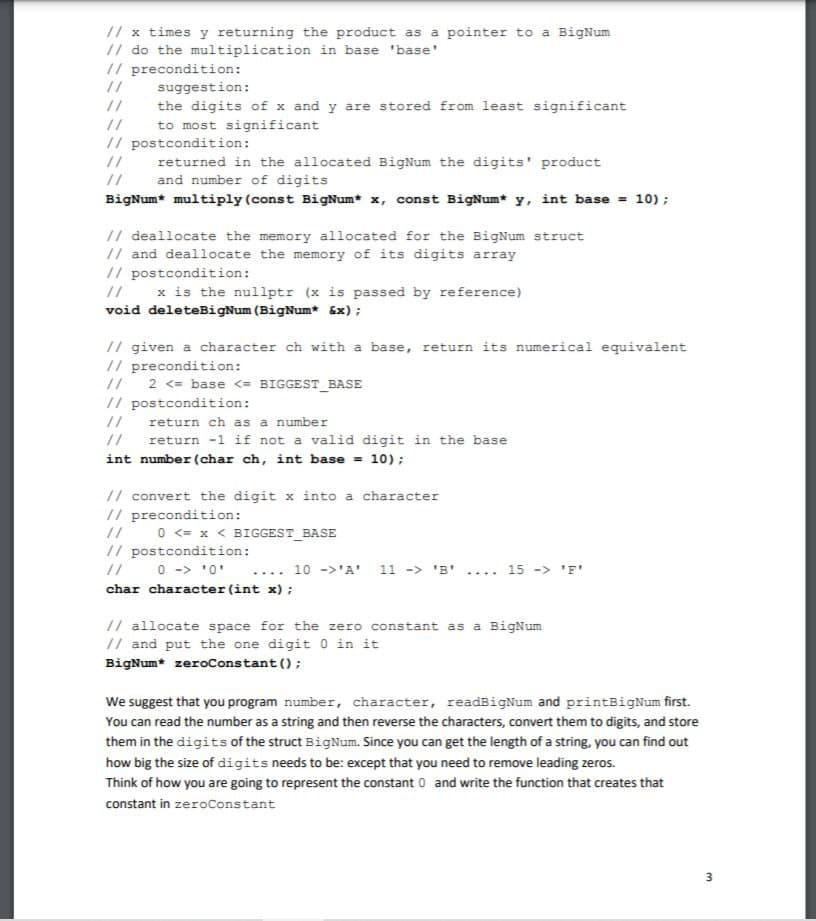
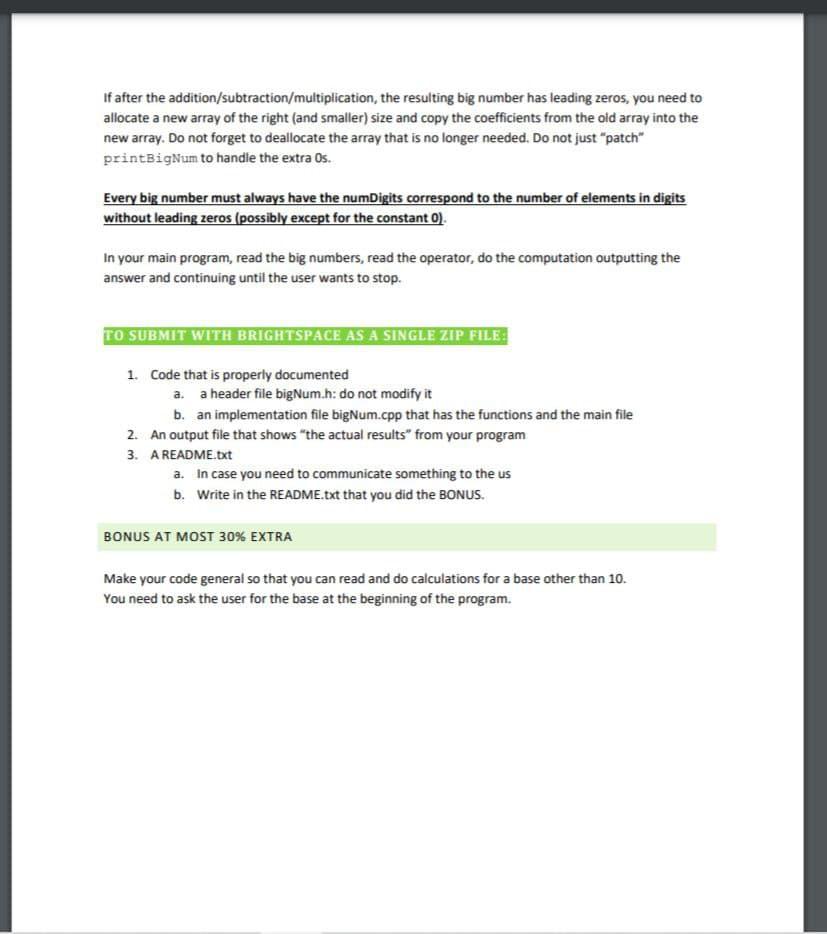
PROBLEM AT HAND How can you represent 'big integers' that are bigger than the biggest long long int in your computer? Can you represent numbers is a base other than base 10? TO IMPLEMENT We represent a 'big num' using the following C++ structure struct BigNum { int numDigits; int *digits; // array of digits // suggestion: least significant digit to most significant digit You will only be representing non-negative numbers. Do your calculations in base 10 (the default argument) and for bonus marks make it any base up to hexadecimal. Your assignment is to implement the following functions which are in the file BigNum.h 1 1/ read from standard input std::cin a number big number 17 (read as a string) 11 convert each character of the string read into a digit using // the base (defaulted to 10) 1/ store all the digits read into a dynamic array in the 1/ struct BigNum without any leading zeros // if the string read has invalid digits, 11 output an error message and ask the user to try again // until a successful number (string) is read // precondition: 2 = y so that the result will not be negative suggestion: the digits of x and y are stored from least significant to most significant 17 postcondition: // returned in the allocated BigNum the digits' difference and number of digits BigNum* subtract (const BigNum* x, const BigNum* y, int base = 10); 2 // * times y returning the product as a pointer to a BigNum 1/ do the multiplication in base "base" // precondition: // suggestion: the digits of x and y are stored from least significant to most significant Il postcondition: returned in the allocated BigNum the digits' product and number of digits BigNum* multiply(const BigNum* x, const BigNum* y, int base = 10); 11 deallocate the memory allocated for the BigNum struct // and deallocate the memory of its digits array // postcondition: x is the nullptr (x is passed by reference) void deleteBigNum (BigNum* Ex); // given a character ch with a base, return its numerical equivalent // precondition: 17 2 '0'... 10 ->'A' 11 -> '' .... 15 -> 'F' char character(int x); 1/ allocate space for the zero constant as a BigNum // and put the one digit 0 in it BigNum* zeroConstant(); We suggest that you program number, character, readBigNum and printBigNum first. You can read the number as a string and then reverse the characters, convert them to digits, and store them in the digits of the struct BigNum. Since you can get the length of a string, you can find out how big the size of digits needs to be: except that you need to remove leading zeros. Think of how you are going to represent the constant o and write the function that creates that constant in zero Constant 3 If after the addition/subtraction/multiplication, the resulting big number has leading zeros, you need to allocate a new array of the right (and smaller) size and copy the coefficients from the old array into the new array. Do not forget to deallocate the array that is no longer needed. Do not just "patch printBigNum to handle the extra Os. Every big number must always have the numDigits correspond to the number of elements in digits without leading zeros (possibly except for the constant O). In your main program, read the big numbers, read the operator, do the computation outputting the answer and continuing until the user wants to stop. TO SUBMIT WITH BRIGHTSPACE AS A SINGLE ZIP FILE: 1. Code that is properly documented a. a header file bigNum.h: do not modify it b. an implementation file bigNum.cpp that has the functions and the main file 2. An output file that shows the actual results from your program 3. A README.txt a. In case you need to communicate something to the us b. Write in the README.txt that you did the BONUS. BONUS AT MOST 30% EXTRA Make your code general so that you can read and do calculations for a base other than 10. You need to ask the user for the base at the beginning of the program. PROBLEM AT HAND How can you represent 'big integers' that are bigger than the biggest long long int in your computer? Can you represent numbers is a base other than base 10? TO IMPLEMENT We represent a 'big num' using the following C++ structure struct BigNum { int numDigits; int *digits; // array of digits // suggestion: least significant digit to most significant digit You will only be representing non-negative numbers. Do your calculations in base 10 (the default argument) and for bonus marks make it any base up to hexadecimal. Your assignment is to implement the following functions which are in the file BigNum.h 1 1/ read from standard input std::cin a number big number 17 (read as a string) 11 convert each character of the string read into a digit using // the base (defaulted to 10) 1/ store all the digits read into a dynamic array in the 1/ struct BigNum without any leading zeros // if the string read has invalid digits, 11 output an error message and ask the user to try again // until a successful number (string) is read // precondition: 2 = y so that the result will not be negative suggestion: the digits of x and y are stored from least significant to most significant 17 postcondition: // returned in the allocated BigNum the digits' difference and number of digits BigNum* subtract (const BigNum* x, const BigNum* y, int base = 10); 2 // * times y returning the product as a pointer to a BigNum 1/ do the multiplication in base "base" // precondition: // suggestion: the digits of x and y are stored from least significant to most significant Il postcondition: returned in the allocated BigNum the digits' product and number of digits BigNum* multiply(const BigNum* x, const BigNum* y, int base = 10); 11 deallocate the memory allocated for the BigNum struct // and deallocate the memory of its digits array // postcondition: x is the nullptr (x is passed by reference) void deleteBigNum (BigNum* Ex); // given a character ch with a base, return its numerical equivalent // precondition: 17 2 '0'... 10 ->'A' 11 -> '' .... 15 -> 'F' char character(int x); 1/ allocate space for the zero constant as a BigNum // and put the one digit 0 in it BigNum* zeroConstant(); We suggest that you program number, character, readBigNum and printBigNum first. You can read the number as a string and then reverse the characters, convert them to digits, and store them in the digits of the struct BigNum. Since you can get the length of a string, you can find out how big the size of digits needs to be: except that you need to remove leading zeros. Think of how you are going to represent the constant o and write the function that creates that constant in zero Constant 3 If after the addition/subtraction/multiplication, the resulting big number has leading zeros, you need to allocate a new array of the right (and smaller) size and copy the coefficients from the old array into the new array. Do not forget to deallocate the array that is no longer needed. Do not just "patch printBigNum to handle the extra Os. Every big number must always have the numDigits correspond to the number of elements in digits without leading zeros (possibly except for the constant O). In your main program, read the big numbers, read the operator, do the computation outputting the answer and continuing until the user wants to stop. TO SUBMIT WITH BRIGHTSPACE AS A SINGLE ZIP FILE: 1. Code that is properly documented a. a header file bigNum.h: do not modify it b. an implementation file bigNum.cpp that has the functions and the main file 2. An output file that shows the actual results from your program 3. A README.txt a. In case you need to communicate something to the us b. Write in the README.txt that you did the BONUS. BONUS AT MOST 30% EXTRA Make your code general so that you can read and do calculations for a base other than 10. You need to ask the user for the base at the beginning of the program