Question
Hello there. I have an issue. I cannot compile my code. There is seems to be an error but i do not see anything wrong.
Hello there. I have an issue. I cannot compile my code. There is seems to be an error but i do not see anything wrong. Maybe a second pair of eyes could help. I will post my error message and the two java files(Factory.java and ArrayDeque.java) that are linked together. The error seems to be in the ArrayDeque.java specifically where it says
protected Factoryf;
and
f = new Factory(t);
This is the error that gets printed :
Error:(21, 15) java: cannot find symbol symbol: class Factory location: class comp2402a2.ArrayDeque
Error:(53, 17) java: cannot find symbol symbol: class Factory location: class comp2402a2.ArrayDeque
My code below:
Factory.java
------------------------------------------------------------------------
package comp2402a2; import java.lang.reflect.Array; /** * An ugly little class for allocating objects and arrays of generic * type T. This is needed to work around limitations of Java generics. */ public class Factory{ Class t; /** * Return the type associated with this factory * @return */ public Class type() { return t; } /** * Constructor - creates a factory for creating objects and * arrays of type t(=T) * @param t0 */ public Factory(Class t0) { t = t0; } /** * Allocate a new array of objects of type T. * @param n the size of the array to allocate * @return the array allocated */ @SuppressWarnings({"unchecked"}) protected T[] newArray(int n) { return (T[])Array.newInstance(t, n); } /** * * @return */ public T newInstance() { T x; try { x = t.getDeclaredConstructor().newInstance(); } catch (Exception e) { x = null; } return x; } }
------------------------------------------------------------------------
ArrayDeque.java
------------------------------------------------------------------------
package comp2402a2; import java.util.AbstractList; /** * An implementation of the List interface that allows for fast modifications * at both the head and tail. * * The implementation is as a circular array. The List item of rank i is stored * at a[(j+i)%a.length]. Insertions and removals at position i take * O(1+min{i, size()-i}) amortized time. * @author morin * * @paramthe type of objects stored in this list * TODO: Implement addAll() and removeAll() efficiently */ public class ArrayDeque extends AbstractList { /** * The class of elements stored in this queue */ protected Factory f; /** * Array used to store elements */ protected T[] a; /** * Index of next element to de-queue */ protected int j; /** * Number of elements in the queue */ protected int n; /** * Grow the internal array */ protected void resize() { T[] b = f.newArray(Math.max(2*n,1)); for (int k = 0; k < n; k++) b[k] = a[(j+k) % a.length]; a = b; j = 0; } /** * Constructor */ public ArrayDeque(Class t) { f = new Factory (t); a = f.newArray(1); j = 0; n = 0; } public int size() { return n; } public T get(int i) { if (i < 0 || i > n-1) throw new IndexOutOfBoundsException(); return a[(j+i)%a.length]; } public T set(int i, T x) { if (i < 0 || i > n-1) throw new IndexOutOfBoundsException(); T y = a[(j+i)%a.length]; a[(j+i)%a.length] = x; return y; } public void add(int i, T x) { if (i < 0 || i > n) throw new IndexOutOfBoundsException(); if (n+1 > a.length) resize(); if (i < n/2) { // shift a[0],..,a[i-1] left one position j = (j == 0) ? a.length - 1 : j - 1; //(j-1)mod a.length for (int k = 0; k <= i-1; k++) a[(j+k)%a.length] = a[(j+k+1)%a.length]; } else { // shift a[i],..,a[n-1] right one position for (int k = n; k > i; k--) a[(j+k)%a.length] = a[(j+k-1)%a.length]; } a[(j+i)%a.length] = x; n++; } public T remove(int i) { if (i < 0 || i > n - 1) throw new IndexOutOfBoundsException(); T x = a[(j+i)%a.length]; if (i < n/2) { // shift a[0],..,[i-1] right one position for (int k = i; k > 0; k--) a[(j+k)%a.length] = a[(j+k-1)%a.length]; j = (j + 1) % a.length; } else { // shift a[i+1],..,a[n-1] left one position for (int k = i; k < n-1; k++) a[(j+k)%a.length] = a[(j+k+1)%a.length]; } n--; if (3*n < a.length) resize(); return x; } public void clear() { n = 0; resize(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
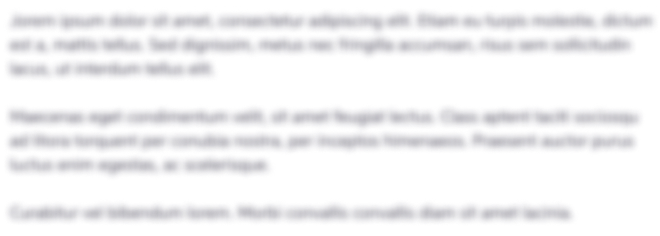
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started