Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Hello, this is for programming with the C++ language. Below is the assignment package. I included the default programs given with assignment. That includes: testfile,
Hello, this is for programming with the C++ language. Below is the assignment package. I included the default programs given with assignment. That includes: testfile, linelist.cpp (needs to be edited), and linelist.h (do not change). Professor said that linelist.h is complete for you, do not touch it. We do have to edit linelist.cpp and then create the client file from scratch as the assignment says below. I attempted this ;however, like they say, close but no cigar, but without the close part. I really need your help, please. Thank you for everything, seriously.
Objective The purpose of this program is to gain experience implementing a List ADT using double links. This assignment will also help you get re-acquainted with C++ and object-oriented techniques Assignment Summary The text edited by a line editor is represented by a doubly nked list of nodes, each containing a string object. There is one external pointer to this list, which points to the "current line in the text being edited. The list has a header node, which contains the string "Top of file - -"and a trailer node, which contains the string "- -- Bottom of file ---". See below for an illustration Your job is to write a simple ine editor. Start the program with entering EDIT file, after which a prompt appears along with a line number representing the current line. If the letter I is entered, you should allow the user to enter a new line of text after the current line. If the letter D is entered the current line should be deleted. If the letter L is entered, the entire lst should be printed. The command P should be used for moving to the previous line, and N should be used for moving to the next line. Entry E signifies exit and saving the text back in the file. Sample Kun 1 This is my first line 2> This is my second line 3> This is my third line 2> I 3> A brand new line is inserted! 1> This is my first line 2> This is my second line 3> A brand new line is inserted! 4> This is my third line 3> D 2> L 1> This is my first line 2> This is my second line 2> E Files You may copy over several files for use with this program by typing: cp /pub/digh/CSC245/Prog1/*. This will include the the specification file for the LineList class that you wi need to use with this assignment. There is also an example of an executable version of this program, and an in put/output file for your editor. This file should be input at the command line You il not have to modify any of the existing code in the specification file. You will be coding the implementation file, and writing your own client file. Name your client file editor.cpp The LineList ADT We want to define a class LineList to represent a doubly-linked list ADT with the following public accessor functions: goToTop goes to the top of the list; the current line is set to access the first line of text goToBottom goes to the bottom of the list; the current line is set to access the last line of text 1nsertLine inserts a new line after the current line, after which the external pointer now points to the new line e deleteLine deletes the current line printList lists all the lines noveNextLine moves the external pointer to the next line novePrevLine moves the external pointer to the previous line getCurrLineNum returns an integer representing the current line number getLength returns an integer representing the current length of the list getCurrLine returns a string representing the line currently being pointed to by the external pointer The user should be able to move into the top and bottom buffer regions as shown in the sample executable. Attempting to delete in these regions causes no error. Attempting to insert in the top buffer moves the inserted line into the top position. Attempting to insert in the bottom buffer moves the inserted line into the bottm position Genera Design of Client File The main function in your client program must use command line arguments to input the name of the external file. So, your main function will need to be to set up as: int ainint arge, char argy Recall that argc will contain the number of items typed on the command line upon execution, and argv is an array containing each of the strings entered. So, if we type EDIT testfile, our argc is 2, argv [O] contains EDIT", and argv[1] contains "testfile" If the user forgets to enter a filename, argc will only be equal to 1. You should check for this scenario, and terminate program execution with a exit 1) in this case. The first task of your client file is to open up the file stored in argv[1), and build and print your inital list based on any strings that are contained within the external file being input. After this initial print, make sure your current line is set to the bottom. You're then ready to continue getting a command from the user, and executing it until the com- mand E is entered. After an E is entered, you will need to write out the strings currently contained in your editor list to the same external file you used for input. This is basically like you're per- forming a save operation ( n vi) Below is the assignment and below that are the default programs.




Below are the programs. testfile is the read me file that I believe was saved via running the client program made by the professor. Next is the linelist.cpp which is used to create our linelist commands and then the linelist.h is complete and should not be changed.
testfile, linelist.h , linelist.cpp
------------------------------------------------------------------------------------------------------
//testfile
This is my first line.
This is my second line.
This is my third line.
-------------------------------------------------------------------------------------------------------
//linelist.cpp
#include
#include "linelist.h"
LineList::LineList()
{
LineNode *line = new LineNode;
LineNode *line2 = new LineNode;
line -> info = topMessage;
currLine = line;
currLineNum = 1;
length = 0;
line2 -> info = bottomMessage;
currLine -> back = NULL;
currLine -> next = line2;
line2 -> back = line;
line2 -> next = NULL;
}
void LineList::goToTop()
{
// Post : Advances currLine to line 1
}
void LineList::goToBottom()
{
// Post : Advances currLine to last line
}
void LineList::insertLine(string newLine)
{
// post : newLine has been inserted after the current line
}
void LineList::deleteLine()
{
// post : deletes the current line leaving currentLine
// pointing to line following
}
void LineList::printList()
{
}
string LineList::getCurrLine() const
{
}
void LineList::moveNextLine()
{
// Post : Advances currLine (unless already at last line)
}
void LineList::movePrevLine()
{
// Post : Advances currLine (unless already at last line)
}
int LineList::getCurrLineNum() const
{
}
int LineList::getLength() const
{
}
-------------------------------------------------------------------------------------------------------
//linelist.h (DO NOT CHANGE)
using namespace std;
#include
const string topMessage = " - - - Top of file - - - ";
const string bottomMessage = " - - - Bottom of file - - - ";
struct LineNode;
class LineList
{
public:
LineList();
void goToTop();
void goToBottom();
void insertLine (string newLine);
void deleteLine ();
void printList();
void moveNextLine();
void movePrevLine();
int getCurrLineNum() const;
int getLength() const;
string getCurrLine() const;
private:
LineNode* currLine;
int currLineNum;
int length;
};
struct LineNode
{
string info;
LineNode* next;
LineNode* back;
};
--------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
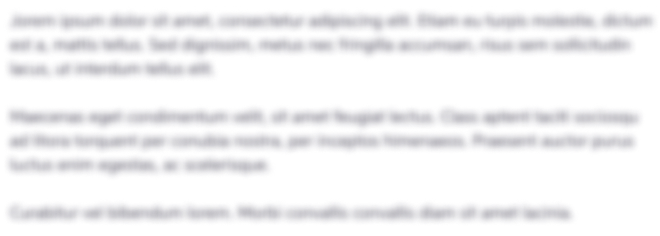
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started