Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
Help ASAP!!!!! Programming Language C++ Assignment Client Program Server please include comments Requirements For this assignment, you will be writing two programs which are designed
Help ASAP!!!!!
Programming Language C++
Assignment
Client Program
Server
please include comments
Requirements For this assignment, you will be writing two programs which are designed to talk to each other. One of your programs will be the activation server and the other will be the activation client. Here is the synopsis of how the two programs interact: Server: As the server starts up, it looks to see if the data file (the file of serial nums/machine IDs) exists. If the file does not exist, it will create an empty one. The name of this file is datafile.txt The server binds to a port and awaits connections. Client: The client will look for an activation file. The name of this file is actFile.txt o If the file cannot be found, proceed to attempt activation. o If the file is found, compare the machine ID in the file with the given machine ID to see if they match. If they do not match, proceed to attempt activation. If they do match, the software has already been activated and the program exits. To continue with the activation process, the client now gets a serial number from the user. The client contacts the server. Server: Server accepts the incoming connection Waits for data to be sent. Client: Send the serial number to the server Wait for a reply from the server Server: Receive the serial number . Check to make sure the serial number is valid for the nurnoses of this assignment a valid serial number is any combination of Check to make sure the serial number is valid. For the purposes of this assignment, a valid serial number is any combination of digits. A string containing characters other than digits would be considered to be an invalid serial number. If the serial number is valid o Send a "good" message back to the client . Wait for the next message If the serial number is invalid Send an "invalid" message back to the client o Close the current connection and wait for a new connection from a new client Client: Receive server reply If the reply is "good" o Send the machine ID to the server o Wait for a reply from the server If the reply is "invalid" o Report to the user that the serial number is invalid o Exit the program erver: Receive the machine ID from client - Try to find serial number in the data file If serial number is found o Compare the given machine ID with the one recorded in the data file o If the machine IDs match Send a "good" message back to the client Close the current connection and wait for a new connection from a new client o If the machine IDs do not match Send an "invalid" message back to the client Close the current connection and wait for a new connection from a new client If serial number is not found o Write the serial number and machine ID into the data file o Send a "good" message back to the client o Close the current connection and wait for a new connection from a new client Client: Receive server reply If reply is "good" o Record the machine ID in the activation file o Report to the user that the activation was successful Exit the program If reply is "invalid" o Report to the user that the activation was unsuccessful o Exit the program Note that in the above sequence of communication steps, once the server has either accepted or rejected the activation, it closes the connection and then goes back to waiting for a new connection. Also note, although it isn't specifically listed in the steps above, our server will also display results to the screen to show what's happening. Activation Server / Client Test Plan Test 1: server data file Start the server RESULTS: o An empty file called dataFile.txt file should be created Test 2: client needs activation Start the client Enter machine ID: abcd RESULTS: o Client should state that it needs to be activated Test 3: activate client Enter serial number: 1234 RESULTS: o Client should state that it was successfully activated O A file called actFile.txt should be created and contains the content: abcd o The server should show on the screen the serial number and machine ID and that both are GOOD o The server data file should be updated to contain the serial number and machine ID Test 4: client does not need to be activated Start the client Enter machine ID: abcd RESULTS: o Client should state that it has already been activated o There should be no interaction between client and server Test 5: client can be reactivated Delete the activation file Start the client Enter machine ID: abcd RESULTS: o Client should state that it needs to be activated Enter serial number: 1234 RESULTS: o Client should state that it was successfully activated o A file called actFile.txt should be created and contains the content: abcd the content: abcd o The server should show on the screen the serial number and machine ID and that both are GOOD o The server data file should not have changed. It should still only contain one activation Test 6: activate another client Delete the activation file Start the client Enter machine ID: qwer RESULTS: o Client should state that it needs to be activated Enter serial number: 4567 RESULTS: o Client should state that it was successfully activated O A file called actFile.txt should be created and contains the content: qwer o The server should show on the screen the serial number and machine ID and that both are GOOD o The server data file should be updated to contain the serial number and machine ID. There should now be two activations recorded in the data file; one for serial number 1234 and another for 4567. Test 7: prevent activation of a previously activated serial (1) Start the client Enter machine ID: zxcv RESULTS: o Client should state that the activation data has been altered, and that the program needs to be activated Enter serial number: 1234 RESULTS: o Client should state that the activation was not successful o The server should show on the screen the serial number is GOOD but the machine ID is INVALID o There should be no change in the server data file o There should be no change in the activation file Test 8: prevent activation of a previously activated serial Test 8: prevent activation of a previously activated serial (2) Delete the activation file Start the client Enter machine ID: zxcv RESULTS: o Client should state that it needs to be activated Enter serial number: 4567 RESULTS: o Client should state that the activation was not successful o No activation file should have been created o The server should show on the screen the serial number is GOOD but the machine ID is INVALID o There should be no change in the server data file Test 9: prevent bad serial number Start the client Enter machine ID: zxcv RESULTS: o Client should state that it needs to be activated Enter serial number: abcd RESULTS: o Client should state that the activation was not successful o No activation file should have been created The server should show on the screen the serial number is INVALID but the machine ID should have never been communicated to the server and therefore the server should not show any further information on the screen There should be no change in the server data file Test 10: server retains data Close the server program Start the server again RESULTS: o The server data file should still be there, and should still contain the two activations which were previously recorded in the data file; one for serial number 1234 and another for 4567. #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
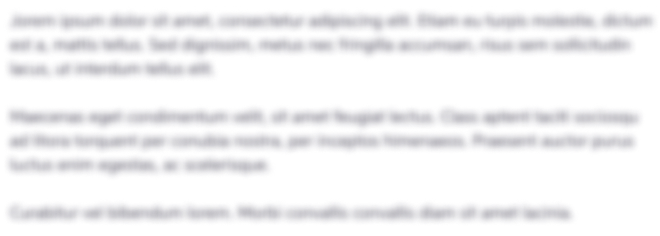
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started