Question
Help making a C++ program for the Battleship game board using four files (without ** double pointers). Use this as a template (This is basically
Help making a C++ program for the Battleship game board using four files (without ** double pointers).
Use this as a template (This is basically what I am looking for, but without the 2D ** pointers):
https://www.chegg.com/homework-help/questions-and-answers/help-making-c-program-battleship-game-board-using-four-files-ship-class-structs-represents-q29666740
The Ship class (no structs) represents a ship that has:
a name (e.g. "my destroyer")
a length (the number of squares it occupies)
a damage (how many of its squares have been hit)
a constructor that takes as parameters (in this order): the Ship's name and the Ship's length, which will be used to initialize the corresponding data members. The damage should be initialized to zero.
getMethods for each data member (getName, getLength, getDamage)
a method called takeHit that increments a Ship's damage by one
_________________________________________________________________________
The BBoard class represents a 10x10 grid that holds some number of Ships. It should have:
a 10x10 array of bools (for keeping track of what squares have been attacked)
a 10x10 array of Ship-pointers (for keeping track of which Ships occupy which squares)
(Please do not use double pointers)
a variable that keeps track of the number of ships that remain un-sunk
a constructor that initializes each element of the boolArray to false and each element of the Ship-pointer array to NULL (or nullptr if you prefer)
a method called getAttacksArrayElement that takes a row and column (in that order) and returns the element at that position of the bool array
a method called getShipsArrayElement that takes a row and column (in that order) and returns the element at that position of the ships array
a method called getNumShipsRemaining that returns how many ships remain un-sunk
a method called placeShip that takes as parameters (in this order): the address of a Ship object, the row and column of the square of the Ship that is closest to (0, 0), and the Ship's orientation (either 'R' if its squares occupy the same row or 'C' if its squares occupy the same column). I give a couple of examples at the end of the specifications
This method will set the elements of the array that the Ship occupies to hold the address of the Ship. If a Ship would not fit entirely on the Board, or if it would overlap any previously placed ships, the ship should not be added to the Board and the method should return false. Otherwise, the ship should be placed on the Board, the number of un-sunk ships should be incremented, and the method should return true.
a method called attack that takes as parameters the row and column of the attack (in that order). If the attack hits a Ship, you should:
record the attack in the bool array
if that square has not been hit before, you should call that Ship's takeHit method
if all of a Ship's squares have been hit, you should print "They sank [insert name of ship here]!" and decrement the number of ships that remain un-sunk (you should only do these once for any ship)
return true (even if that square was previously hit)
If the attack is not a hit, you should record the attack in the bool array and return false.
a method called allShipsSunk that returns true if all ships on the Board have been sunk, but returns false otherwise.
The four files must be named Ship.hpp, Ship.cpp, BBoard.hpp, and BBoard.cpp.
Example of the placeShip method - if we have the following values for the parameters:
a Ship that has a length of 4
the row and column are 6 and 8 respectively
the orientation is 'C'
Then the ship would occupy the following squares:
0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 x 7 x 8 x 9 x
Output should look just like above.
Example:
Example of the placeShip method - if we have the following values for the parameters:
a Ship that has a length of 4
the row and column are 6 and 8 respectively
the orientation is 'C'
Then the ship would occupy the following squares:
0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 x x x 5 6 7 8 9
Step by Step Solution
There are 3 Steps involved in it
Step: 1
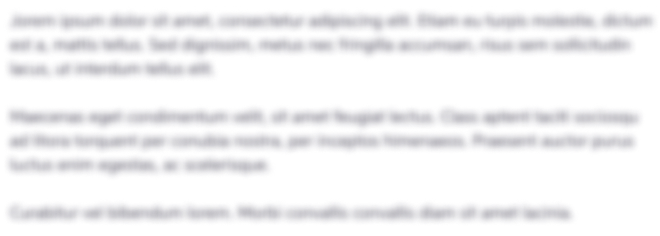
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started