Question
help me figure this out! //#define _CRT_SECURE_NO_WARNINGS #ifdef _WIN32 #include #endif #include #include #include #include #include #include #include #include #ifndef _WIN32 #include #else void usleep(unsigned
help me figure this out!
//#define _CRT_SECURE_NO_WARNINGS
#ifdef _WIN32
#include
#endif
#include
#include
#include
#include
#include
#include
#include
#include
#ifndef _WIN32
#include
#else
void usleep(unsigned int nanosec)
{
Sleep(nanosec / 1000);
}
#endif
// *************** GLOBAL VARIABLES *************************
const float PI = 3.14159;
// --------------- USER INTERFACE VARIABLES -----------------
// Window settings
int windowID; // Glut window ID (for display)
GLUI *glui; // Glui window (for controls)
int Win[2]; // window (x,y) size
// ---------------- ANIMATION VARIABLES ---------------------
// Animation settings
int animate_mode = 0; // 0 = no anim, 1 = animate
int animation_frame = 0; // Specify current frame of animation
// Joint parameters
const float JOINT_MIN = 0.0f;
const float JOINT_MAX = 360.0f;
float joint_rot = 0.0f;
//////////////////////////////////////////////////////
// TODO: Add additional joint parameters here
//////////////////////////////////////////////////////
// *********** FUNCTION HEADER DECLARATIONS ****************
// Initialization functions
void initGlut(char* winName);
void initGlui();
void initGl();
// Callbacks for handling events in glut
void myReshape(int w, int h);
void animate();
void display(void);
// Callback for handling events in glui
void GLUI_Control(int id);
// Functions to help draw the object
void drawSquare(float size);
// Return the current system clock (in seconds)
double getTime();
// ******************** FUNCTIONS ************************
// main() function
// Initializes the user interface (and any user variables)
// then hands over control to the event handler, which calls
// display() whenever the GL window needs to be redrawn.
int main(int argc, char** argv)
{
// Process program arguments
if (argc != 3) {
printf("Usage: demo [width] [height] ");
printf("Using 300x200 window by default... ");
Win[0] = 300;
Win[1] = 200;
}
else {
Win[0] = atoi(argv[1]);
Win[1] = atoi(argv[2]);
}
// Initialize glut, glui, and opengl
glutInit(&argc, argv);
initGlut(argv[0]);
initGlui();
initGl();
// Invoke the standard GLUT main event loop
glutMainLoop();
return 0; // never reached
}
// Initialize glut and create a window with the specified caption
void initGlut(char* winName)
{
// Set video mode: double-buffered, color, depth-buffered
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
// Create window
glutInitWindowPosition(0, 0);
glutInitWindowSize(Win[0], Win[1]);
windowID = glutCreateWindow(winName);
// Setup callback functions to handle events
glutReshapeFunc(myReshape); // Call myReshape whenever window resized
glutDisplayFunc(display); // Call display whenever new frame needed
}
// Quit button handler. Called when the "quit" button is pressed.
void quitButton(int)
{
exit(0);
}
// Animate button handler. Called when the "animate" checkbox is pressed.
void animateButton(int)
{
// synchronize variables that GLUT uses
glui->sync_live();
animation_frame = 0;
if (animate_mode == 1) {
// start animation
GLUI_Master.set_glutIdleFunc(animate);
}
else {
// stop animation
GLUI_Master.set_glutIdleFunc(NULL);
}
}
// Initialize GLUI and the user interface
void initGlui()
{
GLUI_Master.set_glutIdleFunc(NULL);
// Create GLUI window
glui = GLUI_Master.create_glui("Glui Window", 0, Win[0] + 10, 0);
// Create a control to specify the rotation of the joint
GLUI_Spinner *joint_spinner
= glui->add_spinner("Joint", GLUI_SPINNER_FLOAT, &joint_rot);
joint_spinner->set_speed(0.1);
joint_spinner->set_float_limits(JOINT_MIN, JOINT_MAX, GLUI_LIMIT_CLAMP);
///////////////////////////////////////////////////////////
// TODO:
// Add controls for additional joints (rotation points for other letters) here
///////////////////////////////////////////////////////////
// Add button to specify animation mode
glui->add_separator();
glui->add_checkbox("Animate", &animate_mode, 0, animateButton);
// Add "Quit" button
glui->add_separator();
glui->add_button("Quit", 0, quitButton);
// Set the main window to be the "active" window
glui->set_main_gfx_window(windowID);
}
// Performs most of the OpenGL intialization
void initGl(void)
{
// glClearColor (red, green, blue, alpha)
// Ignore the meaning of the 'alpha' value for now
glClearColor(0.7f, 0.7f, 0.9f, 1.0f);
}
// Callback idle function for animating the scene
void animate()
{
// Update geometry
const double joint_rot_speed = 10;
double joint_rot_t = double(int(animation_frame*joint_rot_speed) % int (JOINT_MAX)) / JOINT_MAX;
joint_rot = joint_rot_t * JOINT_MIN + (1 - joint_rot_t) * JOINT_MAX;
///////////////////////////////////////////////////////////
// TODO:
// Modify this function animate letters roation joints
// Note: Nothing should be drawn in this function! OpenGL drawing
// should only happen in the display() callback.
///////////////////////////////////////////////////////////
// Update user interface
glui->sync_live();
// Tell glut window to update itself. This will cause the display()
// callback to be called, which renders the object (once you've written
// the callback).
glutSetWindow(windowID);
glutPostRedisplay();
// increment the frame number.
animation_frame++;
// Wait 50 ms between frames (20 frames per second)
usleep(50000);
}
// Handles the window being resized by updating the viewport
// and projection matrices
void myReshape(int w, int h)
{
// Setup projection matrix for new window
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-w / 2, w / 2, -h / 2, h / 2);
// Update OpenGL viewport and internal variables
glViewport(0, 0, w, h);
Win[0] = w;
Win[1] = h;
}
// display callback
//
// This gets called by the event handler to draw
// the scene, so this is where you need to build
// your scene -- make your changes and additions here.
// All rendering happens in this function. For this Assignment,
// updates to geometry should happen in the "animate" function.
void display(void)
{
// glClearColor (red, green, blue, alpha)
// Ignore the meaning of the 'alpha' value for now
glClearColor(0.7f, 0.7f, 0.9f, 1.0f);
// OK, now clear the screen with the background colour
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// Setup the model-view transformation matrix
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
///////////////////////////////////////////////////////////
// TODO:
// Modify this function draw the scene
// This should include function calls to pieces that
// apply the appropriate transformation matrice and
// render the individual objects.
///////////////////////////////////////////////////////////
const float ARM_LENGTH = 50.0f;
const float ARM_WIDTH = 10.0f;
// Push the current transformation matrix on the stack
glPushMatrix();
// Rotate along the hinge
glRotatef(joint_rot, 0.0, 0.0, 1.0);
// Scale the size of the arm
glScalef(ARM_WIDTH, ARM_LENGTH, 1.0);
// Draw the square for the arm
glColor3f(1.0, 0.0, 0.0);
drawSquare(1.0);
// Retrieve the previous state of the transformation stack
glPopMatrix();
// Execute any GL functions that are in the queue just to be safe
glFlush();
// Now, show the frame buffer that we just drew into.
// (this prevents flickering).
glutSwapBuffers();
}
// Draw a square of the specified size, centered at the current location
void drawSquare(float width)
{
// Draw the square
glBegin(GL_POLYGON);
glVertex2d(-width / 2, -width / 2);
glVertex2d(width / 2, -width / 2);
glVertex2d(width / 2, width / 2);
glVertex2d(-width / 2, width / 2);
glEnd();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
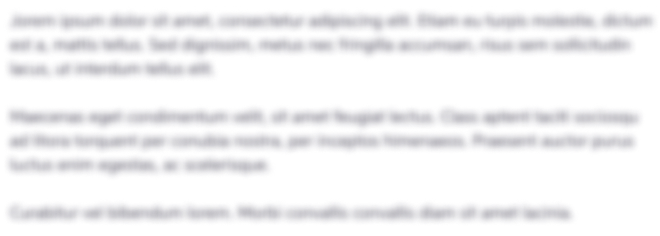
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started