Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Help me understand why top() method does not work in the StackTest.java I get: java: cannot find symbol symbol: method top() location: variable emptyStack of
Help me understand why top() method does not work in the StackTest.java
I get:
java: cannot find symbol symbol: method top() location: variable emptyStack of type java.util.Stack
stack interface class:
public interface Stack
void push ( T elem);
T pop();
T top();
int size();
boolean isEmpty();
}
import java.util.EmptyStackException; import java.util.NoSuchElementException; /** * A singly linked list. * * @author * @version 25/1-2022 */ public class LinkedListimplements Stack { private ListElement first; // First element in list. private ListElement last; // Last element in list. private int size; // Number of elements in list. /** * A list element. */ private static class ListElement { public T data; public ListElement next; public ListElement(T data) { this.data = data; this.next = null; } } /** * Creates an empty list. */ public LinkedList() { this.first = null; this.last = null; this.size = 0; } /** * Inserts the given element at the beginning of this list. * * @param element An element to insert into the list. */ public void addFirst(T element) { ListElement firstElement = new ListElement<>(element); if (this.size == 0){ this.first = firstElement; this.last = firstElement; } else{ firstElement.next = this.first; this.first = firstElement; } this.size ++; } /** * Inserts the given element at the end of this list. * * @param element An element to insert into the list. */ public void addLast(T element) { ListElement lastElement = new ListElement<>(element); if(this.size ==0){ this.first = lastElement; } else{ this.last.next = lastElement; } this.last = lastElement; this.size ++; } /** * @return The head of the list. * @throws NoSuchElementException if the list is empty. */ public T getFirst() { if (this.first != null){ return this.first.data; } else{ throw new NoSuchElementException(); } } /** * @return The tail of the list. * @throws NoSuchElementException if the list is empty. */ public T getLast() { if(this.last != null){ return this.last.data; } else{ throw new NoSuchElementException(); } } /** * Returns an element from a specified index. * * @param index A list index. * @return The element at the specified index. * @throws IndexOutOfBoundsException if the index is out of bounds. */ public T get(int index) { if(index < 0|| index >= this.size){ throw new IndexOutOfBoundsException(); } else{ ListElement element = this.first; for(int i = 0; i < index; i++){ element = element.next; } return element.data; } } /** * Removes the first element from the list. * * @return The removed element. * @throws NoSuchElementException if the list is empty. */ public T removeFirst() { if(this.first != null || this.size != 0){ ListElement list = this.first; this.first = first.next; size --; return list.data; } else{ throw new NoSuchElementException(); } } /** * Removes all of the elements from the list. */ public void clear() { this.first = null; this.last = null; this.size =0; } /** * Adds the element to the top of the stock. * @param elem */ @Override public void push(T elem) { ListElement list = new ListElement<>(elem); if( first == null){ first = list; last = first; } else{ list.next = first; first = list; } size ++; } /** * Removes and returns the top element in stack, * that is the element that was last added. * Throws an EmptyStackException if stack is empty. * @return the top element in the stack. */ @Override public T pop(){ if(isEmpty()){ throw new EmptyStackException(); }else{ ListElement list = first; first = first.next; size --; return list.data; } } /** * returns the top element in the stack without removing it. * Throws an EmptyStackException if stack is empty. * @return the top element. */ @Override public T top() { if(isEmpty()){ throw new EmptyStackException(); }else{ return first.data; } } /** * Returns the number of elements in the stock * @return The number of elements in the stock. */ public int size() { return this.size; } /** * Note that by definition, the list is empty if both first and last * are null, regardless of what value the size field holds (it should * be 0, otherwise something is wrong). * * @return true
if this list contains no elements. */ public boolean isEmpty() { return first == null && last == null; } /** * Creates a string representation of this list. The string * representation consists of a list of the elements enclosed in * square brackets ("[]"). Adjacent elements are separated by the * characters ", " (comma and space). Elements are converted to * strings by the method toString() inherited from Object. * * Examples: * "[1, 4, 2, 3, 44]" * "[]" * * @return A string representing the list. */ public String toString() { ListElementlistOfElements = this.first; String returnString = "["; while(listOfElements != null) { returnString += listOfElements.data; if(listOfElements.next != null){ returnString += ", "; } listOfElements = listOfElements.next; } returnString += "]"; return returnString; } }
Testclasses:
import org.junit.Test; import org.junit.Before; import org.junit.Rule; import org.junit.rules.Timeout; import static org.junit.Assert.*; import java.util.Stack; import static org.hamcrest.MatcherAssert.assertThat; import static org.hamcrest.CoreMatchers.*; import java.lang.Integer; import java.util.EmptyStackException; import java.util.stream.IntStream; /** * Abstract test class for Stack implementations. * * Implementing test classes must only implement the getIntegerStack * method. Be careful not to override ANY other methods! * * @author * @version 2018-12-15 */ public abstract class StackTest{ @Rule public Timeout globalTimeout = Timeout.seconds(5); private Stackstack; private int[] valuesInStack; private int initialStackSize; private Stack emptyStack; @Before public void setUp() { valuesInStack = new int[] {3, 4, 1, -123, 4, 1}; initialStackSize = valuesInStack.length; stack = getIntegerStack(); pushArrayToStack(valuesInStack, stack); emptyStack = getIntegerStack(); } /** * Push an array to the stack, in order. * * @param array An int array. * @param stack A Stack. */ private void pushArrayToStack(int[] array, Stack stack) { for (int i = 0; i < array.length; i++) { stack.push(array[i]); } } /** * This is the only method that implementing classes need to override. * * @return An instance of Stack. */ protected abstract Stack getIntegerStack(); @Test public void topIsLastPushedValue() { // Arrange int value = 1338; // Act emptyStack.push(value); stack.push(value); int emptyStackTop = emptyStack.top(); int stackTop = stack.top(); // Assert assertThat(emptyStackTop, equalTo(value)); assertThat(stackTop, equalTo(value)); } @Test(expected = EmptyStackException.class) //TESTTT public void topExceptionWhenStackIsEmpty() { Stack stack = new Stack<>(); try{ stack.top(); fail(); } catch (EmptyStackException e){ } } @Test(expected = EmptyStackException.class) //TESTTTT public void popExceptionWhenStackIsEmpty() { Stack stack = new Stack<>(); try{ stack.pop(); fail(); } catch(EmptyStackException e){ } } @Test public void popReturnsPushedValuesInReverseOrder() { // Arrange int lastIndex = valuesInStack.length - 1; IntStream .range(0, initialStackSize) // Act .mapToObj(i -> new ResultPair (stack.pop(), valuesInStack[lastIndex - i])) // Assert .forEach(pair -> assertThat(pair.actual, equalTo(pair.expected))); } @Test public void popFiveTimesDecreasesSizeByFive() { /// TESTTT int val = 5; popElements(stack,val); assertThat(stack.size(),equalTo(stack.size()-val)); } @Test public void pushFiveTimesIncreasesSizeByFive() { //TESTTT int val = 5; popElements(stack,val); popReturnsPushedValuesInReverseOrder(); assertThat(stack.size(),equalTo(stack.size()-val)); } @Test public void stateIsValidWhenPushCalledOnce() { // Arrange int val = 2; // Act emptyStack.push(val); // Assert assertThat(emptyStack.size(), equalTo(1)); assertThat(emptyStack.top(), equalTo(val)); } @Test public void isEmptyIsFalseWhenStackIsNotEmpty() { // Act boolean stackIsEmpty = stack.isEmpty(); // Assert assertFalse(stackIsEmpty); } @Test public void isEmptyIsTrueWhenStackIsEmpty() { // Act boolean emptyStackIsEmpty = emptyStack.isEmpty(); // Assert assertTrue(emptyStackIsEmpty); } @Test public void isEmptyIsTrueWhenAllElementsHaveBeenPopped() { // Act popElements(stack, initialStackSize); boolean stackIsEmpty = stack.isEmpty(); // Assert assertTrue(stackIsEmpty); } @Test public void sizeIs0WhenStackIsEmpty() { /// TESTTTT Stack stack = new Stack<>(); assertEquals(0,stack.size()); } @Test public void sizeIs0WhenAllElementsHaveBeenPopped() { //TESTTT popElements(stack,initialStackSize); assertEquals(0,stack.size()); } // HELPERS /** * Pops the desired amount of elements. * * @param stack A Stack. * @param amountOfElements The amount of elements to pop. */ private void popElements(Stack stack, int amountOfElements) { for (int i = 0; i < amountOfElements; i++) { stack.pop(); } } /** * Class used for stream operations when both actual and expected values * need to be gather in conjunction. */ private class ResultPair { public final T actual; public final T expected; public ResultPair(T actual, T expected) { this.actual = actual; this.expected = expected; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
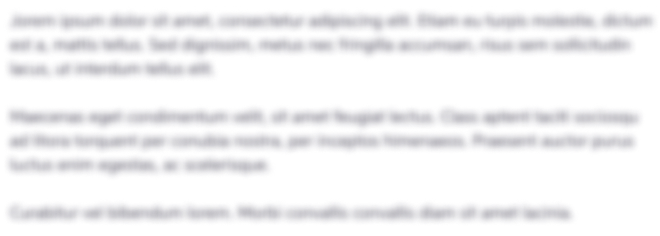
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started