Question
Help me with the following practice question about c++ C++. Modify the problem2.hpp with the complete implementation of the TextLineReader class template. A TextLineReader is
Help me with the following practice question about c++
C++. Modify the problem2.hpp with the complete implementation of the TextLineReader class template.
A TextLineReader is an object that can read a text file one line at a time, parsing each line and turning it into an object of some type. The reason we've implemented it as a class template is so that different TextLineReaders can transform their file into different types of objects; for example, a TextLineReader
Write the implementation of the TextLineReader class template. You may not change any of the public declarations that were provided, but you can add anything you'd like including public or private member functions, private member variables, and so on but you'll need to meet all of the following design requirements.
- The RAII( Resource acquisition is initialization )technique must be used here, which means that a TextLineReader should only be able to exist if opening its file was successful, and should close its file automatically when it is destroyed.
- It should not be possible to copy (either via a copy constructor or copy assignment) a TextFileReader. (Note that this functionality has been provided already; I've specified that the copy constructor and assignment operator are deleted, which makes it impossible to call them.)
- Each call to nextLine should return the transformed version of each line of the file. If the file contains 100 lines of text in the appropriate format, there will be 100 successful calls to nextLine. If there is no more text in the file, nextLine will throw a FileException instead. (See FileException.hpp.)
- The hasNextLine member functiton returns true if there are more lines in the file that haven't yet been returned, and false if all of the lines have been read and transformed.
- If the line parser fails on any line (i.e., by throwing any exception), an exception will be thrown from nextLine. A subsequent call to hasNextLine should return false and a subsequent call to nextLine must always throw a FileException. (See FileException.hpp.)
Things to be aware of
Note that you'll find that the code will not compile and link properly until after you've finished your implementation, so if you immediately try to build it, that is expected to fail; you're not done yet.
We haven't discussed text files, but reading from them and writing to them is actually pretty straightforward, given that we've already used std::cin and std::cout. A std::ifstream is an input stream that reads from a file (that's what the if stands for), while a std::ofstream is an output stream that writes to a file. While most of what we need is pretty much the same as it is when using std::cin or std::cout, there are a couple of concepts that are added to the mix: Files need to be opened (and this can fail) and input files eventually reach an "end" (i.e., if a file has 100 lines in it, we can't continue reading from it once we've read all 100 of them). Lots of information about these parts of the C++ Standard Library can be found at cppreference.com, among other places.
FileException.hpp
#ifndef FILEEXCEPTION_HPP #define FILEEXCEPTION_HPP #includeclass FileException : public std::runtime_error { public: explicit FileException(const std::string& reason) : std::runtime_error{reason} { } }; #endif
problem2.hpp
#ifndef PROBLEM2_HPP #define PROBLEM2_HPP #includetemplate <typename LineType> class TextLineReader { public: using LineParser = std::function const std::string&)>; public: TextLineReader(const std::string& filePath, LineParser lineParser); ~TextLineReader(); TextLineReader(const TextLineReader& other) = delete; TextLineReader& operator=(const TextLineReader& other) = delete; bool hasNextLine() const; LineType nextLine(); }; #endif
main.cpp
#include#include #include #include "FileException.hpp" #include "problem2.hpp" int parseIntLine(const std::string& line) { int value; const char* begin = line.data(); const char* end = line.data() + line.size(); auto result = std::from_chars(begin, end, value); if (result.ptr != end || result.ec != std::errc{}) { throw FileException{"format error: every line must contain only an integer"}; } return value; } int main() { std::string filePath; std::getline(std::cin, filePath); try { TextLineReader<int> reader{filePath, parseIntLine}; while (reader.hasNextLine()) { int value = reader.nextLine(); std::cout << (value * value) << std::endl; } } catch (FileException& e) { std::cout << "FileException: " << e.what() << std::endl; } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
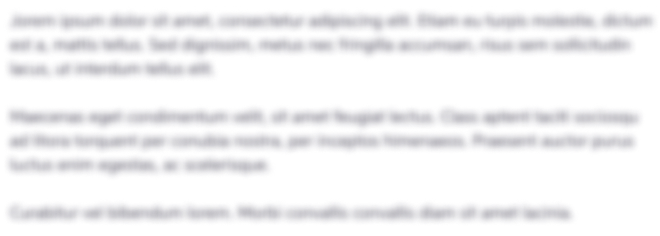
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started