Question
// help on the revision of Java Data structure HW //Each method contains the questions in a form of comments that needed to be implemented
// help on the revision of Java Data structure HW
//Each method contains the questions in a form of comments that needed to be implemented
+getIndex(item:T):int | Get position (index) of an item of type T, return -1 if not there |
+insertAt(index:int,item:T):boolean | Insert an item at position index, where index is passed to the method. Return true if the insertion took place and false if not (e.g., index out of bounds) |
import java.util.Scanner;
public class LList
{ Node head; Node back;
private class Node { T item; Node next;
public Node(T item, Node next) {
this.item = item; this.next = next;
}
public T getItem() { return item; }
} public LList() { super(); // TODO Auto-generated constructor stub }
public int getIndex(T item) { int position = 1; for (Node ptr = head; ptr != null; ptr = ptr.next) { if (ptr.item.equals(item)) { return position; } else { ++position;
} }
return 0; }
public boolean insertAt(int index, T item) { Node temp = head; Node prev = null; int i = 0; while (temp != null && i prev = temp; temp = temp.next; i++;
} return true; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
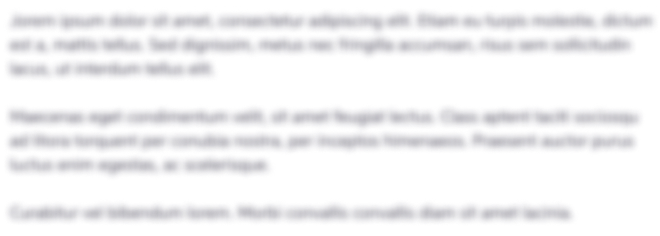
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started