Question
Help with C++ Problem. The Program is working and doing all that it should, but in order for the pair, twopairs, three of kind, fourofkind,
Help with C++ Problem. The Program is working and doing all that it should, but in order for the pair, twopairs, three of kind, fourofkind, flush and straight functions to work in main is if they take in a paramater. How can i make it so these functions dont need to have a parameter? Thank you for your help and tips.
//Card.h
#ifndef CARD_H
#define CARD_H
#include
#include
using namespace std;
// The main class header for the Card class.
class Card {
public:
// declares the public functions
Card(int, int);
void toString();
static string suits[4];
static string faces[13];
string getFace();
string getSuit();
private:
// declares the private fucntions.
string suit;
string face;
};
#endif
//DeckOfCards.h
#ifndef DECKOFCARDS_H
#define DECKOFCARDS_H
#include
#include "Card.h"
// The main class header for the DeckOfCards class.
class DeckOfCards {
public:
// Declares the public function
DeckOfCards();
void shuffle();
Card dealCard();
bool moreCards();
private:
vector deck;
int currentCard;
void swap(int, int);
};
#endif // !DECKOFCARDS_H
//PokerHand.h
#ifndef POKERHAND_H
#define POKERHAND_H
#include
#include "Card.h"
#include"DeckOfCards.h"
using namespace std;
class PokerHand {
private:
public:
PokerHand(vector&);
bool pair(vector&);
bool twoPairs(vector&);
bool threeOfKind(vector&);
bool fourOfKind(vector&);
bool flush(vector&);
bool straight(vector&);
};
#endif
//Card.cpp
#include "Card.h"
#include
#include
string Card::suits[4] = { "DIAMONDS","CLUBS","HEARTS","SPADES" };
string Card::faces[13] = { "2", "3", "4", "5", "6", "7", "8", "9", "10", "JACK", "QUEEN", "KING","ACE" };
/*
* Constructor used to initialize an instance of Cards.
*/
Card::Card(int type, int num) {
suit = suits[type];
face = faces[num];
}
/*
* The toString function prints out the card's face and suit.
*/
void Card::toString() {
string printout = face + " of " + suit;
cout << printout << endl;
// cout << face << " of " << suit;
}
/*
* The getFace function prints out the card's face.
*/
string Card::getFace() {
return face;
}
/*
* The getSuit function prints out the card's suit.
*/
string Card::getSuit() {
return suit;
}
//DeckOfCards.cpp
#include
#include
#include"DeckOfCards.h"
#include
#include
#include
unsigned seed;
using namespace std;
/*
* Constructor used to initialize an instance of DeckOfCards.
*/
DeckOfCards::DeckOfCards() {
currentCard = 0;
// Iterate through the different suits
for (int i = 0; i<4; i++) {
// Iterate through the different face values
for (int j = 0; j<13; j++) {
Card c = Card(i, j);
deck.push_back(c);
}
}
// Shuffle the cards.
shuffle();
}
/*
* Shuffle function to shuffle all of the cards in the deck.
*/
void DeckOfCards::shuffle() {
srand(time(0));
for (int count = 0; count<52; count++) {
int s = rand() % 52;
swap(count, s);
}
}
/*
* The swap function swaps two cards in the deck. The card at position a
* is moved to position b and the card at position b is moves to position a.
*/
void DeckOfCards::swap(int a, int b) {
Card tmp = deck[b];
deck[b] = deck[a];
deck[a] = tmp;
}
/*
* The dealCard function deals the next Card from the deck.
*/
Card DeckOfCards::dealCard() {
currentCard++;
if (moreCards()) {
return deck[currentCard - 1];
}
else {
cout << "The deck is empty." << endl;
}
}
/*
* The moreCards function checks to see if there are more Cards left to deal.
*/
bool DeckOfCards::moreCards() {
if (currentCard < 52) {
return true;
}
else {
return false;
}
}
//PokerHand.cpp
#include
#include
#include
#include"Card.h"
#include "DeckOfCards.h"
#include"PokerHand.h"
using namespace std;
PokerHand::PokerHand(vector& hand) {
// Set up the deck and deal the 5 cards.
DeckOfCards doc = DeckOfCards();
cout << "The cards you have been dealt are: " << endl;
for (int i = 0; i < 5; i++) {
hand.push_back(doc.dealCard());
hand[i].toString();
}
}
bool PokerHand::pair(vector& hand) {
// Check to see for pair(s).
int pairFound = 0;
for (int count = 0; count<5; count++) {
for (int j = count + 1; j<5; j++) {
if ((hand[count].getFace()).compare(hand[j].getFace()) == 0 && count != j) {
pairFound++;
}
}
}
if (pairFound == 1) {
cout << "A pair found!" << endl;
return true;
}
else
return false;
}
bool PokerHand::twoPairs(vector& hand) {
int twoPairsFound = 0;
for (int i = 0; i<5; i++) {
for (int j = i + 1; j<5; j++) {
if ((hand[i].getFace()).compare(hand[j].getFace()) == 0 && i != j) {
twoPairsFound++;
}
}
}
if (twoPairsFound == 2) {
cout << "Two pairs found!" << endl;
return true;
}
else
return false;
}
bool PokerHand::threeOfKind(vector& hand) {
int threeOfKindFound = 0;
for (int count = 0; count<5; count++) {
for (int x = count + 1; x<5; x++) {
for (int k = x + 1; k<5; k++) {
if ((hand[count].getFace()).compare(hand[x].getFace()) == 0 &&
(hand[count].getFace()).compare(hand[k].getFace()) == 0 &&
count != x != k) {
threeOfKindFound++;
}
}
}
}
if (threeOfKindFound) {
cout << "Three of a kind found!" << endl;
return true;
}
else
return false;
}
bool PokerHand::fourOfKind(vector& hand) {
int flag = 0;
for (int count = 0; count<5; count++) {
for (int j = count + 1; j<5; j++) {
for (int k = j + 1; k<5; k++) {
for (int l = k + 1; l<5; l++) {
if ((hand[count].getFace()).compare(hand[j].getFace()) == 0 &&
(hand[count].getFace()).compare(hand[k].getFace()) == 0 &&
(hand[count].getFace()).compare(hand[l].getFace()) == 0 &&
count != j != k != l) {
flag++;
}
}
}
}
}
if (flag) {
cout << "A quadruple found!" << endl;
return true;
}
else
return false;
}
bool PokerHand::flush(vector& hand) {
if ((hand[0].getSuit()).compare(hand[1].getSuit()) == 0 &&
(hand[0].getSuit()).compare(hand[2].getSuit()) == 0 &&
(hand[0].getSuit()).compare(hand[3].getSuit()) == 0 &&
(hand[0].getSuit()).compare(hand[4].getSuit()) == 0) {
cout << "A flush has been found!" << endl;
return true;
}
else
return false;
}
bool PokerHand::straight(vector& hand) {
vector vector2;
for (int count = 0; count<5; count++) {
for (int j = 0; j<13; j++) {
if ((hand[count].getFace()).compare(hand[count].faces[j]) == 0) {
vector2.push_back(j + 1);
}
}
}
sort(vector2.begin(), vector2.end());
if (vector2[0] == vector2[1] - 1 && vector2[0] == vector2[2] - 2 &&
vector2[0] == vector2[3] - 3 && vector2[0] == vector2[4] - 4) {
cout << "A straight found!" << endl;
return true;
}
else
return false;
}
//Main.cpp
#include
#include
#include
#include"Card.h"
#include "DeckOfCards.h"
#include"PokerHand.h"
using namespace std;
// Main method to begin inputing and executing code.
int main() {
DeckOfCards doc = DeckOfCards();
vector hand;
PokerHand ph(hand);
ph.pair(hand);
ph.twoPairs(hand);
ph.threeOfKind(hand);
ph.fourOfKind(hand);
ph.flush(hand);
ph.straight(hand);
system("pause");
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
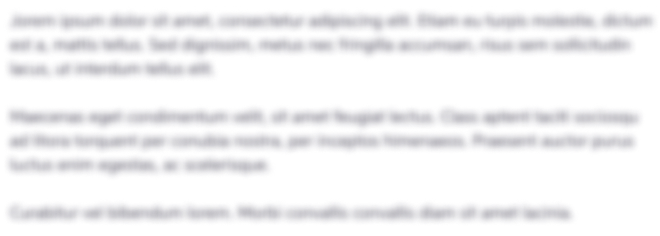
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started