Question
Help with code, I am trying to have my code run but I keep having trouble with it. Here is my code: arrayListType.h: #ifndef ARRAYLISTTYPE_H
Help with code, I am trying to have my code run but I keep having trouble with it. Here is my code:
arrayListType.h:
#ifndef ARRAYLISTTYPE_H #define ARRAYLISTTYPE_H
class arrayListType{ public: bool isEmpty() const; //function to see if the list is empty //postcondition: returns true if list is empty bool isFull() const; //function to see if the list is full //postcondition: returns true is list is full int listSize() const; //function to see number of elements in a list //postcondition: returns value of length int maxListSize() const; //function to see if the max size of list //postcondition: returns value of maxSize void print() const; //function to output the elements of the list //postcondition: elements of the list are output on the standard output bool isItemAtEqual(int location, int item) const; //function to see if item is the same as an item in the list at //specified location //postcondition: returns true is location is the same as item virtual void insertAt(int location, int insertItem) = 0; //function is to insert an item at the end of the list //postcondition: list[length] = insertItem; and length++; // if the list is out of range, a message will appear. virtual void insertEnd(int insertItem) = 0; //function to insert an item at the end of the list //postcondition: list[length] = insertItem; and length++; // if the list is full, a message will appear. void removeAt(int location); //function is to remove an item from the list //postcondition: the list of elements at list[location] is removed // and the length is decremented by 1. // a message will appear is out of range. void retrieveAt(int location, int& retItem) const; //function is to retrieve the element from the list at location. //postcondition: retItem = list[location] // if location is out of range, a message will appear virtual void replaceAt(int location, int repItem) = 0; //function is to replace the element in the list at location //postcondition: list[location] = repItem // if location is out of range, a message will appear void clearList(); //function to remove all elements from list. list size will be 0 //postcondition: length = 0 virtual void remove(int removeItem) = 0; //function to remove removeItem from list //postcondition: is removeItem is found in list, it will be removed // and list will decrement by one arrayListType(int size = 100); //constructor //creates an array of size specified. the default size is 100. //postcondition: the list points to the array, // length = 0, and maxSize = size; arrayListType(const arrayListType& otherList); //copy constructor virtual ~arrayListType(); //destructor protected: int *list; //array to hold list of elements int length; //variable to store length of list int maxSize; //variable to store max size of list };
#endif /* ARRAYLISTTYPE_H */
---------------------------------
orderedArrayListType.h:
#ifndef ORDEREDARRAYLISTTYPE_H #define ORDEREDARRAYLISTTYPE_H
#include "arrayListType.h"
class orderedArrayListType: public arrayListType{ public: void insertAt(int location, int insertItem); void insertEnd(int insertItem); void replaceAt(int location, int repItem); int seqSearch(int searchItem) const; void insert(int insertItem); void remove(int removeItem); orderedArrayListType(int size = 100); //constructor };
#endif /* ORDEREDARRAYLISTTYPE_H */
-------------------------------------
unorderedArrayListType.h:
#ifndef UNORDEREDARRAYLISTTYPE_H #define UNORDEREDARRAYLISTTYPE_H
#include "arrayListType.h"
class unorderedArrayListType: public arrayListType{ public: void insertAt(int location, int insertItem); void insertEnd(int insertItem); void replaceAt(int location, int repItem); int seqSearch(int seachItem) const; void remove(int removeItem); unorderedArrayListType(int size = 100); //constructor };
#endif /* UNORDEREDARRAYLISTTYPE_H */
-------------------------------------------
ArrayListType.cpp:
#include
using namespace std;
bool arrayListType::isEmpty() const{ return (length == 0); } //end isEmpty
bool arrayListType::isFull() const{ return (length == maxSize); } //end isFull
int arrayListType::listSize() const{ return length; } //end listSize
int arrayListType::maxListSize() const{ return maxSize; } //end maxListSize
void arrayListType::print() const{ for (int i = 0; i < length; i++) cout << list[i] << " "; cout << endl; } //end print
bool arrayListType::isItemAtEqual(int location, int item) const{ if (location < 0 || location >= length) { cout << "The location of the item to be removed " << "is out of range." << endl;
return false; } else return (list[location] == item); } //end isItemAtEqual
void arrayListType::removeAt(int location){ if (location < 0 || location >= length) cout << "The location of the item to be removed " << "is out of range." << endl; else { for (int i = location; i < length - 1; i++) list[i] = list[i+1];
length--; } } //end removeAt
void arrayListType::retrieveAt(int location, int& retItem) const{ if (location < 0 || location >= length) cout << "The location of the item to be retrieved is " << "out of range" << endl; else retItem = list[location]; } //end retrieveAt
void arrayListType::clearList(){ length = 0; } //end clearList
arrayListType::arrayListType(int size){ if (size <= 0) { cout << "The array size must be positive. Creating " << "an array of the size 100." << endl;
maxSize = 100; } else maxSize = size;
length = 0;
list = new int[maxSize]; } //end constructor
arrayListType::~arrayListType(){ delete [] list; } //end destructor
arrayListType::arrayListType(const arrayListType& otherList){ maxSize = otherList.maxSize; length = otherList.length;
list = new int[maxSize]; //create the array
for (int j = 0; j < length; j++) //copy otherList list [j] = otherList.list[j]; }//end copy constructor
--------------------------------------------
main.cpp:
#include
using namespace std;
int main(){
arrayListType list1(10);
list1.insertAt(0,1); cout << "Value at location 0 : " << list1.retrieveAt(0) << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
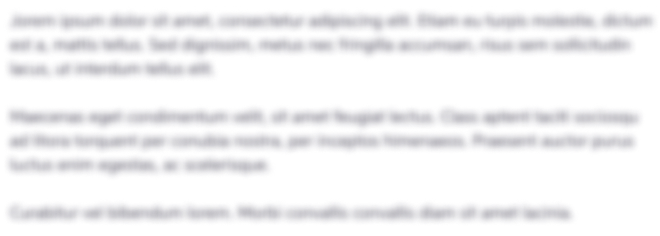
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started