Question
Help with Game of Life JavaFX desktop app with specific guidelines. Your GUI should have all of the components shown above: 1. a GridPane for
Help with Game of Life JavaFX desktop app with specific guidelines.
Your GUI should have all of the components shown above:
1. a GridPane for displaying the cells
2. a Step button for stepping to the next generation
3. a Play/Stop button for playing/stopping an animation that does multiple steps
4. a Rate: label
5. a Slider that affects the rate of the animation
6. a Clear button for clearing all of the cells
7. a pair of radio buttons for selecting between Life and HighLife
8. a single menu (File) with four menu items and two separators:
a. a Clear item that does exactly the same thing as the Clear button mentioned above
b. a separator
c. a Save As item for saving to a file the current state of the simulation, including whether it is a game of Life or High Life
d. a Load Game item for reading and loading the contents of a save file
e. a separator
f. an Exit item for quitting the program using System.exit(0)
HighLife (https://en.wikipedia.org/wiki/Highlife_(cellular_automaton)) is the same as Life except that a non-living cell comes to life if its number of live neighbors is 3 or 6.
When the Save As or Load Game menu item is chosen, you are required to use a FileChooser object to select the file. The Save As should bring up a save dialog. Load should bring up an open dialog.
Unlike the web app, your game of life should wrap around: For the purposes of computing which neighbors are alive, the bottom row should be considered adjacent to the top row and the left column should be considered adjacent to the right column. You could do this by writing if-statements to check for being on the far top, bottom, left, or right as special cases, but it is more concise to use the mod operator, %, as follows:
int liveCount = 0;
for (int i = -1; i
for (int j = -1; j
if (cell[(row + i + DIM) % DIM][(col + j + DIM) % DIM].isAlive)
liveCount++;
The above assumes that you are trying to count the number of live cells surrounding cell[row][col]. DIM is assumed to be a constant that indicates the number of rows and columns in the grid. (DIM is 32 in the first screenshot above.) Declaring a constant such as DIM is good programming practice and makes it much easier to modify the program if we want to change the size of the grid. You should declare such a constant in your program.
Note that the above code includes cell[row][col] in the count, but a cell is not considered a neighbor of itself, so an adjustment needs to be made after the loop if cell[row][col] is alive.
Some details about the Step and Play/Stop buttons:
Whenever the animation is not playing (including when the program starts) the Step button should be enabled and the Play/Stop button should display Play.
Whenever the animation is playing, the Step button should be disabled (execute btnStep.setDisable(true)) and the Play/Stop button should display Stop.
When the user selects Clear, Save As, or Load Game the animation should stop. In order to make it easy to keep track of this logic, it is probably worthwhile having methods for playing and stopping the animation:
Method for playing the animation:
call play() method on the animation
set the text on the Play/Stop button to Stop
set the disable on the Step button to true.
Method for stopping the animation:
call stop() method on the animation
set the text on the Play/Stop button to Play
set the disable on the Step button to false.
If all cells die in an animation then the animation automatically stops the user does not need to click the Stop button to stop it.
The clear button clears everything out to the never alive state.
Use three colors in the simulation rather than two. The first color is for live cells, the second for cells that were alive but are now dead, and the third is for cells that have never been alive so far in the current simulation.
Add a button labeled Random. When the Random button is clicked the game is cleared and then repopulated at random.
In conjunction with the above Random button, provide a JavaFX spinner in the GUI that allows the user to select the probability of a cell being alive. For example, if the user has selected 30 in the spinner, and then hits the Random button, then the game will be cleared and repopulated at random, with each cell having a 30 percent chance of being alive.
Allow the user to set cells alive by dragging the left mouse button across them, and set them to dead by dragging the right mouse button across them. This one seems kind of tricky. My suggestion is to use the setOnDragDetected, startFullDrag, and setOnMouseDragEntered methods in the Node class. Each of your Cells will be a Node. In your Cell constructor you might write something like the following: this.setOnDragDetected(e -> this.startFullDrag()); this.setOnMouseDragEntered(e -> );
Game of Life File Step Play Rate: Clear Life HighLife Game of Life File Step Play Rate: Clear Life HighLifeStep by Step Solution
There are 3 Steps involved in it
Step: 1
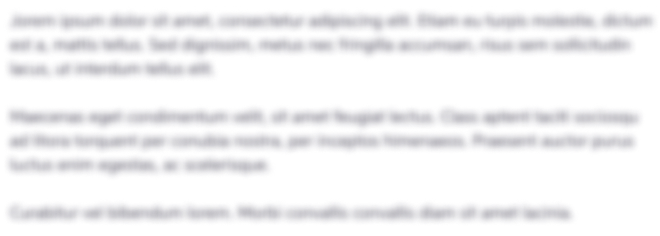
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started