Question
Help with this java assignment Array Set package arraysetpackage; import java.util.Iterator; import java.util.NoSuchElementException; import java.util.Random; public class ArraySet implements SetADT { private static final int
Help with this java assignment
Array Set
package arraysetpackage;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Random;
public class ArraySet
private static final int DEFAULT_SIZE = 20;
private int count;
private T[] setValues;
private Random rand;
public ArraySet (){
this(DEFAULT_SIZE);
} // end of constructor
public ArraySet (int size){
count = 0;
setValues = (T[]) new Object[size];
rand = new Random();
} // end of constructor
public void add(T element) {
if (contains(element))
return;
if (count == setValues.length) {
T[] temp = (T[]) new Object[setValues.length*2];
for (int i = 0; i
temp[i] = setValues[i];
}
setValues = temp;
}
setValues[count] = element;
count++;
}
public void addAll(SetADT
// finish: this method adds all of the input sets elements to this array
}
public boolean contains(T target) {
for (int i = 0; i
if (setValues[i].equals(target))
return true;
return false;
}
public String toString () {
String toReturn = "[";
for (int i = 0; i
toReturn += setValues[i] + " ";
}
toReturn +="]";
return toReturn;
}
public boolean equals(SetADT
// finish: tests to see if this set and the input set have exactly the same
// elements
return false; // this is just generic, you need to change the return
}
public boolean isEmpty() {
return count==0;
}
public Iterator
return new ArraySetIterator
}
public T remove(T element) {
for (int i = 0; i
if (setValues[i].equals(element)) {
T toReturn = setValues[i];
setValues[i] = setValues[count-1];
count--;
return toReturn;
}
}
throw new NoSuchElementException("not present");
}
public T removeRandom() {
// finish: remove and return a random element. you will use the
// local rand object
return null; // this is just generic, you need to change the return
}
public int size() {
return count;
}
public SetADT
// finish: a new set is created and returned. This new set will
// contain all of elements from this set and the input parameter set
return null; // this is just generic, you need to change the return
}
}
array set iterator
package arraysetpackage;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class ArraySetIterator
private int position; //Always points to the next value
private T [] values;
private int count;
public ArraySetIterator (T [] theValues, int aCount) {
position = 0;
values = theValues;
count = aCount;
}
public boolean hasNext() {
return position
}
public T next() {
if (position >= count)
throw new NoSuchElementException("Past " + count + " elements");
position++;
return values[position - 1];
}
public void remove() {
throw new
UnsupportedOperationException("No remove for ArraySet");
}
}
array set tester
package arraysetpackage;
import java.util.Iterator;
public class ArraySetTester {
public static void main(String[] args) {
SetADT
for (int i = 0; i
mySet.add(new String("apple"+i));
System.out.println(mySet);
System.out.println("mysize = "+mySet.size()+ " [expect 12]");
mySet.add(new String ("apple0"));
System.out.println("mysize = "+mySet.size()+ " [expect 12]");
System.out.println("contains 11? = "+mySet.contains(new String("11")));
System.out.println("contains apple11? = "+mySet.contains(new String("apple11")));
try {
String removedItem = mySet.remove("apple7");
System.out.println(mySet);
System.out.println(removedItem+ " was removed");
} catch (Exception e) {
System.out.println("item not found, can't remove");
}
try {
String removedItem = mySet.remove("apple17");
System.out.println(mySet);
System.out.println(removedItem+ " was removed");
} catch (Exception e) {
System.out.println("item not found, can't remove");
}
Iterator
while (iter.hasNext()){
System.out.println(iter.next());
}
SetADT
for (int i = 0; i
mySet2.add(new String("orange"+i));
System.out.println(mySet2);
// add code here to test methods you finish in ArraySet
// after you complete the existing methods, do the Case Study
// Approach 1 will be here in the main
// Approach 2 will be here in ArraySetTester, but you will
// create a local static method that you will call from the main
// Approach 3 will start with uncommenting the prototype in SetADT
// and then creating the method in ArraySet. Finally you will write
// code here to test the new method
}
}
Set ADT
package arraysetpackage;
import java.util.Iterator;
public interface SetADT
public void add (T element); //Adds one element to this set, ignoring duplicates
public void addAll (SetADT
// ignoring duplicates
public T removeRandom (); //Removes and returns a random element from this set
public T remove (T element); //Removes and returns the specified element from this set
public SetADT
// parameter
public boolean contains (T target); //Returns true if this set contains the parameter
//public boolean contains(SetADT
public boolean equals (SetADT
//contain exactly same elements
public boolean isEmpty(); //Returns true if this set contains no elements
public int size(); //Returns the number of elements in this set
public Iterator
public String toString(); //Returns a string representation of this set
}
Case study: Three implementations of contains - comparing approaches to adding functionality Set A contains Set B if every element in Set B is also in Set A. We will compare three ways to determine whether Set A contains Set B Approach 1: Write code in the main method in a test class fill them with data and write a Add code to the main of ArraySetTester to create SetA and SetB, contains operation that tests if SetA contains SetB Question: What is wrong with this approach? Answer: While the code does the operation for the specific collections SetA and SetB, it only applies to these two collections. The code can't be used for other Sets without repeating it. Approach 2: Write a static method in a test class Comment out the code from approach 1 Add a static method to ArraySetTester with prototype public static boolean contains (SetADTStep by Step Solution
There are 3 Steps involved in it
Step: 1
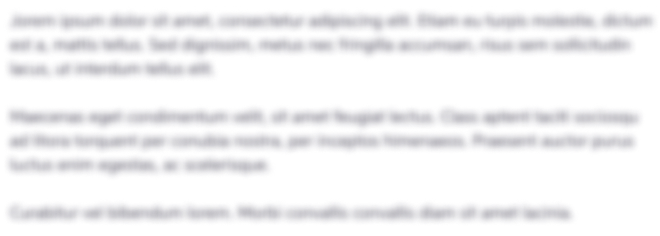
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started