Question
Here are my code: (which about the student list + major) Please help me when user typing their names and major and then submit it.
Here are my code: (which about the student list + major) Please help me when user typing their names and major and then submit it. i want to save those name. When reopen it their names still there on GUI swing package controller;
import model.StudentDAO; import view.MainGui;
public class MainController { private StudentController studentController; private MainGui mainGui; public MainController(StudentDAO studentDAO) { this.mainGui = new MainGui(); this.studentController = new StudentController(studentDAO, mainGui); this.mainGui.setStudentController(studentController); this.mainGui.setVisible(true); } public static void main (String[] args) { StudentDAO studentDAO = new StudentDAO(); new MainController(studentDAO); } } package controller;
import model.Student; import model.StudentDAO; import view.MainGui; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.List;
public class StudentController implements ActionListener { private StudentDAO studentDAO; private MainGui mainGui; public StudentController(StudentDAO studentDAO, MainGui mainGui) { this.studentDAO = studentDAO; this.mainGui = mainGui; mainGui.addGetNameButtonListener(this) updateView(); }
private void updateView() { List
public void addStudent(Student student) { studentDAO.addStudent(student); updateView(); } public void updateStudent(Student oldStudent, Student newStudent) { studentDAO.updateStudent(oldStudent, newStudent); updateView(); } public void deleteStudent(Student student) { studentDAO.deleteStudent(student); updateView(); } @Override public void actionPerformed(ActionEvent e) { if (e.getSource() == mainGui.getGetNameButton()) { openNameInputGui(); } }
public void openNameInputGui() { NameInputGui nameInputGui = new NameInputGui(mainGui, (ActionListener) this); nameInputGui.setVisible(true); } } package model;
public class Student { private String name; private String major; public Student(String name, String major) { this.name = name; this.major = major; } public String getName() { return name; } public String getMajor() { return major; } @Override public String toString() { return "Name: " + name + ", Major: " + major; } } package model;
import java.util.ArrayList; import java.util.List;
public class StudentDAO { private List
import controller.StudentController; import model.Student; import javax.swing.*; import java.awt.event.*;
public class NameInputGui extends javax.swing.JFrame { private JTextField nameField; private JTextField majorField; private JButton submitButton; private MainGui mainGui; private StudentController studentController; public NameInputGui(MainGui mainGui, ActionListener actionListener) { this.mainGui = mainGui; this.studentController = studentController; initComponents(); } private void initComponents() { JPanel inputPanel = new JPanel(); nameField = new JTextField(20); majorField = new JTextField(20); submitButton = new JButton("Submit"); inputPanel.setLayout(new BoxLayout(inputPanel, BoxLayout.Y_AXIS)); inputPanel.add(new JLabel("Name:")); inputPanel.add(nameField); inputPanel.add(new JLabel("Major:")); inputPanel.add(majorField); inputPanel.add(submitButton); // Add inputPanel to the frame add(inputPanel); // Create the frame's properties setTitle("Name Input"); setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); pack(); setLocationRelativeTo(null); submitButton.addActionListener(e -> submitNameInput()); } private void submitNameInput() { String name = nameField.getText(); String major = majorField.getText(); Student newStudent = new Student(name, major); if(studentController != null) { studentController.addStudent(newStudent); } dispose(); } } Please help me edit this code to match this topic created a GUI front-ended, persistent back-ended application.
Try to follow the three-tier design pattern - keep the code related to the back end in it's own classes/package (the model), the frames in their own package (the view), and the code to translate between them in it's own package (the Controller). Pass POJOs (Plain Old Java Object) to the View from the controller. Use the controller classes to pull data from the back end and convert it into Java objects. The model will be opened/closed/updated with methods from the Controller. In other words, the Controller classes should be your go-between to send data from the back-end to the front-end. For the Controller classes, follow the books DAO design pattern.
The design / subject of the program is up to you, but it needs to have at least three screens (one main screen that will spawn others) and provide CRUD functionality - the ability to CREATE new data to add to the back end, READ data from the back end, UPDATE existing values, and DELETE values - for at least two different types of objects.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
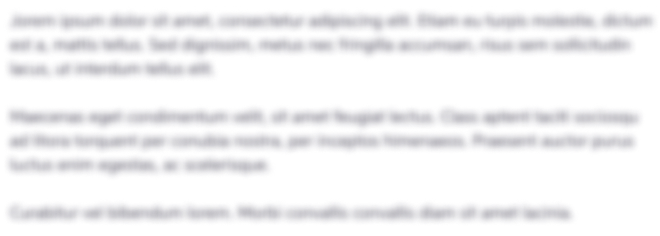
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started