Question
Here are the components of the project, the class declaration and implementation code should be defined separately in .h and .cpp files, a separate tester
Here are the components of the project, the class declaration and implementation code should be defined separately in .h and .cpp files, a separate tester program should be defined to implement object-oriented programming to perform the online banking
Declare and implement class Customer with at least the following members: Data members:
firstName
lastName
address
Member functions:
Customer() - default constructor without parameter.
Customer() - overloaded constructor with parameters of initial value of all data members.
void displayCustomer() - display all customers information.
Declare and implement an abstract base class Account with at least the following members: Data members:
accountNumber
balance
user - should implement composition to create an object of Customer class.
Member functions:
Account() - default constructor without parameter.
Account() - overloaded constructor with parameters of initial value of all data members.
double getBalance() - return current balance of an account.
void setBalance() update balance with argument value.
bool deposit(double amount) -
parameter is the deposit money entered by user
only perform deposit transaction for valid amount and return true, otherwise, return false without changing the balance.
bool withdraw(double amount) -
parameter is the withdraw money entered by user
only perform withdraw and return true if amount is valid and there is sufficient balance in the account. Otherwise, return false without changing the balance.
virtual void displayAccount()
this function should be declared as a virtual function.
it will be overridden by the function in the derived classes in order to implement polymorphism in the tester program.
it should display account number, balance and account users information by calling Customers displayCustomer() function
Declare and implement a derived class CheckingAccount which inherits from base class Account and includes at least the following members: Data members:
transactionFee
Member functions:
CheckingAccount() constructor with parameters of initial value of all data members including the data members inherited from the base class Account.
double getTransactionFee() return the transactionFee
bool deposit(double amount)
override the deposit function in base class Account.
call Accounts deposit function to implement deposit.
charge the transaction fee only if the deposit is implemented successfully by the base class.
bool withdraw(double amount)
override the withdraw function in base class Account
call Accounts withdraw function to implement withdraw.
charge the transaction fee only if the withdraw is implemented successfully by the base class.
void displayAccount()
keep the same name as that in the base class Account in order to implement polymorphism in the tester program.
Override the displayAccount function in the base class by displaying the title of Checking Account and transaction fee.
Declare and implement a derived class SavingsAccount which inherits from base class Account and includes at least the following members: Data members:
interestRate
Member functions:
SavingsAccount(.) - constructor with parameters of initial value of all data members including the data members inherited from the base class Account.
double getInterestRate() return the interestRate
double calcInterest()
calculate the interest, suppose interest = balance * interestRate.
update balance by adding the interest.
return interest.
void displayAccount()-
keep the same name as that in the base class Account in order to implement polymorphism in the tester program.
Override the displayAccount function in the base class by displaying the title of Savings Account, interestRate and interest.
Implement the following tasks in the tester program:
Ask user to enter users information.
Create an object of CheckingAccount for the customer using the input data.
Default account number is 1111.
Transaction fee is $5.00 for each transaction.
Create an object of SavingsAccount for the customer using the input data.
Default account number is 2222.
Interest rate is 2%.
Define a loop to display the service menu and ask user to select the service continually.
Define switch or nested selection structure to process users selected transaction.
Implement object-oriented programming to process users selected transaction.
Implement dynamic binding polymorphism for Display accounts option
Create a dynamic array of Account with 2 elements.
Store the address of checking account object and savings account object in the array.
Use a for loop to call displayAccount function, the derived class version will be implemented to display checking accounts information and savings accounts information appropriately.
Use could exit the program appropriately.
Here is the template of tester program
#include
#include
#include "Account.h"
#include "CheckingAccount.h"
#include "SavingsAccount.h"
using namespace std;
int main()
{
int selection = 0;
string firstName = "";
string lastName = "";
string address = "";
string email = "";
double amount = 0.0;
// input users information
// write your code here
// create CheckingAccount object
// write your code here
// create SavingsAccount object
// write your code here
cout << "Your checking account and savings account are created successfully. " << endl << endl;
// display the menu and ask for the first selection from user
// write your code here
while (selection != 8)
{
switch(selection)
{
case 1:
// implement polymorphism
// to display accounts information
case 2:
// deposit to checking account
// write your code here
case 3:
// deposit to savings account
// write your code here
case 4:
// withdraw from checking account
// write your code here
case 5:
// withdraw from savings account
// write your code here
case 6:
// transfer money from checking account to savings account
// write your code here
case 7:
// transfer money from savings account to checking account
// write your code here
default:
cout << "Invalid selection." << endl;
}
// display the menu and ask for next selection from user
// write your code here
}
cout << "Thanks for using Bank of Wake!" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
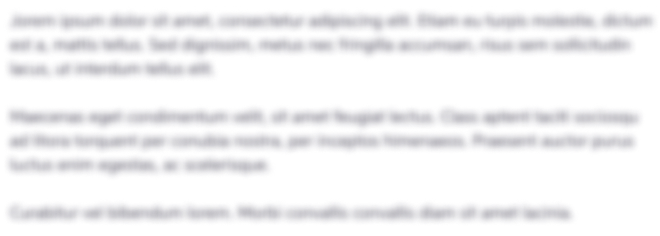
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started