Question
Here I have attached the source code needed to adjust edit for this assignment. USE THE FOLLOWING SOURCE: --------------------------------------------------------------------------------------------- ResizableArrayBag.java --------------------------------------------------------------------------------------------- import java.util.Arrays; public final
Here I have attached the source code needed to adjust edit for this assignment. USE THE FOLLOWING SOURCE:
---------------------------------------------------------------------------------------------
ResizableArrayBag.java
---------------------------------------------------------------------------------------------
import java.util.Arrays; public final class ResizableArrayBagimplements BagInterface { private T[] bag; // Cannot be final due to doubling private int numberOfEntries; private boolean initialized = false; private static final int DEFAULT_CAPACITY = 25; // Initial capacity of bag private static final int MAX_CAPACITY = 10000; /** Creates an empty bag whose initial capacity is 25. */ public ResizableArrayBag() { this(DEFAULT_CAPACITY); } // end default constructor /** Creates an empty bag having a given initial capacity. @param initialCapacity The integer capacity desired. */ public ResizableArrayBag(int initialCapacity) { checkCapacity(initialCapacity); // The cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] tempBag = (T[])new Object[initialCapacity]; // Unchecked cast bag = tempBag; numberOfEntries = 0; initialized = true; } // end constructor /** Creates a bag containing given entries. @param contents An array of objects. */ public ResizableArrayBag(T[] contents) { checkCapacity(contents.length); bag = Arrays.copyOf(contents, contents.length); numberOfEntries = contents.length; initialized = true; } // end constructor /** Adds a new entry to this bag. @param newEntry The object to be added as a new entry. @return True. */ public boolean add(T newEntry) { checkInitialization(); if (isArrayFull()) { doubleCapacity(); } // end if bag[numberOfEntries] = newEntry; numberOfEntries++; return true; } // end add /** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in this bag. */ public T[] toArray() { checkInitialization(); // The cast is safe because the new array contains null entries. @SuppressWarnings("unchecked") T[] result = (T[])new Object[numberOfEntries]; // Unchecked cast for (int index = 0; index /** Sees whether this bag is empty. @return True if this bag is empty, or false if not. */ public boolean isEmpty() { return numberOfEntries == 0; } // end isEmpty /** Gets the current number of entries in this bag. @return The integer number of entries currently in this bag. */ public int getCurrentSize() { return numberOfEntries; } // end getCurrentSize /** Counts the number of times a given entry appears in this bag. @param anEntry The entry to be counted. @return The number of times anEntry appears in this ba. */ public int getFrequencyOf(T anEntry) { checkInitialization(); int counter = 0; for (int index = 0; index /** Tests whether this bag contains a given entry. @param anEntry The entry to locate. @return True if this bag contains anEntry, or false otherwise. */ public boolean contains(T anEntry) { checkInitialization(); return getIndexOf(anEntry) > -1; // or >= 0 } // end contains /** Removes all entries from this bag. */ public void clear() { while (!isEmpty()) remove(); } // end clear /** Removes one unspecified entry from this bag, if possible. @return Either the removed entry, if the removal was successful, or null. */ public T remove() { checkInitialization(); T result = removeEntry(numberOfEntries - 1); return result; } // end remove /** Removes one occurrence of a given entry from this bag. @param anEntry The entry to be removed. @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry) { checkInitialization(); int index = getIndexOf(anEntry); T result = removeEntry(index); return anEntry.equals(result); } // end remove // Locates a given entry within the array bag. // Returns the index of the entry, if located, // or -1 otherwise. // Precondition: checkInitialization has been called. private int getIndexOf(T anEntry) { int where = -1; boolean found = false; int index = 0; while (!found && (index -1, anEntry is in the array bag, and it // equals bag[where]; otherwise, anEntry is not in the array. return where; } // end getIndexOf // Removes and returns the entry at a given index within the array. // If no such entry exists, returns null. // Precondition: 0 = 0)) { result = bag[givenIndex]; // Entry to remove int lastIndex = numberOfEntries - 1; bag[givenIndex] = bag[lastIndex]; // Replace entry to remove with last entry bag[lastIndex] = null; // Remove reference to last entry numberOfEntries--; } // end if return result; } // end removeEntry // Returns true if the array bag is full, or false if not. private boolean isArrayFull() { return numberOfEntries >= bag.length; } // end isArrayFull // Doubles the size of the array bag. // Precondition: checkInitialization has been called. private void doubleCapacity() { int newLength = 2 * bag.length; checkCapacity(newLength); bag = Arrays.copyOf(bag, newLength); } // end doubleCapacity // Throws an exception if the client requests a capacity that is too large. private void checkCapacity(int capacity) { if (capacity > MAX_CAPACITY) throw new IllegalStateException("Attempt to create a bag whose capacity exceeds " + "allowed maximum of " + MAX_CAPACITY); } // end checkCapacity // Throws an exception if receiving object is not initialized. private void checkInitialization() { if (!initialized) throw new SecurityException ("Uninitialized object used " + "to call an ArrayBag method."); } // end checkInitialization } // end ResizableArrayBag
---------------------------------------------------------------------------------------------------------------------
BagInterface.java
---------------------------------------------------------------------------------------------------------------------
public interface BagInterface{ /** Gets the current number of entries in this bag. @return The integer number of entries currently in the bag. */ public int getCurrentSize(); /** Sees whether this bag is empty. @return True if the bag is empty, or false if not. */ public boolean isEmpty(); /** Adds a new entry to this bag. @param newEntry The object to be added as a new entry. @return True if the addition is successful, or false if not. */ public boolean add(T newEntry); /** Removes one unspecified entry from this bag, if possible. @return Either the removed entry, if the removal. was successful, or null. */ public T remove(); /** Removes one occurrence of a given entry from this bag. @param anEntry The entry to be removed. @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry); /** Removes all entries from this bag. */ public void clear(); /** Counts the number of times a given entry appears in this bag. @param anEntry The entry to be counted. @return The number of times anEntry appears in the bag. */ public int getFrequencyOf(T anEntry); /** Tests whether this bag contains a given entry. @param anEntry The entry to locate. @return True if the bag contains anEntry, or false if not. */ public boolean contains(T anEntry); /** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in the bag. Note: If the bag is empty, the returned array is empty. */ public T[] toArray(); // public T[] toArray(); // Alternate // public Object[] toArray(); // Alternate /** Creates a new bag that combines the contents of this bag and anotherBag. @param anotherBag The bag that is to be added. @return A combined bag. */ // public BagInterface union(BagInterface anotherBag); /** Creates a new bag that contains those objects that occur in both this bag and anotherBag. @param anotherBag The bag that is to be compared. @return A combined bag. */ // public BagInterface intersection(BagInterface anotherBag); /** Creates a new bag of objects that would be left in this bag after removing those that also occur in anotherBag. @param anotherBag The bag that is to be removed. @return A combined bag. */ // public BagInterface difference(BagInterface anotherBag); } // end BagInterface
---------------------------------------------------------------------------------------------------------------------
SetInterface.java (You may not need this class, however if you do it's here)
---------------------------------------------------------------------------------------------------------------------
public interface SetInterface{ public int getCurrentSize(); public boolean isEmpty(); /** Adds a new entry to this set, avoiding duplicates. @param newEntry The object to be added as a new entry. @return True if the addition is successful, or false if the item is already in the set. */ public boolean add(T newEntry); /** Removes a specific entry from this set, if possible. @param anEntry The entry to be removed. @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry); public T remove(); public void clear(); public boolean contains(T anEntry); public T[] toArray(); } // end SetInterface
---------------------------------------------------------------------------------------------------------------------
ResizableArrayBagDemo.java
---------------------------------------------------------------------------------------------------------------------
public class ResizableArrayBagDemo { public static void main(String[] args) { // A bag whose initial capacity is small BagInterfaceaBag = new ResizableArrayBag(3); testIsEmpty(aBag, true); System.out.println("Adding to the bag more strings than its initial capacity."); String[] contentsOfBag = {"A", "D", "B", "A", "C", "A", "D"}; testAdd(aBag, contentsOfBag); testIsEmpty(aBag, false); String[] testStrings2 = {"A", "B", "C", "D", "Z"}; testFrequency(aBag, testStrings2); testContains(aBag, testStrings2); // Removing strings String[] testStrings3 = {"", "B", "A", "C", "Z"}; testRemove(aBag, testStrings3); System.out.println(" Clearing the bag:"); aBag.clear(); testIsEmpty(aBag, true); displayBag(aBag); } // end main // Tests the method add. private static void testAdd(BagInterface aBag, String[] content) { System.out.print("Adding to the bag: "); for (int index = 0; index aBag, String[] tests) { for (int index = 0; index aBag, boolean correctResult) { System.out.print("Testing isEmpty with "); if (correctResult) System.out.println("an empty bag:"); else System.out.println("a bag that is not empty:"); System.out.print("isEmpty finds the bag "); if (correctResult && aBag.isEmpty()) System.out.println("empty: OK."); else if (correctResult) System.out.println("not empty, but it is empty: ERROR."); else if (!correctResult && aBag.isEmpty()) System.out.println("empty, but it is not empty: ERROR."); else System.out.println("not empty: OK."); System.out.println(); } // end testIsEmpty // Tests the method getFrequencyOf. private static void testFrequency(BagInterface aBag, String[] tests) { System.out.println(" Testing the method getFrequencyOf:"); for (int index = 0; index aBag, String[] tests) { System.out.println(" Testing the method contains:"); for (int index = 0; index aBag) { System.out.println("The bag contains " + aBag.getCurrentSize() + " string(s), as follows:"); Object[] bagArray = aBag.toArray(); for (int index = 0; index Open Resizable ArrayBag and rename as ArraySet.java Re-write 'add' methods to prevent the addition of duplicate entries, using We can remove getFrequencyOf' method. - Global replace of 'Bag' with Set' either the contains' method or getIndexOf Update the test class - replace the 'testGetFrequencyOf method and add a method to verify that duplicates can't be added
Step by Step Solution
There are 3 Steps involved in it
Step: 1
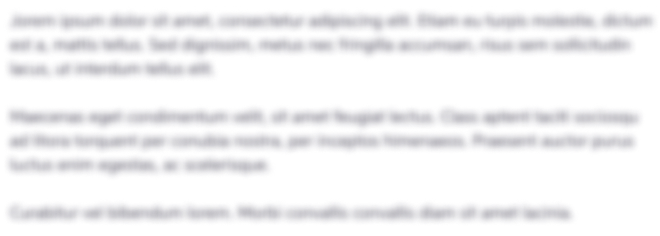
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started