Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
Here is a C + + class definition for an abstract data type Map from string to double, representing the concept of a function mapping
Here is a C class definition for an abstract data type Map from string to double, representing the concept of a function mapping strings to doubles. For example, we could represent a collection of students and their GPAs: "Fred" maps to "Ethel" maps to "Lucy" maps to etc. We'll call the strings the keys and the doubles the values. No two keys in the map are allowed to be the same eg so "Fred" appears no more than once as a key although two keys might map to the same value as in the example, where both of the keys "Fred" and "Lucy" map to the value To make things simpler for you, the case of letters in a string matters, so that the strings Fred and fReD are not considered duplicates. class Map public: Map; Create an empty map ie one whose size is bool empty; Return true if the map is empty, otherwise false. int size; Return the number of keyvalue pairs in the map. bool insertconst std::string& key, const double& value; If key is not equal to any key currently in the map and if the keyvalue pair can be added to the map, then do so and return true. Otherwise, make no change to the map and return false indicating that either the key is already in the map, or the map has a fixed capacity and is full bool updateconst std::string& key, const double& value; If key is equal to a key currently in the map, then make that key no longer map to the value that it currently maps to but instead map to the value of the second parameter; in this case, return true. Otherwise, make no change to the map and return false. bool insertOrUpdateconst std::string& key, const double& value; If key is equal to a key currently in the map, then make that key no longer map to the value that it currently maps to but instead map to the value of the second parameter; in this case, return true. If key is not equal to any key currently in the map, and if the keyvalue pair can be added to the map, then do so and return true. Otherwise, make no change to the map and return false indicating that the key is not already in the map and the map has a fixed capacity and is full bool eraseconst std::string& key; If key is equal to a key currently in the map, remove the keyvalue pair with that key from the map and return true. Otherwise, make no change to the map and return false. bool containsconst std::string& key; Return true if key is equal to a key currently in the map, otherwise false. bool getconst std::string& key, double& value; If key is equal to a key currently in the map, set value to the value in the map which the key maps to and return true. Otherwise, make no change to the value parameter of this function and return false. bool getint i std::string& key, double& value; If i size copy into the key and value parameters the key and value of the keyvalue pair in the map whose key is strictly greater than exactly i keys in the map and return true. Otherwise, leave the key and value parameters unchanged and return false. void swapMap& other; Exchange the contents of this map with the other one. ; When we don't want a function to change a key or value parameter, we pass that parameter by constant reference. Passing it by value would have been perfectly fine for this problem, but we're requiring you to use the const reference alternative because that will be more suitable after we make some generalizations in a later problem. Notice that the comment for the get function implies that for a item map mm mmget x y will copy the least key in the map into x and its corresponding value into y because the least key is greater than keys in the map; mmget x y will copy the greatest key and its corresponding value because the greatest item is greater than items in the map The words greater than, least, etc., are all interpreted in the context of what the operator for string indicates about the relative order of two strings: Map mm; mminsertLittle Ricky", ; mminsertEthel; mminsertRicky; mminsertLucy; mminsertEthel; mminsertFred; mminsertLucy; assertmmsize; duplicate "Ethel" and "Lucy" were not added string x; double y; mmget x y; assertx "Ethel"; "Ethel" is greater than exactly items in mm mmget x y; assertx "Ricky"; "Ricky" is greater than exactly items in mm mmget x y; assertx
Here is a C class definition for an abstract data type Map from string to double, representing the concept of a function mapping strings to doubles. For example, we could represent a collection of students and their GPAs: "Fred" maps to "Ethel" maps to "Lucy" maps to etc. We'll call the strings the keys and the doubles the values. No two keys in the map are allowed to be the same eg so "Fred" appears no more than once as a key although two keys might map to the same value as in the example, where both of the keys "Fred" and "Lucy" map to the value To make things simpler for you, the case of letters in a string matters, so that the strings Fred and fReD are not considered duplicates.
class Map
public:
Map; Create an empty map ie one whose size is
bool empty; Return true if the map is empty, otherwise false.
int size; Return the number of keyvalue pairs in the map.
bool insertconst std::string& key, const double& value;
If key is not equal to any key currently in the map and if the
keyvalue pair can be added to the map, then do so and return true.
Otherwise, make no change to the map and return false indicating
that either the key is already in the map, or the map has a fixed
capacity and is full
bool updateconst std::string& key, const double& value;
If key is equal to a key currently in the map, then make that key no
longer map to the value that it currently maps to but instead map to
the value of the second parameter; in this case, return true.
Otherwise, make no change to the map and return false.
bool insertOrUpdateconst std::string& key, const double& value;
If key is equal to a key currently in the map, then make that key no
longer map to the value that it currently maps to but instead map to
the value of the second parameter; in this case, return true.
If key is not equal to any key currently in the map, and if the
keyvalue pair can be added to the map, then do so and return true.
Otherwise, make no change to the map and return false indicating
that the key is not already in the map and the map has a fixed
capacity and is full
bool eraseconst std::string& key;
If key is equal to a key currently in the map, remove the keyvalue
pair with that key from the map and return true. Otherwise, make
no change to the map and return false.
bool containsconst std::string& key;
Return true if key is equal to a key currently in the map, otherwise
false.
bool getconst std::string& key, double& value;
If key is equal to a key currently in the map, set value to the
value in the map which the key maps to and return true. Otherwise,
make no change to the value parameter of this function and return
false.
bool getint i std::string& key, double& value;
If i size copy into the key and value parameters the
key and value of the keyvalue pair in the map whose key is strictly
greater than exactly i keys in the map and return true. Otherwise,
leave the key and value parameters unchanged and return false.
void swapMap& other;
Exchange the contents of this map with the other one.
;
When we don't want a function to change a key or value parameter, we pass that parameter by constant reference. Passing it by value would have been perfectly fine for this problem, but we're requiring you to use the const reference alternative because that will be more suitable after we make some generalizations in a later problem.
Notice that the comment for the get function implies that for a item map mm mmget x y will copy the least key in the map into x and its corresponding value into y because the least key is greater than keys in the map; mmget x y will copy the greatest key and its corresponding value because the greatest item is greater than items in the map The words greater than, least, etc., are all interpreted in the context of what the operator for string indicates about the relative order of two strings:
Map mm;
mminsertLittle Ricky", ;
mminsertEthel;
mminsertRicky;
mminsertLucy;
mminsertEthel;
mminsertFred;
mminsertLucy;
assertmmsize; duplicate "Ethel" and "Lucy" were not added
string x;
double y;
mmget x y;
assertx "Ethel"; "Ethel" is greater than exactly items in mm
mmget x y;
assertx "Ricky"; "Ricky" is greater than exactly items in mm
mmget x y;
assertx
Step by Step Solution
There are 3 Steps involved in it
Step: 1
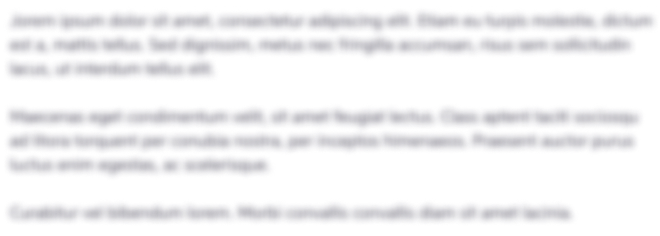
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started