Question
Here is assignment 3 public abstract class Item { private double price; private long UPC; public Item(double price, long uPC) { this.price = price; this.UPC
Here is assignment 3
public abstract class Item { private double price; private long UPC; public Item(double price, long uPC) { this.price = price; this.UPC = uPC; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public long getUPC() { return UPC; } public void setUPC(long uPC) { UPC = uPC; } public String toString() { return " Price=" + price + ", UPC=" + UPC; } }
//Book class
public class Book extends Item { //Declaring instance variables
private String title;
private String ISBN;
// Book constructor
public Book(String title, String iSBN, double price, long UPC) {
super(price, UPC); this.title = title;
this.ISBN = iSBN;
}
//toString method is used to display the contents of an object inside it public String toString() {
return "Book :: Title=" + title + ", ISBN=" + ISBN + super.toString();
}
//Setters and getters public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getISBN() {
return ISBN;
}
public void setISBN(String iSBN) {
ISBN = iSBN;
}
public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((ISBN == null) ? 0 : ISBN.hashCode()); result = prime * result + ((title == null) ? 0 : title.hashCode()); return result; }
public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (!(obj instanceof Book)) return false; Book other = (Book) obj; if (ISBN == null) { if (other.ISBN != null) return false; } else if (!ISBN.equals(other.ISBN)) return false; if (title == null) { if (other.title != null) return false; } else if (!title.equals(other.title)) return false; return true; }
}
// Pen.java
public class Pen extends Item { private String color;
public Pen(double price, long uPC, String color) { super(price, uPC); this.color = color; }
public String getColor() { return color; }
public void setColor(String color) { this.color = color; }
public String toString() { return "Pen :: Color=" + color + super.toString(); }
public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((color == null) ? 0 : color.hashCode()); return result; }
public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (!(obj instanceof Pen)) return false; Pen other = (Pen) obj; if (color == null) { if (other.color != null) return false; } else if (!color.equals(other.color)) return false; return true; }
}
// Test.java
public class Test {
public static void main(String[] args) { //Creating instance of Book class Book b1=new Book("Java Programming","123-345-4567",45.50,123456789); Book b2=new Book("Java Programming","123-345-4567",45.50,123456789); //Displaying the Book class info System.out.println(b1); // Comparing the two Book class objects if(b1.equals(b2)) { System.out.println("Book#1 and Book#2 are same"); } else { System.out.println("Book#1 and Book#2 are not same"); } //Creating Instances of Pen class Pen p1=new Pen(3.45,234567888,"Red"); Pen p2=new Pen(2.45,234567888,"Blue");
//Displaying the Pen class info System.out.println(p1); System.out.println(p2); // Comparing the two Pen class objects if(p1.equals(p2)) { System.out.println("Pen#1 and Pen#2 are same"); } else { System.out.println("Pen#1 and Pen#2 are not same"); } }
}
The purpose of this assignment is to practice using polymorphism, interfaces and the compare To method. Problem- Building on the class hierarchy from assignment 3: 1. Create an interface called Discount, which has 2 methods: + a. to calculate the sales discount for items in the store, and b. to set the discount amount for an item. + c. Note the discount will not adjust the price variable of an item; it will reduce the price by the discount amount when the toString method is called (so the toString code must be changed). d. Note getPricel) will return the non discounted price. 2. In the driver program, create an array of Items, containing at least 2 items of each type (Book, Textbook, Novel, and Pen). Using polymorphism display the details of each Item in the array. 3. Use a cast to access a Textbook and Pen in the array to do sets and gets for course and color. Show that the code works by producing suitable outputs. 4. Finally have the item class implement ComparableStep by Step Solution
There are 3 Steps involved in it
Step: 1
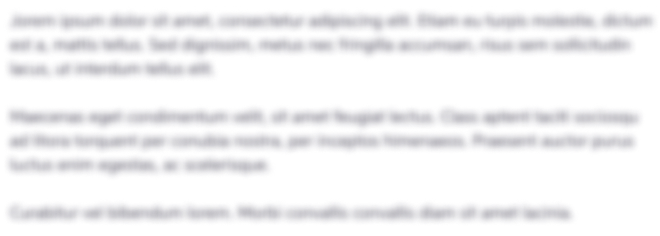
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started