Question
Here is my code: // Define arrays const rowWin = [ [O, O, O], [, , ], [, , ] ] const colWin = [
Here is my code:
// Define arrays const rowWin = [ ["O", "O", "O"], ["", "", ""], ["", "", ""] ]
const colWin = [ ["", "X", ""], ["", "X", ""], ["", "X", ""] ]
const diagonalWin = [ ["", "", "O"], ["", "O", ""], ["O", "", ""] ]
const diagonalWinInverse = [ ["X", "", ""], ["", "X", ""], ["", "", "X"] ]
// evaluatePlay function. // Within this function, write the code to evaluate weather a winning play has been made or not // Your program must be able to evaluate all the grids defined above. // The function should inform the user of which sign has won and which sign has lost // You may add additional parameters to assist you // define evaluatePlay function that takes a 2D array as an argument. function evaluatePlay(grid) { // Create a variable to hold the winner. let winner = ''; // Iterate through each row in the grid to check for a full row of matching characters. for (let i = 0; i < grid.length; i++) { if (grid[i][0] === grid[i][1] && grid[i][1] === grid[i][2]) { if (grid[i][0] !== '-') { winner = grid[i][0]; } } } // Iterate through each column in the grid to check for a full column of matching characters. for (let i = 0; i < grid.length; i++) { if (grid[0][i] === grid[1][i] && grid[1][i] === grid[2][i]) { if (grid[0][i] !== '-') { winner = grid[0][i]; } } } // Iterate through the diagonals in the grid to check for a full diagonal of matching characters. if (grid[0][0] === grid[1][1] && grid[1][1] === grid[2][2]) { if (grid[0][0] !== '-') { winner = grid[0][0]; } }
if (grid[0][2] === grid[1][1] && grid[1][1] === grid[2][0]) { if (grid[0][2] !== '-') { winner = grid[0][2]; } }
// Log the results to the console. if (winner === 'X') { console.log('X Won'); console.log('O Lost'); } else if (winner === 'O') { console.log('O Won'); console.log('X Lost'); }
} // Call the evaluatePlay function and pass the grids to be evaluated. evaluatePlay(rowWin); evaluatePlay(colWin); evaluatePlay(diagonalWin); evaluatePlay(diagonalWinInverse);
Lecturers Review: So there is no result for colWin and rowWin. This is because your for loops are a bit wonky, please have a look at where they start and end. You only need one for loop to iterate through your 2D array once. Once you fix this your program will run perfectly.
Please help fix this issue according to lecturers comment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
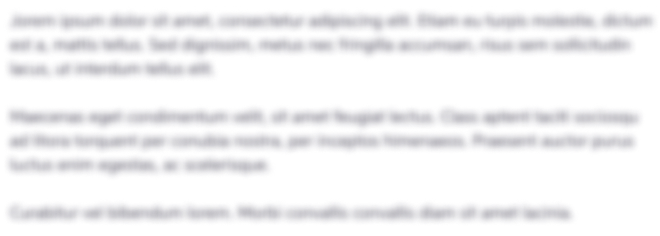
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started