Question
Here is some code that you can use as a template: import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp extends Application {
Here is some code that you can use as a template:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp extends Application { public void start(Stage primaryStage) { Pane aPane = new Pane(); // Create the labels // ... ADD CODE HERE ... // // Create the lists String[] titles = {"Star Wars", "Java is cool", "Mary Poppins", "The Green Mile"}; String[] years = {"1978", "2002", "1968", "1999"}; String[] lengths = {"124", "93", "126", "148"}; // ... ADD CODE HERE ... // // Create the buttons. The following code shows how to set the font, // background color and text color of a button: // b.setStyle("-fx-font: 12 arial; -fx-base: rgb(0,100,0); " + // "-fx-text-fill: rgb(255,255,255);"); // ... ADD CODE HERE ... // // Dont forget to add the components to the window, set the title, // make it non-resizable, set Scene dimensions and then show the stage // ... ADD CODE HERE ... // } public static void main(String[] args) { launch(args); } }
Now we will also use the newly created Pane in another interface. Create a class callled DVDCollectionApp2 to represent the GUI shown on the next page, which is similar, but has only one ListView (which is a list of DVDs now, instead of Strings) and contains three TextField objects. Here is some code that you can use to get you started:
import javafx.application.Application; import javafx.collections.FXCollections; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.control.TextField; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp2 extends Application { public void start(Stage primaryStage) { Pane aPane = new Pane(); // Create the labels Label label1 = new Label("DVDs"); Label label2 = new Label("Title"); Label label3 = new Label("Year"); Label label4 = new Label("Length"); // Create the TextFields TextField tField = new TextField(); TextField yField = new TextField(); TextField lField = new TextField(); // Create the lists ListView tList = new ListView(); // Create the buttons DVDButtonPane buttonPane = new DVDButtonPane(); // Add all the components to the window aPane.getChildren().addAll(label1, label2, label3, label4, tField, yField, lField, tList, buttonPane); primaryStage.setTitle("My DVD Collection"); primaryStage.setResizable(false); primaryStage.setScene(new Scene(aPane, 548,268)); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
We will now make adjustments to the code to populate the interface with a DVDCollection object as its model. In the DVDCollectionApp2 class, add an attribute to store the model as follows:
private DVDCollection model;
Then create the following constructor, which will set that attribute to be the example DVDCollection that was defined in that model class:
public DVDCollectionApp2() { model = DVDCollection.example1(); }
Recall that we used the setItems() method to add some items to a ListView:
ListView yList = new ListView(); String[] years = {"1978", "2002", "1968", "1999"}; yList.setItems(FXCollections.observableArrayList(years));
Modify your code so that the tList ListView contains the list of DVD objects that are contained in the DVDCollection model. Here is what you should see:
public class DVD implements Comparable { private String title; private int year; private int duration; public DVD () { this ("",0,0); } public DVD (String newTitle, int y, int minutes) { title = newTitle; year = y; duration = minutes; } public int compareTo(Object obj) { if (obj instanceof DVD) { DVD aDVD = (DVD)obj; return title.compareTo(aDVD.title); } return 0; } public String getTitle() { return title; } public int getDuration() { return duration; } public int getYear() { return year; } public void setTitle(String t) { title = t; } public void setDuration(int d) { duration = d; } public void setYear(int y) { year = y; } public String toString() { return ("DVD (" + year + "): \"" + title + "\" with length: " + duration + " minutes"); } public static void main(String args[]) { System.out.println(DVD.parseFrom("Rush Hour 2,2001,118")); } }
public class DVDCollection { public static final int MAX_DVDS = 100; private DVD[] dvds; private int dvdCount; public DVDCollection() { dvds = new DVD[MAX_DVDS]; } public DVD[] getDvds() { return dvds; } public int getDvdCount() { return dvdCount; } public String toString() { return ("DVD Collection of size " + dvdCount); } public void add(DVD aDvd) { if (dvdCount MAX_DVDS) dvds[dvdCount++] = aDvd; } public boolean remove(String title) { for (int i=0; idvdCount; i++) { DVD d = dvds[i]; if (dvds[i].getTitle().equals(title)) { dvds[i] = dvds[dvdCount-1]; dvdCount--; return true; } } return false; } public static DVDCollection example1() { DVDCollection c = new DVDCollection(); c.add(new DVD("If I Was a Student", 2007, 65)); c.add(new DVD("Don't Eat Your Pencil !", 1984, 112)); c.add(new DVD("The Exam", 2001, 180)); c.add(new DVD("Tutorial Thoughts", 2003, 128)); c.add(new DVD("Fried Monitors", 1999, 94)); return c; } }
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp extends Application { public void start(Stage primaryStage) { Pane aPane = new Pane(); // Create the labels // ... ADD CODE HERE ... // // Create the lists String[] titles = {"Star Wars", "Java is cool", "Mary Poppins", "The Green Mile"}; String[] years = {"1978", "2002", "1968", "1999"}; String[] lengths = {"124", "93", "126", "148"}; // ... ADD CODE HERE ... // // Create the buttons // The following code shows how to set the font, // background color and text color of a button: // b.setStyle("-fx-font: 12 arial; -fx-base: rgb(0,100,0); " + // "-fx-text-fill: rgb(255,255,255);"); // ... ADD CODE HERE ... // // Dont forget to add the components to the window, set the title, // make it non-resizable, set Scene dimensions and then show the stage // ... ADD CODE HERE ... // } public static void main(String[] args) { launch(args); } }
import javafx.scene.layout.Pane; public class DVDButtonPane extends Pane { // Replace this with your own code }
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.control.TextField; import javafx.scene.layout.Pane; import javafx.stage.Stage; public class DVDCollectionApp2 extends Application { public void start(Stage primaryStage) { Pane aPane = new Pane(); // Create the labels Label label1 = new Label("DVDs"); Label label2 = new Label("Title"); Label label3 = new Label("Year"); Label label4 = new Label("Length"); // Create the TextFields TextField tField = new TextField(); TextField yField = new TextField(); TextField lField = new TextField(); // Create the lists ListView tList = new ListView(); // Create the buttons DVDButtonPane buttonPane = new DVDButtonPane(); // Add all the components to the window aPane.getChildren().addAll(label1, label2, label3, label4, tField, yField, lField, tList, buttonPane); primaryStage.setTitle("My DVD Collection"); primaryStage.setResizable(false); primaryStage.setScene(new Scene(aPane, 548,268)); primaryStage.show(); } public static void main(String[] args) { launch(args); } }Create a class called DVDCollectionApp which will represent the graphical user interface (i.e., GUI) for a DVDCollection object. The GUI will have the following components laid out manually (i.e., a Layout should NOT be used): three Label objects, 3 ListView objects and 3 Button objects as shown below. In this example, the ListView components have widths of 200, 60 and 60, respectively and they all have a height of 150. The Add, Delete and Stats buttons have widths of 95, 95 and 60, respectively and all three have a height of 30. The buttons use 12pt Arial font. The margin around the window and between components is 10. The window is non-resizable and the overall window dimensions are 249 x 228 My DVD Collection Title Length 124 93 126 148 Year Star Wars Java is cool Mary Poppins The Green Mile 1978 2002 1968 1999 Add Delete Stats
Step by Step Solution
There are 3 Steps involved in it
Step: 1
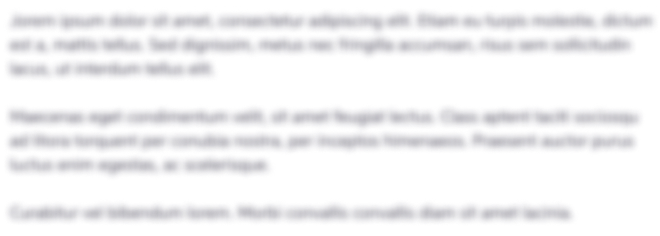
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started