Question
Here is what the output should be Welcome to Battleship! Enter the board height (1 to 99): 8 Enter the board width (1 to 675):
Here is what the output should be
Welcome to Battleship! Enter the board height (1 to 99): 8 Enter the board width (1 to 675): 5 Enter the number of ships (1 to 9): 3 My Ships: A B C D E 0 ~ ~ ~ ~ ~ 1 ~ ~ ~ ~ ~ 2 ~ ~ ~ ~ ~ 3 ~ ~ ~ ~ ~ 4 ~ ~ ~ ~ ~ 5 ~ ~ ~ ~ ~ 6 ~ ~ ~ ~ ~ 7 ~ ~ ~ ~ ~ Vertical or horizontal? (v/h): v Enter the ship length (1 to 8): 3 Enter the x-coord (A to E): C Enter the y-coord (0 to 5): 3 My Ships: A B C D E 0 ~ ~ ~ ~ ~ 1 ~ ~ ~ ~ ~ 2 ~ ~ ~ ~ ~ 3 ~ ~ 1 ~ ~ 4 ~ ~ 1 ~ ~ 5 ~ ~ 1 ~ ~ 6 ~ ~ ~ ~ ~ 7 ~ ~ ~ ~ ~ Vertical or horizontal? (v/h): H Enter the ship length (1 to 5): 4 Enter the x-coord (A to B): A Enter the y-coord (0 to 7): 0 My Ships: A B C D E 0 2 2 2 2 ~ 1 ~ ~ ~ ~ ~ 2 ~ ~ ~ ~ ~ 3 ~ ~ 1 ~ ~ 4 ~ ~ 1 ~ ~ 5 ~ ~ 1 ~ ~ 6 ~ ~ ~ ~ ~ 7 ~ ~ ~ ~ ~ Vertical or horizontal? (v/h): v Enter the ship length (1 to 8): 5 Enter the x-coord (A to E): e Enter the y-coord (0 to 3): 2 Would you like to play again? (y): y Enter the board height (1 to 99): 2 Enter the board width (1 to 675): 1 Enter the number of ships (1 to 9): 1 My Ships: A 0 ~ 1 ~ Vertical or horizontal? (v/h): h Enter the ship length (1 to 1): 1 Enter the x-coord (A to A): a Enter the y-coord (0 to 1): 1 Would you like to play again? (y): w Thanks for playing!
Here is the java code we are working on, But we are completely stucked. Please help. Change anything where appropriate. Ignore comments if it does not make sense.
import java.util.Scanner; import java.util.Random;
public class Battleship {
/** * This method converts a String representing a base (or radix) 26 number into a decimal (or * base 10) number. The String representation of the base 26 number uses the letters of the * Latin alphabet to represent the 26 digits. That is, A represents 0, B represents 1, C * represents 2, ..., Y represents 24, and Z represents 25. * * A couple of examples: BAAA = 1 * 26^3 + 0 * 26^2 + 0 * 26^1 + 0 * 26^0 = 17576 ZERTY = 25 * * 26^4 + 4 * 26^3 + 17 * 26^2 + 19 * 26^1 + 24 * 26^0 = 11506714 * * For this method: - use Math.pow to calculate the powers of 26. - don't assume that the input * is in any particular case; use toUpperCase(). - don't check that the input is only 'A' to * 'Z'. - calculate the value of each digit relative to 'A'. - start from either the first or * last character, and calculate the exponent based on the index of each character. * * @param coord The coordinate value in base 26 as described above. * @return The numeric representation of the coordinate. */ public static int coordAlphaToNum(String coord) { // FIXME Scanner scnr = new Scanner(System.in); String[] Alpha = new String[] {"A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"};
int i; int j; int k; int intCoord = 0; String stringCoord; char charCoord; int coordAlpha = 0;
for (i = 0; (i
for (j = 0; (j
}
coordAlpha = (int) (coordAlpha + Math.pow(26, i) * intCoord); }
return coordAlpha; }
/** * This method converts an int value into a base (or radix) 26 number, where the digits are * represented by the 26 letters of the Latin alphabet. That is, A represents 0, B represents 1, * C represents 2, ..., Y represents 24, and Z represents 25. A couple of examples: 17576 is * BAAA, 11506714 is ZERTY. * * The algorithm to convert an int to a String representing these base 26 numbers is as follows: * - Initialize res to the input integer - The next digit is determined by calculating the * remainder of res with respect to 26 - Convert this next digit to a letter based on 'A' - Set * res to the integer division of res and 26 - Repeat until res is 0 * * @param coord The integer value to covert into an alpha coordinate. * @return The alpha coordinate in base 26 as described above. If coord is negative, an empty * string is returned. */ public static String coordNumToAlpha(int coord) { int valName = coord; int min = 0; int max = 99999999; int i; int j; String result = "";
String[] Alpha = new String[] {"A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"}; String representAlpha = ""; Scanner sc = new Scanner(System.in);
while (valName max) { System.out.println("Invalid value."); valName = sc.nextInt();
} for (i = 0; valName > 0; ++i) { j = valName % 26; valName = (valName - j) / 26; representAlpha = representAlpha + Alpha[j];
} for (int a = representAlpha.length() - 1; a >= 0; a--) { result = result + representAlpha.charAt(a); }
return result; }
/** * Prompts the user for an integer value, displaying the following: "Enter the valName (min to * max): " Note: There should not be a new line terminating the prompt. valName should contain * the contents of the String referenced by the parameter valName. min and max should be the * values passed in the respective parameters. * * After prompting the user, the method will read an int from the console and consume an entire * line of input. If the value read is between min and max (inclusive), that value is returned. * Otherwise, "Invalid value." terminated by a new line is output and the user is prompted * again. * * @param sc The Scanner instance to read from System.in. * @param valName The name of the value for which the user is prompted. * @param min The minimum acceptable int value (inclusive). * @param min The maximum acceptable int value (inclusive). * @return Returns the value read from the user. */ public static int promptInt(Scanner sc, String valName, int min, int max) { int boardHeight = 0; int boardWidth = 0; int shipNum = 0; int shipLength = 0; int ycoordVal = 0;
if (valName == "board height" && (min == 1 && max == 99)) { System.out.println("Enter the board height (1 to 99): "); boardHeight = sc.nextInt();
while (boardHeight max) { System.out.println("Invalid value."); System.out.println("Enter the board height (1 to 99): "); boardHeight = sc.nextInt(); } return boardHeight; } if (valName == "board width" && (min == 1 && max == 675)) { System.out.println("Enter the board width (1 to 675): "); boardWidth = sc.nextInt();
while (boardWidth max) { System.out.println("Invalid value."); System.out.println("Enter the board width (1 to 675): "); boardWidth = sc.nextInt();
}
return boardWidth;
}
if (valName == "ship number" && (min == 1 && max == 9)) { System.out.println("Enter the number of ships (1 to 9):"); shipNum = sc.nextInt();
while (shipNum max) { System.out.println("Invalid value."); System.out.println("Enter the number of ships (1 to 9):"); shipNum = sc.nextInt(); }
return shipNum;
}
if (valName == "ship length") { System.out.println("Enter the ship length (1 to " + max + "):"); shipLength = sc.nextInt();
while (shipLength max) { System.out.println("Invalid value."); System.out.println("Enter the ship length (1 to " + max + "):"); shipLength = sc.nextInt(); }
return shipLength;
}
if (valName == "ycoord") { System.out.println("Enter the y-coord (0 to " + max + "): "); ycoordVal = sc.nextInt();
while (ycoordVal max) { System.out.println("Invalid value."); System.out.println("Enter the y-coord (0 to " + max + "): "); ycoordVal = sc.nextInt(); }
return ycoordVal;
}
return 0; }
/** * Prompts the user for an String value, displaying the following: "Enter the valName (min to * max): " Note: There should not be a new line terminating the prompt. valName should contain * the contents of the String referenced by the parameter valName. min and max should be the * values passed in the respective parameters. * * After prompting the user, the method will read an entire line of input, trimming any trailing * or leading whitespace. If the value read is (lexicographically ignoring case) between min and * max (inclusive), that value is returned. Otherwise, "Invalid value." terminated by a new line * is output and the user is prompted again. * * @param sc The Scanner instance to read from System.in. * @param valName The name of the value for which the user is prompted. * @param min The minimum acceptable String value (inclusive). * @param min The maximum acceptable String value (inclusive). * @return Returns the value read from the user. */ public static String promptStr(Scanner sc, String valName, String min, String max) { String xcoord = ""; int minVal = 0; int maxVal = 0; int xcoordVal = 0; minVal = coordAlphaToNum(min); maxVal = coordAlphaToNum(max);
if (valName.equals("xcoord")) { System.out.println("Enter the x-coord (A to " + max + "): "); xcoord = sc.next(); xcoord = xcoord.toUpperCase(); xcoordVal = coordAlphaToNum(xcoord);
while (xcoordVal maxVal) { System.out.println("Invalid value."); System.out.println("Enter the x-coord (A to " + max + "): "); xcoord = sc.next().trim(); xcoord = xcoord.toUpperCase(); } return xcoord; }
return ""; }
/** * Prompts the user for an char value. The prompt displayed is the contents of the String * referenced by the prompt parameter. Note: There should not be a new line terminating the * prompt. * * After prompting the user, the method will read an entire line of input and return the first * non-whitespace character in lower case. * * @param sc The Scanner instance to read from System.in * @param prompt The user prompt. * @return Returns the first non-whitespace character (in lower case) read from the user. If * there are no non-whitespace characters read, the null character is returned. */ public static char promptChar(Scanner sc, String prompt) { char returnChar = '0'; String input; if (prompt == "Would you like to play again? (y): ") { System.out.print("Would you like to play again? (y): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0);
while (returnChar != 'y' && returnChar != 'n') { System.out.println("Invalid value."); System.out.print("Would you like to play again? (y): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0); }
return returnChar; }
if (prompt == "Vertical or horizontal? (v/h): ") { System.out.print("Vertical or horizontal? (v/h): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0);
while (returnChar != 'v' && returnChar != 'h') { System.out.println("Invalid value."); System.out.print("Vertical or horizontal? (v/h): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0); }
return returnChar; }
if (prompt == "No room for ship. Try again? (y): ") { System.out.print("No room for ship. Try again? (y): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0);
while (returnChar != 'y' && returnChar != 'n') { System.out.println("Invalid value."); System.out.print("No room for ship. Try again? (y): "); input = sc.next().trim(); input = input.toLowerCase(); returnChar = input.charAt(0); }
if (returnChar == 'y') { return 'y'; } else { return 'n'; }
} return returnChar; }
/** * Initializes a game board so that all the entries are Config.WATER_CHAR. * * @param board The game board to initialize. */ public static void initBoard(char board[][]) { Scanner sc = new Scanner(System.in); int numShip; for (int i = 0; i
}
}
/** * Prints the game boards as viewed by the user. This method is used to print the game boards as * the user is placing their ships and during the game play. * * Some notes on the display: - Each column printed will have a width of Config.MAX_COL_WIDTH. - * Each row is followed by an empty line. - The values in the headers and cells are to be right * justified. * * @param board The board to print. * @param caption The board caption. */ public static void printBoard(char board[][], String caption) { System.out.println(caption);
for (int i = 0; i
for (int j = 0; j
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
/** * Determines if a sequence of cells of length len in a game board is clear or not. This is used * to determine if a ship will fit on a given game board. The x and y coordinates passed in as * parameters represent the top-left cell of the ship when considering the grid. * * @param board The game board to search. * @param xcoord The x-coordinate of the top-left cell of the ship. * @param ycoord The y-coordinate of the top-left cell of the ship. * @param len The length of the ship. * @param dir true if the ship will be vertical, otherwise horizontal * @return 1 if the cells to be occupied by the ship are all Config.WATER_CHAR, -1 if the cells * to be occupied are not Config.WATER_CHAR, and -2 if the ship would go out-of-bounds * of the board. */ public static int checkWater(char board[][], int xcoord, int ycoord, int len, boolean dir) { int status = -2; int count1 = 0, count2 = 0; //if coord+dir is out of bound, return -2 if (dir) {
if((ycoord+len)> board.length) { return -2; }
for (int i = 0; i
if (board[ycoord + i][xcoord] == '~') { // board[ycoord+i][xcoord] = id count1++; }
else {
count2++; }
}
if (count1 >= len) {
status = 1;
} else if (count2 >= len) {
status = -1;
}
else { if((xcoord+len)> board[0].length) { return -2; }
for (int i = 0; i
if (board[ycoord][xcoord + i] == '~') { count1++; }
else {
count2++; }
if (count1 == board[i].length && count1 >= len) {
status = 1;
}
else if (count2 == board[i].length && count2 >= len) {
status = -1;
}
}
} }
return status; }
/** * Checks the cells of the game board to determine if all the ships have been sunk. * * @param board The game board to check. * @return true if all the ships have been sunk, false otherwise. */ public static boolean checkLost(char board[][]) { // FIXME return false; }
/** * Places a ship into a game board. The coordinate passed in the parameters xcoord and ycoord * represent the top-left coordinate of the ship. The ship is represented on the game board by * the Character representation of the ship id. (For this method, you can assume that the id * parameter will only be values 1 through 9.) * * @param board The game board to search. * @param xcoord The x-coordinate of the top-left cell of the ship. * @param ycoord The y-coordinate of the top-left cell of the ship. * @param len The length of the ship. * @param dir true if the ship will be vertical, otherwise horizontal. * @param id The ship id, assumed to be 1 to 9. * @return false if the ship goes out-of-bounds of the board, true otherwise. */ public static boolean placeShip(char board[][], int xcoord, int ycoord, int len, boolean dir, int id) { int i;
if (dir) { if((ycoord+len)> board.length) { return false; } for (i = 0; i } if (dir == false) { if((xcoord+len)> board.length) { return false; } for (i = 0; i // addShip(boardPrime, boardOpp, i + 1, rand); ////fixme // else { // return false; return false; } /** * Randomly attempts to place a ship into a game board. The random process is as follows: 1 - * Pick a random boolean, using rand. True represents vertical, false horizontal. 2 - Pick a * random integer, using rand, for the x-coordinate of the top-left cell of the ship. The number * of integers to choose from should be calculated based on the width of the board and length of * the ship such that the placement of the ship won't be out-of-bounds. 3 - Pick a random * integer, using rand, for the y-coordinate of the top-left cell of the ship. The number of * integers to choose from should be calculated based on the height of the board and length of * the ship such that the placement of the ship won't be out-of-bounds. 4 - Verify that this * random location can fit the ship without intersecting another ship (checkWater method). If * so, place the ship with the placeShip method. * * It is possible for the configuration of a board to be such that a ship of a given length may * not fit. So, the random process will be attempted at most Config.RAND_SHIP_TRIES times. * * @param board The game board to search. * @param len The length of the ship. * @param id The ship id, assumed to be 1 to 9.. * @param rand The Random object. * @return true if the ship is placed successfully, false otherwise. */ public static boolean placeRandomShip(char board[][], int len, int id, Random rand) { // FIXME return false; } /** * This method interacts with the user to place a ship on the game board of the human player and * the computer opponent. The process is as follows: 1 - Print the user primary board, using the * printBoard. 2 - Using the promptChar method, prompt the user with "Vertical or horizontal? * (v/h) ". A response of v is interpreted as vertical. Anything else is assumed to be * horizontal. 3 - Using the promptInt method, prompt the user for an integer representing the * "ship length", where the minimum ship length is Config.MIN_SHIP_LEN and the maximum ship * length is width or height of the game board, depending on the input of the user from step 1. * 4 - Using the promptStr method, prompt the user for the "x-coord". The maximum value should * be calculated based on the width of the board and the length of the ship. You will need to * use the coordAlphaToNum and coordNumToAlpha methods to covert between int and String values * of coordinates. 5 - Using the promptInt method, prompt the user for the "y-coord". The * maximum value should be calculated based on the width of the board and the length of the * ship. 6 - Check if there is space on the board to place the ship. 6a - If so: - Place the * ship on the board using placeShip. - Then, call placeRandomShip to place the opponents ships * of the same length. - If placeRandomShip fails, print out the error message (terminated by a * new line): "Unable to place opponent ship: id", where id is the ship id, and return false. 6b * - If not: - Using promptChar, prompt the user with "No room for ship. Try again? (y): " - * If the user enters a 'y', restart the process at Step 1. - Otherwise, return false. * * @param sc The Scanner instance to read from System.in. * @param boardPrime The human player board. * @param boardOpp The opponent board. * @param id The ship id, assumed to be 1 to 9. * @param rand The Random object. * @return true if ship placed successfully by player and computer opponent, false otherwise. */ public static boolean addShip(Scanner sc, char boardPrime[][], char boardOpp[][], int id, Random rand) { int i = 0; printBoard(boardPrime, "My ships: "); String xcoord = ""; int ycoord = 0; int ycoordRand = 0; int xcoordValRand = 0; int xcoordVal = 0; int shipLength = 0; int shipLengthRand = 0; String min = ""; String max = ""; char dirVal = '0'; boolean dir = true; boolean dirRand = true; int pass = 0; char checkVal; // char y = 'a'; dirVal = promptChar(sc, "Vertical or horizontal? (v/h): "); if (dirVal == 'v') { dir = true; shipLength = promptInt(sc, "ship length", 1, boardPrime.length); min = coordNumToAlpha(0).toUpperCase(); // FIXME max = coordNumToAlpha(boardPrime.length - 1).toUpperCase(); xcoord = promptStr(sc, "xcoord", min, max); xcoordVal = coordAlphaToNum(xcoord); ycoord = promptInt(sc, "ycoord", 0, boardPrime.length - shipLength); } else if (dirVal == 'h') { dir = false; shipLength = promptInt(sc, "ship length", 1, boardPrime[0].length); min = coordNumToAlpha(0).toUpperCase(); // FIXME max = coordNumToAlpha(boardPrime[0].length - shipLength); max = max.toUpperCase(); xcoord = promptStr(sc, "xcoord", min, max); xcoordVal = coordAlphaToNum(xcoord); ycoord = promptInt(sc, "ycoord", 1, boardPrime.length - 1); } if ((checkWater(boardPrime, xcoordVal, ycoord, shipLength, dir) != -2) || (checkWater(boardPrime, xcoordVal, ycoord, shipLength, dir) != -1)) { placeShip(boardPrime, xcoordVal, ycoord, shipLength, dir, id); } /* * else { int y = promptChar (sc, "No room for ship. Try again? (y): "); if (y == 'y') { * addShip(sc, boardPrime, boardOpp, id, rand) ; } * * else { return false; //* } */ dirRand = rand.nextBoolean(); // sambung balik macam placeShip normal.tapi guna random variable if (dirRand) { shipLengthRand = rand.nextInt(0) + (boardPrime.length + 1); min = coordNumToAlpha(0).toUpperCase(); // FIXME max = coordNumToAlpha(boardPrime.length - 1).toUpperCase(); xcoord = promptStr(sc, "xcoord", min, max); xcoordVal = coordAlphaToNum(xcoord); ycoord = promptInt(sc, "ycoord", 0, boardPrime.length - shipLength); } else if (dirRand == false) { shipLengthRand = rand.nextInt(0) + (boardPrime.length + 1); min = coordNumToAlpha(0).toUpperCase(); // FIXME max = coordNumToAlpha(boardPrime[0].length - shipLength); max = max.toUpperCase(); xcoord = promptStr(sc, "xcoord", min, max); xcoordValRand = coordAlphaToNum(xcoord); ycoordRand = promptInt(sc, "ycoord", 1, boardPrime.length - 1); } if ((checkWater(boardOpp, xcoordValRand, ycoordRand, shipLengthRand, dir) != -2) || (checkWater(boardOpp, xcoordValRand, ycoordRand, shipLengthRand, dir) != -1)) { placeRandomShip(boardOpp, shipLengthRand, id, rand); } return false; } /** * Checks the state of a targeted cell on the game board. This method does not change the * contents of the game board. * * @return 3 if the cell was previously targeted. 2 if the shot would be a miss. 1 if the shot * would be a hit. -1 if the shot is out-of-bounds. */ public static int takeShot(char[][] board, int x, int y) { // FIXME return 0; } /** * Interacts with the user to take a shot. The procedure is as follows: 1 - Using the promptStr * method, prompt the user for the "x-coord shot". The maximum value should be based on the * width of the board. You will need to use the coordAlphaToNum and coordNumToAlpha methods to * covert between int and String values of coordinates. 2 - Using the promptInt method, prompt * the user for the "y-coord shot". The maximum value should be calculated based on the width of * the board. 3 - Check the shot, using the takeShot method. If it returns: -1: Print out an * error message "Coordinates out-of-bounds!", terminated by a new line. 3: Print out an error * message "Shot location previously targeted!", terminated by a new line. 1 or 2: Update the * cells in board and boardTrack with Config.HIT_CHAR or Config.MISS_CHAR accordingly. This * process should repeat until the takeShot method returns 1 or 2. * * @param sc The Scanner instance to read from System.in. * @param board The computer opponent board (containing the ship placements). * @param boardTrack The human player tracking board. */ public static void shootPlayer(Scanner sc, char[][] board, char[][] boardTrack) { // FIXME } /** * Takes a random shot on the game board. The random process works as follows: 1 - Pick a random * valid x-coordinate 2 - Pick a random valid y-coordinate 3 - Check the shot, using the * takeShot method. This process should repeat until the takeShot method returns 1 or 2, then * update the cells in board with Config.HIT_CHAR or Config.MISS_CHAR accordingly. * * Note: Unlike the placeRandomShip method, this method continues until it is successful. This * may seem risky, but in this case the random process will terminate (find an untargeted cell) * fairly quickly. For more details, see the appendix of the Big Program 1 subject. * * @param rand The Random object. * @param board The human player game board. */ public static void shootComputer(Random rand, char[][] board) { // FIXME } /** * This is the main method for the Battleship game. It consists of the main game and play again * loops with calls to the various supporting methods. When the program launches (prior to the * play again loop), a message of "Welcome to Battleship!", terminated by a newline, is * displayed. After the play again loop terminiates, a message of "Thanks for playing!", * terminated by a newline, is displayed. * * The Scanner object to read from System.in and the Random object with a seed of Config.SEED * will be created in the main method and used as arguments for the supporting methods as * required. * * Also, the main method will require 3 game boards to track the play: - One for tracking the * ship placement of the user and the shots of the computer, called the primary board with a * caption of "My Ship". - One for displaying the shots (hits and misses) taken by the user, * called the tracking board with a caption of "My Shots"; and one for tracking the ship * placement of the computer and the shots of the user. - The last board is never displayed, but * is the primary board for the computer and is used to determine when a hit or a miss occurs * and when all the ships of the computer have been sunk. Notes: - The size of the game boards * are determined by the user input. - The game boards are 2d arrays that are to be viewed as * row-major order. This means that the first dimension represents the y-coordinate of the game * board (the rows) and the second dimension represents the x-coordinate (the columns). * * @param args Unused. */ public static void main(String[] args) { Scanner sc = new Scanner(System.in); Random rand = new Random(); int i = 0; System.out.println("Welcome to Battleship!"); char option = 'y'; while (option != 'n') { int boardHeight = promptInt(sc, "board height", 1, 99); int boardWidth = promptInt(sc, "board width", 1, 675); System.out.println(""); int shipNum = promptInt(sc, "ship number", 1, 9); char[][] board = new char[boardHeight][boardWidth]; char[][] boardOpp = new char[boardHeight][boardWidth]; initBoard(board); addShip(sc, board, boardOpp, i, rand); option = promptChar(sc, "Would you like to play again? (y): "); } System.out.println("Thanks for playing!"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
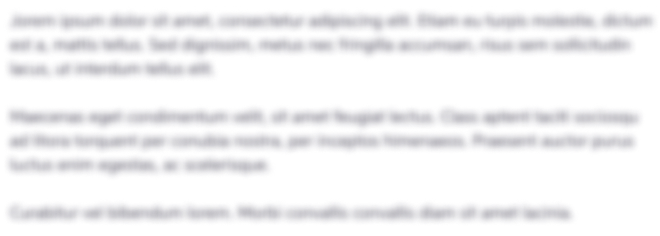
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started