Question
Here's the link to my assigment: https://www.dropbox.com/s/mawyte4ivhadipw/CSC260_P5-Palindrone_StacksQueues.pdf?dl=0 Queue.h #ifndef QUEUE_H #define QUEUE_H #include using namespace std; // Stack template template class Queue { private: T
Here's the link to my assigment:
https://www.dropbox.com/s/mawyte4ivhadipw/CSC260_P5-Palindrone_StacksQueues.pdf?dl=0
Queue.h
#ifndef QUEUE_H
#define QUEUE_H
#include
using namespace std;
// Stack template
template
class Queue
{
private:
T *queueArray; // Points to the queue array
int queueSize; // The queue size
int front; // Subscript of the queue front
int rear; // Subscript of the queue rear
int numItems; // Number of items in the queue
public:
// Constructor
Queue(int);
// Copy constructor
Queue(const Queue &);
// Destructor
~Queue();
// Queue operations
void enqueue(T);
void dequeue(T &);
bool isEmpty() const;
bool isFull() const;
void clear();
};
//***************************************************************
// This constructor creates an empty queue of a specified size. *
//***************************************************************
template
Queue
{
queueArray = new T[s];
queueSize = s;
front = -1;
rear = -1;
numItems = 0;
}
//***************************************************************
// Copy constructor *
//***************************************************************
template
Queue
{
// Allocate the queue array.
queueArray = new T[obj.queueSize];
// Copy the other object's attributes.
queueSize = obj.queueSize;
front = obj.front;
rear = obj.rear;
numItems = obj.numItems;
// Copy the other object's queue array.
for (int count = 0; count < obj.queueSize; count++)
queueArray[count] = obj.queueArray[count];
}
//***************************************************************
// Destructor *
//***************************************************************
template
Queue
{
delete [] queueArray;
}
//***************************************************************
// Function enqueue inserts a value at the rear of the queue. *
//***************************************************************
template
void Queue
{
if (isFull())
cout << "The queue is full. ";
else
{
// Calculate the new rear position
rear = (rear + 1) % queueSize;
// Insert new item
queueArray[rear] = item;
// Update item count
numItems++;
}
}
//***************************************************************
// Function dequeue removes the value at the front of the queue *
// and copies t into num. *
//***************************************************************
template
void Queue
{
if (isEmpty())
cout << "The queue is empty. ";
else
{
// Move front
front = (front + 1) % queueSize;
// Retrieve the front item
item = queueArray[front];
// Update item count
numItems--;
}
}
//***************************************************************
// isEmpty returns true if the queue is empty, otherwise false. *
//***************************************************************
template
bool Queue
{
bool status;
if (numItems)
status = false;
else
status = true;
return status;
}
//***************************************************************
// isFull returns true if the queue is full, otherwise false. *
//***************************************************************
template
bool Queue
{
bool status;
if (numItems < queueSize)
status = false;
else
status = true;
return status;
}
//*****************************************************************
// clear sets the front and rear indices, and sets numItems to 0. *
//*****************************************************************
template
void Queue
{
front = queueSize - 1;
rear = queueSize - 1;
numItems = 0;
}
#endif
Stack.h
#ifndef STACK_H
#define STACK_H
#include
using namespace std;
// Stack template
template
class Stack
{
private:
T *stackArray;
int stackSize;
int top;
public:
//Constructor
Stack(int);
// Copy constructor
Stack(const Stack&);
// Destructor
~Stack();
// Stack operations
void push(T);
void pop(T &);
bool isFull();
bool isEmpty();
};
//***************************************************
// Constructor *
//***************************************************
template
Stack
{
stackArray = new T[size];
stackSize = size;
top = -1;
}
//***************************************************
// Copy constructor *
//***************************************************
template
Stack
{
// Create the stack array.
if (obj.stackSize > 0)
stackArray = new T[obj.stackSize];
else
stackArray = NULL;
// Copy the stackSize attribute.
stackSize = obj.stackSize;
// Copy the stack contents.
for (int count = 0; count < stackSize; count++)
stackArray[count] = obj.stackArray[count];
// Set the top of the stack.
top = obj.top;
}
//***************************************************
// Destructor *
//***************************************************
template
Stack
{
if (stackSize > 0)
delete [] stackArray;
}
//*************************************************************
// Member function push pushes the argument onto *
// the stack. *
//*************************************************************
template
void Stack
{
if (isFull())
{
cout << "The stack is full. ";
}
else
{
top++;
stackArray[top] = item;
}
}
//*************************************************************
// Member function pop pops the value at the top *
// of the stack off, and copies it into the variable *
// passed as an argument. *
//*************************************************************
template
void Stack
{
if (isEmpty())
{
cout << "The stack is empty. ";
}
else
{
item = stackArray[top];
top--;
}
}
//*************************************************************
// Member function isFull returns true if the stack *
// is full, or false otherwise. *
//*************************************************************
template
bool Stack
{
bool status;
if (top == stackSize - 1)
status = true;
else
status = false;
return status;
}
//*************************************************************
// Member function isEmpty returns true if the stack *
// is empty, or false otherwise. *
//*************************************************************
template
bool Stack
{
bool status;
if (top == -1)
status = true;
else
status = false;
return status;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
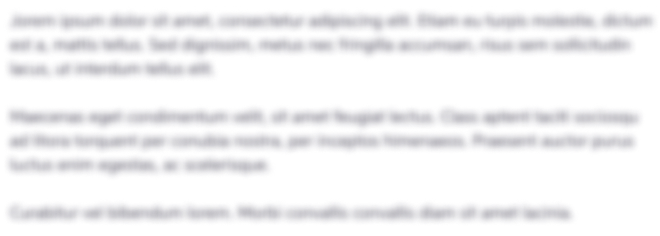
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started