Question
Hey, Good Afternoon. Whenever I run my program I get an error saying int cannot be converted to a string . I have 2 files.
Hey, Good Afternoon. Whenever I run my program I get an error saying int cannot be converted to a string
. I have 2 files. We use jGrasp and both files go hand in hand.
/** * Program that prints. This program prints. * * Project 1. * @author david estrada - comp 1210 - section 008. * @version 01/14/2020. */ public class UserInfo { private String firstName = ""; private String lastName = ""; private String location = ""; private int age; private int status; private static final int OFFLINE = 0, ONLINE = 1; //constructor /** *@param firstNameIn of the user. *@param lastNameIn of the user. .*/ public UserInfo(String firstNameIn, String lastNameIn) { firstName = firstNameIn; lastName = lastNameIn; location = "Not specified"; age = 0; status = OFFLINE; } //methods /**Javadoc comment. *@return output of the user. */ public String toString() { String output = "Name: " + firstName + " " + lastName + " "; output += "Location: " + location + " "; output += "Age: " + age + " "; output += "Status: "; if (status == OFFLINE) { output += "Offline"; } else { output += "Online"; } return output; } /**Javadoc comment. *@param locationIn of the user. */ public void setLocation(String locationIn) { location = locationIn; } /**Javadoc comment. *@param ageIn explains if the Age is set. *@return isSet explains if the Age is set. */ public boolean setAge(int ageIn) { boolean isSet = false; if (ageIn > 0) { age = ageIn; isSet = true; } /**Javadoc comment.*/ return isSet; } /**Javadoc comment. *@return getAge */ public int getAge() { return age; } /**Javadoc comment. *@return getLocation */ public int getLocation() { return status; } /**Javadoc comment. * */ public void logOff() { status = OFFLINE; } /**Javadoc comment. *logOn */ public void logOn() { status = ONLINE; } }
2nd file
/** * Program prints user info. User inputs info. * * Assignment 4. * @author david estrada - comp 1210 - section 008. * @version 02/03/2020. */ public class UserInfoDriver { /** * Introduces the Driver. * @param args this program introduces Driver. */ public static void main(String[] args) { UserInfo user1 = new UserInfo("Pat", "Doe"); System.out.println(" " + user1); user1.setLocation("Auburn"); user1.setAge(19); user1.logOn(); System.out.println(" " + user1); UserInfo user2 = new UserInfo("Sam", "Jones"); System.out.println(" " + user2); user2.setLocation("Atlanta"); user2.setAge(21); user2.logOn(); System.out.println(" " + user2); } }
Activity: Writing Classes Page 1 of 10 Terminology UML class diagram encapsulation attributestate behavior class method header class header instance variable calling method method declaration method invocation return statement parameters constructor visibility for access modifier accessor method Sutator= Goals By the end of this activity you should be able to do the following Create a class with methods that accept parameters and return a value Understand the constructor and the toString method of a class Create a class with main that uses the class created above Create a jGRASP project containing all of the classes for your program Generate a UML class diagram and documentation Create a GRASP canvas for your program and run the program in the canvas Description In this activity, you will create two classes: obe called User Info and another called UserInfoDriver which contains a main method that uses the first class (ie, UserinfoDriver creates instances of User Info and calls its methods). You will also create a project, UML class diagram, and canvas for the program Part 1: User Info java - Create and test the User Info class. Create your User Info class and add the following comments (there will be no main method in this claus). Save this class in a file named Userinfo.java as soon as you have entered the code below. Don't forget to add your Javadoc comments; the constructor and each method will need one. public class User Into 11 Instande variable // constructor // methods Instance variables (or fields) represent the information that an object of the class needs to store. Declare the following variables after the comment instance Table first Name: a String for the user's first name last Name: a String for the user's last name location: a String for the user's location age: an int for the user's age status: int indicates whether the user is online or offline Hint: Use the private access modifier so that values cannot be directly accessed from outside of the object, for example, declare fursame as follows: Private String name: Constants store values that cannot be changed. Use constants to represent the two possible statuses of the user. Constants are usually grouped with the instance variables Page 1 of 10 Activity: Writing Classes Page 2 of 10 private static final art OFFLINE = 0, OUTLINE = 1; The constructor is used in conjunction with the new operator to create an instance of UserInfo and initialize the fields. It should be placed right below the fields and before the methods in the class that is right after the comment constructor in your class. The constructor does not have a re s pe, and it always has the same name as the class. The serinfo contractor will take two parameters as put representing the first and last name of the user. The names for these parameters can be anything: however, many professional programmers use the convention of adding the suits to the corresponding field name as shown below. public User Info String first anein. String lastein) In the constructor, store the first and last name in the appropriate instance variables: Firstame-firettamen; lastName - lastNameIn; Because you do not have inputs for age, location and status, you will need to set those to default values - location - "Hot specified": age = 0; status = OFFLINE; Compile your program, click the Interactions tab, and create a new UserInfo object called u and display it by entering the following in Interactions tab. UserInfo u - new UserInfo(Jane. "Lane"); User Infoeda3da69 Note that the odd looking value (clasaname hashcode, which is the object's default toString value, is not very useful When we print or display we weally want to see some text that tells us something about the object. In the Workbench tab, find and unfold it to see its fields. We can see that the fields isu do have the values that we set with constructor. The solution is to add a to Soring method to our Userinfo class. (bj 120 Userinfo) Useinfo firstame- Jane" obj 409 lang String) priste ja lang String astame > Lane 10 l ang String) puste jarelang String ocation - Not specied" (ob 421 salong String) private ja lang age-0 : private int declared in Userno status = 0 priteit: declared inserito OFFLINEpuste final statici: declared in Userno ONLINE = 1 pristal sait decredinci Page 2 of 10 Activity: Writing Classes Page 3 of 10 The toString method returns a String representation of the object. Create and return a local String variable called output that contains information about the Userinfo object. Place this method after the methods comment // -eshods This method returns a String public String tostring() { String output - Names + tiretame + - + lastName + " "; output + "Location: + location + " "; - output +- "Age + age + " "; output Status + status return output; After you have successfully compiled your class, click the Interactions tab, and create a new Userinfo object called and display it by entering the following in Interactions tab as you did above. This time, when u is evaluated, the toString method is implicitly invoked on u and the return value is displayed User Into u - new VeerInto("Jane", "Lane"); Namci Jane Lane Location. Not specified Age: 0 Status: In the example above, you can also display by entering the print statement in interactions: System.out.println(); When the is entered without a semi-colon, it is an expression rather than a statement. You could also enter u.toString() as an expression. Remember, in the Interactions tab, expressions are evaluated and the result is immediately displayed. Since u is an object, it "evaluates to the result of calling its to sing method. The methods of your class describe what your object can do the behaviors of an object) For a Userinfo object, we want to be able to set the location and age of the user and also to change the status. First, create a set method for location: Method does not retum a value. public void setLocation(String locationin) { location - location in; After compiling your class, test it in the interactions pane: User Info u = new UacrInfo("Jane. "Lanc"); u.setLocation Auburn"); Name: Jane Lane Location Auburn Agen D Status: 0 Page 3 of 10 Activity: Writing Classes Page 4 of 10 Add a method to set the value of age. This will only change age if the age is greater than 0, and it will return a boolean value true or false) indicating whether the age was set: public boolean setAge(int ageIn) { - boolean isset = false; Method returns either true or false. --if(ageIn > 0) { age - agen; isset = true; - return isset; Add a method to return the age (fill in the blank) Notice this method takes no parameters, but returns an int value instead of void)- public int getAge() { return Method returns an int value. Finally, add a method to return the location of the user (fill in the blanks). public getLocation() { return ; Add the following two methods to allow the user to log off and on: public void logorr() { status = OFFLINE; public void logon() { status - ONLINE; The actual values (O and I) for offline status and online status are not used in the code because they are declared as constants. This makes code easier to read and modify later. You should also hide the values when printing the class information. Modify the toString method as follows to print Offline rather than 0 and Online rather than 1: public String tostring() { = String output - Names - + firstName + .. + lastName + " "; - output - Location: - location + " "; output t=Ages tage + " "; output += Status if(status -- OFFLINE) { If statement uses the String output t= Offline "Online" or "Online rather than 0 or 1 for status in the return yelset output = Online"; value for the toString method. return output; Page 4 of 10 Activity: Writing Classes Page 5 of 10 After compiling your class, test each of your methods in the interactions pane. Your output should be as follows: User Info = new UserInfo[Jane. "Lane); Name: Jane Lane Location: Not specified Ages 0 Status: Online u.setAge(23) u.setLocation Auburn"); w. logon(); Name: Jane Lane Location Auburn Age: 23 L Status: Online Part 2: User Info Driver.java-Create UserinfoDriver class with main method; create a project file and generate the UML class diagram for the program, create canvas for UserInfoDriver and run the program in the canvas UserInfoDriver class with main - You are to create another class, Userinfo Driver, which should have a main method that creates tuo instances of Userinfo and invokes methods on these instances. In the code below to instances of Userinfo are created and assigned to variables user1 and user2 respectively. These instances are printed after they are created. Then after several methods are called on instances, the instances are printed again Enter the code below incrementally i.e., write the skeleton code first and save it as Userinfo Driver.java in the same folder as UserInfo. Then compile run at strategic points to ensure that the program is correct down to that point and that you understand what each statement does. Don't forget to add your Javadoc comments 3 public class User Infobriser publie state and String args) sartot USA ); ) sertato art System.out.println sort.stocation r.sagt r.log (); System.out.println(" GAT) Somes) Userna USAY2 - Osortafocs System.out.println( 2) sara. cattolan 2. 21) war. ) System.out.println(" user); = Web-CAT - After you have created the GRASP project in the next section, you should submit your serint.jave and rerne rez.jere files to Web-CAT via the Web-CAT button on the project toolbar Page 5 of 10 - Estimate of Problem Coverage (Unknown!) Problem coverage: unknown Your code failed to compile correctly against the reference tests. This is most likely because you have not named your class(es) as required in the assignment, have failed to provide one or more required methods, or have failed to use the required signature for a method. Failure to follow these constraints will prevent the proper assessment of your solution and your tests. The following specific error(s) were discovered while compiling reference tests against your submission: 04/UserInfoDriver Tests.java: 169: error: incompatible types: int cannot be converted to String String testVar = d.getLocation(); 04/UserInfoDriverTests.java:202: error: incompatible types: int cannot be converted to String String testVar = d.getLocation(); 04/UserInfoDriver Tests.java:318: error: cannot find symbol d.logoff(); symbol: method logoff) location : variable d of type UserInfo 3 errorsStep by Step Solution
There are 3 Steps involved in it
Step: 1
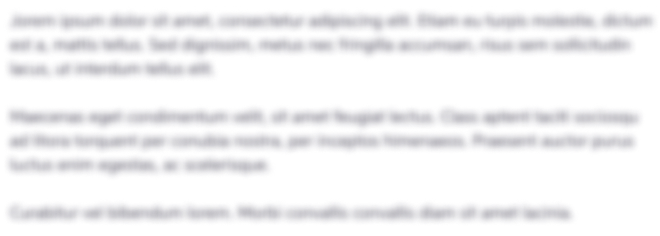
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started