Question
Hey guys ! Need help ! Can not get my code to run from my nodelist. When i run it referencing my array it works,
Hey guys ! Need help ! Can not get my code to run from my nodelist. When i run it referencing my array it works, however when I try to reference my nodelist, it does not work please help!:
package zipcode;
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner;
public class ZipCodeDriver { public static void main(String[] args) { //file contening the data that will be loaded into the array String filename = "zipcodes.txt";
// Load data try { Scanner infile = new Scanner(new File(filename)); ZipCodeList1 myZipCodelist = new ZipCodeList1(); ZipCode c; while(infile.hasNext()){ c = new ZipCode(); c.setZipcode(infile.nextLine()); c.setTown(infile.nextLine()); c.setState(infile.nextLine()); myZipCodelist.add(c); } infile.close(); System.out.println("Loaded " + myZipCodelist.getSize() + " zip codes"); // Search for the data String search = ""; Scanner keyboard = new Scanner(System.in); while(true){ System.out.print("Hello, please enter zip code to search (type done to stop): "); search = keyboard.next(); if(search.equalsIgnoreCase("done")) break; c = myZipCodelist.find(search); if(c == null) System.out.println("Sorry but the zip code entered was not found"); else System.out.println("Found " + search + ": [" + c + "]"); } // In case we don''t find the data } catch (FileNotFoundException e) { System.out.println(e.getMessage()); } }
}
--
package zipcode;
public class ZipCode {
//add components private String zipcode; private String town; private String state;
//add constructors public String getZipcode() { return zipcode; } public void setZipcode(String zipcode) { this.zipcode = zipcode; } public String getTown() { return town; } public void setTown(String town) { this.town = town; } public String getState() { return state; } public void setState(String state) { this.state = state; } public String toString(){ return "ZipCode: " + zipcode + ", Town: " + town + ", State: " + state; } } --
package zipcode;
public class ZipCodeList { // Add components; The limit of the size, the array holding the data and a counter private static int MAX_LIST_SIZE = 1000; private ZipCode[] zipcodes; private int count; //counter public ZipCodeList(){ zipcodes = new ZipCode[MAX_LIST_SIZE]; count = 0; } //Limit of the array public void add(ZipCode c){ if(count == MAX_LIST_SIZE){ System.out.println("Could not add " + c); } else{ zipcodes[count++] = c; } } //find the zipcode public ZipCode find(String code){ for(int i = 0; i < count; i++){ if(zipcodes[i].getZipcode().equals(code)) return zipcodes[i]; } // not found return null; } //find size public int getSize(){ return count; } }
--
import zipcode.ZipCode;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * * @author camil */ public class ZipCodeListNode { public ZipCode data; public ZipCodeListNode next; public ZipCodeListNode(ZipCode data, ZipCodeListNode next) { this.data = data; this.next = next; } public ZipCodeListNode(ZipCode data) { this.data = data; } } class ZipCodeList1 { private int count; private ZipCodeListNode head;
public ZipCodeList1() { head = null; count = 0; }
public void add(ZipCode c) { head = new ZipCodeListNode(c, head); count++; }
public ZipCode find(String code) { ZipCodeListNode start = head; while(start != null) { if(start.data.getZipcode().equals(code)) { return start.data; } start = start.next; }
return null; // not found }
public int getSize() { return count; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
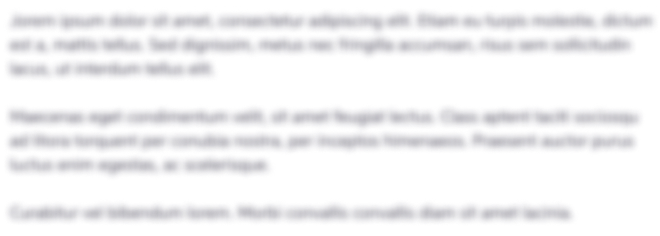
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started