Question
Hey, I need a big help over here with this C++ Problem. I need to develop a program in which I implement the LinkedBag class,
Hey, I need a big help over here with this C++ Problem. I need to develop a program in which I implement the LinkedBag class, but I am having trouble running the files together. I will provide the source files, they are exact as the book, I just need to add these 3 new functions. PLEASE INCLUDE A MAIN.CPP FILE TO VERIFY THAT IT'S RUNNING.
Instructions:
By using a list of integers numbers, entered by the user, implement an invocation of the Copy Constructor from LinkedBag. Also, create three functions of Linked Bag and implement them.
The functions are:
1. AddLast: Function that add a number at the end of the list.
2. RemoveItem: Function that delete an element without alter the order of the list.
3. Display: Function that display the content of the list.
Node.h
#ifndef _NODE
#define _NODE
#include
#include
#include
using namespace std;
template
class Node
{
private:
ItemType item; // A data item
Node
public:
Node();
Node(const ItemType& anItem);
Node(const ItemType& anItem, Node
void setItem(const ItemType& anItem);
void setNext(Node
ItemType getItem() const ;
Node
}; // end Node
#endif
Node.cpp
#include "Node.h"
#include
template
Node
{
} // end default constructor
template
Node
{
} // end constructor
template
Node
item(anItem), next(nextNodePtr)
{
} // end constructor
template
void Node
{
item = anItem;
} // end setItem
template
void Node
{
next = nextNodePtr;
} // end setNext
template
ItemType Node
{
return item;
} // end getItem
template
Node
{
return next;
} // end getNext
LinkedBag.h
#ifndef _LINKED_BAG
#define _LINKED_BAG
#include
#include
#include
#include "BagInterface.h"
#include "Node.h"
using namespace std;
template
class LinkedBag : public BagInterface
{
private:
Node
int itemCount; // Current count of bag items
// Returns either a pointer to the node containing a given entry
// or the null pointer if the entry is not in the bag.
Node
public:
LinkedBag();
LinkedBag(const LinkedBag
virtual ~LinkedBag(); // Destructor should be virtual
int getCurrentSize() const;
bool isEmpty() const;
bool add(const ItemType& newEntry);
bool remove(const ItemType& anEntry);
void clear();
bool contains(const ItemType& anEntry) const;
int getFrequencyOf(const ItemType& anEntry) const;
vector
}; // end LinkedBag
#endif
LinkedBag.cpp
#include "LinkedBag.h"
#include "BagInterface.h"
#include "Node.h"
#include
using namespace std;
template
LinkedBag
{
} // end default constructor
template
LinkedBag
{
itemCount = aBag.itemCount;
Node
if (origChainPtr == nullptr)
headPtr = nullptr; // Original bag is empty
else
{
// Copy first node
headPtr = new Node
headPtr->setItem(origChainPtr->getItem());
// Copy remaining nodes
Node
origChainPtr = origChainPtr->getNext(); // Advance original-chain pointer
while (origChainPtr != nullptr)
{
// Get next item from original chain
ItemType nextItem = origChainPtr->getItem();
// Create a new node containing the next item
Node
// Link new node to end of new chain
newChainPtr->setNext(newNodePtr);
// Advance pointer to new last node
newChainPtr = newChainPtr->getNext();
// Advance original-chain pointer
origChainPtr = origChainPtr->getNext();
} // end while
newChainPtr->setNext(nullptr); // Flag end of chain
} // end if
} // end copy constructor
template
LinkedBag
{
clear();
} // end destructor
template
bool LinkedBag
{
return itemCount == 0;
} // end isEmpty
template
int LinkedBag
{
return itemCount;
} // end getCurrentSize
template
bool LinkedBag
{
// Add to beginning of chain: new node references rest of chain;
// (headPtr is null if chain is empty)
Node
nextNodePtr->setItem(newEntry);
nextNodePtr->setNext(headPtr); // New node points to chain
// Node
headPtr = nextNodePtr; // New node is now first node
itemCount++;
return true;
} // end add
template
vector
{
vector
Node
int counter = 0;
while ((curPtr != nullptr) && (counter < itemCount))
{
bagContents.push_back(curPtr->getItem());
curPtr = curPtr->getNext();
counter++;
} // end while
return bagContents;
} // end toVector
template
bool LinkedBag
{
Node
bool canRemoveItem = !isEmpty() && (entryNodePtr != nullptr);
if (canRemoveItem)
{
// Copy data from first node to located node
entryNodePtr->setItem(headPtr->getItem());
// Delete first node
Node
headPtr = headPtr->getNext();
// Return node to the system
nodeToDeletePtr->setNext(nullptr);
delete nodeToDeletePtr;
nodeToDeletePtr = nullptr;
itemCount--;
} // end if
return canRemoveItem;
} // end remove
template
void LinkedBag
{
Node
while (headPtr != nullptr)
{
headPtr = headPtr->getNext();
// Return node to the system
nodeToDeletePtr->setNext(nullptr);
delete nodeToDeletePtr;
nodeToDeletePtr = headPtr;
} // end while
// headPtr is nullptr; nodeToDeletePtr is nullptr
itemCount = 0;
} // end clear
template
int LinkedBag
{
int frequency = 0;
int counter = 0;
Node
while ((curPtr != nullptr) && (counter < itemCount))
{
if (anEntry == curPtr->getItem())
{
frequency++;
} // end if
counter++;
curPtr = curPtr->getNext();
} // end while
return frequency;
} // end getFrequencyOf
template
bool LinkedBag
{
return (getPointerTo(anEntry) != nullptr);
} // end contains
// private
// Returns either a pointer to the node containing a given entry
// or the null pointer if the entry is not in the bag.
template
Node
{
bool found = false;
Node
while (!found && (curPtr != nullptr))
{
if (anEntry == curPtr->getItem())
found = true;
else
curPtr = curPtr->getNext();
} // end while
return curPtr;
} // end getPointerTo
BagInterface.h
#ifndef _BAG_INTERFACE
#define _BAG_INTERFACE
#include
using namespace std;
template
class BagInterface
{
public:
/** Gets the current number of entries in this bag.
@return The integer number of entries currently in the bag. */
virtual int getCurrentSize() const = 0;
/** Sees whether this bag is empty.
@return True if the bag is empty, or false if not. */
virtual bool isEmpty() const = 0;
/** Adds a new entry to this bag.
@post If successful, newEntry is stored in the bag and
the count of items in the bag has increased by 1.
@param newEntry The object to be added as a new entry.
@return True if addition was successful, or false if not. */
virtual bool add(const ItemType& newEntry) = 0;
/** Removes one occurrence of a given entry from this bag,
if possible.
@post If successful, anEntry has been removed from the bag
and the count of items in the bag has decreased by 1.
@param anEntry The entry to be removed.
@return True if removal was successful, or false if not. */
virtual bool remove(const ItemType& anEntry) = 0;
/** Removes all entries from this bag.
@post Bag contains no items, and the count of items is 0. */
virtual void clear() = 0;
/** Counts the number of times a given entry appears in bag.
@param anEntry The entry to be counted.
@return The number of times anEntry appears in the bag. */
virtual int getFrequencyOf(const ItemType& anEntry) const = 0;
/** Tests whether this bag contains a given entry.
@param anEntry The entry to locate.
@return True if bag contains anEntry, or false otherwise. */
virtual bool contains(const ItemType& anEntry) const = 0;
/** Empties and then fills a given vector with all entries that
are in this bag.
@return A vector containing all the entries in the bag. */
virtual vector
}; // end BagInterface
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
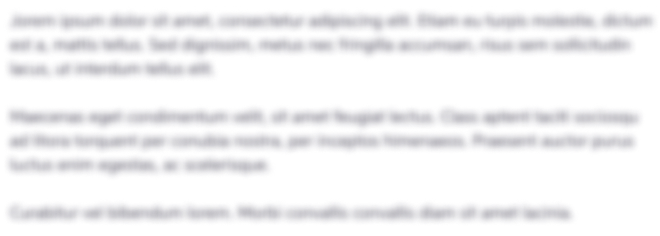
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started