Question
Hey, I need help doing my assignment, CST8116 Assignment 02 Software Development Process, design and create an Object Oriented Java Program Instructions The Software Development
Hey, I need help doing my assignment,
CST8116 Assignment 02
Software Development Process, design and create an Object Oriented Java Program
Instructions
- The Software Development Process as presented by Cay Horstmann [1] will be used as the basis for this lab assignment.
1) Understand the problem
2) Develop and Describe an Algorithm
3) Test Algorithm with Simple Inputs
4) Translate the Algorithm into Java
5) Compile and Test Your Program
1) Understand the problem
- A client (a person who wants a program developed) who owns a car rental company wants a program developed that will estimate the litres of gas needed to drive a car a given distance in kilometers on a highway for a road-trip. Currently staff at the rental desk need to ask the customer the estimated mileage the car will be driven on the highway, they then need to look up the fuel efficiency of the car to be rented and use that within the math needed to determine the amount of gasoline in litres the customer will need for their road-trip. A typical fuel economy for the rental company's cars is 6.8L/100Km, most customers have round trips of 900Km on the highway, for example from Ottawa to Toronto. The program should allow the entry of the distance to drive, the litres per 100Km for efficiency, and output the Litres of gasoline needed, rounded up to a whole number when producing the result.
- You would only need to input 6.8 for the efficiency, work with 100Km internally in the program as part of your math.
- Write out briefly how you would do each step, as if you were the staff member. Including examples of math calculations you would perform either by hand, or with the assistance of a calculator.
- Type this into your MS Word document as part of your submission.
2) Develop and Describe an Algorithm including Classes
- Determine what objects, the properties of the objects, and behaviors would be required for an object oriented program, start with simple UML class diagrams and as you work out more details document the design with a detailed UML class diagram.
- Write pseudo-code as the basis of your algorithm, as well as create a flowchart. You should reference the lecture notes, as well as your textbook by Joyce Farrell [2] as learning resources for this part of the assignment.
- You should show object instantiation in the flowchart as variable declarations, then use method calls to methods. Note, you are not required to document get/set accessor, mutator methods in your algorithm or flowchart in detailed step-by-step, only method main and any method(s) that perform math calculations.
- You may hand-draw the flowchart and UML class diagram for this assignment instead of using software, just use a ruler and print legibly.
- Alternatively a software program like Diagrams.net can be used for flowchart while a software program like UMLet can be used to create the UML class diagrams. Other programs like MS Visio, or even MS Word can be used instead.
- Your name as author must appear within any diagrams you create.
- If working on paper either scan the diagram into your computer, or use a cell phone to take a picture and email it to yourself. Both your pseudocode and flowchart should be placed into your MS Word document, the pseudocode as text while the flowchart should be an image. Note: The pseudocode and flowchart should document the exact same algorithm.
- Your UML diagram provides a high-level view of the Structure of the objects, while the pseudocode and flowcharts provide the basis for the sequential logic within method main.
3) Test Algorithm with Simple Inputs
- As per the lecture notes, use a table within your MS Word document to test the algorithm. Consider picking numbers that might be expected as input and work through the algorithm documenting expected outputs, you may use a calculator.
- If there is a problem with the algorithm based on this desk-check correct the pseudocode and flowchart, UML class diagram(s) and repeat this step again.
4) Translate the Algorithm into Java
- You are to use the Eclipse IDE to create your Java program, use a project name like Assignment 02.
- Dont forget to comment your code files, with the expected code header.
- You are not required to copy and paste code into the MS Word document, however your .java file(s) must be submitted.
5a) Compile and Run Your Program
- Compile and run your program, using your documented test values.
- Take a screen shot of Command prompt window, or a rectangular selection of the Eclipse Console View depending on your development environment, ensure your name as output by the program is captured in the image.
5b) Test Your Program
- Re-create your testing table from step 3 in this section, but document what the program outputs are, do they match expectations?
- Test with some invalid inputs, and document what happens. Note that some input will crash your program, this is okay as you may not know how to fix this at this point in the course. Document what the error messages are in your test plan. Suggested invalid tests: enter a String instead of a number.
- The test plan for the program must be a separate test plan in your document, if you provide only one test plan table for both testing the algorithm and testing the program you will not be awarded marks for both.
Microsoft Word Document Format
See the template example, suggested headings below:
1) Understand the problem
- Brief paragraph
2) Develop and Describe an Algorithm
- UML class diagrams, Pseudocode, Flowchart
3) Test Algorithm with Simple Inputs
- Test plan as table
4) Compile and Run Your Program
- Screen shot of running program with student name as output
5) Test Your Program
- Second test plan as table, including notes on what happens with invalid inputs.
6) References:
- Document any sources you used that are either from your textbooks, or external from the lecture handouts.
- Note: You are not required to reference class Math from the Java API, it is assumed you will refer to the API documentation as part of completing this lab work.
While you are not required to copy and paste your source code inside the MS Word document, you must upload your .java file(s) in addition to the MS Word document.
Grading (18 points)
Criteria | Missing / Poor (0) | Below Expectations (1) | Meets Expectations (2) |
Understand the problem | Missing or poorly done. | Not all requirements are documented, steps are not in a sequential order. | Problem solution statement is correct, with logical sequence of steps. |
Algorithm: UML class diagram | Missing or poorly done, or program does not use objects designed by the student. | Class diagram(s) are not in correct format, properties and methods may not be assigned correctly to the classes and / or there is only one class that contains all of the program functionality. | Class diagram(s) are correct format, properties and methods are assigned to appropriate classes, based on the word problem. |
Algorithm: pseudocode | Missing or poorly done, or program does not use objects designed by the student. | Does not meet all requirements: Correct format, steps are in an order that produces correct results. | Meets requirements: Correct format, steps are in order that produces correct results. |
Algorithm: flowchart | Missing or poorly done, or program does not use objects designed by the student. | Does not meet all requirements: Flowchart has start, stop, input/output, processing, and sequential flow and / or flowchart algorithm matches pseudocode algorithm and flowchart parts are put together correctly. | Meets all requirements: Flowchart has start, stop, input/output, processing, and sequential flow and / or flowchart algorithm matches pseudocode algorithm and flowchart parts are put together correctly. |
Test Plan: Algorithm | Missing or poorly done. | Does not meet all requirements: Has correct table format as seen in lecture, tests one valid value with entries in the row for each column. | Meets all requirements: Has correct table format as seen in lecture, tests one valid value with entries in the row for each column. |
Source Code | Missing or poorly done, missing student full name in programmer comment at top of file and / or program does not use classes created by the student. | Does not meet all requirements: Comment header is complete and correct. Has appropriate class name, permits inputs, performs calculations with class Math as needed, has required outputs. | Meets all requirements: Comment header is complete and correct. Has appropriate class name, permits inputs, performs calculations with class Math as needed, has required outputs. |
Screen Shot Running Program | Missing or poorly done or students name is not in the output of the program within the screen shot. | Does not meet all requirements: Screen shot must show inputs, outputs, including students full name. | Meets all requirements: Screen shot must show inputs, outputs, including students full name. |
Test Plan: Program | Missing or poorly done. | Does not meet all requirements: Has correct table format as seen in lecture, tests valid values with entries in the row for each column. Documents errors when input is invalid. | Meets all requirements: Has correct table format as seen in lecture, tests valid values with entries in the row for each column. Documents errors when input is invalid. |
Submission | Missing | Student does not upload both the MS Word document and .java file. And / or places them inside a zip file. | Student uploads the MS Word document, and .java program as two separate files (no zip file was used). |
Submission Requirements
- Follow your lab professors requirements for submission of lab work, you will need to submit your MS Word document and your Java source code file(s).
References
[1] Cay Horstmann. (2019). Big Java Early Objects. 7th Ed. Wiley.
[2] Joyce Farrell. (2018). Programming Logic & Design Comprehensive. 9th Ed. Cengage Learning.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
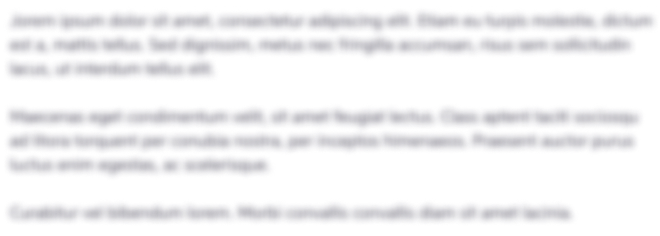
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started