Question
Hi, everyone I have a errors in this part of the program. I hope someone can help me fixed these errors in LinkedList.cpp f so
Hi, everyone
I have a errors in this part of the program. I hope someone can help me fixed these errors in LinkedList.cpp f so i can compile whole the program properly. Showing all 57 tests in the main.cpp passing without failure after compiling. I will give an thumbs up or great rating in your answer.
Thank your help.
Here is the errors need fixing (you will find this parts in the LinkedList.cpp):
Here all the parts of the program:
(1) LinkedList.cpp
#include#include "LinkedList.h" LinkedList::LinkedList(){ first = NULL; } LinkedList::~LinkedList(){ Node *x = first; while(x != NULL) { Node *y = x; x = x ->next; delete y; } } LinkedList::LinkedList(const LinkedList &origList){ int count = 0; Node *x = origList.first; while(x != NULL) { insert(x->data, count++); x = x ->next; } } const LinkedList & LinkedList::operator=(const LinkedList & rightHandSide){ resetList(); int count = 0; Node *x = rightHandSide.first; while(x != NULL) { insert(x->data, count++); x = x ->next; } return *this; } bool LinkedList::isEmpty() const{ return mySize == 0; } int LinkedList::getListSize() const{ return mySize; } void LinkedList::display(std::ostream & out) const{ Node *x = first; while(x != NULL) { out data next; } out next; delete y; } first = NULL; mySize = 0; } LinkedList::ErrorCode LinkedList::insert(ListElement item, int pos){ int size = getListSize(); if(pos size) { return ILLEGAL_LIST_POSITION; } Node *x = new Node; x->data = item; if(pos == 0) { x->next = first; first = x; } else { Node *y = first; while(pos != 1) { y = y ->next; pos--; } x->next = y->next; y->next = x; } mySize++; return NO_ERROR; } LinkedList::ErrorCode LinkedList::erase(int pos){ int size = getListSize(); if(pos = size) { return ILLEGAL_LIST_POSITION; } if(pos == 0) { Node *y = first; first = first->next; delete y; } else { Node *y = first; while(pos != 1) { y = y ->next; pos--; } Node *x = y->next; y->next = y->next->next; delete x; } mySize--; return NO_ERROR; } LinkedList::ErrorCode LinkedList::move(int n, int m){ int size = getListSize(); if(n = size) { return ILLEGAL_LIST_POSITION; } if(m = size) { return ILLEGAL_LIST_POSITION; } if(m != n) { Node *a = first; int count = 0; while(a != NULL) { if(n == count) { break; } a = a ->next; count++; } ListElement temp = a->data; erase(n); insert(temp, m); } return NO_ERROR; } void LinkedList::reverse(){ Node *current = first; Node *prev = NULL, *next = NULL; while (current != NULL) { next = current->next; // Reverse current node's pointer current->next = prev; prev = current; current = next; } first = prev; } LinkedList::ErrorCode LinkedList::getListElement(int posStart, int posEnd, ListElement rv[]) const { int size = getListSize(); if(posStart = size) { return ILLEGAL_LIST_POSITION; } if(posEnd = size) { return ILLEGAL_LIST_POSITION; } if(posEnd next; count++; } a = x; while(a != y->next) { rv[index++] = a->data; a = a->next; } return NO_ERROR; }
(2) LinkedList.h
#include
#include
#ifndef LINKED_LIST_H
#define LINKED_LIST_H
using namespace std;
class LinkedList
{
public:
typedef int ListElement;
private:
/******** Data Members ********/
class Node
{
public:
ListElement data;
Node * next;
};
Node *first; // pointer to first element in linked list
int mySize; // current size of list
public:
/******** Function Members ********/
enum ErrorCode { ILLEGAL_LIST_POSITION = -1, NO_ERROR = 0 };/******** Error Codes ********/
LinkedList();//--- Definition of class constructor
~LinkedList();//--- Definition of class destructor
LinkedList(const LinkedList & origList);//--- Definition of copy constructor
const LinkedList & operator=(const LinkedList & rightHandSide);//--- Definition of assignment operator
bool isEmpty() const;//--- Definition of isEmpty()
/***** mutators *****/
void resetList();//--- Definition of resetList()
ErrorCode insert(ListElement item, int pos);//--- Definition of insert()
ErrorCode erase(int pos);//--- Definition of erase()
ErrorCode move(int n, int m);//--Definition of move operation()
void reverse();//--Definition of reverse()
// Accessors
ErrorCode getListElement(int posStart, int posEnd, ListElement rv[]) const;//--- Definition of getListElement()
int getListSize() const;//--- Definition of getListSize()
void display(std::ostream & out) const;//--- Definition of display()
}; //--- end of List class
LinkedList operator+(const LinkedList & x, const LinkedList & y);//--- Definition of + operator
int operator==(const LinkedList & x, const LinkedList & y);//--- Definition of == operator
#endif
(3) MyFileIO.cpp
#include
#include
#include
#include
#include
#include
#include "MyFileIO.h"
bool fileExists(std::string st)
{
bool result;
std::ifstream infile(st.c_str());
result = infile.good();
if (infile.is_open() == true)
{
infile.close();
}
return (result);
}
bool writefile(std::string filename, char *ptr, int length)
{
bool result = false;
std::ofstream outfile(filename.c_str(), std::ios::out | std::ios::binary);
if (outfile.is_open() == true)
{
outfile.write(ptr, length);
outfile.close();
result = true;
}
return(result);
}
std::string nowtoString()
{
time_t now = time(0);
tm tstruct;
char buf[80];
localtime_s(&tstruct, &now);
strftime(buf, sizeof(buf), "%Y-%m-%d.%X", &tstruct);
std::string st(buf);
return st;
}
int build_file_array(std::string st, int ptr, char data[])
{
getChars(0, st.length(), data, ptr, st);
return (ptr + st.length());
}
void getChars(int beginst, int endst, char data[], int ptr, std::string st)
{
int i;
for (i = 0; i
{
data[i + ptr] = st[beginst + i];
}
}
std::string valueOf(int num)
{
std::stringstream ss;
ss
return(ss.str());
}
std::string getCRLF()
{
std::string CRLF = "\x0D\x0A";
return CRLF;
}
std::string getCode(int ptr, char data[])
{
int code = 0;
for (int i = 0; i
{
code = code + (int)data[i];
}
return (valueOf(code) + getCRLF());
}
(4) MyFileIO.h
#ifndef MYFILEIO_H
#define MYFILEIO_H
#include
#include
bool fileExists(std::string st);
bool writefile(std::string filename, char *ptr, int length);
std::string nowtoString();
void getChars(int beginst, int endst, char data[], int ptr, std::string st);
int build_file_array(std::string st, int ptr, char data[]);
std::string valueOf(int num);
std::string getCRLF();
std::string getCode(int ptr, char data[]);
#endif
(5) Main.cpp
#include
#include
#include
#include "LinkedList.h"
#include "MyFileIO.h"
bool compareArray(int array1[], int array2[], int count);
void testCases(bool extraCredit);
int main()
{
testCases(false); // for extra credit test put true, otherwise false
return 0;
}
void testCases(bool extraCredit)
{
std::string FILENAME = "LLresults.txt";
std::string outString;
std::string PASSED = " Passed";
std::string FAILED = " Failed";
std::string TEST_CASE = "Test Case #";
int array[50]; // holds max list size I need for testing
int array1[] = { 11, 22, 33, 44 }; // pattern used for testing
LinkedList::ErrorCode tempInt;
LinkedList empty1; // empty list
LinkedList a; // list a used for testing
int temp8;
int testCount = 1; // used to track test numbers
bool temp;
char ch[100000]; // array large enough for the file output
int char_ptr = 0; // file index pointer
// Test Case #1 - test isEmpty
temp = empty1.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #2 - check empty list with getListSize()
int size = a.getListSize();
if (size == 0)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #3 - test insert() and isEmpty() with one element
tempInt = a.insert(11, 0); // insert at first position
temp = a.isEmpty();
if (temp == false && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #4 - test getListSize() with one element
size = a.getListSize();
if (size == 1)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #5 - test that the one element is correct
tempInt = a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 1) == true && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #6 - test resetList from a non-empty list
a.resetList();
temp = a.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #7 - add 2 element to the beginning position
a.resetList();
a.insert(22, 0); // insert at first position
a.insert(11, 0); // insert at first position
size = a.getListSize();
if (size == 2)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #8 - test that the elements are correct
tempInt = a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 2) == true && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #9 - test that the list can be emptied with 2 elements in it
a.resetList();
temp = a.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #10 - insert from the beginning and last position
a.resetList();
a.insert(22, 0); // insert at first position
a.insert(11, 0); // insert at first position
a.insert(33, 2); // insert at last position
size = a.getListSize();
if (size == 3)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #11 - test that the 3 elements are correct
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 3) == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #12 - test that the 3 element list can be emptied
a.resetList();
temp = a.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #13 - insert elements to the front end and middle
a.resetList();
a.insert(22, 0); // insert at first position
a.insert(11, 0); // insert at first position
a.insert(44, 2); // insert at last position
a.insert(33, 2); // insert at middle position
size = a.getListSize();
if (size == 4)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #14 - test that the elements are correct
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 4) == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #15 - test the list can be emptied
a.resetList();
temp = a.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #16 - test an illegal insertion
a.resetList();
a.insert(22, 0); // insert at first position
a.insert(11, 0); // insert at first position
a.insert(44, 2); // insert at last position
a.insert(33, 2); // insert at middle position
tempInt = a.insert(99, -1); // insert in an illegal position
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 4) == true && tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #17 - test an illegal insertion
tempInt = a.insert(99, 5); // insert in an illegal position
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 4) == true && tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #18 - test an illegal erase
tempInt = a.erase(-1); // erase from an illegal position
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 4) == true && tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #19 - test an illegal erase
tempInt = a.erase(4); // erase from an illegal position
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 4) == true && tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #20 - test erase from an empty list
tempInt = empty1.erase(0); // erase from an empty list
temp = empty1.isEmpty();
if (temp == true && tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #21 - test erase from the end of the list
tempInt = a.erase(3); // erase from back of list
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 3) == true && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #22 - test erase from the middle of the list
tempInt = a.erase(1); // erase from middle of list
array1[1] = 33;
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 2) == true && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #23 - test erase from the front of the list
tempInt = a.erase(0); // erase from front of list
array1[0] = 33;
a.getListElement(0, a.getListSize() - 1, array);
if (compareArray(array, array1, 1) == true && tempInt == LinkedList::NO_ERROR)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #24 test erasing the last element so the list is empty
tempInt = a.erase(0); // erase the last element
temp = a.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #25 copy constructor - test if the lists are equal
a.insert(33, 0); // insert at first position
a.insert(11, 0); // insert at first position
a.insert(44, 2); // insert at last position
a.insert(22, 1); // insert at middle position
LinkedList b(a); // use copy constructor
int array2[] = { 11, 22, 33, 44 };
b.getListElement(0, b.getListSize() - 1, array);
temp = compareArray(array, array2, 4);
if ((temp == true) && (b.getListSize() == 4))
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #26 copy constructor - test shallow copy
b.insert(99, 1);
a.getListElement(1, 1, array);
temp8 = array[0];
if (temp8 == 22)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #27 assignment operator - test that the lists are equal
b.resetList();
b = a; // use assignment operator
b.getListElement(0, b.getListSize() - 1, array);
temp = compareArray(array, array2, 4);
if ((temp == true) && (b.getListSize() == 4))
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #28 assignment operator - shallow copy test
b.insert(99, 1);
a.getListElement(1, 1, array);
temp8 = array[0];
if (temp8 == 22)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #29 - test reverse() with empty list
empty1.reverse();
temp = empty1.isEmpty();
if (temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #30 - test reverse() with even number of elements
a.reverse(); // here a has 11, 22,33,44
a.getListElement(0, a.getListSize() - 1, array);
int array3[] = { 44, 33, 22, 11 };
temp = compareArray(array, array3, 4);
if ((temp == true) && (a.getListSize() == 4))
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #31 - test reverse() with odd number of elements
a.insert(55, 0);
a.reverse();
a.getListElement(0, a.getListSize() - 1, array);
int array4[] = { 11, 22, 33, 44, 55 };
temp = compareArray(array, array4, 5);
if ((temp == true) && (a.getListSize() == 5))
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #32 - test reverse() with 1 element
empty1.insert(55, 0);
empty1.reverse();
empty1.getListElement(0, empty1.getListSize() - 1, array);
array4[0] = 55;
temp = compareArray(array, array4, 1);
if ((temp == true) && (empty1.getListSize() == 1))
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #33 - test getListElement() for illegal positions
empty1.resetList();
tempInt = empty1.getListElement(-1, 1, array);
if (tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #34 - test getListElement() for illegal positions
empty1.resetList();
tempInt = a.getListElement(1, 0, array);
if (tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #35 - test getListElement() for illegal positions
empty1.resetList();
tempInt = a.getListElement(1, 5, array); // a has 5 elements now
if (tempInt == LinkedList::ILLEGAL_LIST_POSITION)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #36 - test move() from first to last position
empty1.resetList();
a.resetList();
a.insert(44, 0);
a.insert(11, 1);
a.insert(22, 2);
a.insert(33, 3);
tempInt = a.move(0, 3); // move first to last
a.getListElement(0, a.getListSize() - 1, array);
int array5[] = { 11, 22, 33, 44 };
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::NO_ERROR && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #37 - test move() from last to first position
a.resetList();
a.insert(22, 0);
a.insert(33, 1);
a.insert(44, 2);
a.insert(11, 3);
tempInt = a.move(3, 0); // move last to first
a.getListElement(0, a.getListSize() - 1, array);
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::NO_ERROR && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #38 - test move() from first to middle position
a.resetList();
a.insert(33, 0);
a.insert(11, 1);
a.insert(22, 2);
a.insert(44, 3);
tempInt = a.move(0, 2); // move first to middle
a.getListElement(0, a.getListSize() - 1, array);
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::NO_ERROR && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #39 move() - test move() from first to middle position
a.resetList();
a.insert(11, 0);
a.insert(44, 1);
a.insert(22, 2);
a.insert(33, 3);
tempInt = a.move(1, 3); // move first to middle
a.getListElement(0, a.getListSize() - 1, array);
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::NO_ERROR && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #40 - test move() error conditions
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
a.insert(44, 3);
tempInt = a.move(-1, 3); // move first to middle
a.getListElement(0, a.getListSize() - 1, array);
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::ILLEGAL_LIST_POSITION && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #41 move() - test move() error conditions
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
a.insert(44, 3);
tempInt = a.move(0, 4); // move first to middle
a.getListElement(0, a.getListSize() - 1, array);
temp = compareArray(array, array5, 4);
if (tempInt == LinkedList::ILLEGAL_LIST_POSITION && temp == true)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
if (extraCredit == true)
{
// Test Case #42 + operator - 2 empty lists
a.resetList();
b.resetList();
LinkedList c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
temp = c.isEmpty();
if (temp == true && c.getListSize() == 0)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #43 + operator - 2 lists with 1 element
a.resetList();
a.insert(11, 0);
b.resetList();
b.insert(-11, 0);
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
int array6[] = { 11, -11 };
temp = compareArray(array, array6, 2);
if (temp == true && c.getListSize() == 2)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #44 + operator - 2 lists with 1 element and empty
a.resetList();
a.insert(11, 0);
b.resetList();
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
temp = compareArray(array, array6, 1);
if (temp == true && c.getListSize() == 1)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #45 + operator - 2 lists, empty and 1 element
a.resetList();
b.resetList();
a.insert(11, 0);
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
temp = compareArray(array, array6, 1);
if (temp == true && c.getListSize() == 1)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #46 + operator - 2 lists with equal lengths of 3 elements each
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
b.resetList();
b.insert(-11, 0);
b.insert(-22, 1);
b.insert(-33, 2);
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
int array7[] = { 11, -11, 22, -22, 33, -33 };
temp = compareArray(array, array7, 6);
if (temp == true && c.getListSize() == 6)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #47 + operator - 2 lists where a has 5 elements and b has 3
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
a.insert(44, 3);
a.insert(55, 4);
b.resetList();
b.insert(-11, 0);
b.insert(-22, 1);
b.insert(-33, 2);
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
int array8[] = { 11, -11, 22, -22, 33, -33, 44, 55 };
temp = compareArray(array, array8, 8);
if (temp == true && c.getListSize() == 8)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #48 + operator - 2 lists where b has 5 elements and a has 3
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
b.resetList();
b.insert(-11, 0);
b.insert(-22, 1);
b.insert(-33, 2);
b.insert(-44, 3);
b.insert(-55, 4);
c = a + b;
c.getListElement(0, c.getListSize() - 1, array);
int array9[] = { 11, -11, 22, -22, 33, -33, -44, -55 };
temp = compareArray(array, array9, 8);
if (temp == true && c.getListSize() == 8)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #49 == operator - test 2 empty lists
a.resetList();
b.resetList();
if (a == b)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #50 == operator - test 1 empty list and one list with elements
a.resetList();
b.resetList();
b.insert(-11, 0);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #51 == operator - test 2 lists 11 and -11
a.resetList();
a.insert(11, 0);
b.resetList();
b.insert(-11, 0);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #52 == operator - test 2 lists 11 and 11
a.resetList();
a.insert(11, 0);
b.resetList();
b.insert(11, 0);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #53 == operator - test 2 lists 11,22,33,44 and 44,33,22,11
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
a.insert(44, 3);
b.resetList();
b.insert(44, 0);
b.insert(33, 1);
b.insert(22, 2);
b.insert(11, 3);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #54 == operator - test 2 lists 11,33,22,44 and 33,44,22,11
a.resetList();
a.insert(11, 0);
a.insert(33, 1);
a.insert(22, 2);
a.insert(44, 3);
b.resetList();
b.insert(33, 0);
b.insert(44, 1);
b.insert(22, 2);
b.insert(11, 3);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #56 == operator - test 2 lists 11,22,33,44 and 11,22,33,44,44
a.resetList();
a.insert(11, 0);
a.insert(22, 1);
a.insert(33, 2);
a.insert(44, 3);
b.resetList();
b.insert(11, 0);
b.insert(22, 1);
b.insert(33, 2);
b.insert(44, 3);
b.insert(44, 4);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #56 == operator - test lists 11,33,22,44 and 33,44,11,11
a.resetList();
a.insert(11, 0);
a.insert(33, 1);
a.insert(22, 2);
a.insert(44, 3);
b.resetList();
b.insert(33, 0);
b.insert(44, 1);
b.insert(11, 2);
b.insert(11, 3);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
// Test Case #57 == operator - test lists List x contains 1,1,1,2,2 and 1,2,1,2,1
a.resetList();
a.insert(1, 0);
a.insert(1, 1);
a.insert(1, 2);
a.insert(2, 3);
a.insert(2, 4);
b.resetList();
b.insert(1, 0);
b.insert(2, 1);
b.insert(1, 2);
b.insert(2, 3);
b.insert(1, 4);
if (a == b)
outString = TEST_CASE + valueOf(testCount) + PASSED + getCRLF();
else
outString = TEST_CASE + valueOf(testCount) + FAILED + getCRLF();
std::cout
char_ptr = build_file_array(outString, char_ptr, ch);
testCount++;
}
outString = getCRLF();
char_ptr = build_file_array(outString, char_ptr, ch);
outString = getCode(char_ptr, ch);
char_ptr = build_file_array(outString, char_ptr, ch);
outString = nowtoString();
char_ptr = build_file_array(outString, char_ptr, ch);
writefile(FILENAME, ch, char_ptr);
}
bool compareArray(int array1[], int array2[], int count)
{
bool rv = true;
for (int i = 0; i
{
if (array1[i] != array2[i])
rv = false;
}
return rv;
}
This program needs to passed all 57 tests by fixing/modifying the functions in LinkedList.cpp.
Here is my output (before i started modifying the functions in LinkedList.cpp so please fixed the errors in screenshots and if you could put your solution along with screenshot of your output) :
Step by Step Solution
There are 3 Steps involved in it
Step: 1
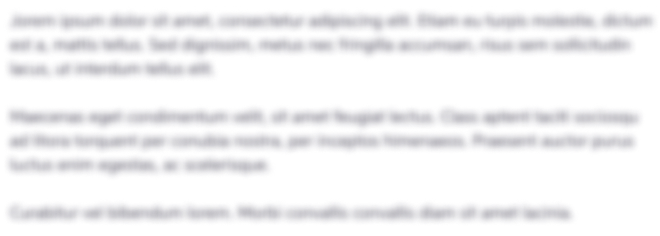
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started