Question
Hi I am somewhat new here so I apologize if there are any formatting issues...I have gotten my code for a project for a coding
Hi I am somewhat new here so I apologize if there are any formatting issues...I have gotten my code for a project for a coding club to mostly work, but the last problem I cant seem to find a fix for is that i keep getting this "Exception in thread "main" java.lang.AssertionError: Expected: 1, but got: 2". Below I will include my Source material with the intended project outcome, my Linked List Class and my Test class I have written, any help on why its not working owould be great! thank you.
SOURCE:
package edu.unomaha.csci3320.interfaces; public interface Sequence { /** * Inserts the given element at the specified index position within the * sequence. The element currently at that index position (and all subsequent * elements) are shifted to the right. * * @param index - the index at which the given element is to be inserted * @param x - the element to insert * @return true if and only if adding the element changed the sequence * @throws IndexOutOfBoundsException if the index is invalid * @throws NullPointerException if the given element is null */ boolean add(int index, T x) throws IndexOutOfBoundsException, NullPointerException; /** * Adds the specified element to the end of the sequence. * * @param x - the element to add * @return true if and only if adding the element changed the sequence * @throws NullPointerException if the given element is null */ boolean add(T x) throws NullPointerException; /** * Removes all of the elements from the sequence. */ void clear(); /** * Check if the given element belongs to the sequence. * * @param x - the element to check for * @return true if and only if the sequence contains the given element * @throws NullPointerException if the given element is null */ boolean contains(T x) throws NullPointerException; /** * Returns the element at the given position in the sequence. * * @param index - the index of the element to return * @return the element at the given position in the sequence * @throws IndexOutOfBoundsException if the index is invalid */ T get(int index) throws IndexOutOfBoundsException; /** * Returns the index position of the first occurrence of the given element * within the sequence or -1 if it does not belong. * * @param x - the element to search for * @return the index position of the first occurrence of the given element * within the sequence or -1 if it does not belong * @throws NullPointerException if the given element is null */ int indexOf(T x) throws NullPointerException; /** * Check if the sequence is empty. * * @return true if and only if the sequence is empty. */ boolean isEmpty(); /** * Removes the element at the given position in the sequence. * * @param index the index position of the element to be removed * @return the element at the given position in the sequence * @throws IndexOutOfBoundsException if the index is invalid */ T remove(int index) throws IndexOutOfBoundsException; /** * Remove the first occurrence of the given element from the sequence (if * present). * * @param x - the element to be removed from this sequence * @return true if and only if removing the element changed the sequence * @throws NullPointerException if the given element is null */ boolean remove(T x) throws NullPointerException; /** * Replaces the element at the given position of the sequence with the specified * element. * * @param index - index of the element to replace * @param x - the new element * @throws IndexOutOfBoundsException if the index is invalid * @throws NullPointerException if the given element is null */ void set(int index, T x) throws IndexOutOfBoundsException, NullPointerException; /** * Returns the number of elements in this sequence. * * @return the number of elements in this sequence */ int size(); /** * Returns an array containing all of the elements in this sequence (preserving * their order). * * @return an array containing all of the elements in this sequence (preserving * their order) */ Object[] toArray(); }
NEXT MY LINKED LIST CLASS
NEXT MY LINKED LIST CLASS
package edu.unomaha.csci3320.interfaces; import java.util.Arrays; public class LinkedList> implements Sequence { //Private inner class representing the nodes of the linked list private static class Node { T data; Node next; Node(T data) { this.data = data; } } private Node head; //Reference to the first node in the linked list private int size; //Number of element in the linked list @Override public boolean add(int index, T x) throws IndexOutOfBoundsException, NullPointerException { if (x == null) { throw new NullPointerException("Element cannot be null."); } if (index < 0 || index > size) { throw new IndexOutOfBoundsException("Invalid Index"); } if (index == 0) { addFirst(x); //If index is 0, add the element at the beginning of the list } else { head = addRecursive(head, index, x); //Otherwise recursively add the element at the given index } size++; return true; } //Recursive helper method ot add the element at the given index private Node addRecursive(Node current, int index, T x) { if (index == 0) { Node newNode = new Node<>(x); newNode.next = current; return newNode; } //Otherwise, recursively traverse to the next node and add the element at the appropriate index current.next = addRecursive(current.next, index - 1, x); return current; } @Override public boolean add(T x) throws NullPointerException { return add(size, x); //Add the element at the end of the list (same as add(size,x)) } @Override public void clear() { head = null; //Remove all elements by setting the head to null size = 0; //Reset the size to zero } @Override public boolean contains(T x) throws NullPointerException { if (x == null) { throw new NullPointerException("Element cannot be null."); } return containsRecursive(head, x); //Check if the element is present in the linked list recursively } //Recursive helper method to check if the element is present in the linked list private boolean containsRecursive(Node current, T x) { if (current == null) { return false; //If current node is null the element isnt present } if (current.data.equals(x)) { return true; //If the current node contains the element return true } //Otherwise, recursively check in the next node return containsRecursive(current.next, x); } @Override public T get(int index) throws IndexOutOfBoundsException { //checkIndex(index); //Check if the index is valid return getRecursive(head, index); //Get the element at the given index recusively } //Recursive helper method to get the element at the given index private T getRecursive(Node current, int index) { if (index == 0) { return current.data; //If index is 0, return the data of the current node } //Otherwise recursively traverse to thte next node and get the element at the appropriate index return getRecursive(current.next, index - 1); } @Override public int indexOf(T x) throws NullPointerException { if (x == null) { throw new NullPointerException("Element cannot be null"); } return indexOfRecursive(head, x, 0); //Find the index of the first occurence of the elements recursively } //Recursive helper method to find the index of the first occurance of the element private int indexOfRecursive(Node current, T x, int index) { if (current == null) { return -1; //If the current node is null the element is not present } if (current.data.equals(x)) { return index; //If the current node contains the element return its index } //Otherwise, recursively check in the next node return indexOfRecursive(current.next, x, index + 1); } @Override public boolean isEmpty() { return size == 0; //Return true if the size is 0 } @Override public T remove(int index) throws IndexOutOfBoundsException { //checkIndex(index); //Check if index is valid if (index == 0) { return removeFirst(); //If index is 0 remove the first element and return it } else { T removedElement = removeRecursive(head, index - 1); //Remove the element at the given index recursively size--; return removedElement; } } //Recursive helper method to remove the element at the given index private T removeRecursive(Node current, int index) { if (index == 0) { Node removedNode = current.next; current.next = removedNode.next; return removedNode.data; //If index is 0 remove the node and return its data } //Otherwise, Recursively reaverse to the next node and remove the element at the appropriate index return removeRecursive(current.next, index - 1); } @Override public boolean remove(T x) throws NullPointerException { if (x == null) { throw new NullPointerException("Element cannot be null"); } if (head == null) { return false; // If the list is empty, the element cannot be removed } if (head.data.equals(x)) { removeFirst(); //If the head contains the element remove the head return true; } //Otherwise recursively check for the element and remove it if it is found return removeRecursive(head, x); } //Recursive helper method to remove the first occurrence of the element private boolean removeRecursive(Node current, T x) { if (current.next == null) { return false; //if the next node is nll the element is not present } if (current.next.data.equals(x)) { Node removedNode = current.next; current.next = removedNode.next; removedNode.next = null; //Remove the next node and update the links return true; } //Otherwise recursively check in the next node return removeRecursive(current.next, x); } @Override public void set(int index, T x) throws IndexOutOfBoundsException, NullPointerException { checkIndex(index); //Check if index is valid if (x == null ) { throw new NullPointerException("Element cannot be null"); } setRecursive(head, index, x); //Set the element at the given index recursively } //Recursive helper method to set the element at the given index private void setRecursive(Node current, int index, T x) { if (index == 0) { current.data = x; //If index is 0 update the data of the current node } else { //Otherwise recursively traverse to the next node and set the element at the appropriate index setRecursive(current.next, index - 1, x); } } @Override public int size() { return size; //return the number of elements in the linked list } @Override public Object[] toArray() { Object[] array = new Object[size]; //Array to hold all the elements toArrayRecursive(head, array, 0); //Convert the linked list to the array recursively return array; } //Recursive helper method to convert the linked list to an array private void toArrayRecursive(Node current, Object[] array, int index) { if (current == null) { return; //if the current node is null stop recursion } array[index] = current.data; //Add the data of the current node to the array toArrayRecursive(current.next, array, index + 1); //Recursively continue to the next node. } private void addFirst(T x) { Node newNode = new Node<>(x); newNode.next = head; head = newNode; size++; } private T removeFirst() { if (head == null) { throw new IndexOutOfBoundsException("List is empty"); } T removedElement = head.data; head = head.next; size--; return removedElement; } private Node getNode(int index) { Node current = head; for (int i = 0; i < index; i++) { current = current.next; } return current; } private void checkIndex(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException("Invalid index"); } } @Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append("["); toStringRecursive(head, sb); sb.append("]"); return sb.toString(); } private void toStringRecursive(Node current, StringBuilder sb) { if (current == null) { return; } sb.append(current.data); if (current.next != null) { sb.append(", "); } toStringRecursive(current.next, sb); } }
NEXT THE TEST CLASS I ATTEMPTED
package edu.unomaha.csci3320.interfaces; public class LLTestClass { private Sequence linkedList; public LLTestClass() { linkedList = new LinkedList<>(); // Initialize a new LinkedList before each test method } // Test adding elements and size with a large number of elements public void testAddAndSize() { assertTrue(linkedList.isEmpty()); // Assert that the list is empty initially assertEquals(0, linkedList.size()); // Assert that the list size is 0 initially for (int i = 0; i < 100000; i++) { assertTrue(linkedList.add(i)); // Add elements from 0 to 99999 assertEquals(i + 1, linkedList.size()); // Assert that the list size is increasing correctly } } // Test adding elements and getting them back with a large number of elements public void testAddAndGet() { for (int i = 0; i < 100000; i++) { assertTrue(linkedList.add(i)); // Add elements from 0 to 99999 } for (int i = 0; i < 100000; i++) { assertEquals(Integer.valueOf(i), linkedList.get(i)); // Get elements and assert their values } } // Test removing elements and checking containment with a large number of elements public void testRemoveAndContains() { for (int i = 0; i < 100000; i++) { assertTrue(linkedList.add(i)); // Add elements from 0 to 99999 } for (int i = 0; i < 100000; i++) { assertTrue(linkedList.contains(i)); // Check that the elements are contained in the list //assertTrue(linkedList.remove(i)); // Remove elements and assert that they are removed successfully assertFalse(linkedList.contains(i)); // Assert that the elements are no longer contained in the list } assertTrue(linkedList.isEmpty()); // Assert that the list is empty after removing all elements } // Test clearing the list with a large number of elements public void testClear() { for (int i = 0; i < 100000; i++) { assertTrue(linkedList.add(i)); // Add elements from 0 to 99999 } assertFalse(linkedList.isEmpty()); // Assert that the list is not empty before clearing linkedList.clear(); // Clear the list assertTrue(linkedList.isEmpty()); // Assert that the list is empty after clearing assertEquals(0, linkedList.size()); // Assert that the list size is 0 after clearing } // Custom assertTrue method to throw AssertionError if the condition is false private void assertTrue(boolean condition) { if (!condition) { throw new AssertionError("Expected true, but got false."); } } // Custom assertFalse method to throw AssertionError if the condition is true private void assertFalse(boolean condition) { if (condition) { throw new AssertionError("Expected false, but got true."); } } // Custom assertEquals method to throw AssertionError if the values are not equal private void assertEquals(int expected, int actual) { if (expected != actual) { throw new AssertionError("Expected: " + expected + ", but got: " + actual); } } // Custom assertEquals method to throw AssertionError if the Integer values are not equal private void assertEquals(Integer expected, Integer actual) { if (!expected.equals(actual)) { throw new AssertionError("Expected: " + expected + ", but got: " + actual); } } // Main method to run the tests public static void main(String[] args) { LLTestClass test = new LLTestClass(); test.testAddAndSize(); test.testAddAndGet(); test.testRemoveAndContains(); test.testClear(); System.out.println("All tests passed for the worst-case scenario."); } }
The output I am getting is as follows: Exception in thread "main" java.lang.AssertionError: Expected: 1, but got: 2 at edu.unomaha.csci3320.interfaces.LLTestClass.assertEquals(LLTestClass.java:79) at edu.unomaha.csci3320.interfaces.LLTestClass.testAddAndSize(LLTestClass.java:18) at edu.unomaha.csci3320.interfaces.LLTestClass.main(LLTestClass.java:93)
Process finished with exit code 1
Had surgery in the middle of this program but I have fall term starting soon with two different new courses I will need these skills to be (at minimum) functional for. Therefore, I need to understand this better, so if anyone can help me figure out the issue here with a brief explanation (I am obviously not BRAND new to coding but I am new to projects like this and joined this to try and get a leg up before fall but I don't really understand so it would mean a lot, if its not overly complicated, if I could get a brief explanation as to WHY I need to change the things I do. Thanks so much for your time. Additionally if you notice any other major errors functionally that I haven't found yet that would be great. I really appreciate the insight. Sorry for such a long post Ive just been stuck for literally hours and have another entire project to get through. Thanks so much for your time :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
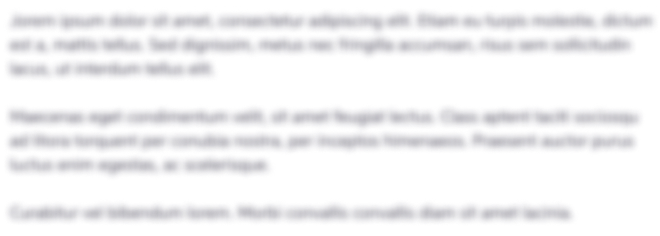
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started