Question
Hi, i have a hard time on useing JavaFx. How can i save the first and last name on a combobox like the examples in
Hi, i have a hard time on useing JavaFx. How can i save the first and last name on a combobox like the examples in image. And also other button
here is my code
package bsnContactGUI;
import java.io.File;
import java.nio.file.Path;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.control.MenuItem;
import javafx.scene.control.TextField;
import javafx.scene.image.ImageView;
import javafx.stage.Stage;
public class BusConController {
public static JFrame frame;
private String txtFieldValue;
private String fileName;
private boolean fileSaved;
static ComboBox comboBox = new ComboBox();
boolean valid;
@FXML
private MenuItem mOpen;
@FXML
private MenuItem mSave;
@FXML
private MenuItem mSaveAs;
@FXML
private MenuItem mExit;
@FXML
private MenuItem mAbout;
@FXML
private Label lbContacts;
@FXML
private Label lbFirstName;
@FXML
private Label lbCompanyS;
@FXML
private Label lbPhone;
@FXML
private Label lbLastName;
@FXML
private Label lbeMail;
@FXML
private Button btnNew;
@FXML
private Button btnAdd;
@FXML
private Button btnUpdate;
@FXML
private Button btnDelet;
@FXML
private ImageView imageView;
@FXML
private TextField txtLastName;
@FXML
private TextField txtEMail;
@FXML
private TextField txtCompany;
@FXML
private TextField txtFirstName;
@FXML
private TextField txtPhone;
@FXML
void btnAdd(ActionEvent event) {
// create nexw client
ContactRecord contact = new ContactRecord(txtFirstName.getText(), txtLastName.getText(), txtPhone.getText(),
txtEMail.getText(), txtCompany.getText());
updateClientFields(contact);
// add the client to contact list
ContactRecordList.cList.add(contact);
// add client to combobox
comboBox.getItems().add(txtFieldValue);
// update selected item in combobox
// comboBox.sets(0);
// update button state
setDefaultButtonState();
}
@FXML
void btnDelet(ActionEvent event) {
if (JOptionPane.showConfirmDialog(frame, String.format("Delete %s?", comboBox.getValue()), "Delete",
JOptionPane.CANCEL_OPTION, JOptionPane.WARNING_MESSAGE) == JOptionPane.YES_OPTION) {
int idx = comboBox.getSelectionModel().getSelectedIndex();
comboBox.getSelectionModel().clearSelection(idx);
ContactRecordList.cList.remove(idx);
}
}
@FXML
void btnNew(ActionEvent event) {
clearTextFields();
setNewButtonState();
txtFirstName.requestFocus();
}
@FXML
void btnUpdate(ActionEvent event) {
int idx = comboBox.getSelectionModel().getSelectedIndex();
ContactRecord contact = ContactRecordList.cList.get(idx);
updateClientFields(contact);
// update the combo box
comboBox.getItems().add(idx, contact.getFLName());
comboBox.getItems().remove(idx + 1);
comboBox.getSelectionModel().select(idx);
}
@FXML
void comboBox(ActionEvent event) {
updateTextFields(comboBox.getSelectionModel().getSelectedIndex());
setDefaultButtonState();
}
@FXML
void mAboutAction(ActionEvent event) {
try {
Parent aboutWindow = (Parent) FXMLLoader.load(getClass().getResource("About.fxml"));
Scene scene = new Scene(aboutWindow);
Stage stage = new Stage();
stage.setTitle("About");
// stage.getIcons().add(new Image(new FileInputStream("images/slcclogo.png")));
stage.setScene(scene);
stage.show();
} catch (Exception e) {
System.out.println("Can't open Help window.");
}
}
@FXML
void mExitAction(ActionEvent event) {
System.exit(0);
}
@FXML
void mOpenAction(ActionEvent event) {
JFileChooser fileChooser = new JFileChooser();
fileChooser.setCurrentDirectory(new File("."));
int result = fileChooser.showOpenDialog(frame);
// if user clicked Cancel button on dialog, return
if (result == JFileChooser.CANCEL_OPTION)
return;// System.exit(1);
// return Path representing the selected file
Path filePath = fileChooser.getSelectedFile().toPath();
fileName = filePath.toString();
// clear comboBox and list
if (!ContactRecordList.cList.isEmpty()) {
// comboBox.setSelectionModel(new saComboBoxModel());
ContactRecordList.cList.clear();
}
// read the file and load the comboBox
if (ContactRecordList.readContactFile(fileName)) {
loadContactComboBox();
setDefaultButtonState();
fileSaved = true;
} else {// no records read - set buttons
setNewButtonState();
}
}
@FXML
void mSaveAction(ActionEvent event) {
if (ContactRecordList.cList.size() == 0) {
JOptionPane.showMessageDialog(frame, "There are not contacts to save!", "Woops...",
JOptionPane.ERROR_MESSAGE);
return;
}
if (!fileSaved) {
openFileSaverDialog();
}
ContactRecordList.writeContactFile(fileName);
}
@FXML
void mSaveAsAction(ActionEvent event) {
if (ContactRecordList.cList.size() == 0) {
JOptionPane.showMessageDialog(frame, "There are not contacts to save!", "Woops...",
JOptionPane.ERROR_MESSAGE);
return;
}
if (openFileSaverDialog())
ContactRecordList.writeContactFile(fileName);
}
@FXML
void txtCompany(ActionEvent event) {
BusConController.this.txtFieldValue = BusConController.this.txtCompany.getText();
BusConController.this.valid = BC_Validate.isValidCompanyName(BusConController.this.txtFieldValue);
if (!BusConController.this.valid) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Error");
alert.setHeaderText("");
String s = "Invalid Company Name";
alert.setContentText(s);
alert.show();
} else {
// BusConController.this.txtEMail.requestFocus();
}
}
@FXML
void txtEmail(ActionEvent event) {
BusConController.this.txtFieldValue = BusConController.this.txtEMail.getText();
BusConController.this.valid = BC_Validate.isValidEmail(BusConController.this.txtFieldValue);
if (!BusConController.this.valid) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Error");
alert.setHeaderText("");
String s = "Invalid Email";
alert.setContentText(s);
alert.show();
} else {
BusConController.this.txtCompany.requestFocus();
}
}
@FXML
void txtFirstName(ActionEvent event) {
BusConController.this.txtFieldValue = BusConController.this.txtFirstName.getText();
BusConController.this.valid = BC_Validate.isValidFirstName(BusConController.this.txtFieldValue);
if (!BusConController.this.valid) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Error");
alert.setHeaderText("");
String s = "Invalid First Name";
alert.setContentText(s);
alert.show();
} else {
BusConController.this.txtLastName.requestFocus();
}
}
@FXML
void txtLastName(ActionEvent event) {
BusConController.this.txtFieldValue = BusConController.this.txtLastName.getText();
BusConController.this.valid = BC_Validate.isValidLastName(BusConController.this.txtFieldValue);
if (!BusConController.this.valid) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Error");
alert.setHeaderText("");
String s = "Invalid Last Name";
alert.setContentText(s);
alert.show();
} else {
BusConController.this.txtPhone.requestFocus();
}
}
@FXML
void txtPhone(ActionEvent event) {
BusConController.this.txtFieldValue = BusConController.this.txtPhone.getText();
BusConController.this.valid = BC_Validate.isValidPhone(BusConController.this.txtFieldValue);
if (!BusConController.this.valid) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Error");
alert.setHeaderText("");
String s = "Invalid Phone Number";
alert.setContentText(s);
alert.show();
} else {
BusConController.this.txtEMail.requestFocus();
}
}
void clearTextFields() {
txtFirstName.setText("");
txtLastName.setText("");
txtPhone.setText("");
txtEMail.setText("");
txtCompany.setText("");
}
private boolean openFileSaverDialog() {
JFileChooser fileChooser = new JFileChooser();
fileChooser.setCurrentDirectory(new File("."));
int result = fileChooser.showSaveDialog(null);
// if user clicked Cancel button on dialog, return
if (result == JFileChooser.CANCEL_OPTION)
return false;// System.exit(1);
// return Path representing the selected file
fileName = fileChooser.getSelectedFile().getName();
return true;
}
void loadContactComboBox() {
for (ContactRecord item : ContactRecordList.cList) {
comboBox.getItems().add(item.getFLName());
// System.out.println(item);
}
comboBox.getSelectionModel().select(0);
updateTextFields(0);
}
void setNewButtonState() { // if file not found/loaded | new button clicked
btnNew.setDisable(false);
btnAdd.setDisable(false);
btnUpdate.setDisable(true);
btnDelet.setDisable(true);
}
void setDefaultButtonState() {// if file loaded successfully | new contact added
btnNew.setDisable(false);
btnAdd.setDisable(true);
btnUpdate.setDisable(false);
btnDelet.setDisable(false);
}
void updateClientFields(ContactRecord contact) {
contact.setFirstName(txtFirstName.getText());
contact.setLastName(txtLastName.getText());
contact.setPhoneNumber(txtPhone.getText());
contact.setEmailAddress(txtEMail.getText());
contact.setCompany(txtCompany.getText());
}
void updateTextFields(int selectedIndex) {
ContactRecord contact = ContactRecordList.cList.get(selectedIndex);
txtFirstName.setText(contact.getFirstName());
txtLastName.setText(contact.getLastName());
txtPhone.setText(contact.getPhoneNumber());
txtEMail.setText(contact.getEmailAddress());
txtCompany.setText(contact.getCompany());
}
}
package bsnContactGUI;
import java.io.FileInputStream;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.stage.Stage;
public class BusConRun
extends Application
{
public void start(Stage stage)
throws Exception
{
Parent root =
(Parent)FXMLLoader.load(getClass().getResource("BusCon.fxml"));
Scene scene = new Scene(root);
stage.setTitle("");
//stage.getIcons().add(new Image(new FileInputStream("images/slcclogo.png")));
stage.setScene(scene);
stage.show();
}
public static void main(String[] args)
{
launch(args);
}
}
package bsnContactGUI;
public class ContactRecordApp
{
public static void main(String [] args)
{
ContactRecordList contactList = new ContactRecordList();
contactList.readContactFile("contacts.ser");
contactList.processContactFile();
contactList.writeContactFile("contacts.ser");
}//end main
}//end class ContactRecordTest
about.fxml
"1.0" encoding="UTF-8"?>
"200.0" prefWidth="300.0" xmlns="http://javafx.com/javafx/10.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="bsnContactGUI.BusConController">
"133.0" layoutY="14.0" text="About" />
"51.0" layoutY="40.0" prefHeight="128.0" prefWidth="198.0" text="Test About" />
"34.0" fitWidth="56.0" layoutX="18.0" layoutY="5.0" pickOnBounds="true" preserveRatio="true" />
BusCon.fxml
"1.0" encoding="UTF-8"?>
"350.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/10.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="bsnContactGUI.BusConController">
"36.0" prefWidth="600.0">
"false" text="File">
"mOpen" mnemonicParsing="false" onAction="#mOpenAction" text="Open" />
"mSave" mnemonicParsing="false" onAction="#mSaveAction" text="Save" />
"mSaveAs" mnemonicParsing="false" onAction="#mSaveAsAction" text="Save As..." />
"mExit" mnemonicParsing="false" onAction="#mExitAction" text="Exit" />
Step by Step Solution
There are 3 Steps involved in it
Step: 1
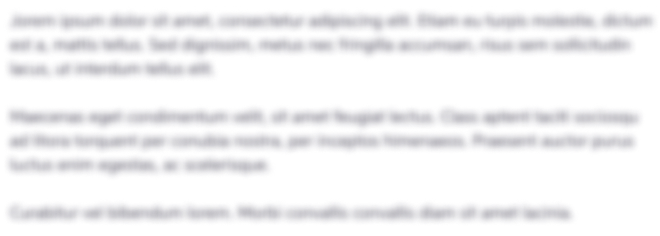
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started