Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Hi I need help in Java. My code has to display (and apply) an overdraft fee when there is no money in the account. Here
Hi I need help in Java.
My code has to display (and apply) an overdraft fee when there is no money in the account. Here is all my code, please help me figure this out.
Account.java
public class Account { private static int counter = 1000; private int accountNumber; private double balance; //constructor public Account() { counter++; this.accountNumber = counter; this.balance = 0; } //Overload Constructor public Account(double balance) { this.balance = balance; counter++; this.accountNumber = counter; } //getters public int getAccountNumber() { return accountNumber; } public double getBalance() { return balance; } //setter public void setBalance(double balance) { this.balance = balance; } public void withdraw(double amount) { balance -= amount; } public void deposit(double amount) { balance += amount; } @Override public String toString() { return getClass().getSimpleName()+"{" + "AccountNumber: " + accountNumber + ", Balance: " + String.format("$%.2f",balance) + '}'; } }
Checking.java
public class Checking extends Account{ private static final double OVERDRAFT_FEE = 20.00; public Checking(double balance) { super(balance); } public Checking() { super(); } @Override public void withdraw(double amount) { if(getBalance()<1) { setBalance(getBalance()-OVERDRAFT_FEE); System.out.println("Charging an overdraft fee of $"+OVERDRAFT_FEE+" because account is below $0"); } super.withdraw(amount); } }
Savings.java
public class Savings extends Account{ private static final double FEE= 10.00, INTEREST = 0.015; private int counter =0; public Savings() { super(); } public Savings(double balance) { super(balance); } @Override public void withdraw(double amount) { if(getBalance()<500.00) { System.out.println("Charging a fee of $"+FEE+" because you are below $500"); setBalance(getBalance()-FEE); } super.withdraw(amount); } @Override public void deposit(double amount) { counter++; System.out.println("This is deposit "+counter+" to this account."); if(counter>5) { System.out.println("Charging a Fee of $"+FEE); setBalance(getBalance()-FEE); } super.deposit(amount); } public void interest() { double interest = getBalance()*INTEREST; System.out.println("Customer earned $"+String.format("%.2f",interest)+" in interest"); //add the interest to balance setBalance(getBalance()+interest); } }
Driver.java
import java.util.Scanner; public class Driver { public static void main(String[] args) { //Create a Checking Account Checking checking = new Checking(0.0); Savings savings = new Savings(0.0); int choice; Scanner input = new Scanner(System.in); double amount; do { choice = menu(); System.out.println(); switch (choice) { case 1: System.out.print("How much would you like to withdraw from Checking?"); amount = input.nextDouble(); checking.withdraw(amount); break; case 2: System.out.print("How much would you like to withdraw from Savings?"); amount = input.nextDouble(); savings.withdraw(amount); break; case 3: System.out.print("How much would you like to deposit into Checking?"); amount = input.nextDouble(); checking.deposit(amount); System.out.println("Doing default deposit"); break; case 4: System.out.print("How much would you like to deposit into Savings?"); amount = input.nextDouble(); savings.deposit(amount); System.out.println("This is deposit number " + "to this account"); break; case 5: //System.out.println("Your balance for checking 10001 is " + checking); System.out.printf("Your balance for Checking 10001 is $%.2f%n",checking.getBalance()); break; case 6: //System.out.println(savings); System.out.printf("Your balance for Savings 10002 is $%.2f%n",savings.getBalance()); break; case 7: savings.interest(); break; case 8: System.out.println("Thanks for using this app."); break; default: System.out.println("Wrong choice...Try Again."); break; } }while (choice!=8); } public static int menu() { System.out.print("1. Withdraw from Checking 2. Withdraw from Savings 3. Deposit to Checking" + " 4. Deposit to Savings 5. Balance Of checking 6. Balance of savings" + " 7. Award Interest to savings now 8. Quit Enter your choice: "); Scanner input = new Scanner(System.in); return input.nextInt(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
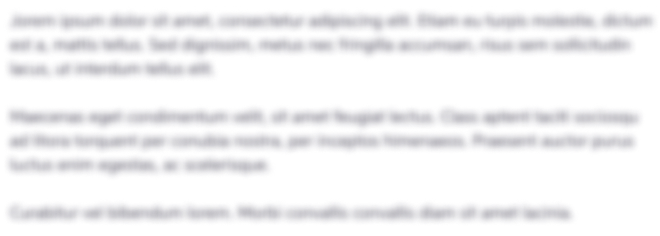
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started