Question
Hi, I would like to code something that shifts the Caesar Cipher by the amount K. A Caesar Cipher is an encrypting style that shifts
Hi,
I would like to code something that shifts the Caesar Cipher by the amount K.
A Caesar Cipher is an encrypting style that shifts an entire messages' letters by three spaces.
For example,
ABC would be CDE in the Caesar shift.
I would like to code something that would be able to shift the entire Caesar shift by the amount K, which is imputted by the user.
Please help me out.
This is the current code that I have:
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; import java.util.Scanner;
public class CaesarCipher { public static void main(String[] args) throws FileNotFoundException { final int DEFAULT_KEY = 3; int key = DEFAULT_KEY; String inFile = ""; String outFile = ""; int files = 0;
for (int i = 0; i < args.length; i++) { String arg = args[i]; if (arg.charAt(0) == '-') } char option = arg.charAt(1); if (option == 'd') { key = -key; } else { usage(); return; } } else { files++; if (files == 1) { inFile = arg; } else if (files == 2) { outFile = arg; } } } if (files != 2) { usage(); return; }
Scanner in = new Scanner(new File(inFile)); in.useDelimiter(""); PrintWriter out = new PrintWriter(outFile);
while (in.hasNext()) { char from = in.next().charAt(0); char to = encrypt(from, key); out.print(to); } in.close(); out.close(); }
/** Encrypts upper- and lowercase characters by shifting them according to a key. @param ch the letter to be encrypted @param key the encryption key @return the encrypted letter */ public static char encrypt(char ch, int key) { int base = 0; if ('A' <= ch && ch <= 'Z') { base = 'A'; } else if ('a' <= ch && ch <= 'z') { base = 'a'; } else { return ch; } int offset = ch - base + key; final int LETTERS = 26; if (offset > LETTERS) { offset = offset - LETTERS; } else if (offset < 0) { offset = offset + LETTERS; } return (char) (base + offset); }
/** Prints a message describing proper usage. */ public static void usage() { System.out.println("Usage: java CaesarCipher [-d] infile outfile"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
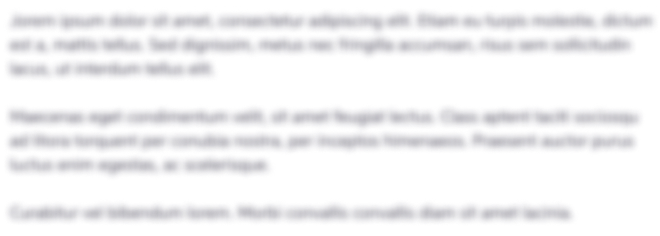
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started