Question
Hi if someone could help me with this Python program dealing with a Graph depth-first search with a stack, I would greatly appreciate it very
Hi if someone could help me with this Python program dealing with a Graph depth-first search with a stack, I would greatly appreciate it very much!
Using Python, you will write a program that implements the Algorithm below:
StackDFS(G, node) > visited
Input: G = (V, E), a graph
node, the starting vertex in G
Output: visited, an array of size |V| such that visited|i| is TRUE if we have visited node i, FALSE otherwise
S < CreateStack()
visited < CreateArray(|V|)
for i < 0 to |V| do
visited|i| < FALSE
Push(S, node)
while not IsStackEmpty(S) do
c < Pop(s)
visited|c| < TRUE
foreach v in AdjacencyList(G, c) do
if not visited|v| then
Push(S, v)
return visited
- Use an adjacency matrix (i.e., a two-dimensional array, or, in Python, a list of lists).
- You may use ANY list method you wish (e.g., append, pop, etc.).
IMPLEMENTATION DETAILS:
- Your program should begin by prompting the user for the number of vertices, V, in the graph, G.
- Your program will represent the graph G using an adjacency matrix, which is a square matrix with a row and a column for each vertex. Thus, your program will need to create a matrix M that consists of a V x Vtwo-dimensional array -- in Python, a list of lists. (I recommend that your program initialize each element of the matrix equal to zero.)
- Next, your program should prompt the user to indicate which elements in the matrix should be assigned the value of 1 (i.e., information about vertices). Recall that each element in the matrix is the intersection of a row and a column.
- The result of step 4 should be an adjacency matrix representation of a graph, G.
- At this point, your program should print the newly-created adjacency matrix on the screen.
- Next, your program should prompt the user to specify node -- i.e., the starting vertex in G.
- From here, you then proceed with the implementation of Algorithm 2.3, with the following enhancements:
- Be sure to use the newly-created adjacency matrix, instead of an adjacency list.
- Immediately following line 5 (but before line 6) of Algorithm 2.3, your program should print the values currently on the stack.
- At the end of the while block, your program should print the values currently on the stack.
In effect, the result of step 8 above should be a display of the stack evolution for your implementation of the DFS algorithm on graph G.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
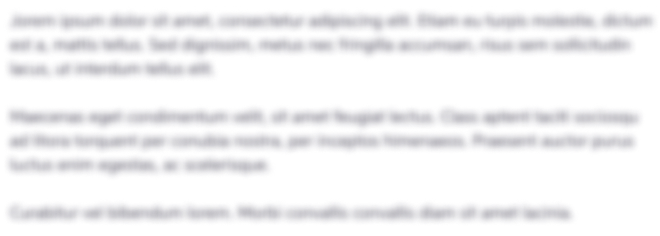
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started