Question
Hi I'm stuck in this question, Can you rectify where I have gone wrong Task 1 In the file go.py, implement a class Board representing
Hi I'm stuck in this question, Can you rectify where I have gone wrong Task 1 In the file go.py, implement a class Board representing a square Go board. The constructor should take an optional integer parameter size with a default value of 19 to indicate the size of the board. The size must be between 2 and 26, inclusive. If this is not the case, raise an AssertionError with the error message "Illegal board size: must be between 2 and 26.". The constructor should create a square board of the given size with all points initialised as empty. Implement the method get_size(self) that returns the size of the board. Implement the method __str__(self) to convert a Board to a string that when printed gives an "ASCII art" representation of the board with coordinates marked as letters along the columns and rows. Click on the "Expand" button below for an example. Use the following characters to represent the points on the board empty: "." black: "@" white: "O" Task 2 Implement a method set_colour(self, coords, colour_name) that changes the colour at the coordinates given by coords. coords must be a string of two lower case letters where the first letter specifies the column and the second letter specifies the row. colour_name must be one of the strings "empty", "black", "white". If coords is not a string of length 2, raise an AssertionError with the string "invalid coordinates". If the letter for column or row are out of range, raise an AssertionError with the message "column out of range" or "row out of range", respectively. If the colour name is invalid, raise an AssertionError with the message "invalid colour name". Implement a method get_colour(self, corrds) that returns the colour of the point at the given coordinates. Coordinates and colour names are as described above. Check for valid coordinates as above. Task 3 Extend the constructor of class Board to accept an additional optional argument from_strings. If present, this argument must be a list of strings where each string represents a row of the board with the character encoding used in the __str__() method, but without the intervening spaces and the coordinate letters. For example, for a board of size 19, from_strings must be a list of 19 strings, each having exactly 19 characters from the set ".@O". Check the validity of from_strings and raise AssertionErrors with the following messages for invalid inputs (the letter x should indicate the row coordinate of the invalid row): "input is not a list" "length of input list does not match size" "row x is not a string" "length of row x does not match size" "invalid character in row x" Implement a method to_strings(self) that returns a representation of the board as a list of strings in the same format as that accepted by the __init__() method. Task 4 Outside the class, define a function load_board(filename) that takes as an argument the name of a text file, reads a Go position from that file and returns a Board object representing the position. The file must contain one line for each row of the board, using the characters ".", "@", and "O" as above to represent the colours. Define a function save_board(filename, board) that takes as arguments a filename and a Board object and saves the position to a text file in the same format as that accepted by load_board(). Task 5 In class Board, define two new methods: to_integer(self) returns an integer encoding of the position set_from_integer(self, integer_encoding) sets the colours of all points of the board according to the given integer_encoding. This doesnt change the size of the board! The integer encoding of a board position is based on the ternary number system. The ternary system uses the digits 00, 11, and 22 to represent numbers as follows. If d_0, d_1, \ldots, d_n \in \{0, 1, 2\}d0,d1,,dn{0,1,2} are ternary digits, then n = d_n \cdot 3^n + d_{n-1} \cdot 3^{n-1} + \cdots + d_0 \cdot 3^0n=dn3n+dn13n1++d030 is the integer represented by the sequence (d_n d_{n-1}\cdots d_0)_3(dndn1d0)3 of digits. For example, (21)_3 = 2\cdot 3 + 1 = 7(21)3=23+1=7 and (210)_3 = 2 \cdot 9 + 1 \cdot 3 + 0 = 21(210)3=29+13+0=21. If we replace the colours of the points of the Go board by digits (empty = 0, black = 1, white = 2) and concatenate all rows of the board to a single row of digits, we get a ternary number that can then be converted to an integer. The method to_integer(self) is supposed to do exactly that. For example, the 3 \times 333 board used in the example for Task 2 looked like this: a b c a O . O b . @ . c @ O . Reading this off as ternary digits gives us (202010120)_3(202010120)3, which equals 14676 in decimal. Hint: To convert an integer nn to ternary representation, as required by set_from_integer(), note that you get the last digit by computing n \bmod 3nmod3. You get the next digit by computing \lfloor n/3 floor \bmod 3n/3mod3 and so on. For example, heres how to get the last two digits of the ternary representation of 14676: 14676 \bmod 3 = \textbf{0}14676mod3=0. 14676/3 = 489214676/3=4892. 4892 \bmod 3 = \textbf{2}4892mod3=2. def load_board(filename): result = " " with open(filename) as f: print(f) for index, line in enumerate(f): if index == 0: result += ' '+' '.join([chr(alphabets + 65) for alphabets in range(len(line) - 1)]) + ' ' # the alphabetical column heading result += f"{19 - index:2d}" result += ' ' + ' '.join(line.strip()) + ' ' print(type(result)) result=list(result) print(type(result)) size=len(result) #return (result) print(len(result)) return Board(from_strings = result) # # file=open(filename, "r") # lst = [] # for line in file: # lst.append(line) # for i in range(len(lst)): # lst[i] = lst[i][:len(lst[i]) - 1] # Board.size=len(lst) # return Board(from_strings=lst) def save_board(filename, board): with open(filename, "wt") as f: f.write(board) from string import ascii_uppercase as letters class Board: #Dictionary created for the colours and the respected symbols points = {'E': '.', 'B': '@', 'W': 'O'} #Constructor def __init__(self,size=19,from_strings=None): assert 2 <= size <= 26, "Illegal board size: must be between 2 and 26." if from_strings != None: if type(from_strings) != list: raise AssertionError("input is not a list") if len(from_strings) != size: raise AssertionError("length of input list does not match size") for i in range(size): if type(from_strings[i]) != str: raise AssertionError("row " + str(i) + " is not a string") if len(from_strings[i]) != size: raise AssertionError("length of row " + str(i) + " does not match size") for j in range(size): if from_strings[i][j] not in ".@O": raise AssertionError("invalid character in row " + str(i)) self.size = size self.grid = [['E'] * size for _ in range(size)] self.from_strings = [] if from_strings is None else from_strings def get_size(self): #Returns the size of the grid created by the constructor return self.size def __str__(self): # creating the grid padding = ' ' # Creating a variable with a space assigned so that it acts as a padding to the rows that have a single digit heading = ' ' + ' '.join(letters[:self.size]) # Alphabetical heading is created lines = [heading] # adding the alphabetical heading into a list named lines to which the rows will be added later for r, row in enumerate(self.grid): if len(self.grid) < 10: # for the grid with a size less than 10 to add the space to the start of the row for the single digits to be aligned if (self.from_strings): line = " " + f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = " " + f'{self.size - r} ' + ' '.join(self.points[x] for x in row) lines.append(line) else: # for the grids that are larger than 9 if r > 9: # for rows 1 to 9 the single digits are aligned according to the first digit from the right of the two digit rows if (self.from_strings): line = f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = f'{self.size - r} ' + ' '.join(self.points[x] for x in row) line = padding + line # adding the space using the variable padding to the row created lines.append(line) # adding the row to the list of rows else: # for the rows 10 onwards - as there is no requirement to add a padding it is not added here if (self.from_strings): line = f'{self.size - r} ' + ' '.join(self.from_strings[r]) else: line = f'{self.size - r} ' + ' '.join(self.points[x] for x in row) # creation of the row lines.append(line) # adding the newly created row to the list of rows return ' '.join(lines) def _to_row_and_column(self, coords): # destructure coordinates like "B2" to "B" and 2 alpha, num = coords colnum = ord(alpha) - ord('A') + 1 rownum = self.size - int(num) + 1 assert 1 <= rownum <= self.size,"row out of range" assert 1 <= colnum <= self.size,'column out of range' return rownum, colnum def set_colour(self, coords, colour_name): rownum, colnum = self._to_row_and_column(coords) assert len(coords)==2 or len(coords)==3, "invalid coordinates" assert colour_name in self.points,"invalid colour name" self.grid[rownum - 1][colnum - 1] = colour_name def get_colour(self, coords): rownum, colnum = self._to_row_and_column(coords) return self.grid[rownum - 1][colnum - 1] def to_strings(self): padding = ' ' lines = [] for r, row in enumerate(self.grid): if self.from_strings: lines.append(''.join(self.from_strings[r])) else: lines.append(''.join(self.points[x] for x in row)) return lines def to_integer(self): digit_colour="" for line in self.to_strings(): for character in line: if character=='.': character=0 elif character=='O': character=1 elif character=='@': character=2 character=str(character) digit_colour+=character return int(digit_colour,3) # return ''.join(self.to_int[x] for line in self.grid for x in line) def set_from_integer(self, integer_encoding): n = int(integer_encoding) list1 = [] p=[] m=[] t=[] while n != 0: rem = n % 3 list1.append(rem) n = n // 3 list1.reverse() list1=["." if item ==0 else item for item in list1] list1=["O" if item ==1 else item for item in list1] list1=["@" if item ==2 else item for item in list1] for i in range(0,len(list1),3): p.append(list1[i:i+3]) #print(p) for x in p: m=''.join(map(str,x)) t.append(m) #print(Board(self.size,t)) self.from_strings=t b = Board(5) print(b) print(b.get_size()) b = Board() print(b) b=Board(5) b.set_colour("B1", "W") print(b) b.set_colour("C1", "B") print(b) print(b.get_colour("C1")) b =Board(3, ["O.O", ".@.", "@O."]) b.set_colour("B1", "W") print(b) print(b.to_strings()) c =Board(b.get_size(), b.to_strings()) print(str(b) == str(c)) b = load_board("l19.txt") print(b) save_board("l19b.txt",b) print(b) b = Board(3, ["O.O", ".@.", "@O."]) print(b.to_integer()) l19 = load_board("l19.txt") print(l19.to_integer()) c = Board(3) c.set_from_integer(14676) print(c)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
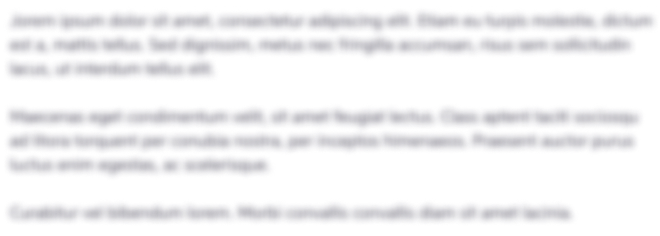
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started